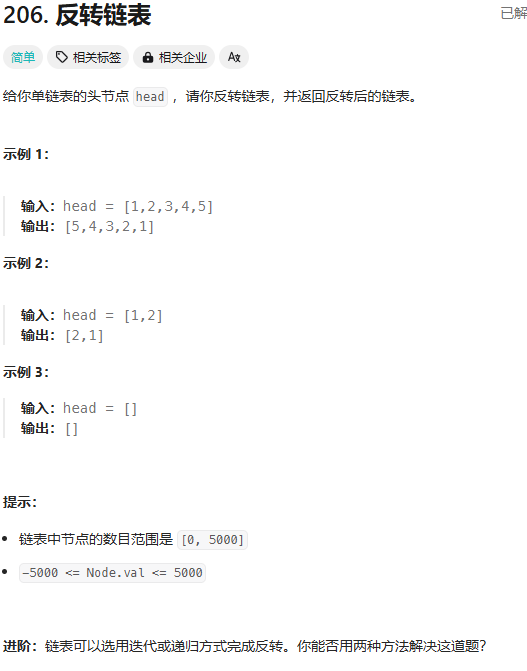
class Solution {
public:
ListNode* reverseList(ListNode* head) {
ListNode* temp;
ListNode* cur = head;
ListNode* pre =nullptr;
while(cur != nullptr){
temp= cur->next;
cur->next =pre;
pre = cur;
cur = temp;
}
return pre;
}
};
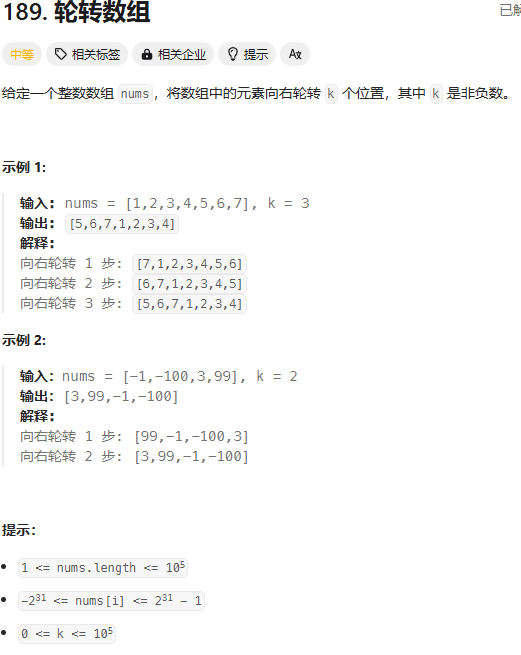
class Solution {
public:
void rotate(vector<int>& nums, int k) {
int n = nums.size();
vector<int> newArr(n);
for(int i=0;i<n;++i)
{
newArr[(i+k)%n] = nums[i];
}
nums.assign(newArr.begin(),newArr.end());
}
};
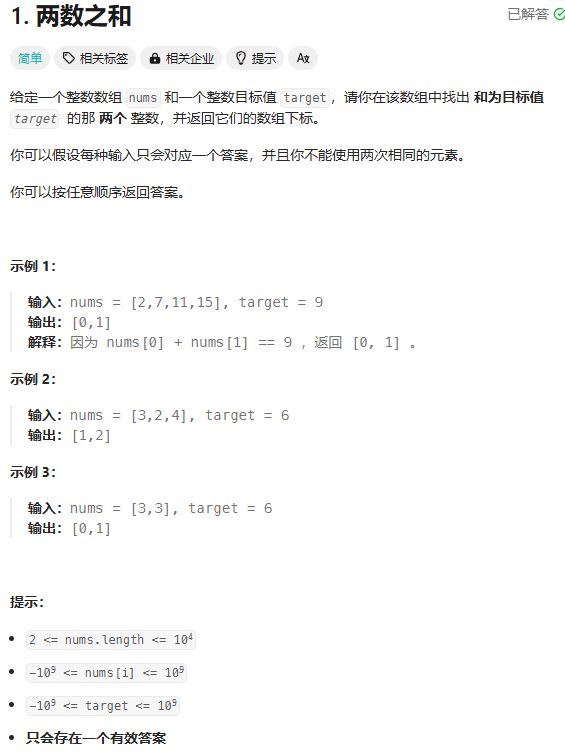
class Solution {
public:
vector<int> twoSum(vector<int>& nums, int target) {
unordered_map<int,int> hashtable;
for(int i=0;i<nums.size();i++){
auto it = hashtable.find(target - nums[i]);
if(it != hashtable.end()){
return{it->second,i};
}
hashtable[nums[i]] = i;
}
return{};
}
};
