首先在项目中引入Windows Script Host Object Model,引入方式如下图。
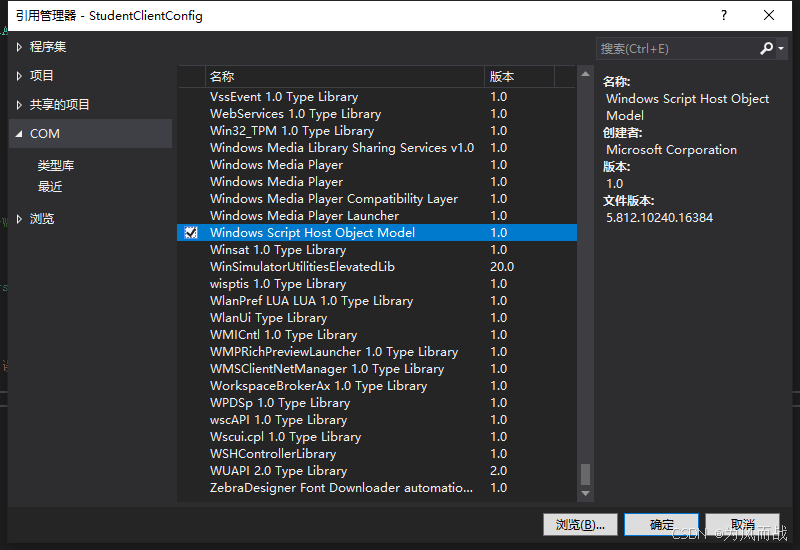
对于桌面快捷方式的修改无非就是将现有的快捷方式修改和添加新的快捷方式。
1、遍历桌面快捷方式,代码如下。
cs
string desktopPath = Environment.GetFolderPath(Environment.SpecialFolder.Desktop);
string[] files = Directory.GetFiles(desktopPath, "*.lnk");
foreach (string file in files)
{
WshShell shell = new WshShell();
IWshShortcut shortcut = (IWshShortcut)shell.CreateShortcut(file);
Debug.WriteLine($"快捷方式名称: {Path.GetFileNameWithoutExtension(file)}");
Debug.WriteLine($"目标路径: {shortcut.TargetPath}");
Debug.WriteLine($"工作目录: {shortcut.WorkingDirectory}");
Debug.WriteLine($"描述: {shortcut.Description}");
Debug.WriteLine($"图标位置: {shortcut.IconLocation}");
//Debug.WriteLine();
}
2、创建一个快捷方式,代码如下。
cs
// 创建WScript.Shell对象
WshShell _shell = new WshShell();
// 创建快捷方式
IWshShortcut _shortcut = (IWshShortcut)_shell.CreateShortcut(@"C:\Users\Public\Desktop\来个快捷方式.lnk");
// 设置快捷方式的属性
_shortcut.TargetPath = @"C:\Program Files\ScreenToGif\ScreenToGif.exe";
_shortcut.WorkingDirectory = @"C:\Program Files\ScreenToGif";
_shortcut.Description = "来个快捷方式";
_shortcut.IconLocation = "C:\\Program Files\\ScreenToGif\\ScreenToGif.exe,0";
// 保存快捷方式
_shortcut.Save();
Debug.WriteLine("快捷方式已创建。");
3、设置开机启动项。
cs
//AppDomain.CurrentDomain.BaseDirectory 获取当前程序所在文件夹
//Application.Current.Shutdown(); 退出当前程序
string appPath = @"C:\Program Files\ScreenToGif\ScreenToGif.exe"; // 替换为你的应用程序路径
string keyName = @"Software\Microsoft\Windows\CurrentVersion\Run";
RegistryKey key = Registry.CurrentUser.CreateSubKey(keyName);
if (key != null)
{
key.SetValue("来个快捷方式", appPath); // "YourAppName"是注册表项的名称
key.Close();
Debug.WriteLine("应用程序已设置为开机启动。");
}
4、有些时候设置好桌面的快捷方式,但是不能马上显示,需要刷新一下桌面才行,下面的代码是刷新桌面的代码。
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Runtime.InteropServices;
namespace XXXX
{
public class DesktopRefurbish
{
/// <summary>
/// 桌面刷新
/// </summary>
[DllImport("shell32.dll")]
public static extern void SHChangeNotify(HChangeNotifyEventID wEventId, HChangeNotifyFlags uFlags, IntPtr dwItem1, IntPtr dwItem2);
public static void DeskRef()
{
SHChangeNotify(HChangeNotifyEventID.SHCNE_ASSOCCHANGED, HChangeNotifyFlags.SHCNF_IDLIST, IntPtr.Zero, IntPtr.Zero);
}
}
#region public enum HChangeNotifyFlags
[Flags]
public enum HChangeNotifyFlags
{
SHCNF_DWORD = 0x0003,
SHCNF_IDLIST = 0x0000,
SHCNF_PATHA = 0x0001,
SHCNF_PATHW = 0x0005,
SHCNF_PRINTERA = 0x0002,
SHCNF_PRINTERW = 0x0006,
SHCNF_FLUSH = 0x1000,
SHCNF_FLUSHNOWAIT = 0x2000
}
#endregion//enum HChangeNotifyFlags
#region enum HChangeNotifyEventID
[Flags]
public enum HChangeNotifyEventID
{
SHCNE_ALLEVENTS = 0x7FFFFFFF,
SHCNE_ASSOCCHANGED = 0x08000000,
SHCNE_ATTRIBUTES = 0x00000800,
SHCNE_CREATE = 0x00000002,
SHCNE_DELETE = 0x00000004,
SHCNE_DRIVEADD = 0x00000100,
SHCNE_DRIVEADDGUI = 0x00010000,
SHCNE_DRIVEREMOVED = 0x00000080,
SHCNE_EXTENDED_EVENT = 0x04000000,
SHCNE_FREESPACE = 0x00040000,
SHCNE_MEDIAINSERTED = 0x00000020,
SHCNE_MEDIAREMOVED = 0x00000040,
SHCNE_MKDIR = 0x00000008,
SHCNE_NETSHARE = 0x00000200,
SHCNE_NETUNSHARE = 0x00000400,
SHCNE_RENAMEFOLDER = 0x00020000,
SHCNE_RENAMEITEM = 0x00000001,
SHCNE_RMDIR = 0x00000010,
SHCNE_SERVERDISCONNECT = 0x00004000,
SHCNE_UPDATEDIR = 0x00001000,
SHCNE_UPDATEIMAGE = 0x00008000,
}
#endregion
}
5、关闭防火墙,代码如下。
cs
try
{
ProcessStartInfo psi = new ProcessStartInfo
{
FileName = "netsh",
Arguments = "advfirewall set allprofiles state off",
UseShellExecute = false,
RedirectStandardOutput = true,
CreateNoWindow = true
};
Process process = Process.Start(psi);
process.WaitForExit();
Debug.WriteLine("Windows 防火墙已关闭。");
}
catch (Exception ex)
{
Debug.WriteLine("关闭 Windows 防火墙时出错: " + ex.Message);
}