-
- 前言
- 简介
-
- [演示 ⇒ (最简单的原理,开发并不这样,理解一下就好)](#演示 ⇒ (最简单的原理,开发并不这样,理解一下就好))
- [演示 ⇒ 接近真实注解开发(好好理解一下)](#演示 ⇒ 接近真实注解开发(好好理解一下))
- [Controller 讲解](#Controller 讲解)
- [RequestMapper ⇒ 没啥记的,第一个案例看看就行了](#RequestMapper ⇒ 没啥记的,第一个案例看看就行了)
- [RestFul 风格 ⇒ 简洁、有层次](#RestFul 风格 ⇒ 简洁、有层次)
- [重定向和转发 ⇒ 一般都用return,不是很建议用这两个方法。](#重定向和转发 ⇒ 一般都用return,不是很建议用这两个方法。)
-
- 写项目遇到的问题(return返回的都是路径,但是重定向路径呢?怎么写)
- [回显除了 model ,还有 modelMap](#回显除了 model ,还有 modelMap)
- [过滤器 Filter 、Filter乱码 web.xml 过滤器](#过滤器 Filter 、Filter乱码 web.xml 过滤器)
- 过滤器
- [Json 和 Jackson](#Json 和 Jackson)
-
- [@ResponseBody注解 (方法)/ @RestController(类)](#@ResponseBody注解 (方法)/ @RestController(类))
- [fastjson ⇒ 用于 java 和 json 进行转换 ⇒ 目前知道有这个功能,以后不会可以百度一下](#fastjson ⇒ 用于 java 和 json 进行转换 ⇒ 目前知道有这个功能,以后不会可以百度一下)
- Ajax
- 拦截器
-
- [User 和 Password 登录案例](#User 和 Password 登录案例)
- 文件上传下载(需要的时候,去复制粘贴哦)
前言
ssm: mybatis + Spring +SpringMVC ⇒ MVC 三层架构
模型(Dao、Service)、视图(Jsp)、控制器(Servlet)⇒ 转发或重定向
简介
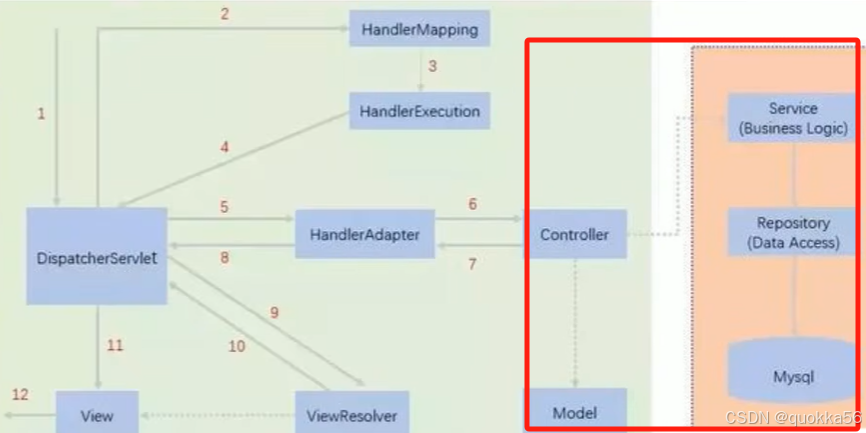
演示 ⇒ (最简单的原理,开发并不这样,理解一下就好)
演示 ⇒ 接近真实注解开发(好好理解一下)
重要的源码献上
web.xml:
<!DOCTYPE web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"
"http://java.sun.com/dtd/web-app_2_3.dtd" >
<web-app>
<display-name>Archetype Created Web Application</display-name>
