对话框通常是一个顶层窗口,出现在程序最上层,用于实现短期任务或者简洁的用户交互
一、消息对话框(QMessageBox)
1、QMessageBox类成员函数实现
1)实例化 QMessageBox类 对象
2)设置对象属性
3)显示对话框
QMessageBox::QMessageBox( //构造函数函数名
QMessageBox::Icon icon, //图标
const QString &title, //窗口标题
const QString &text, //窗口文本内容
QMessageBox::StandardButtons buttons = NoButton,//按键
QWidget *parent = nullptr) //父组件
参数1:是一个该类提供的内部枚举类型Constant Value Description
QMessageBox::NoIcon 0 不提供图标.
QMessageBox::Question 4 提供一个问号的图标
QMessageBox::Information 1 提供一个 i 符号的图标
QMessageBox::Warning 2 提供一个感叹号的图标表示警告
QMessageBox::Critical 3 提供一个叉号图标表示错误
参数4:是给对话框上提供的用于用户操作的按钮,也是一个枚举类型,如果需要提供多个按钮,中间使用位或隔开Constant Value Description
QMessageBox::Ok 0x00000400 An "OK" button defined with the AcceptRole
QMessageBox::Open 0x00002000 An "Open" button defined with the AcceptRole
QMessageBox::Save 0x00000800 A "Save" button defined with the AcceptRole
QMessageBox::Cancel 0x00400000 A "Cancel" button defined with the RejectRole
QMessageBox::Close 0x00200000 A "Close" button defined with the RejectRole
......
2、静态函数实现
系统提供4个静态成员函数:information、question、warning、critical
[static] QMessageBox::StandardButton//返回的是一个按钮
QMessageBox::information( //函数名
QWidget *parent, //父组件
const QString &title, //对话框标题
const QString &text, //对话框文本内容
QMessageBox::StandardButtons buttons = Ok, //提供的按钮 QMessageBox::StandardButton defaultButton = NoButton)
二、颜色对话框(QColorDialog)
1、所需头文件 QColorDialog 颜色对话框类 QColor 颜色类
2、一般不会对这个对话框进行太多设置,所以用静态成员函数实现
[static] QColor //返回一个颜色类对象
QColorDialog::getColor( //函数名
const QColor &initial = Qt::white, //打开对话框后的初始颜色
QWidget *parent = nullptr, //父组件
const QString &title = QString()) //对话框标题
三、字体对话框(QFrotDialog)
1、所需头文件 QFrotDialog 字体对话框类 QFrot字体类
2、一般不会对这个对话框进行太多设置,所以用静态成员函数实现
[static] QFont //返回值一个字体类的对象
QFontDialog::getFont( //函数名
bool *ok, //用于地址传递,判断用户是否选中了某个字体
const QFont &initial, //初始字体,对话框中的第一个字体,如果用户点击了取消,则将该字体作为函数返回值
QWidget *parent = nullptr, //父组件
const QString &title = QString()) //对话框标题
四、文件对话框(QFileDialog)
1、所需头文件 QFileDialog 文件对话框类
2、一般不会对这个对话框进行太多设置,所以用静态成员函数实现
[static] QString //返回用户选中的文件路径
QFileDialog::getSaveFileName( //函数名
QWidget *parent = nullptr, //父组件
const QString &caption = QString(), //对话框标题
const QString &dir = QString(), //遍历文件系统时的起始路径