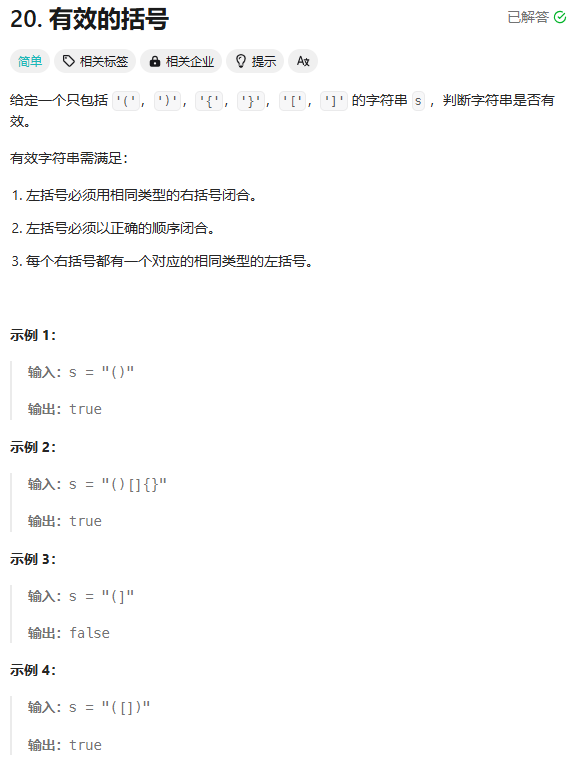
class Solution {
public:
bool isValid(string s) {
if(s.size() % 2 !=0) return false;
stack<char>st;
for(int i = 0;i<s.size();i++){
if(s[i]=='(')st.push(')');
else if(s[i]=='[')st.push(']');
else if(s[i]=='{')st.push('}');
else if(st.empty()||st.top() != s[i]) return false;
else st.pop();
}
return st.empty();
}
};
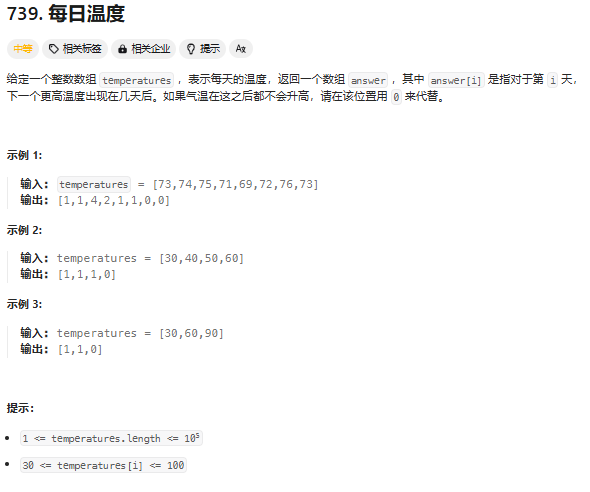
class Solution {
public:
vector<int> dailyTemperatures(vector<int>& temperatures) {
int n = temperatures.size();
vector<int> ans(n);
stack<int> s;
for (int i = 0; i < n; ++i) {
while (!s.empty() && temperatures[i] > temperatures[s.top()]) {
int previousIndex = s.top();
ans[previousIndex] = i - previousIndex;
s.pop();
}
s.push(i);
}
return ans;
}
};
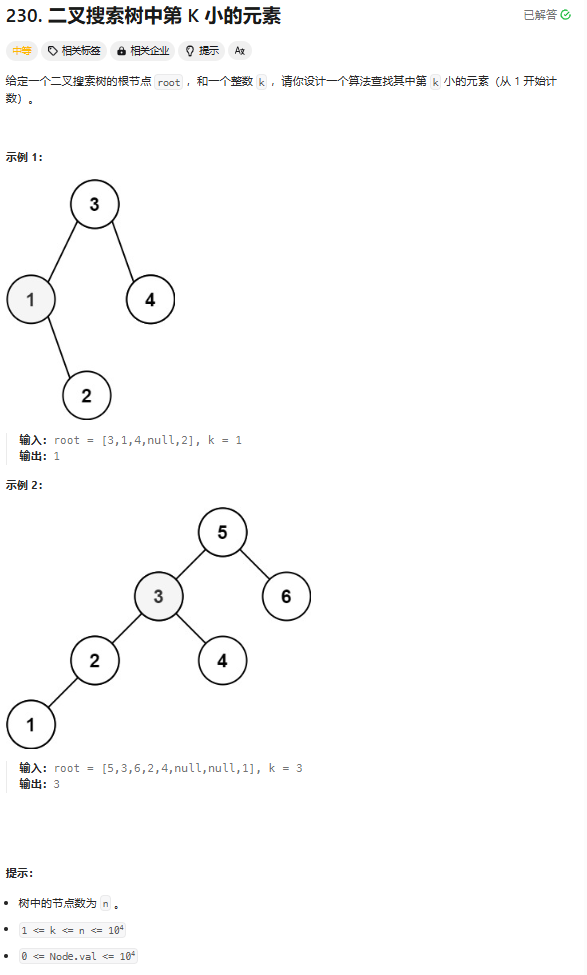
class Solution {
public:
int kthSmallest(TreeNode* root, int k) {