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc-servlet.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
springmvc-servlet.xml:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
">
<!-- IOC注解支持 -->
<context:component-scan base-package="controller" />
<!-- 静态资源解析-->
<mvc:default-servlet-handler />
<!-- MVC注解支持 -->
<mvc:annotation-driven />
<!-- 配置内部视图解析器 -->
<!-- 视图路径:/WEB-INF/jsp/*.jsp -->
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/jsp/" />
<property name="suffix" value=".jsp" />
</bean>
<!-- 静态资源解析 -->
<mvc:resources location="/static/" mapping="/static/**" />
<!-- 全局异常处理 -->
<bean
class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver">
<property name="exceptionMappings">
<props>
<prop key="java.lang.RuntimeException">error</prop>
</props>
</property>
</bean>
</beans>
Controller 讲解
曾经的@兄弟 | 组件 |
---|---|
@Component | 组件 |
@Service | service |
@Controller | controller |
@Repository | dao |
RequestMapper ⇒ 没啥记的,第一个案例看看就行了
RestFul 风格 ⇒ 简洁、有层次
演示
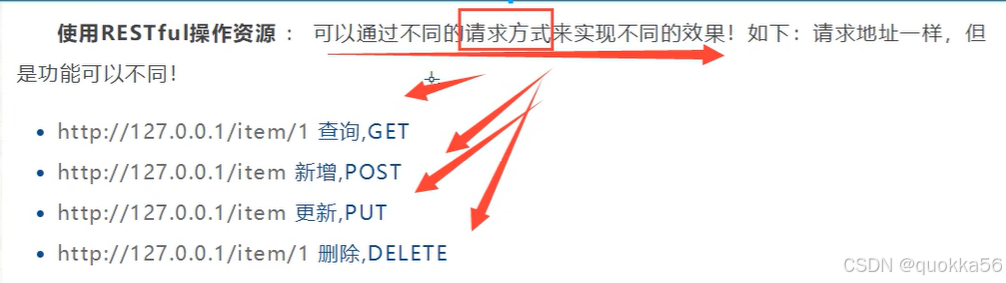
讲解

我们可以把利用这种方式,吧变量 绑定 在 url 地址栏中。
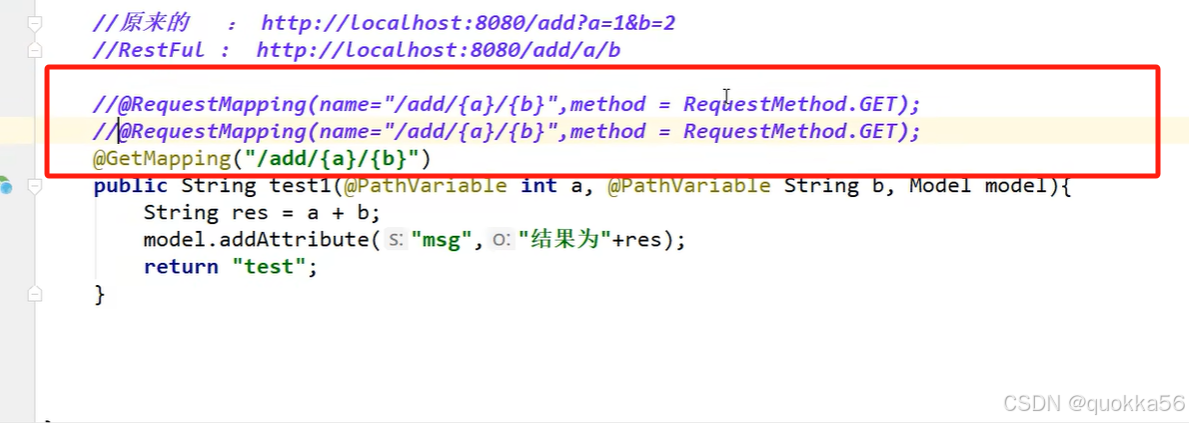
如上图,我们可以看到,目前有两种方法来限定提交的方式,但是尽量用 下面这种 哦 ~ ~。毕竟方便、快捷、准确率高嘛,而且还挺安全 ~ ~。
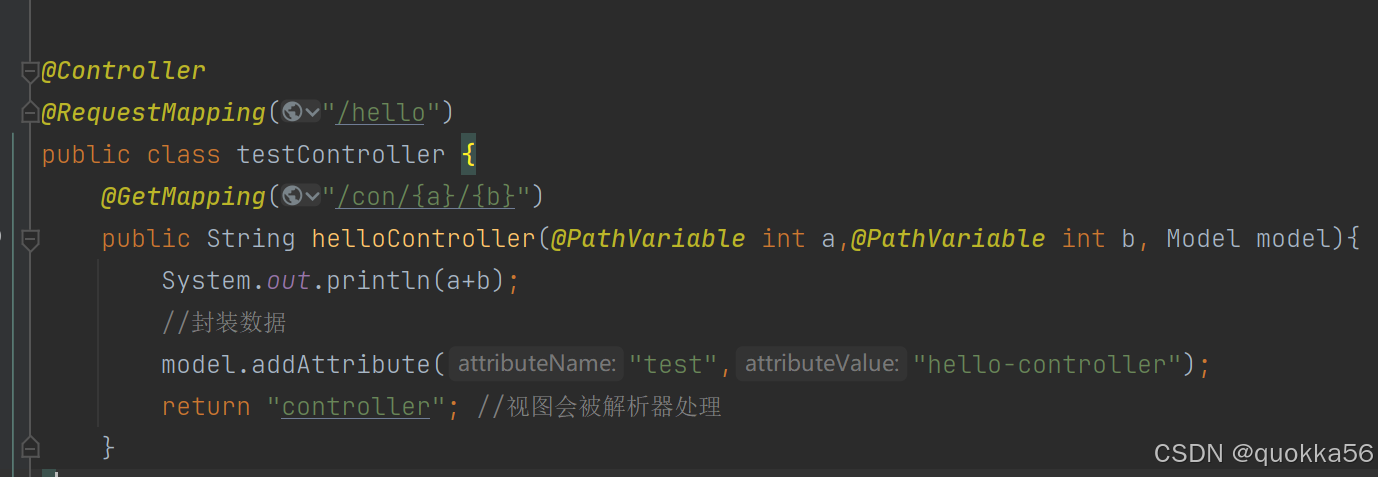
重定向和转发 ⇒ 一般都用return,不是很建议用这两个方法。
resp.sendRedirect("xx.jsp")
.
req.getRequestDispatcher("\xx.jsp").forword(req,resp);
.
