前言:
|-------|--------------------|-------|
| HSSF | 针对于excel03之前版本 | .xls |
| XSSF | 针对于excel07之后版本 | .xlsx |
| SXSSF | 针对于excel07之后版本,升级版 | .xlsx |
apache-poi官网图片
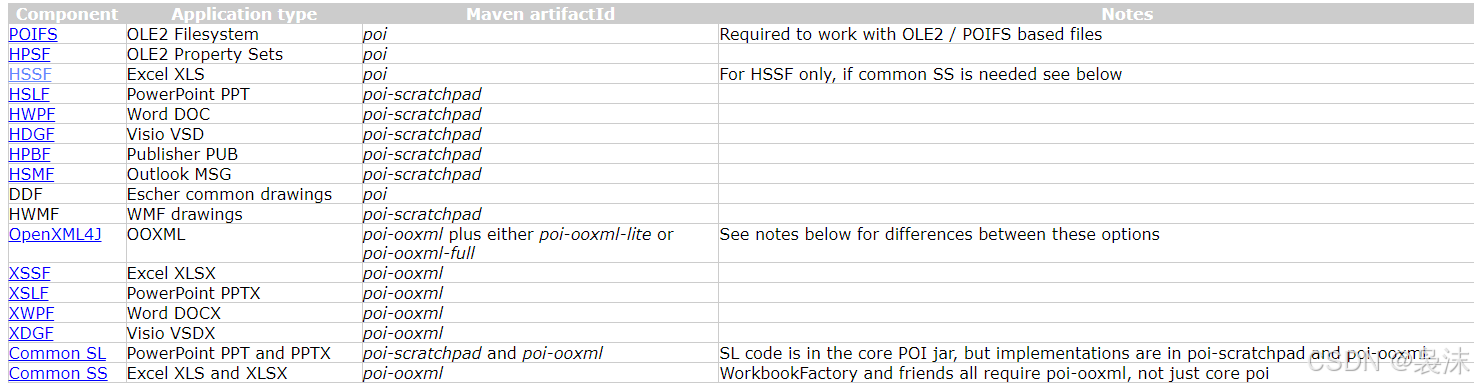
首先引入相关文档:
java
<dependencies>
<!-- 03- 版本的excel .xls -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<!-- 07+ 版本的excel .xlsx -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
</dependencies>
一、读取操作
目标excel文档如下:
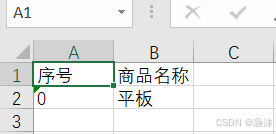
- 获取通过文件流读取Excel的工作簿Workbook
- 通过workbook 获取工作表
- 通过sheet获取row行
- 通过row获取cell单元格
- 使用cell.getStringCellValue()获取单元格的值
java
public static void main(String[] args) throws Exception {
readExcel07();
// readExcel07All();
}
/**
* 使用文件流读取.xlsx中的某个数据
* @throws Exception
*/
public static void readExcel07() throws Exception{
//1.通过文件流读取Excel工作部
FileInputStream inputStream = new FileInputStream("./测试.xlsx");
//2.获取工作簿
Workbook workbook = new XSSFWorkbook(inputStream);
//3.获取工作表
Sheet sheet = workbook.getSheet("07xlsx版本");
//4.获取行
Row row = sheet.getRow(0);
//5.获取列
Cell cell = row.getCell(0);
// RichTextString cellValue = cell.getRichStringCellValue();
String cellValue = cell.getStringCellValue();
System.out.println(cellValue);
//关闭资源
workbook.close();
inputStream.close();
}
/**
* 读取文件中所有的非空数据
* @throws Exception
*/
public static void readExcel07All() throws Exception{
//1.通过文件流读取Excel工作部
FileInputStream inputStream = new FileInputStream("./测试.xlsx");
//2.获取工作簿
Workbook workbook = new XSSFWorkbook(inputStream);
//3.获取工作表
Sheet sheet = workbook.getSheet("07xlsx版本");
//4.获取行
Row row = sheet.getRow(0);
if(row!=null){
for (int i = 0; i < row.getRowNum(); i++) {
//5.获取列
Cell cell = row.getCell(i);
if(cell!=null){
System.out.print(cell.getStringCellValue() + "/t");
}
}
}
//关闭资源
workbook.close();
inputStream.close();
}
运行结果如下:
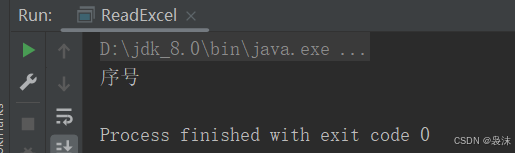
二、使用依赖向excel中写入相关数据
java
public static void main(String[] args) throws Exception {
// createDemoXLS();
createDemoXLSX();
}
public static void createDemoXLS() throws Exception {
//首先创建工作簿
Workbook workbook = new HSSFWorkbook();
//创建工作表
Sheet sheet = workbook.createSheet("03xls版本");
//创建第一行
Row row = sheet.createRow(0);
//创建单元格
Cell cell0 = row.createCell(0);
cell0.setCellValue("序号");
Cell cell1 = row.createCell(1);
cell1.setCellValue("商品名称");
//创建第二行
Row row1 = sheet.createRow(1);
Cell cell10 = row1.createCell(0);
cell10.setCellValue("0");
Cell cell11 = row1.createCell(1);
cell11.setCellValue("电脑");
//输出流
FileOutputStream fileOutputStream = new FileOutputStream("./测试.xls");
workbook.write(fileOutputStream);
//关闭输出流
workbook.close();
