绑值语法( {{}} )
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
{{ message }}
<div>
<!-- {{num++}},不要这么写,别在这里运算,否则可能出错 -->
{{num}}
</div>
<div>{{bool}}</div>
<div>{{ arr.find(v => v.name === '张三')?.age }}</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.js"></script>
<script>
var app = new Vue({
el: '#app',//element
//把vue的实例绑定到id是app的上面
//
data: {
message: 'Hello I\'m lmm',
num: 1,
bool: true,
arr: [{ name: '张三', age: 20 }]
}
})
</script>
</body>
</html>
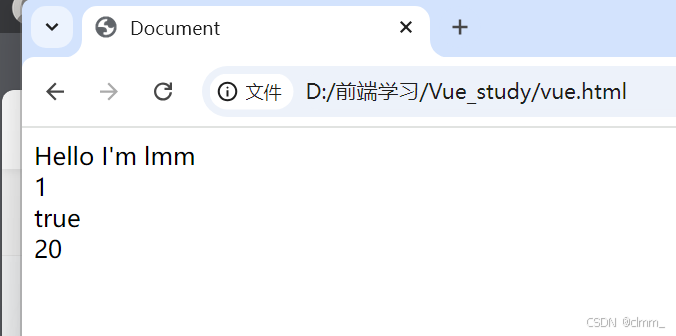
渲染html(v-html)
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
{{ message }}
<div>
<!-- {{num++}},不要这么写,别在这里运算,否则可能出错 -->
{{num}}
</div>
<div>{{bool}}</div>
<div>{{ arr.find(v => v.name === '张三')?.age }}</div>
<div v-html="htmlStr"></div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.js"></script>
<script>
var app = new Vue({
el: '#app',//element
//把vue的实例绑定到id是app的上面
//
data: {
message: 'Hello I\'m lmm',
num: 1,
bool: true,
arr: [{ name: '张三', age: 20 }],
htmlStr: '<strong>我是lmm</strong>'
}
})
</script>
</body>
</html>
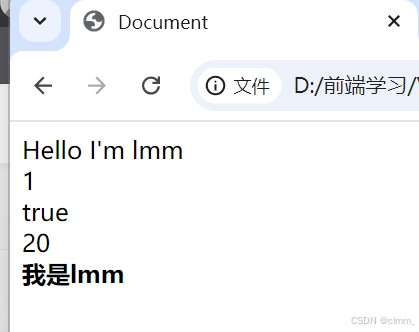
双向绑定(v-model)
双向绑定,同步更新
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
{{ message }}
<div>
<!-- {{num++}},不要这么写,千万别在这里运算,否则可能出错 -->
{{num}}
</div>
<div>{{bool}}</div>
<div>{{ arr.find(v => v.name === '张三')?.age }}</div>
<div v-html="htmlStr"></div>
<div>
<input type="text" v-model="count">
<div>{{count}}</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.js"></script>
<script>
var app = new Vue({
el: '#app',//element
//把vue的实例绑定到id是app的上面
//
data: {
message: 'Hello I\'m lmm',
num: 1,
bool: true,
arr: [{ name: '张三', age: 20 }],
htmlStr: '<strong>我是lmm</strong>',
count: ''
}
})
</script>
</body>
</html>
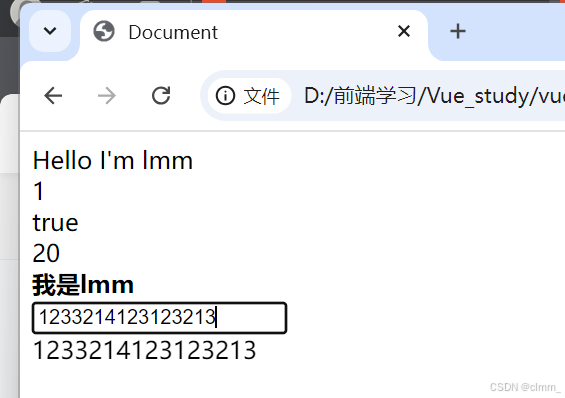
判断(v-if)
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
{{ message }}
<div>
<!-- {{num++}},不要这么写,千万别在这里运算,否则可能出错 -->
{{num}}
</div>
<div>{{bool}}</div>
<div>{{ arr.find(v => v.name === '张三')?.age }}</div>
<div v-html="htmlStr"></div>
<div>
<input type="text" v-model="count">
<div>{{count}}</div>
</div>
<div>
<div v-if="color === '红色'">红色</div>