public String test ( @RequestParam ( "userName" String name ) ) { xxx }
获取前端 的 参数:
写项目遇到的问题(return返回的都是路径,但是重定向路径呢?怎么写)
一般来说,return 返回的都是jsp页面,重定向可以使用以下方式去找路径
return
"redirect:/get";
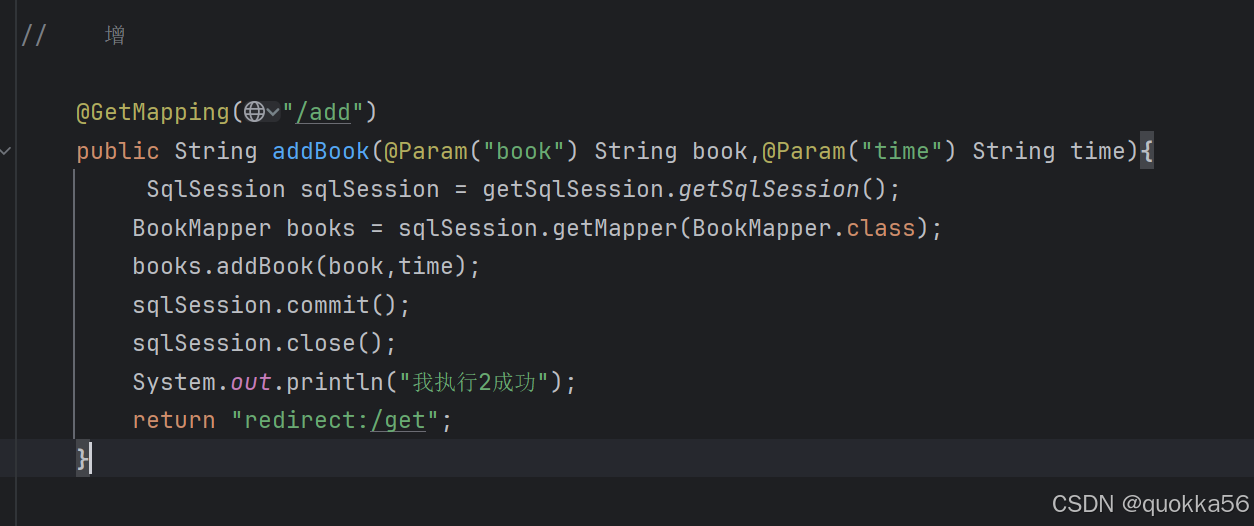
回显除了 model ,还有 modelMap
过滤器 Filter 、Filter乱码 web.xml 过滤器
过滤器
<filter>
<filter-name>encoding</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encoding</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Json 和 Jackson
是一种轻量级的数据交换格式,目前使用的特别特别特别广泛!
示例:'{"name":"xxx","age":15}'
@ResponseBody注解 (方法)/ @RestController(类)
会返回字符串,而不会走视图解析器,会直接return字符串。
fastjson ⇒ 用于 java 和 json 进行转换 ⇒ 目前知道有这个功能,以后不会可以百度一下
① 导包 ⇒ maven 中找
