题意:将 OpenAI 的响应保存在 Azure 函数中到 Blob 存储
问题背景:
I used blob trigger template to create an Azure function that is triggered by new file updated in Azure blob storage. I am using python v2 to create Azure function in VScode. My issue is not able to store openai result into a new blob file. I noticed my function didn't have a function.json file. I am not sure if this is the problem for my issue. I tried redeploy couple times still didn't create a function.json.
我使用 Blob 触发器模板创建了一个 Azure 函数,该函数由 Azure Blob 存储中的新文件更新触发。我在 VSCode 中使用 Python v2 创建 Azure 函数。我的问题是无法将 OpenAI 的结果存储到一个新的 Blob 文件中。我注意到我的函数没有生成 `function.json` 文件,不确定这是否是导致问题的原因。我尝试重新部署了几次,但仍然没有生成 `function.json` 文件。
python
import azure.functions as func
import logging
import os
import openai
import PyPDF2
import io
openai.api_type = "azure"
openai.api_version = "2023-05-15"
openai.api_base = os.getenv("OPENAI_API_BASE") # Your Azure OpenAI resource's endpoint value.
openai.api_key = os.getenv("OPENAI_API_KEY")
app = func.FunctionApp()
@app.blob_trigger(arg_name="myblob", path="mypath/{name}",
connection="<My_BLOB_CONNECTION_SETTING>")
@app.blob_trigger_output(arg_name="outputblob", path="openai-outputs/text.txt",
connection="<My_BLOB_CONNECTION_SETTING>")
def blob_trigger(myblob: func.InputStream, outputblob: func.Out[str]) -> str:
logging.info(f"Python blob trigger function processed blob"
f"Name: {myblob.name}"
f"Blob Size: {myblob.length} bytes")
pdf_reader = PyPDF2.PdfReader(io.BytesIO(myblob.read()))
text = ""
for page in pdf_reader.pages:
text += page.extract_text()
logging.info(
f"textyyy: {text}")
messages= [
# my prompt
]
response = openai.ChatCompletion.create(
engine="testing3",
messages=messages
)
logging.info(f"response1111: {response}"
f"response['choices'][0]['message']['content']: {response['choices'][0]['message']['content']}")
outputblob.set(response['choices'][0]['message']['content'])
i followed this azure documentationto write the above code. It started failed to deploy to Azure function portal.
我按照这个 [Azure 文档](https://azure.microsoft.com/documentation) 编写了上述代码,但开始无法部署到 Azure Function 门户。
问题解决:
I was able to save the response of the OpenAI to blob storage using this code.
我能够使用以下代码将 OpenAI 的响应保存到 Blob 存储中。
I also used python v2. In python V2 function.json
file are not available. Binding is created using @app.blob_trigger
for trigger binding, @app.blob_input
for input binding,@app.blob_output
for output binding.
我也使用了 Python v2。在 Python v2 中,`function.json` 文件不可用。绑定是通过 `@app.blob_trigger` 创建触发器绑定,通过 `@app.blob_input` 创建输入绑定,通过 `@app.blob_output` 创建输出绑定。
PyPDF2
package is used to work with .pdf
files and perform operation on the file.
`PyPDF2` 包用于处理 `.pdf` 文件并对文件执行操作。
I have used @app.blob_trigger
to trigger the function code, @app.blob_input
to read the content of the file and @app.blob_output
to modify the file.
我使用了 `@app.blob_trigger` 来触发函数代码,`@app.blob_input` 来读取文件内容,以及 `@app.blob_output` 来修改文件。
function_app.py
:
python
import azure.functions as func
import logging
import os
import openai
openai.api_type = "azure"
openai.api_base = os.getenv("OPENAI_API_BASE")
openai.api_version = "2023-07-01-preview"
openai.api_key = os.getenv("OPENAI_API_KEY")
app = func.FunctionApp()
@app.blob_trigger(arg_name="myblob", path="response",
connection="BlobStorageConnectionString")
@app.blob_input(arg_name="inputblob",path="response/hello.txt",connection="BlobStorageConnectionString")
@app.blob_output(arg_name="output",path="chatgpt-conversation/conversation.txt",connection="BlobStorageConnectionString2")
def blob_trigger(myblob: func.InputStream, inputblob: str, output:func.Out[bytes]):
logging.info(f"Python blob trigger function processed blob"
f"Name: {myblob.name}"
f"Blob Size: {myblob.length} bytes")
content= inputblob
logging.info(f"Contentof the file is : {content}")
ai_responses=[]
response = openai.ChatCompletion.create(
engine="test_chatgpt",
messages=[
{"role": "system", "content": "You are an AI assistant that helps people find information."},
{"role":"user","content": content}
])
ai_responses.append(f"Input: {content}\nResponse: {response['choices'][0]['message']['content']}\n\n")
logging.info(ai_responses)
output.set("\n".join(ai_responses))
local.settings.json
python
{
"IsEncrypted": false,
"Values": {
"AzureWebJobsStorage": "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx",
"FUNCTIONS_WORKER_RUNTIME": "python",
"AzureWebJobsFeatureFlags": "EnableWorkerIndexing",
"OPENAI_API_BASE":"https://<xxxxxx>.openai.azure.com/",
"OPENAI_API_KEY": "xxxxxxxxxxxxxxxxxxxx",
"BlobStorageConnectionString":"xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx",
"BlobStorageConnectionString2":"xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
}
}
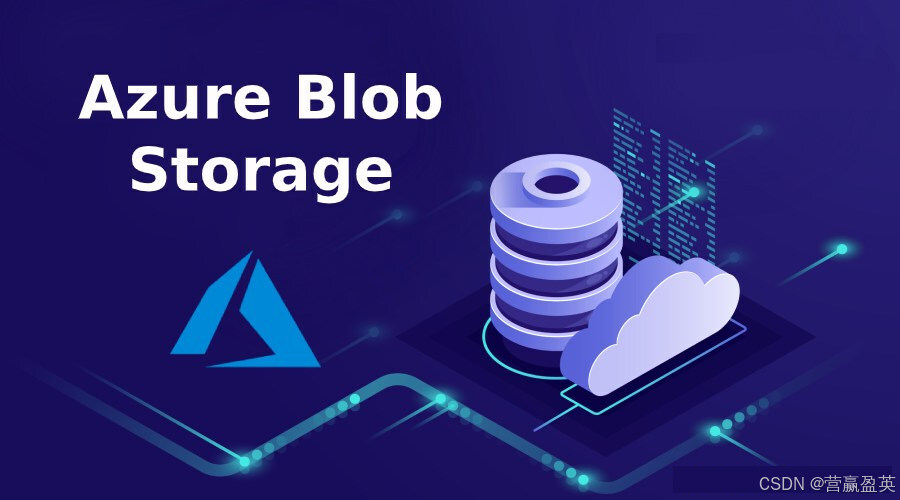