stack<TreeNode*>stack;
while(stack.size() > 0 || root !=nullptr){
while(root!= nullptr){
stack.push(root);
root = root->left;
}
root = stack.top();
stack.pop();
--k;
if(k == 0){
break;
}
root = root->right;
}
return root->val;
}
};
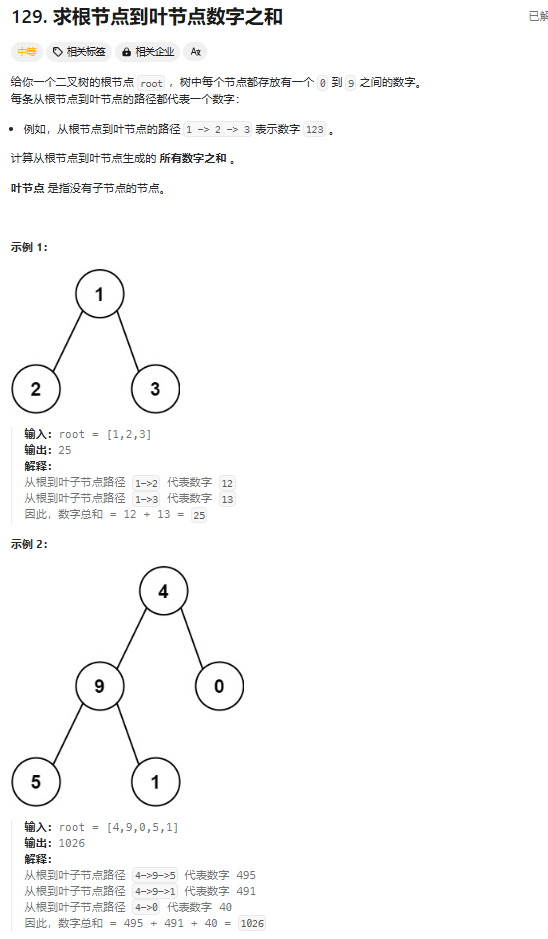
class Solution {
public:
int dfs(TreeNode* root, int preSum){
if(root == nullptr){
return 0;
}
int sum = preSum * 10 + root->val;
if(root->left == nullptr && root->right == nullptr){
return sum;
}else{
return dfs(root->left,sum) + dfs(root->right,sum);
}
}
int sumNumbers(TreeNode* root) {
return dfs(root,0);
}
};
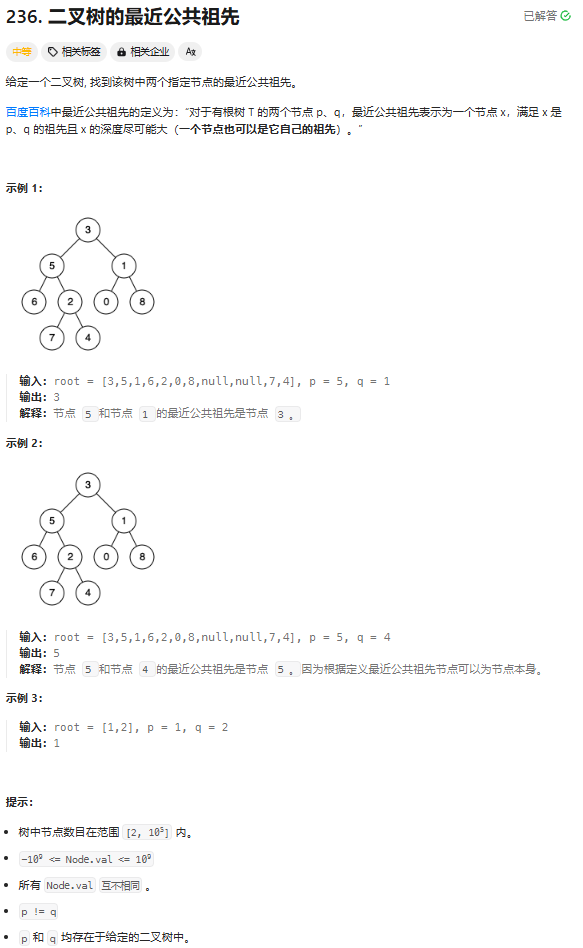
class Solution {
public:
TreeNode* lowestCommonAncestor(TreeNode* root, TreeNode* p, TreeNode* q) {
if (root == q || root == p || root == NULL) return root;
TreeNode* left = lowestCommonAncestor(root->left, p, q);