②使用。例如: xxx.json ,xxx.toJsonString()
示例:
报错细微问题讲解(有个报错,很重要,要小心)
我在导入 这个包的时候,添加了以上的解决乱码统一代码 的时候,有以下报错,我一直在排错。但是还是一直不能解决问题。
<!-- https://mvnrepository.com/artifact/com.alibaba/fastjson -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>2.0.49</version>
</dependency>
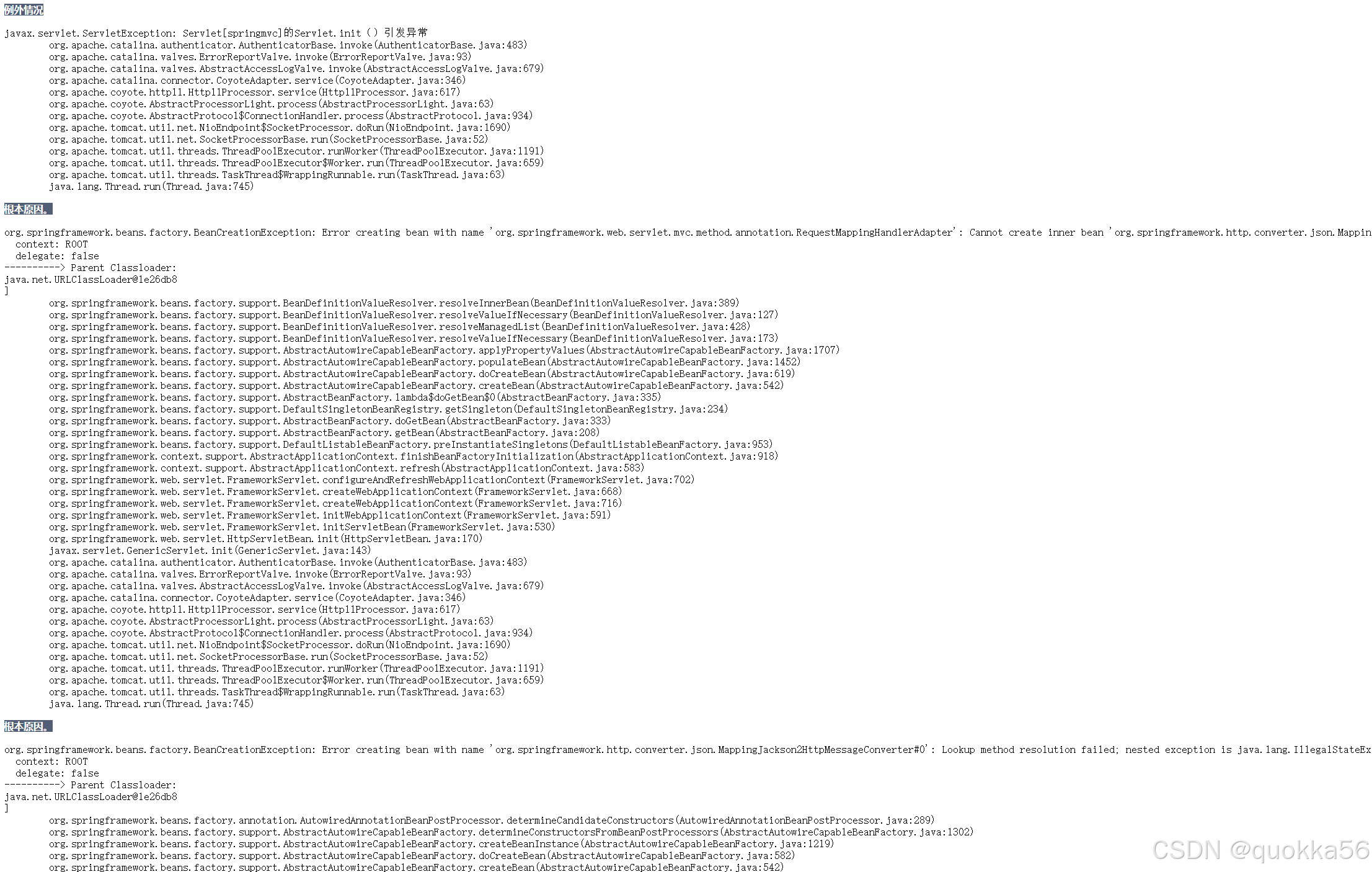
==============================================
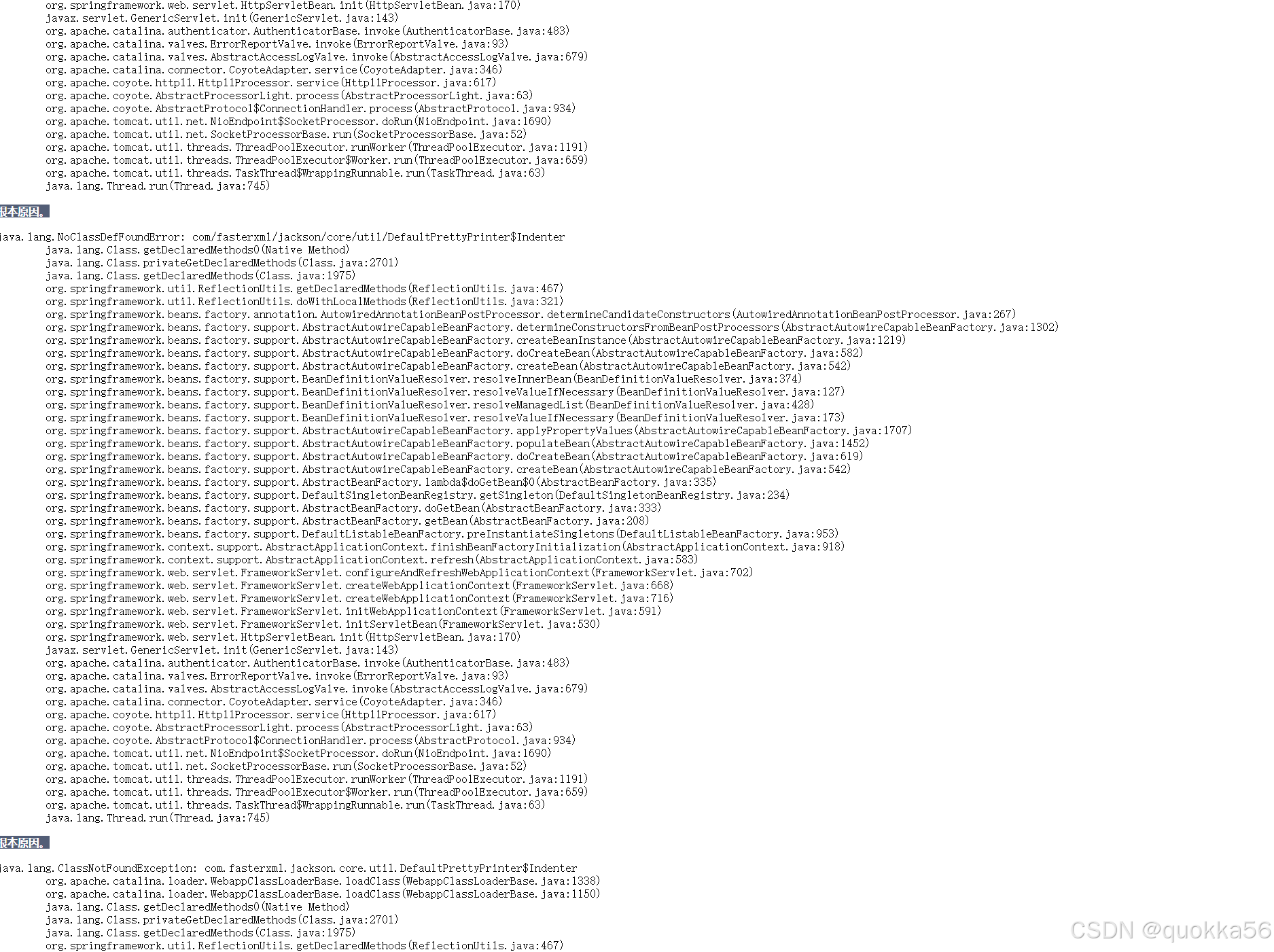
后来发现有一个大神说,少了一个jar包,我 附到下面了。添加之后则解决。
<!-- https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-databind -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.16.1</version>
</dependency>
来自一个小白得到结论:排错一定很重要,要看完所有的报错信息,找一个最重要的查找,而不是一直去查找最后一个报错,这样效率真的很低很低
Ajax
我们为什么要学习 ajax ?
