QT QGraphicsView实现预览图片显示缩略图功能QT creator Qt5.15.2
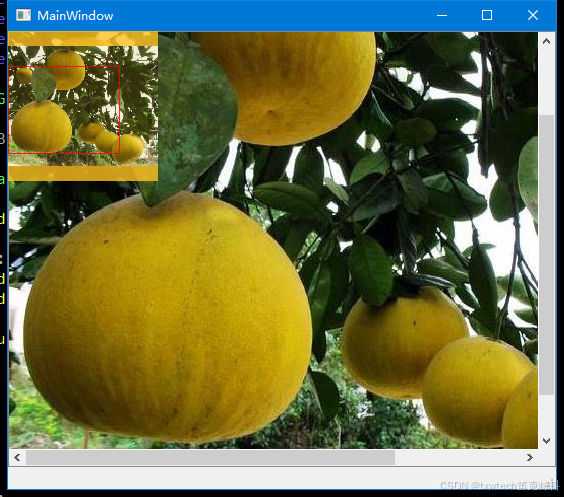
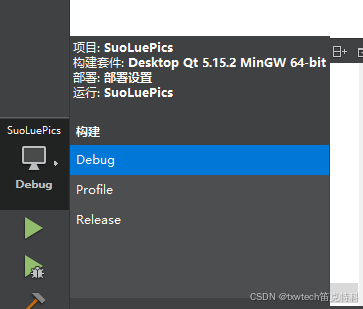
头文件:
#ifndef TGRAPHICSVIEW_H
#define TGRAPHICSVIEW_H
#include <QGraphicsView>
#include <QMainWindow>
#include <QObject>
#include <QWidget>
class TGraphicsView : public QGraphicsView
{
Q_OBJECT
public:
TGraphicsView(QWidget *parent = 0);
private slots:
void scrollBarValueChanged();
private:
void resizeEvent(QResizeEvent *event) override;
void updateThumbRoi();
struct PosInfo
{
int min;
int max;
int value;
int page;
};
private:
class Thumb;
Thumb *thumb;
};
class TGraphicsView::Thumb : public QWidget
{
Q_OBJECT
public:
using PosInfo = TGraphicsView::PosInfo;
Thumb(QWidget* parent = 0);
void updateImage();
void updateRoi(const PosInfo& xinfo, const PosInfo& yinfo);
private:
void paintEvent(QPaintEvent *event) override;
private:
QPixmap background;
QRect roi;
};
#endif // TGRAPHICSVIEW_H
cpp文件:
TGraphicsView::TGraphicsView(QWidget *parent) :
QGraphicsView(parent)
{
thumb = new Thumb(this);
QScrollBar* hsb = horizontalScrollBar();
connect(hsb, &QScrollBar::valueChanged, this, &TGraphicsView::scrollBarValueChanged);
QScrollBar* vsb = verticalScrollBar();
connect(vsb, &QScrollBar::valueChanged, this, &TGraphicsView::scrollBarValueChanged);
}
void TGraphicsView::resizeEvent(QResizeEvent *event)
{
QGraphicsView::resizeEvent(event);
thumb->updateImage();
updateThumbRoi();
}
void TGraphicsView::scrollBarValueChanged()
{
updateThumbRoi();
}
void TGraphicsView::updateThumbRoi()
{
QScrollBar* hsb = horizontalScrollBar();
PosInfo xinfo;
xinfo.min = hsb->minimum();
xinfo.max = hsb->maximum();
xinfo.value = hsb->value();
xinfo.page = hsb->pageStep();
QScrollBar* vsb = verticalScrollBar();
PosInfo yinfo;
yinfo.min = vsb->minimum();
yinfo.max = vsb->maximum();
yinfo.value = vsb->value();
yinfo.page = vsb->pageStep();
thumb->updateRoi(xinfo, yinfo);
}
/
TGraphicsView::Thumb::Thumb(QWidget* parent) :
QWidget(parent)
{
setFixedSize(150, 150);
}
void TGraphicsView::Thumb::paintEvent(QPaintEvent *)
{
QPainter painter(this);
painter.setOpacity(0.8);
painter.fillRect(QRect(0, 0, width(), height()), QColor(255, 192, 32));
int xoff = (width() - background.width()) / 2;
int yoff = (height() - background.height()) / 2;
painter.drawPixmap(xoff, yoff, background);
/* 绘制ROI */
//painter.setPen(QColor(32, 32, 32));
painter.setPen(QColor(255, 0, 0));
painter.setBrush(Qt::NoBrush);
painter.drawRect(roi.adjusted(xoff, yoff, xoff, yoff));
}
void TGraphicsView::Thumb::updateImage()
{
QGraphicsView* view = dynamic_cast<QGraphicsView*>(parent());
QRectF rect = view->sceneRect();
qreal ratio = qMin(width() / rect.width(), height() / rect.height());
background = QPixmap(rect.width() * ratio, rect.height() * ratio);
QPainter painter(&background);
QGraphicsScene* sc = view->scene();
sc->render(&painter);
update();
}
void TGraphicsView::Thumb::updateRoi(const PosInfo& xinfo, const PosInfo& yinfo)
{
int w = background.width();
int xwhole = ((xinfo.max - xinfo.min) + xinfo.page);
int roiLeft = w * xinfo.value / xwhole;
int roiWidth = w * xinfo.page / xwhole;
int h = background.height();
int ywhole = ((yinfo.max - yinfo.min) + yinfo.page);
int roiTop = h * yinfo.value / ywhole;
int roiHeight = h * yinfo.page / ywhole;
roi = QRect(roiLeft, roiTop, roiWidth, roiHeight);
update();
}
工程代码: