作业:1、手动实现stack部分功能
2、手动实现queue部分功能
1、
头文件
#ifndef HEAD_H
#define HEAD_H
#include <iostream>
#include<cstring>
#define MAX 10
using namespace std;
class Stack
{
private:
int* data; //存储栈的容器,指向堆区空间
int top; //记录栈顶下标的元素
int max; //记录栈的最大容量
public:
Stack(); //无参构造
Stack(int); //有参构造
~Stack(); //析构函数
Stack& operator=(Stack& other); //赋值函数
int& mytop();
//判空
bool empty();
//size 返回容纳的元素数
int mysize();
//栈顶插入
void mypush(int);
//出栈
Stack& mypop();
};
#endif // HEAD_H
源文件
#include"head.h"
Stack ::Stack() //无参构造
{
data = new int[MAX];
max = MAX;
top = -1;
cout<<"无参构造了一个容量为10的栈"<<endl;
}
Stack ::Stack(int num) //有参构造
{
data = new int[num];
max = num;
top = -1;
cout<<"有参构造了一个容量为"<<num<<"的栈"<<endl;
}
Stack ::~Stack() //析构函数
{
top = -1;
delete[] data;
data=NULL;
cout<<"析构函数调用"<<endl;
}
//赋值函数
Stack& Stack ::operator=(Stack& other)
{
if(this!=&other) //自检
{
delete [] data;
data = new int[other.max];
this->top=other.top;
this->max = other.max;
for(int i=0;i<max;i++)
{
data[i]=other.data[i];
}
}
cout<<"赋值函数调用"<<endl;
return *this;
}
//访问栈顶元素
int& Stack :: mytop()
{
return data[top];
}
//判空
bool Stack ::empty()
{
return top==-1;
}
//size 返回容纳的元素数
int Stack :: mysize()
{
return top+1;
}
//栈顶插入
void Stack ::mypush(int value)
{
//插入逻辑
if((top+1)<=max) //未满
{
data[++top] = value;
}else
{
cout<<"栈已满"<<endl;
}
}
//出栈
Stack& Stack::mypop()
{
//出栈逻辑
if(empty())
{
cout<<"栈已空"<<endl;
}else
{
top--;
}
return *this;
}
main
#include"head.h"
int main()
{
Stack s1; //无参构造
s1.mypush(1); //调用入栈
s1.mypush(12);
s1.mypush(3);
s1.mypush(99);
cout<<"s1.szie ="<<s1.mysize()<<endl; //调用size
cout<<"s1 栈顶为"<<s1.mytop()<<endl; //调用top
cout<<"**********************************************"<<endl;
Stack s2(5); //有参构造
s2=s1; //赋值函数
cout<<"s2.szie ="<<s2.mysize()<<endl;
cout<<"s2 栈顶为"<<s2.mytop()<<endl;
s2.mypop(); //调用出栈
cout<<"s2 栈顶为"<<s2.mytop()<<endl;
return 0;
}
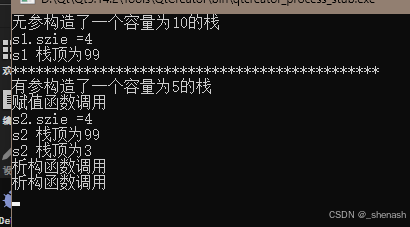
2、
头文件
#ifndef QUEUE_H
#define QUEUE_H
#include <iostream>
#define MAX 10
using namespace std;
class Queue
{
private:
int *data; //存储队列的容器
int front; //记录队头下标
int tail; //记录队尾下标
int capacity; //记录队列最大容量
public:
Queue(); //无参构造
Queue(int); //有参构造
~Queue(); //析构函数
Queue& operator=(Queue &other); //赋值函数
int& myfront(); //访问第一个元素
int& mytail(); //访问最后一个元素
bool empty(); //判空
bool full(); //判满
int getsize(); //获取当前元素数量
void mypush(int); //入队
Queue& mypop(); //出队
void queue_show();
};
#endif // QUEUE_H
源文件
#include"queue.h"
//无参构造
Queue ::Queue()
{
data = new int[MAX];
front = tail =0;
capacity = MAX;
cout<<"无参构造了容量为"<<capacity<<"的队列"<<endl;
}
Queue ::Queue(int max) //有参构造
{
data= new int[max];
front = tail =0;
capacity = max;
cout<<"有参构造了容量为"<<capacity<<"的队列"<<endl;
}
Queue:: ~Queue() //析构函数
{
delete []data;
cout<<"析构函数调用成功"<<endl;
}
Queue& Queue ::operator=(Queue &other) //赋值函数
{
if(this!=&other) //自检
{
delete [] data;
data = new int[other.capacity];
this->front=other.front;
this->tail = other.tail;
this->capacity=other.capacity;
for(int i=front;i!=tail;i=(i+1)%capacity)
{
data[i]=other.data[i];
}
}
cout<<"赋值函数调用"<<endl;
return *this;
}
int& Queue ::myfront() //访问第一个元素
{
return data[front];
}
int& Queue ::mytail() //访问最后一个元素
{
return data[tail-1];
}
//判空
bool Queue ::empty()
{
return front==tail;
}
//判满
bool Queue ::full()
{
return (tail+1)%capacity==front;
}
//获取当前元素数量
int Queue ::getsize()
{
return (tail-front+capacity)%capacity;
}
//入队
void Queue ::mypush(int value)
{
if(full())
{
cout<<"队列满"<<endl;
}else
{
data[tail]=value;
tail= (tail+1)%capacity;
}
}
//出队
Queue& Queue ::mypop()
{
if(empty())
{
cout<<"队列已空"<<endl;
}else
{
front = (front+1)%capacity;
}
return *this;
}
void Queue ::queue_show()
{
if(empty())
{
cout<<"队列为空"<<endl;
}
else
{
cout<<"队列中的元素为:";
for(int i=front;i!=tail;i=(i+1)%capacity)
{
cout<<data[i]<<'\t';
}
cout<<endl;
}
}
主文件
#include"queue.h"
int main()
{
Queue q1;
q1.mypush(3);
q1.mypush(2);
q1.mypush(4);
q1.mypush(5);
q1.mypush(1);
cout<<"队列第一个元素为:"<<q1.myfront()<<endl;
cout<<"队列最后一个元素为:"<<q1.mytail()<<endl;
cout<<"队列中有:"<<q1.getsize()<<"个元素"<<endl;
cout<<"***************************************************"<<endl;
Queue q2(4);
q2.mypop();
q2=q1;
q2.queue_show();
q2.mypop();
q2.queue_show();
cout<<"***************************************************"<<endl;
return 0;
}
效果图
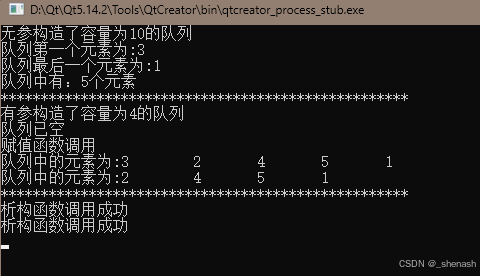
思维导图