1.装饰者模式概念
允许在不修改原有类的情况下动态地为对象添加新的功能。它通过创建一个包装对象(即装饰器),并在运行时将这个包装对象附加到现有的对象上,从而达到扩展功能的目的。
2.装饰者的组成
1)抽象组件(Component)
抽象组件是一个接口或抽象类,定义了所有组件(包括具体组件和装饰器)的公共接口。
2)具体组件(Concrete Component)
具体组件实现了抽象组件接口中的方法,提供了基本的功能。
3)装饰器(Decorator)
装饰器也是一个接口或抽象类,继承自抽象组件接口。
4)具体装饰器(Concrete Decorator)
具体装饰器实现了装饰器接口中的方法,为被装饰的对象添加新的功能。
3.举个例子
想给自己的宠物狗(Concrete Component)穿衣服(Concrete Decorator)
想给自己的宠物狗(Concrete Component)穿衣服(Concrete Decorator)戴眼镜(Concrete Decorator)
如下图:
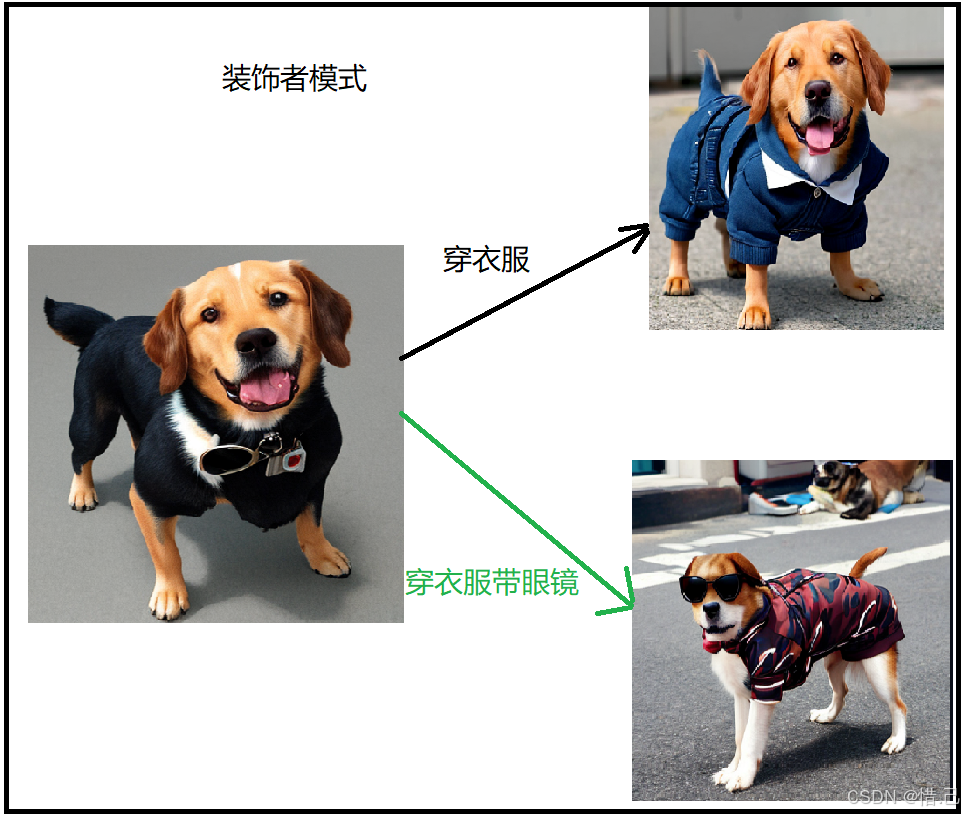
宠物猫===>衣服==》墨镜
4实现代码:
1)抽象组件
java
package org.xiji.Decorator;
/**
* 组件抽象类
*/
public interface Component {
/**
* 父组件操作
*/
void introduce();
}
2)具体组件
猫
java
package org.xiji.Decorator;
public class Cat implements Component {
@Override
public void introduce() {
System.out.println("这是一只猫");
}
}
狗
java
package org.xiji.Decorator;
public class Dog implements Component{
@Override
public void introduce() {
System.out.println("这是一只狗");
}
}
3)装饰器
java
package org.xiji.Decorator;
/**
* 装饰器抽象类
*/
public abstract class Decorator implements Component{
/**
* 包含组件接口
*/
Component component;
public Decorator(Component component) {
this.component = component;
}
/**
* 调用父组件方法
*/
@Override
public void introduce() {
component.introduce();
}
}
4)具体装饰器
墨镜
java
package org.xiji.Decorator;
/**
* 墨镜
*/
public class Sunglasses extends Decorator{
public Sunglasses(Component component) {
super(component);
}
@Override
public void introduce() {
super.introduce();
this.wearSunglasses();
}
public void wearSunglasses()
{
System.out.println("带墨镜");
}
}
红色衣服
java
package org.xiji.Decorator;
