Linux第一个小程序-进度条
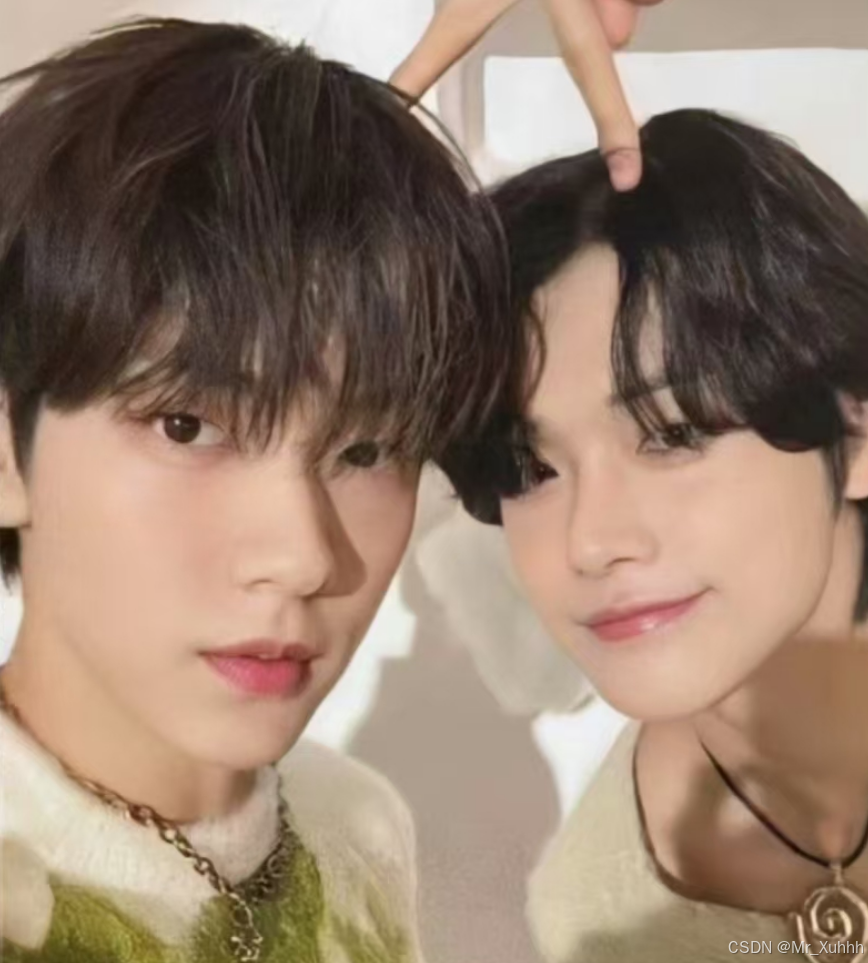
\r&&\n
回车概念
换行概念
老式打字机的例子
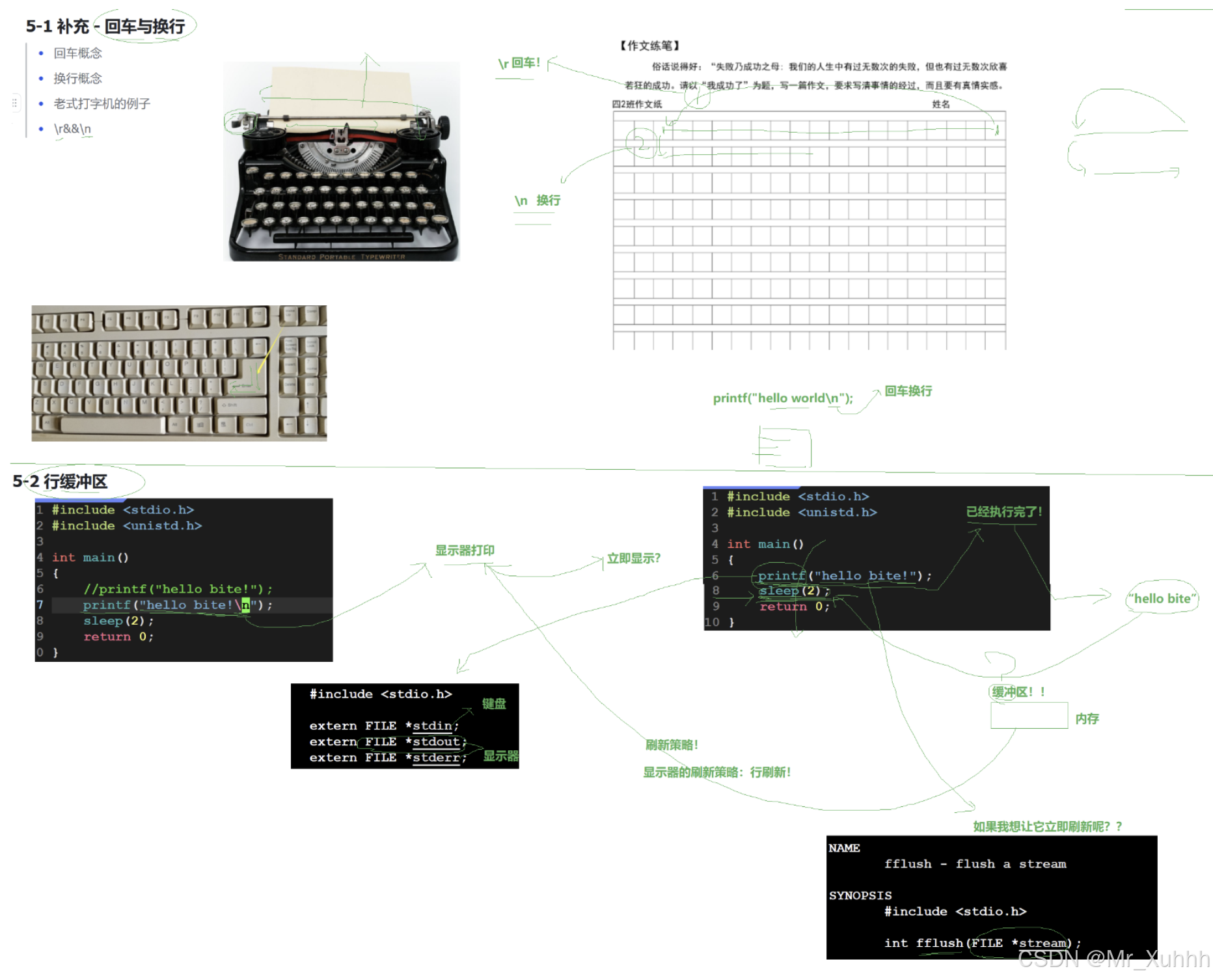
行缓冲区概念
什么现象?
C++
#include <stdio.h>
int main()
{
printf("hello Makefile!\n");
sleep(3);
return 0;
}
什么现象??
#include <stdio.h>
int main()
{
printf("hello Makefile!");
sleep(3);
return 0;
}
什么现象???
#include <stdio.h>
int main()
{
printf("hello Makefile!");
fflush(stdout);
sleep(3);
return 0;
}
版本1:
process.h
C
#pragma once
#include <stdio.h>
//v1
void process();
process.c
C
#include "process.h"
#include <string.h>
#include <unistd.h>
#define SIZE 101
#define STYLE '='
// v1: 展示进度条基本功能
void process()
{
int rate = 0;
char buffer[SIZE];
memset(buffer, 0, sizeof(buffer));
const char *lable = "|/-\\";
int len = strlen(lable);
while(rate <= 100)
{
printf("[%-100s][%d%%][%c]\r", buffer, rate, lable[rate%len]);
fflush(stdout);
buffer[rate] = STYLE;
rate++;
usleep(10000);
}
printf("\n");
}
版本2:
makefile:
BIN=process
#SRC=$(shell ls *.c)
SRC=$(wildcard *.c)
OBJ=$(SRC:.c=.o)
CC=gcc
RM=rm -f
$(BIN):$(OBJ)
@$(CC) $^ -o $@
@echo "链接 $^ 成 $@"
%.o:%.c
@$(CC) -c $<
@echo "编译... $< 成 $@"
.PHONY:clean
clean:
@$(RM) $(OBJ) $(BIN)
.PHONY:test
test:
@echo $(BIN)
@echo $(SRC)
@echo $(OBJ)
#$(BIN):$(OBJ)
# gcc $(OBJ) -o $(BIN)
#$(OBJ):$(SRC)
# gcc -c $(SRC) -o $(OBJ)
#
#.PHONY:clean
#clean:
# $(RM) $(BIN) $(OBJ)
#mytest:mytest.o
# gcc mytest.o -o mytest
#mytest.o:test.c
# gcc -c test.c -o mytest.o
#
#.PHONY:clean
#clean:
# rm -f *.o mytest
#mytest:test.o
# gcc test.o -o mytest
#test.o:test.s
# gcc -c test.s -o test.o
#test.s:test.i
# gcc -S test.i -o test.s
#test.i:test.c
# gcc -E test.c -o test.i
#
#.PHONY:clean
#clean:
# rm -f *.i *.s *.o mytest
#mytest:test.c
# gcc test.c -o mytest
#
#.PHONY:clean
#clean:
# rm -f mytest
process.h
C
#pragma once
#include <stdio.h>
//v1
void process();
//v2
void FlushProcess(const char*, double total, double current);
process.c
C
#include "process.h"
#include <string.h>
#include <unistd.h>
#define SIZE 101
#define STYLE '='
//v2: 根据进度,动态刷新一次进度条
void FlushProcess(const char *tips, double total, double current)
{
const char *lable = "|/-\\";
int len = strlen(lable);
static int index = 0;
char buffer[SIZE];
memset(buffer, 0, sizeof(buffer));
double rate = current*100.0/total;
int num = (int)rate;
int i = 0;
for(; i < num; i++)
buffer[i] = STYLE;
printf("%s...[%-100s][%.1lf%%][%c]\r", tips, buffer, rate, lable[index++]);
fflush(stdout);
index %= len;
if(num >= 100)printf("\n");
}
// v1: 展示进度条基本功能
void process()
{
int rate = 0;
char buffer[SIZE];
memset(buffer, 0, sizeof(buffer));
const char *lable = "|/-\\";
int len = strlen(lable);
while(rate <= 100)
{
printf("[%-100s][%d%%][%c]\r", buffer, rate, lable[rate%len]);
fflush(stdout);
buffer[rate] = STYLE;
rate++;
usleep(10000);
}
printf("\n");
}
main.c
C
#include "process.h"
#include <unistd.h>
#include <time.h>
#include <stdlib.h>
//函数指针类型
typedef void (*call_t)(const char*,double,double);
double total = 1024.0;
//double speed = 1.0;
double speed[] = {1.0, 0.5, 0.3, 0.02, 0.1, 0.01};
//回调函数
void download(int total, call_t cb)
{
srand(time(NULL));
double current = 0.0;
while(current <= total)
{
cb("下载中", total, current); // 进行回调
if(current>=total) break;
// 下载代码
int random = rand()%6;
usleep(5000);
current += speed[random];
if(current>=total) current = total;
}
}
void uploadload(int total, call_t cb)
{
srand(time(NULL));
double current = 0.0;
while(current <= total)
{
cb("上传中", total, current); // 进行回调
if(current>=total) break;
// 下载代码
int random = rand()%6;
usleep(5000);
current += speed[random];
if(current>=total) current = total;
}
}
int main()
{
download(1024.0, FlushProcess);
printf("download 1024.0MB done\n");
download(512.0, FlushProcess);
printf("download 512.0MB done\n");
download(256.0,FlushProcess);
printf("download 256.0MB done\n");
download(128.0,FlushProcess);
printf("download 128.0MB done\n");
download(64.0,FlushProcess);
printf("download 64.0MB done\n");
uploadload(500.0, FlushProcess);
return 0;
}
load 512.0MB done\n");
download(256.0,FlushProcess);
printf("download 256.0MB done\n");
download(128.0,FlushProcess);
printf("download 128.0MB done\n");
download(64.0,FlushProcess);
printf("download 64.0MB done\n");
uploadload(500.0, FlushProcess);
return 0;
}