<div v-if="color === '蓝色'">蓝色</div>
<div v-else>黑色</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.js"></script>
<script>
var app = new Vue({
el: '#app',//element
//把vue的实例绑定到id是app的上面
//
data: {
message: 'Hello I\'m lmm',
num: 1,
bool: true,
arr: [{ name: '张三', age: 20 }],
htmlStr: '<strong>我是lmm</strong>',
count: '',
color: '红色'
}
})
</script>
</body>
</html>
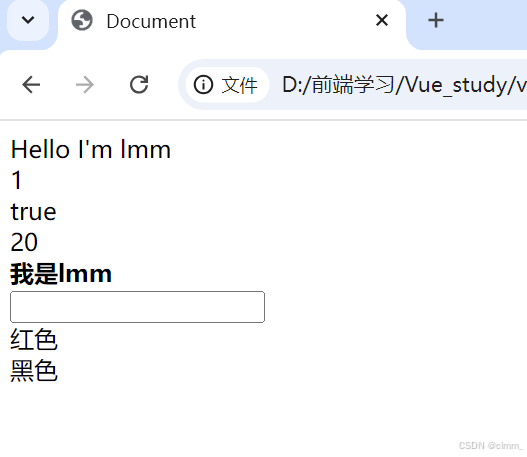
绑定属性(v-bind)
这个有更简写的版本,后面再补充
:
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
{{ message }}
<div>
<!-- {{num++}},不要这么写,千万别在这里运算,否则可能出错 -->
{{num}}
</div>
<div>{{bool}}</div>
<div>{{ arr.find(v => v.name === '张三')?.age }}</div>
<div v-html="htmlStr"></div>
<div>
<input type="text" v-model="count">
<div>{{count}}</div>
</div>
<div>
<div v-if="color === '红色'">红色</div>
<div v-if="color === '蓝色'">蓝色</div>
<div v-else>黑色</div>
</div>
<div>
<!-- <a href="https://www.baidu.com/">百度一下</a> -->
<!-- v-bind可以更灵活,不像这个是写死的 -->
<a v-bind:href="url">搜索一下</a>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.js"></script>
<script>
var app = new Vue({
el: '#app',//element
//把vue的实例绑定到id是app的上面
//
data: {
message: 'Hello I\'m lmm',
num: 1,
bool: true,
arr: [{ name: '张三', age: 20 }],
htmlStr: '<strong>我是lmm</strong>',
count: '',
color: '红色',
url: 'https://www.taobao.com/'
}
})
</script>
</body>
</html>
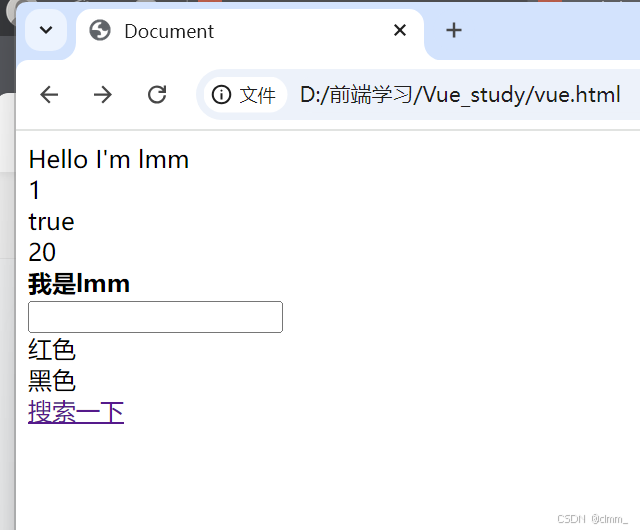
事件绑定(v-on)
@
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
{{ message }}
<div>
<!-- {{num++}},不要这么写,千万别在这里运算,否则可能出错 -->
{{num}}
</div>
<div>{{bool}}</div>
<div>{{ arr.find(v => v.name === '张三')?.age }}</div>
<div v-html="htmlStr"></div>
<div>
<input type="text" v-model="count">
<div>{{count}}</div>
</div>
<div>
<div v-if="color === '红色'">红色</div>
<div v-if="color === '蓝色'">蓝色</div>
<div v-else>黑色</div>
</div>
<div>
<!-- <a href="https://www.baidu.com/">百度一下</a> -->
<!-- v-bind可以更灵活,不像这个是写死的 -->
<a v-bind:href="url">搜索一下</a>
</div>
<div style="width: 100px; height: 100px; background-color: red;" v-on:click="clickDiv" id="testColor">
点我
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.js"></script>
<script>
