可扩展对话框
知识点
MainLayout->setSizeConstraint(QLayout::SetFixedSize);//固定窗口大小
extension.h
cpp
#ifndef EXTENSION_H
#define EXTENSION_H
#include <QDialog>
class Extension : public QDialog
{
Q_OBJECT
public:
Extension(QWidget *parent = nullptr);
~Extension();
private slots:
void ShowDetailInfo();
private:
void CreateBaseInfo();
void CreateDetailInfo();
QWidget* BaseWidget;
QWidget* DetailWidget;
};
#endif // EXTENSION_H
extension.cpp
cpp
#include "extension.h"
#include <QVBoxLayout>
#include <QLabel>
#include <QLineEdit>
#include <QComboBox>
#include <QPushButton>
#include <QDialogButtonBox>
#include <QHBoxLayout>
Extension::Extension(QWidget *parent)
: QDialog(parent)
{
setWindowTitle(tr("Extension Dialog"));
CreateBaseInfo();
CreateDetailInfo();
QVBoxLayout* MainLayout = new QVBoxLayout(this);
MainLayout->addWidget(BaseWidget);
MainLayout->addWidget(DetailWidget);
//MainLayout->setSizeConstraint(QLayout::SetFixedSize);//固定窗口大小
MainLayout->setSpacing(10);
}
Extension::~Extension() {}
void Extension::ShowDetailInfo()
{
if(DetailWidget->isHidden())
{
DetailWidget->show();
return;
}
DetailWidget->hide();
}
void Extension::CreateBaseInfo()
{
BaseWidget = new QWidget;
QLabel* NameLabel = new QLabel(tr("姓名:"));
QLineEdit* NameLineEdit = new QLineEdit;
QLabel* SexLabel = new QLabel("性别:");
QComboBox* SexComboBox = new QComboBox;
SexComboBox->insertItem(0,tr("男"));
SexComboBox->insertItem(1,tr("女"));
QGridLayout* LeftLayout = new QGridLayout;
LeftLayout->addWidget(NameLabel,0,0);
LeftLayout->addWidget(NameLineEdit,0,1);
LeftLayout->addWidget(SexLabel,1,0);
LeftLayout->addWidget(SexComboBox,1,1);
QPushButton* OkBtn = new QPushButton(tr("确定"));
QPushButton* DetailBtn = new QPushButton(tr("详细"));
QDialogButtonBox* BtnBox = new QDialogButtonBox(Qt::Vertical);
BtnBox->addButton(OkBtn,QDialogButtonBox::ActionRole);
BtnBox->addButton(DetailBtn,QDialogButtonBox::ActionRole);
//主布局
QHBoxLayout* MainLayout = new QHBoxLayout(BaseWidget);
MainLayout->addLayout(LeftLayout);
MainLayout->addWidget(BtnBox);
//事件
connect(DetailBtn,SIGNAL(clicked()),this,SLOT(ShowDetailInfo()));
}
void Extension::CreateDetailInfo()
{
DetailWidget = new QWidget;
QLabel* AgeLabel = new QLabel(tr("年龄"));
QLineEdit* AgeLineEdit = new QLineEdit(tr("30"));
QLabel* DepartmentLabel = new QLabel(tr("部门:"));
QComboBox* DepartmentComboBox = new QComboBox;
DepartmentComboBox->addItem(tr("部门1"));
DepartmentComboBox->addItem(tr("部门2"));
DepartmentComboBox->addItem(tr("部门3"));
DepartmentComboBox->addItem(tr("部门4"));
QLabel* EmailLabel = new QLabel(tr("Email:"));
QLineEdit* EmailEdit = new QLineEdit;
//布局
QGridLayout* MainLayout = new QGridLayout(DetailWidget);
MainLayout->addWidget(AgeLabel,0,0);
MainLayout->addWidget(AgeLineEdit,0,1);
MainLayout->addWidget(DepartmentLabel,1,0);
MainLayout->addWidget(DepartmentComboBox,1,1);
MainLayout->addWidget(EmailLabel,2,0);
MainLayout->addWidget(EmailEdit,2,1);
DetailWidget->hide();
}
main.cpp
cpp
#include "extension.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
Extension w;
w.show();
return a.exec();
}
运行图
初始化隐藏
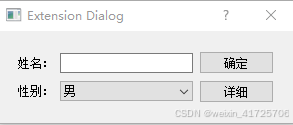
展现--点击--详细按钮
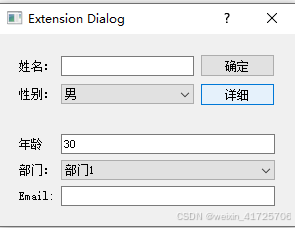