TreeNode* right = lowestCommonAncestor(root->right, p, q);
if (left != NULL && right != NULL) return root;
if (left == NULL) return right;
return left;
}
};
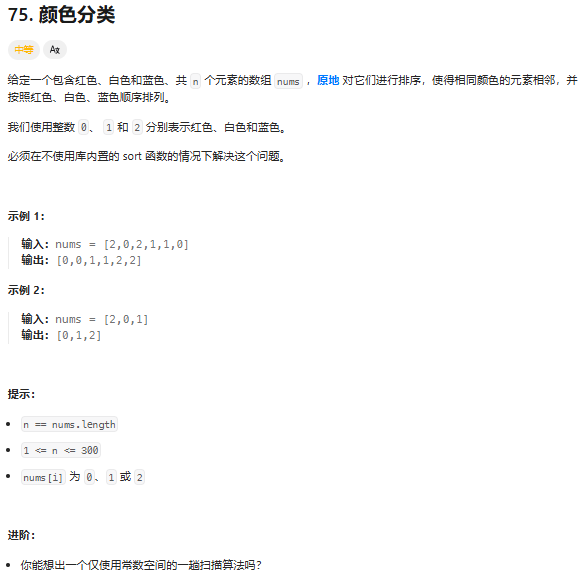
class Solution {
public:
void sortColors(vector<int>& nums) {
int n = nums.size();
int ptr = 0;
for(int i=0;i<n;i++){
if(nums[i]==0){
swap(nums[i],nums[ptr]);
ptr++;
}
}
for(int i = ptr;i<n;i++){
if(nums[i] == 1){
swap(nums[i],nums[ptr]);
ptr++;
}
}
}
};
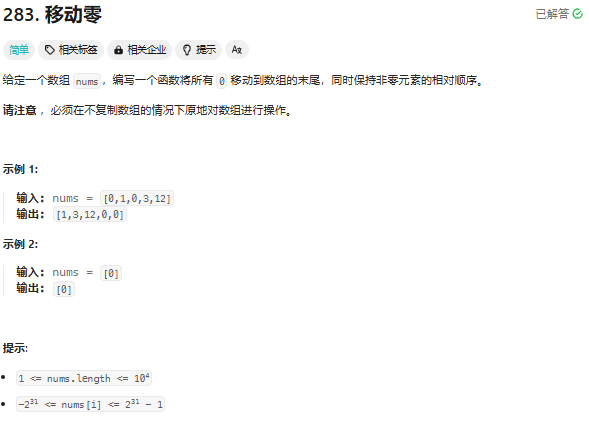
class Solution {
public:
void moveZeroes(vector<int>& nums) {
int n = nums.size(),right = 0,left = 0;
while(right < n){
if(nums[right]!=0){
swap(nums[left],nums[right]);
left++;
}
right++;
}
}
};
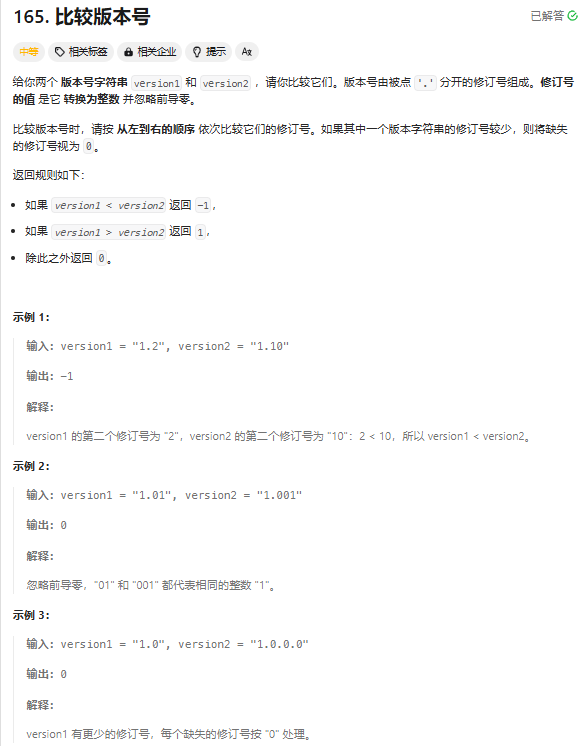
class Solution {
public:
int compareVersion(string version1, string version2) {