var app = new Vue({
el: '#app',//element
//把vue的实例绑定到id是app的上面
//
data: {
message: 'Hello I\'m lmm',
num: 1,
bool: true,
arr: [{ name: '张三', age: 20 }],
htmlStr: '<strong>我是lmm</strong>',
count: '',
color: '红色',
url: 'https://www.taobao.com/'
},
methods: {
clickDiv() {
let color = document.getElementById('testColor').style.backgroundColor
if (color === 'red') {
document.getElementById('testColor').style.backgroundColor = 'blue'
}
else {
document.getElementById('testColor').style.backgroundColor = 'red'
}
}
}
})
</script>
</body>
</html>
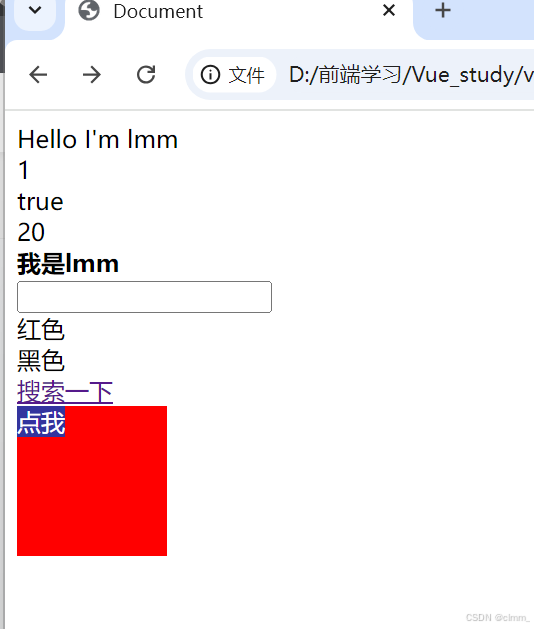
循环(v-for)
这里有些语法要精通一下
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
{{ message }}
<div>
<!-- {{num++}},不要这么写,千万别在这里运算,否则可能出错 -->
{{num}}
</div>
<div>{{bool}}</div>
<div>{{ arr.find(v => v.name === '张三')?.age }}</div>
<div v-html="htmlStr"></div>
<div>
<input type="text" v-model="count">
<div>{{count}}</div>
</div>
<div>
<div v-if="color === '红色'">红色</div>
<div v-if="color === '蓝色'">蓝色</div>
<div v-else>黑色</div>
</div>
<div>
<!-- <a href="https://www.baidu.com/">百度一下</a> -->
<!-- v-bind可以更灵活,不像这个是写死的 -->
<a v-bind:href="url">搜索一下</a>
</div>
<div style="width: 100px; height: 100px; background-color: red;" v-on:click="clickDiv" id="testColor">
点我
</div>
<div>
<div v-for="(item,index) in fruits" :key="index">{{index + '.'+item}}</div>
<div v-for="item in users" :key="item.name">{{item.name}}</div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.js"></script>
<script>
var app = new Vue({
el: '#app',//element
//把vue的实例绑定到id是app的上面
//
data: {
message: 'Hello I\'m lmm',
num: 1,
bool: true,
arr: [{ name: '张三', age: 20 }],
htmlStr: '<strong>我是lmm</strong>',
count: '',
color: '红色',
url: 'https://www.taobao.com/',
fruits: ['苹果', '香蕉', '橘子', '橘子'],
users: [{ name: 'lmm' }, { name: 'gcd' }]
},
methods: {
clickDiv() {
let color = document.getElementById('testColor').style.backgroundColor
if (color === 'red') {
document.getElementById('testColor').style.backgroundColor = 'blue'
}
else {
document.getElementById('testColor').style.backgroundColor = 'red'
}
}
}
})
</script>
</body>
</html>