笨的,我们从后端 传递过来的 json 数据,肯定需要接收呀
Ajax的优点
Ajax最大的优点就是,发送请求的时候不会影响用户的操作,相当于两条平行线一样,"你忙你的,我忙我的",不需要去等待页面的跳转而浪费时间
使用 ajax
①先下载 JQuery 包
官网拿走: https://jquery.com/download/
②导包
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
或者引入js文件
<script src="xxx.js"></script>
③使用示例代码呈上,如下
$.ajax({
url: "/api/getUserInfo",
type: "GET",
data: { userId: 123 },
dataType: "json",
success: function(result) {
console.log(result);
},
error: function(xhr, status, error) {
console.log(error);
}
});
写ajax时的问题
在写ajax的时候,我遇到了一个问题
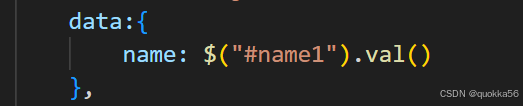
我在想未什么得不到 返回的 json 中的 name属性值呢?
在查找了半天答案知道自己错了,并且错的很离谱
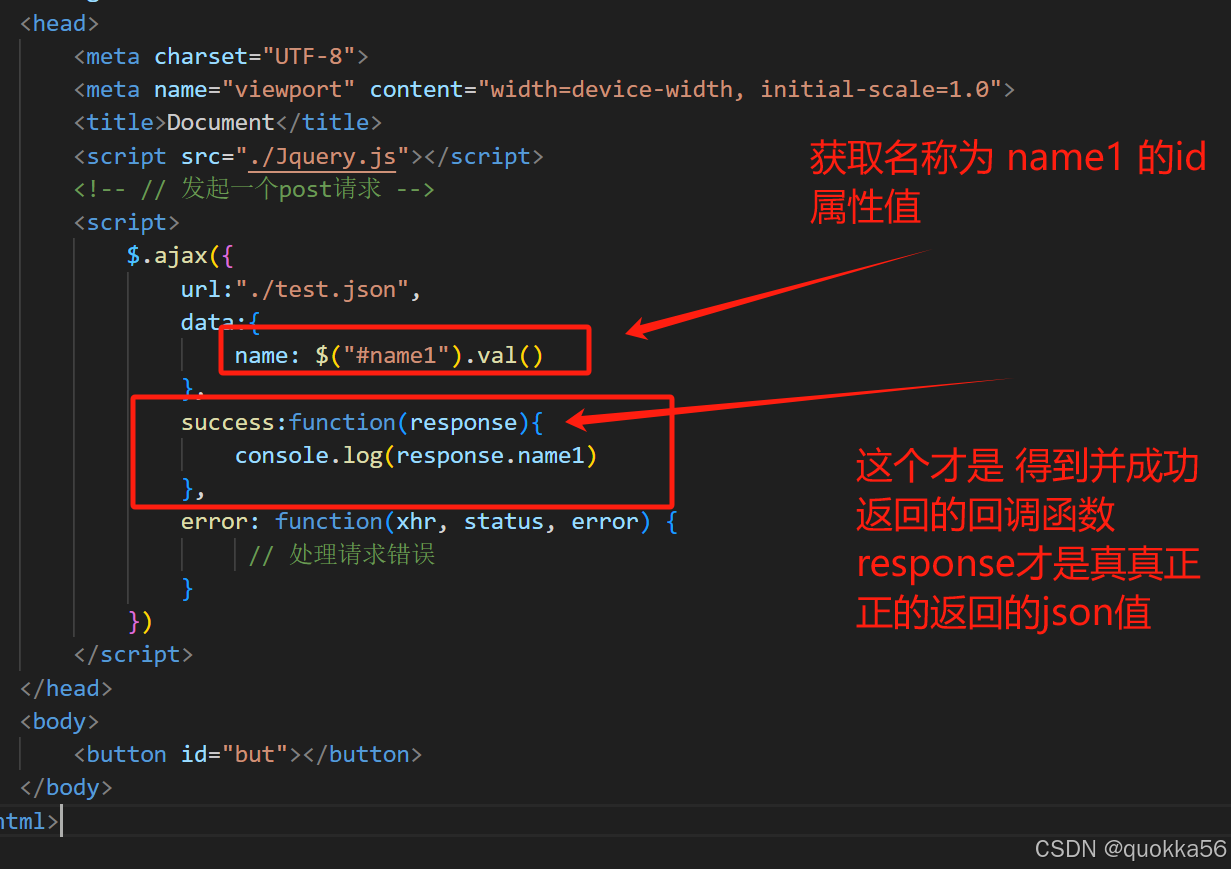
拦截器
自带 资源过滤 ,只会拦截 controller 里面的请求,而不会拦截 jsp/html/css等资源。减少了资源损耗。
User 和 Password 登录案例
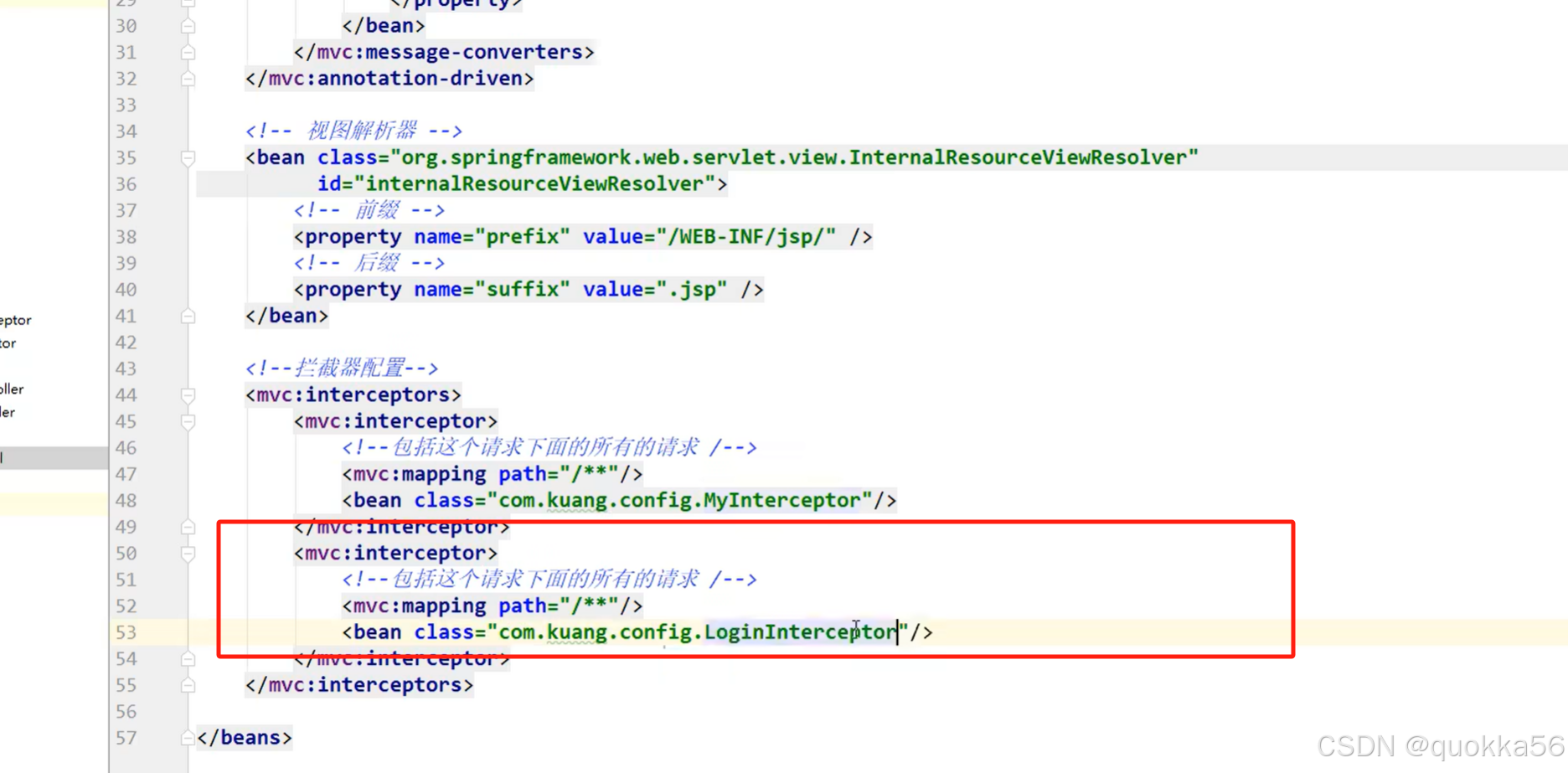
解释一下: contains ⇒ 包含 (英汉互译)
插一嘴,session节点注销,要记得哦。
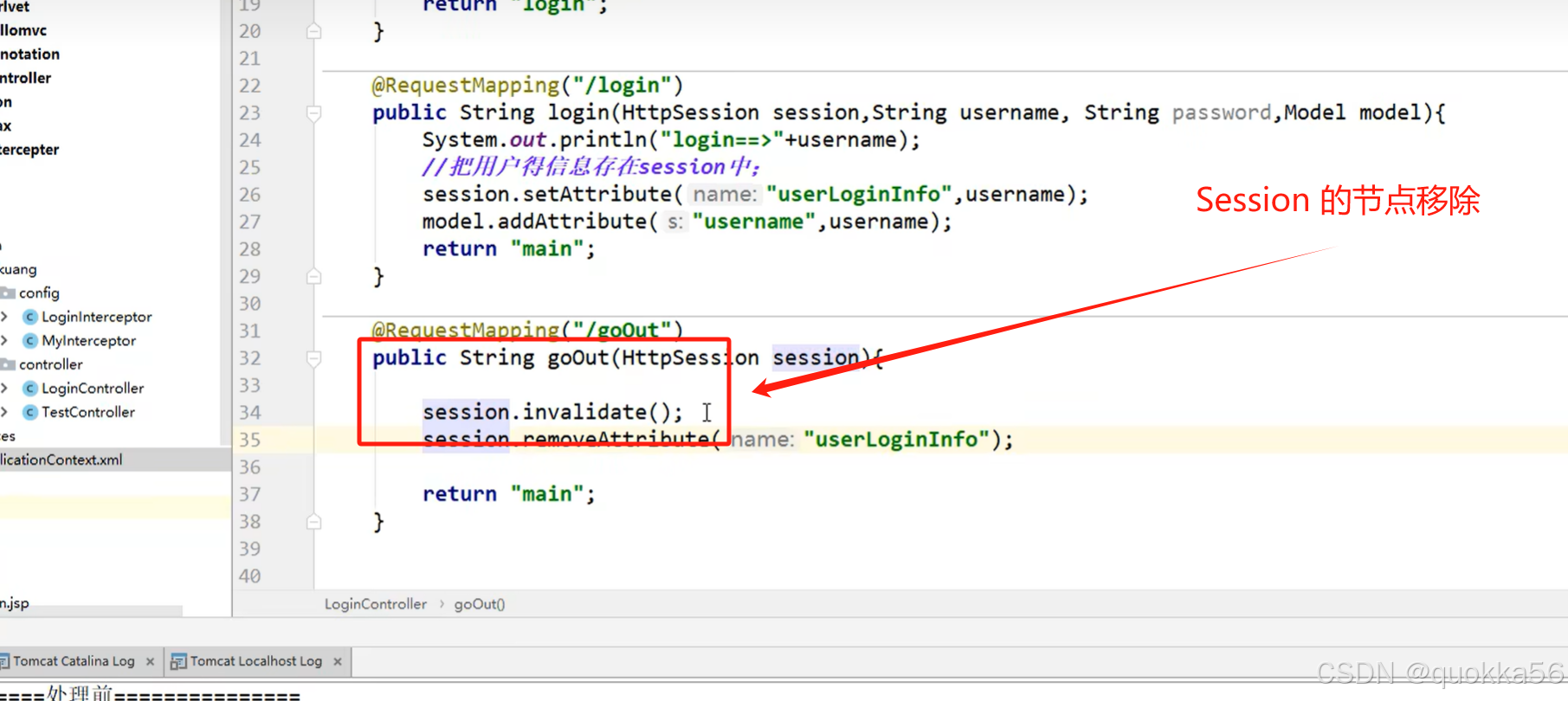
文件上传下载(需要的时候,去复制粘贴哦)
第一步:导包