int n = version1.length(), m = version2.length();
int i = 0,j = 0;
while(i <n||j<m){
int x =0;
for(;i<n&&version1[i]!='.';i++){
x= x*10-'0'+version1[i];
}
i++;
int y=0;
for(;j<m&&version2[j]!='.';j++){
y = y*10-'0'+version2[j];
}
j++;
if(x!=y){
return x > y ? 1 : -1;
}
}
return 0;
}
};
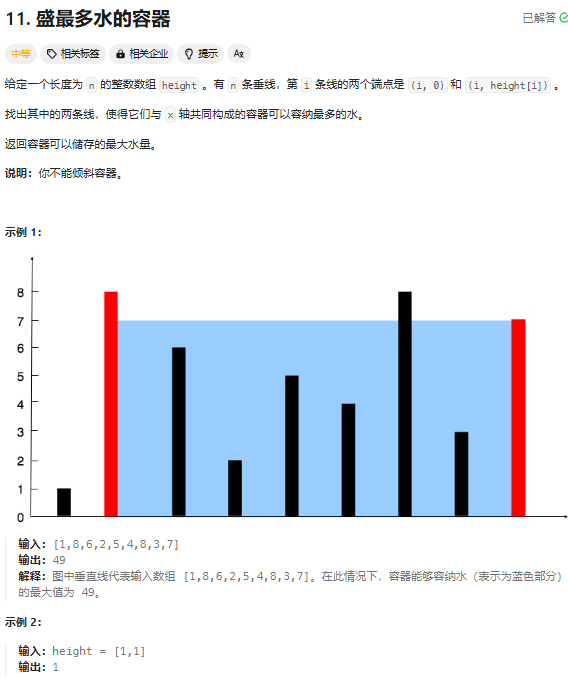
class Solution {
public:
int maxArea(vector<int>& height) {
int l = 0, r = height.size()-1;
int ans = 0;
while(l < r){
int area = min(height[l],height[r]) * (r-l);
ans = max(ans,area);
if(height[l] <= height[r]){
l++;
}
else{
r--;
}
}
return ans;
}
};
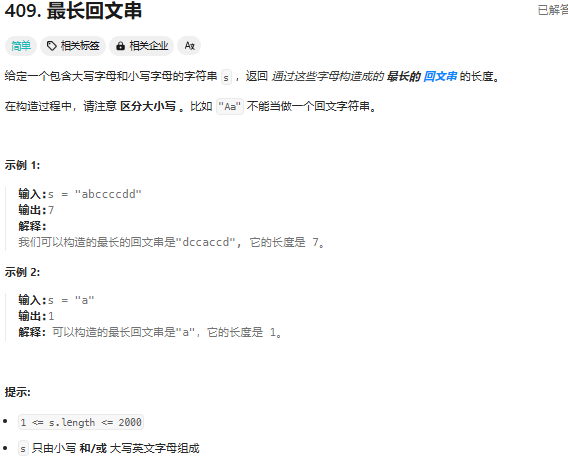
class Solution {
public:
int longestPalindrome(string s) {
unordered_map<char,int>count;
int ans = 0;
for(char c:s)
++count[c];
for(auto p:count){
int v = p.second;
ans += v/2 *2;
if(v% 2==1 and ans %2 ==0)
++ans;
}
return ans;
}
};