const QString &filter = QString()) //过滤器
五、QT中的io操作
1、依赖的类是QFile
2、使用QFile类实例化对象,用该对象对文件进行读写操作
3、可以使用构造函数打开文件,也可以调用无参构造,使用open函数打开文件
4、文件读写函数,read、readLine、readAll 、write
5、关闭文件close
仿文本编辑器
ui
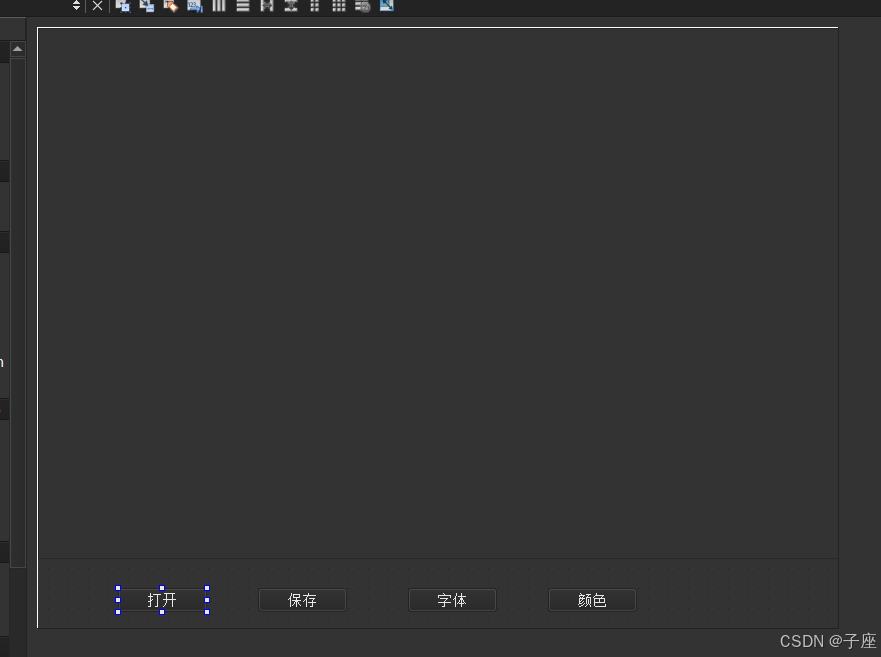
槽函数
cpp
#include "widget.h"
#include "ui_widget.h"
#include <QColor>//颜色类
#include <QColorDialog>//颜色对话框
#include <QMessageBox>//对话框
#include <QFontDialog>//字体对话框
#include <QFont>//字体类
#include <QFileDialog>//文件对话框
#include <QFile>//文件类
#include <QDebug>//信息调试类
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
}
Widget::~Widget()
{
delete ui;
}
//颜色
void Widget::on_pushButton_4_clicked()
{
QColor c = QColorDialog::getColor(QColor(255,0,0),//初始颜色
this,//父组件
"颜色");//对话框标题
//对选择的颜色进行判断
if(c.isValid())
{
ui->textEdit->setTextColor(c);
}else
{
QMessageBox::information(this,"提示","未选择颜色");
}
}
//字体
void Widget::on_pushButton_3_clicked()
{
bool ok = false; // 定义变量用于接收操作
QFont f = QFontDialog::getFont(&ok,//接收用户操作
QFont("隶书",10,10),//初始字体
this,//父组件
"选择字体"//对话框标题
);
//判断用户操作
if(ok)
{
//选中了某个字体
//ui->textEdit->setFont(f);//设置所有文本
ui->textEdit->setCurrentFont(f);//设置选中文本
ui->textEdit->setFontItalic(true);//斜体
}else
{
QMessageBox::information(this,"提示","未选择字体");
}
}
//保存
void Widget::on_pushButton_2_clicked()
{
QString fileName = QFileDialog::getSaveFileName(this,//父组件
"保存",//对话框标题
"./",//起始目录
"所有文件(*.*);;图片(*.png *.jpg *.gif);;文本(*.txt);;原文件(*.cpp)");//过滤器
//输出选择的文件
qDebug()<<fileName;
//QT中的文件操作
QFile file(fileName);//使用得到的文件路径创建员工文件对象
//以写的形式打开文件
if(!file.open(QFile::WriteOnly|QFile::Text|QFile::Truncate))
{ // 写 以文本的形式 清空
//打开失败
QMessageBox::warning(this,"警告","创建失败");
return ;
}
//打开成功
//获取ui界面上文本编辑器上的类容
QString msg = ui->textEdit->toPlainText();
//将信息写入文件
file.write(msg.toLocal8Bit());
//QSring -> 字节数组
//关闭文件
file.close();
}
//打开
void Widget::on_pushButton_clicked()
{
QString fileName = QFileDialog::getOpenFileName(this,"打开",
"./",
"所有文件(*.*);;图片(*.png *.jpg *.gif);;文本(*.txt);;原文件(*.cpp)");
QFile file(fileName);
//读的形式打开
if(!file.open(QFile::ReadOnly|QFile::Text))
{
//打开失败
QMessageBox::warning(this,"警告","打开失败");
return ;
}
//获取信息
QString msg = file.readAll();
//转换格式
msg.toStdString();
//显示文本
ui->textEdit->setText(msg);
//关闭文件
file.close();
}