第二步:写页面
第三部:配置
<!-- 处理文件上传与下载-->
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<!-- 必须和用户JSP 的pageEncoding属性一致,以便正确解析表单的内容 -->
<property name="defaultEncoding" value="UTF-8"></property>
<!-- 文件最大大小(字节) 1024*1024*50=50M-->
<property name="maxUploadSize" value="52428800"></property>
<!--resolveLazily属性启用是为了推迟文件解析,以便捕获文件大小异常-->
<property name="resolveLazily" value="true"/>
</bean>
jsp页面:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>图片上传</title>
</head>
<body>
<form action="${pageContext.request.contextPath }/clazz/upload" method="post" enctype="multipart/form-data">
<label>班级编号:</label><input type="text" name="cid" readonly="readonly" value="${param.cid}"/><br/>
<label>班级图片:</label><input type="file" name="xxx"/><br/>
<input type="submit" value="上传图片"/>
</form>
</body>
</html>
文件上传
1 // 文件上传
2 // @RequestMapping(value = "/upload", method = RequestMethod.POST) 等价于 @PostMapping()
3 @PostMapping(value = "upload")
4 @ResponseBody
5 public String fileUpload(MultipartFile[] uploadFile) {
6 for (MultipartFile file : uploadFile) {
7 //获取原始文件名
8 String originalFilename = file.getOriginalFilename();
9
10
11 try {
12 // 生成UUID
13 // 修正文件名出现乱码 防止文件名重复造成覆盖
14 originalFilename = new String(originalFilename.getBytes("ISO-8859-1"), "UTF-8");
15 } catch (UnsupportedEncodingException e) {
16 throw new RuntimeException(e);
17 }
18 System.out.println("文件名:" + originalFilename);
19 // UUID随机
20 String newFile = UUID.randomUUID().toString() + "_" + originalFilename;
21
22 // 指定文件上传路径
23 // Windows版--方式
24 String serverWin = "E:\\Chrom\\Download\\pictures\\";
25
26 // Linux版--方式
27 // String serverLinux = "/home";
28
29 File fileWin = new File(serverWin);
30 // 文件夹是否存在
31 if (!fileWin.exists()) {
32 fileWin.mkdir();
33 }
34 try {
35 // 文件与文件夹
36 file.transferTo(new File(serverWin + newFile));