fileOutputStream.close();
}
public static void createDemoXLSX() throws Exception {
//首先创建工作簿
Workbook workbook = new XSSFWorkbook();
//创建工作表
Sheet sheet = workbook.createSheet("07xlsx版本");
//创建第一行
Row row = sheet.createRow(0);
//创建单元格
Cell cell0 = row.createCell(0);
cell0.setCellValue("序号");
Cell cell1 = row.createCell(1);
cell1.setCellValue("商品名称");
//创建第二行
Row row1 = sheet.createRow(1);
Cell cell10 = row1.createCell(0);
cell10.setCellValue("0");
Cell cell11 = row1.createCell(1);
cell11.setCellValue("平板");
//输出流
FileOutputStream fileOutputStream = new FileOutputStream("./测试.xlsx");
workbook.write(fileOutputStream);
//关闭输出流
workbook.close();
fileOutputStream.close();
}
三、批量插入
java
public static void main(String[] args) throws Exception {
// BatchXlsInsert();
// BatchXlsxInsert();
BatchXlsxBigInsert();
}
//大内存插入数据 .xls 03-
public static void BatchXlsInsert() throws Exception{
//记录当前时间
long start = System.currentTimeMillis();
//先创建工作部
Workbook workbook = new HSSFWorkbook();
//工作表
Sheet sheet = workbook.createSheet("03测试");
//调用复合组件
rowOriginSheet(sheet);
//输出
FileOutputStream outputStream = new FileOutputStream("./03BatchInsert.xls");
workbook.write(outputStream);
long end = System.currentTimeMillis();
System.out.println("创建03.xls耗时 ====> "+(end-start)/1000 + "s");
}
//大内存插入问题 07+ .xlsx
public static void BatchXlsxInsert() throws Exception{
//记录当前时间
long start = System.currentTimeMillis();
//先创建工作部
Workbook workbook = new XSSFWorkbook();
//工作表
Sheet sheet = workbook.createSheet("07测试");
//调用复合组件
rowOriginSheet(sheet);
//输出
FileOutputStream outputStream = new FileOutputStream("./07BatchInsert.xlsx");
workbook.write(outputStream);
long end = System.currentTimeMillis();
System.out.println("创建07.xls耗时 ====>"+(end-start)/1000 + "s");
}
//大内存插入问题 07+ .xlsx 升级版
public static void BatchXlsxBigInsert() throws Exception{
//记录当前时间
long start = System.currentTimeMillis();
//先创建工作部
Workbook workbook = new SXSSFWorkbook();
//工作表
Sheet sheet = workbook.createSheet("07测试");
//调用复合组件
rowOriginSheet(sheet);
//输出
FileOutputStream outputStream = new FileOutputStream("./07BatchBigInsert.xlsx");
workbook.write(outputStream);
long end = System.currentTimeMillis();
System.out.println("创建07.xls耗时 ====>"+(end-start)/1000 + "s");
}
public static void rowOriginSheet(Sheet sheet){
//创建行
// for (int i = 0; i < 65536; i++) {
for (int i = 0; i < 1045536; i++) {
Row row = sheet.createRow(i);
for (int j = 0; j < 20; j++) {
Cell cell = row.createCell(j);
cell.setCellValue(j+1);
}
}
}
其中,HSSFWorkbook只能插入少于65536行的数据,而XSSFWorkbook可以插入少于1045536条数据,但是其插入的速度较慢,因此使用SXSSF进行了升级,插入的速度更快。