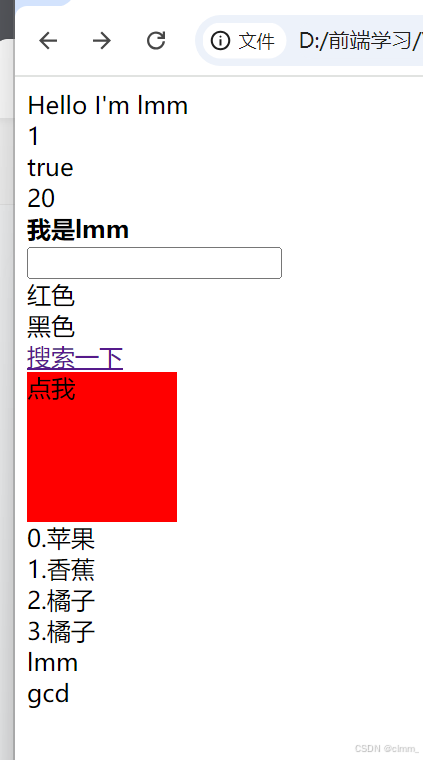
动态class与style绑定
补一下,对象字面量语法
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<style>
.active {
color: red;
}
</style>
<body>
<div id="app">
{{ message }}
<div>
<!-- {{num++}},不要这么写,千万别在这里运算,否则可能出错 -->
{{num}}
</div>
<div>{{bool}}</div>
<div>{{ arr.find(v => v.name === '张三')?.age }}</div>
<div v-html="htmlStr"></div>
<div>
<input type="text" v-model="count">
<div>{{count}}</div>
</div>
<div>
<div v-if="color === '红色'">红色</div>
<div v-if="color === '蓝色'">蓝色</div>
<div v-else>黑色</div>
</div>
<div>
<!-- <a href="https://www.baidu.com/">百度一下</a> -->
<!-- v-bind可以更灵活,不像这个是写死的 -->
<a v-bind:href="url">搜索一下</a>
</div>
<div style="width: 100px; height: 100px; background-color: red;" v-on:click="clickDiv" id="testColor">
点我
</div>
<div>
<div v-for="(item,index) in fruits" :key="index">{{index + '.'+item}}</div>
<div v-for="item in users" :key="item.name">{{item.name}}</div>
</div>
<div style="display: flex; margin-top: 30px;">
<select v-model="currentMenu">
<option value="首页">首页</option>
<option value="教师">教师</option>
<option value="学生">学生</option>
</select>
<!-- 动态绑定style和class -->
<div style="padding: 0 10px;" :style="{fontSize: item === currentMenu?'20px':'14px'}"
:class="{'active':item === currentMenu}" v-for="item in menus" :key="item">{{item}}
</div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.js"></script>
<script>
var app = new Vue({
el: '#app',//element
//把vue的实例绑定到id是app的上面
//
data: {
message: 'Hello I\'m lmm',
num: 1,
bool: true,
arr: [{ name: '张三', age: 20 }],
htmlStr: '<strong>我是lmm</strong>',
count: '',
color: '红色',
url: 'https://www.taobao.com/',
fruits: ['苹果', '香蕉', '橘子', '橘子'],
users: [{ name: 'lmm' }, { name: 'gcd' }],
menus: ['首页', '教师', '学生'],
currentMenu: '首页',
},
methods: {
clickDiv() {
let color = document.getElementById('testColor').style.backgroundColor
if (color === 'red') {
document.getElementById('testColor').style.backgroundColor = 'blue'
}
else {
document.getElementById('testColor').style.backgroundColor = 'red'
}
}
}
})
</script>
</body>
</html>
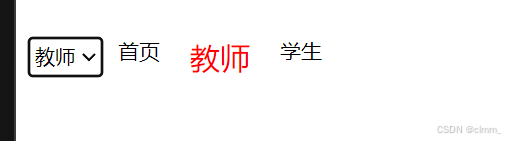