37 } catch (IOException e) {
38 throw new RuntimeException(e);
39 }
40 }
41 return "success";
42 }
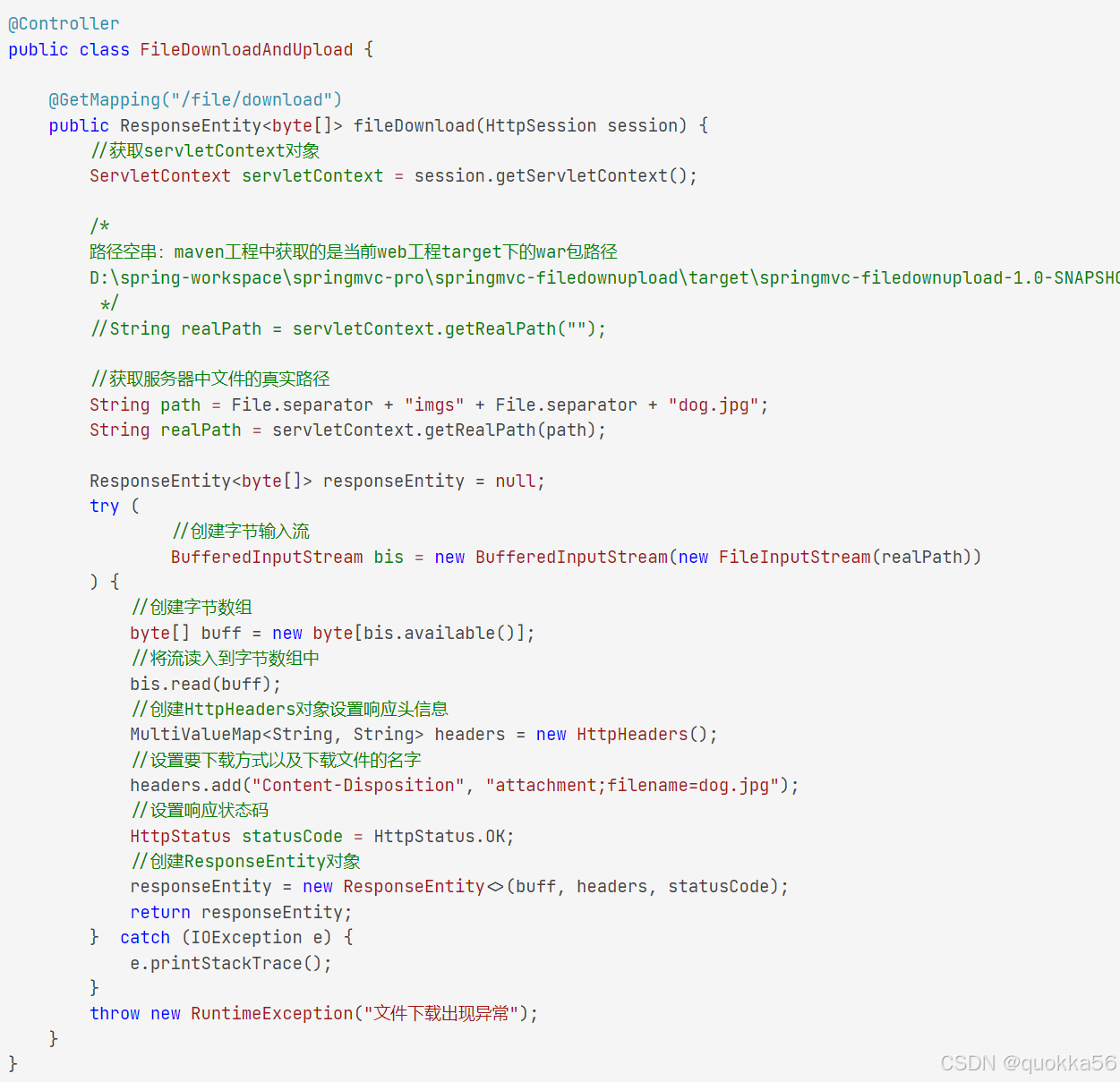
文件下载
// 文件下载
@GetMapping(value = "download")
public ResponseEntity<byte[]> download(HttpServletRequest httpServletRequest, String filename) {
// 指定文件下载目录
// 文件在服务器的目录
String fileDownloadWin = "E:\\Chrom\\Download\\pictures\\";
// 指定文件下载名称
File file = new File(fileDownloadWin + File.separator + filename);
HttpHeaders httpHeaders = new HttpHeaders();
filename = getFilename(httpServletRequest, filename);
httpHeaders.setContentDispositionFormData("attachment", filename);
httpHeaders.setContentType(MediaType.APPLICATION_OCTET_STREAM);
try {
return new ResponseEntity<>(FileUtils.readFileToByteArray(file), httpHeaders, HttpStatus.OK);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
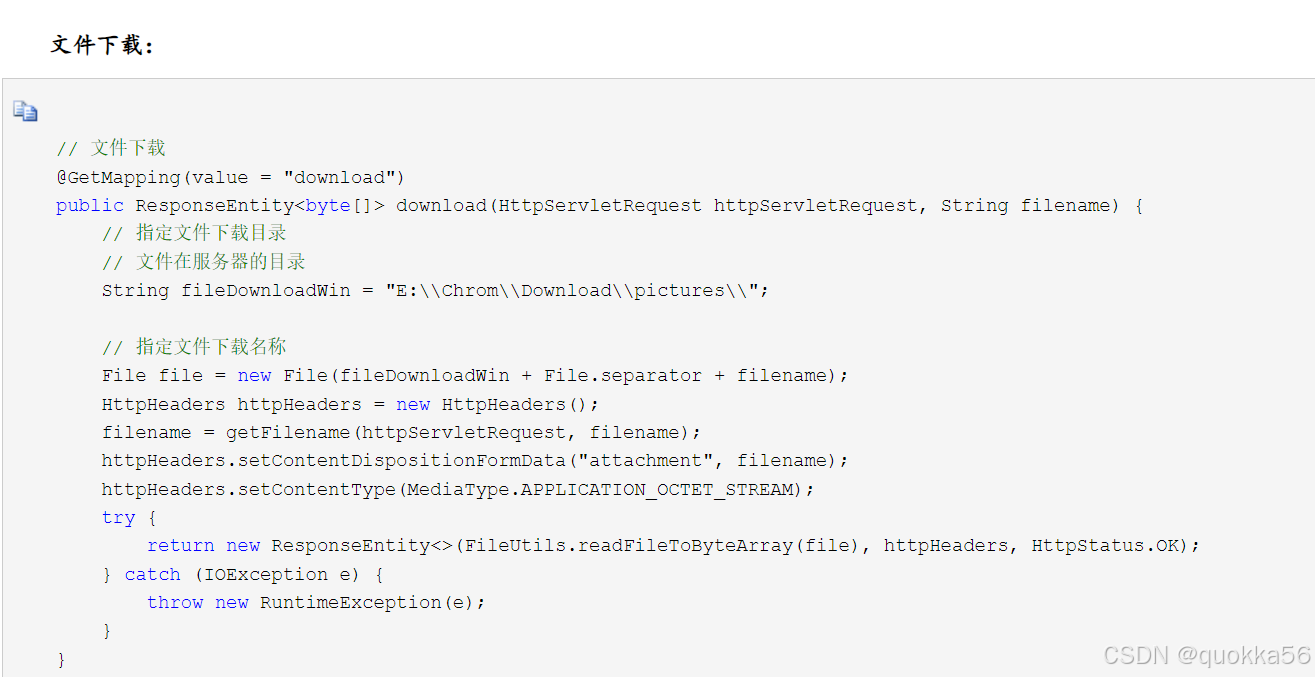