注释很详细,直接上代码
项目结构
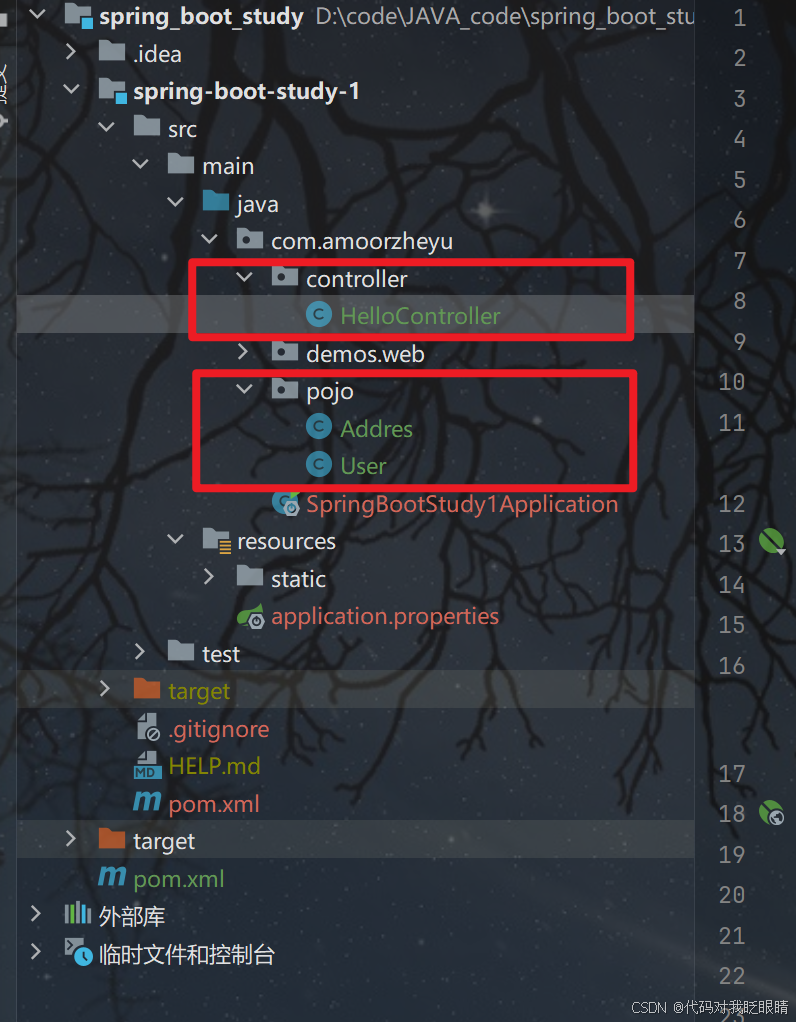
源码
HelloController
java
package com.amoorzheyu.controller;
import com.amoorzheyu.pojo.User;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.*;
import java.time.LocalDateTime;
import java.util.Arrays;
import java.util.List;
/* 注解标识请求处理类 */
@RestController
public class HelloController {
/* 标识请求路径 */
//test
@RequestMapping( "/hello")
public String hello(){
System.out.println("hello world~");
return "hello world~";
}
//简单参数的接收---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
/*
* 直接接收名字需要一一对应,若无则为null */
@RequestMapping( "/simpleParameter1")
public String hello2(String name, Integer age){
String str = "hello world~" + name+",age:"+age;
System.out.println(str);
return str;
}
/*
* 可使用RequestParam修改变量映射名,默认为必须,如果未传则会报错
* @value:映射的变量名
* required:是否必须,默认为true
* defaultValue:默认值
* */
@RequestMapping( "/simpleParameter2")
public String hello3(@RequestParam(value = "name111", defaultValue = "unknown user",required = false) String name,
@RequestParam(value = "age111", defaultValue = "18",required = false) Integer age){
String str = "hello world~" + name+",age:"+age;
System.out.println(str);
return str;
}
//实体参数--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
/* 简单实体参数,需要字段名一一对应 */
@RequestMapping( "/entityParameter1")
public String hello4(User user){
String str = "hello world~" + user.getName()+",age:"+user.getAge();
System.out.println(str);
return str;
}
/* 复杂实体参数,同理,传值为addres.province的形式 */
@RequestMapping( "/entityParameter2")
public String hello5(User user){
String str = "hello world~" + user.getName()+",age:"+user.getAge()+",province:"+user.getAddres().getProvince()+",city:"+user.getAddres().getCity();
System.out.println(str);
return str;
}
//数组集合参数----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
/* 数组参数,请求参数名与形参中数组变量名相同,可以直接使用数组封装 */
@RequestMapping( "/arrayParameter1")
public String hello6(String[] name){
System.out.println(Arrays.toString(name));
return Arrays.toString(name);
}
/* 集合参数,请求参数名与形参中集合变量名相同,通过@RequestParam绑定参数关系 */
@RequestMapping( "/arrayParameter2")
public String hello7(@RequestParam List<String> hobby){
System.out.println(hobby);
return hobby.toString();
}
//日期时间类型参数--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
@RequestMapping( "/dateParameter")
public String hello8(@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") LocalDateTime updateTime){
System.out.println(updateTime);
return updateTime.toString();
}
//json类型参数----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
@RequestMapping( "/jsonParameter")
public String hello9(@RequestBody User user){
System.out.println(user);
return user.toString();
}
//路径参数----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
@RequestMapping(value = "/pathVariable/{id}/{type}")
public String hello10(@PathVariable("id") String id, @PathVariable("type") String type){
System.out.println(id+" "+type);
return id+" "+type;
}
}
Address
java
package com.amoorzheyu.pojo;
public class Address {
private String province;
private String city;
@Override
public String toString() {
return "Addres{" +
"province='" + province + '\'' +
", city='" + city + '\'' +
'}';
}
public String getProvince() {
return province;
}
public void setProvince(String province) {
this.province = province;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
}
User
java
package com.amoorzheyu.pojo;
public class User {
private Integer age;
private String name;
private Address address;
public Address getAddres() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "User{" +
"age=" + age +
", name='" + name + '\'' +
", addres=" + address +
'}';
}
}