/**
* 红色衣服
*/
public class RedClothes extends Decorator{
public RedClothes(Component component) {
super(component);
}
@Override
public void introduce() {
super.introduce();
this.wearRedClothes();
}
/**
*
* 穿一件红色的衣服
*/
public void wearRedClothes() {
System.out.println("穿一件红色的衣服");
}
}
蓝色衣服
java
package org.xiji.Decorator;
public class BlueClothes extends Decorator{
public BlueClothes(Component component) {
super(component);
}
@Override
public void introduce() {
super.introduce();
this.wearBlueClothes();
}
/**
* 穿了一键蓝色的衣服
*/
public void wearBlueClothes(){
System.out.println("穿了一件蓝色的衣服");
}
}
5)测试类
java
package org.xiji.Decorator;
/**
* 装饰者模式测试类
*/
public class DecoratorMain
{
public static void main(String[] args) {
Component dog = new Dog();
dog.introduce();
//dog ==> blueClothes ==> Sunglasses
System.out.println("=======================");
System.out.println("给狗穿一件蓝色的衣服");
dog = new BlueClothes(dog);
dog.introduce();
System.out.println("=======================");
//把狗的衣服换下
dog = new Dog();
System.out.println("=======================");
System.out.println("给狗穿上红色衣服");
dog = new RedClothes(dog);
System.out.println("给狗带上墨镜");
dog = new Sunglasses(dog);
dog.introduce();
System.out.println("=======================");
//创建一只宠物猫
Component cat = new Cat();
cat.introduce();
System.out.println("=======================");
System.out.println("给猫穿一件红色的衣服");
cat = new RedClothes(cat);
System.out.println("给猫带上墨镜");
cat =new Sunglasses(cat);
cat.introduce();
System.out.println("=======================");
}
}
5.运行结果
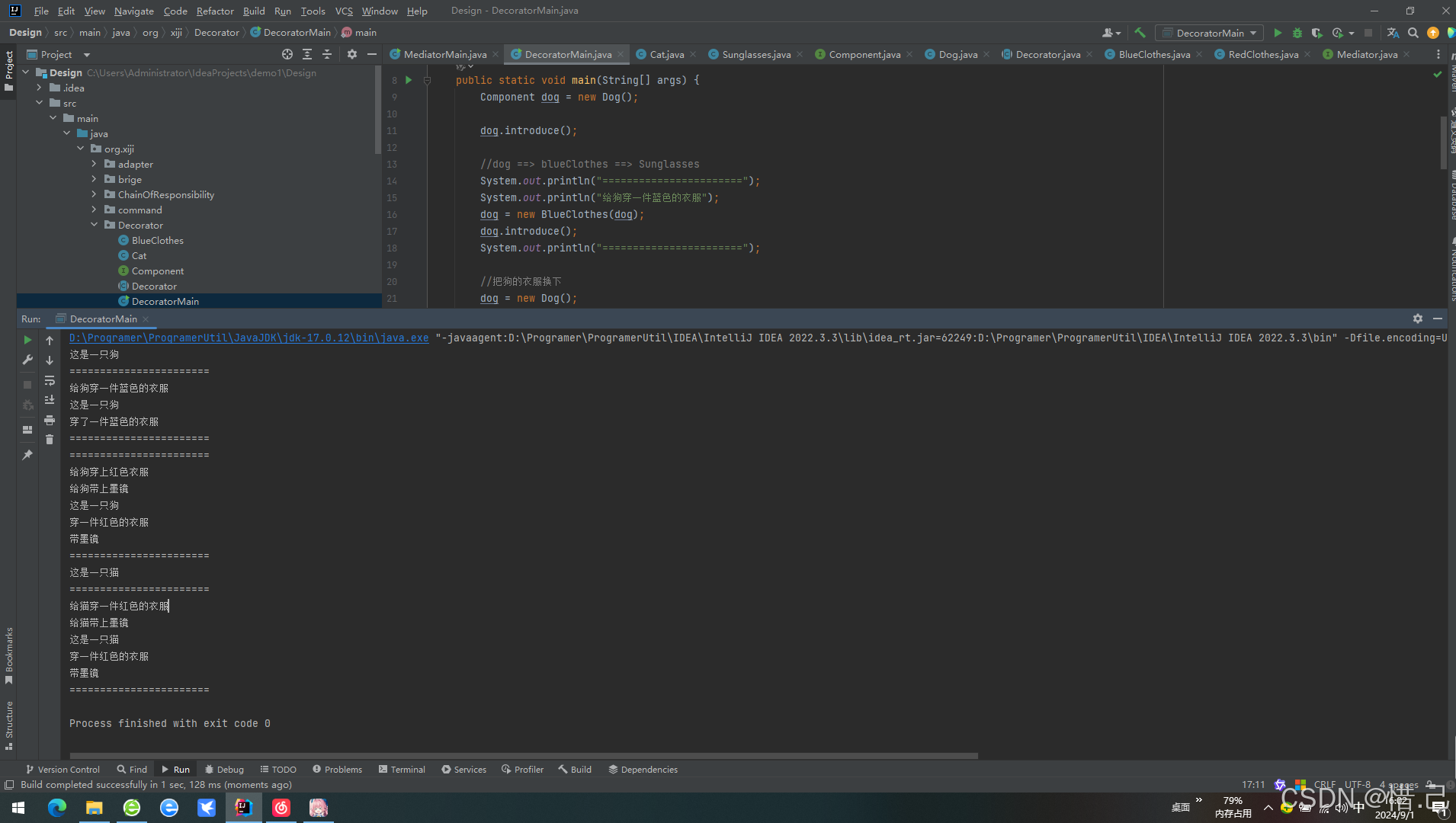