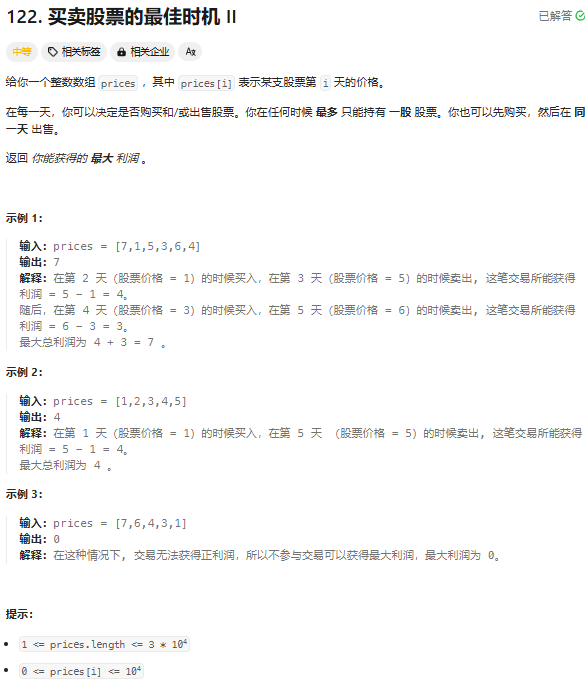
class Solution {
public:
int maxProfit(vector<int>& prices) {
int profit = 0;
for(int i=1;i<prices.size();i++){
int tmp = prices[i] - prices[i-1];
if(tmp > 0)
profit += tmp;
}
return profit;
}
};
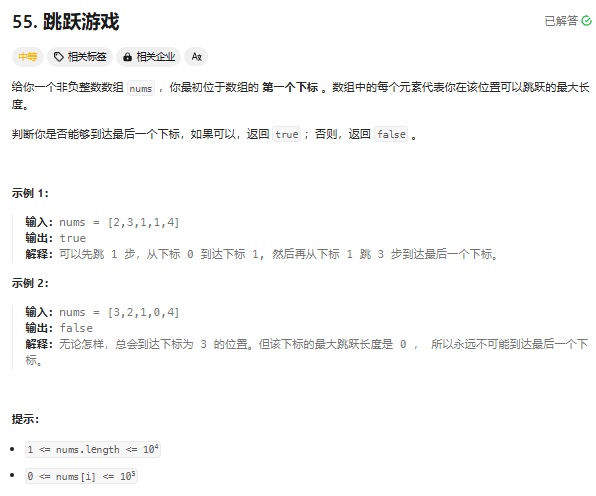
class Solution {
public:
bool canJump(vector<int>& nums) {
int n = nums.size();
int rightmost = 0;
for(int i=0;i<n;i++){
if(i<=rightmost){
rightmost = max(rightmost,i+nums[i]);
if(rightmost >= n-1){
return true;
}
}
}
return false;
}
};
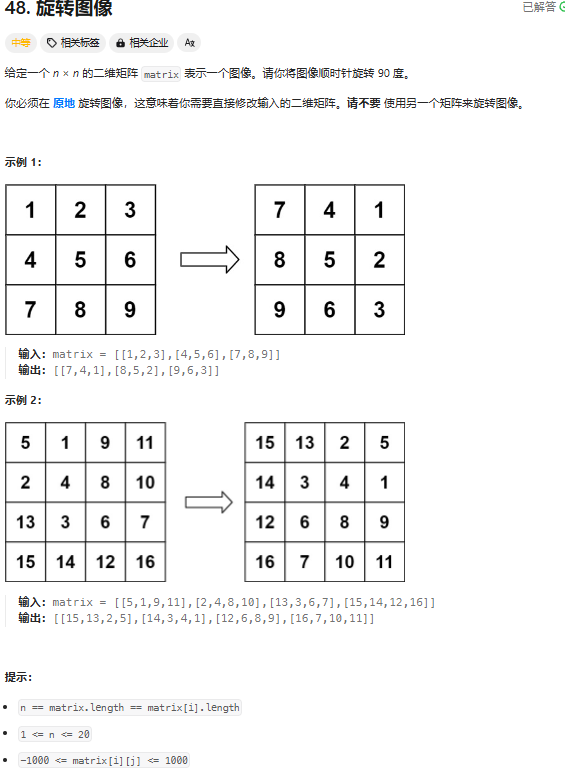
class Solution {
public:
void rotate(vector<vector<int>>& matrix) {
int n = matrix.size();
for(int i=0;i<n / 2;i++){
for(int j=0;j<n;j++){
swap(matrix[i][j],matrix[n-i-1][j]);
}
}
for(int i=0;i<n;i++){
for(int j=0;j<i;j++){
swap(matrix[i][j],matrix[j][i]);
}
}
}
};
17.排序数组查找第一个和最后一个位置
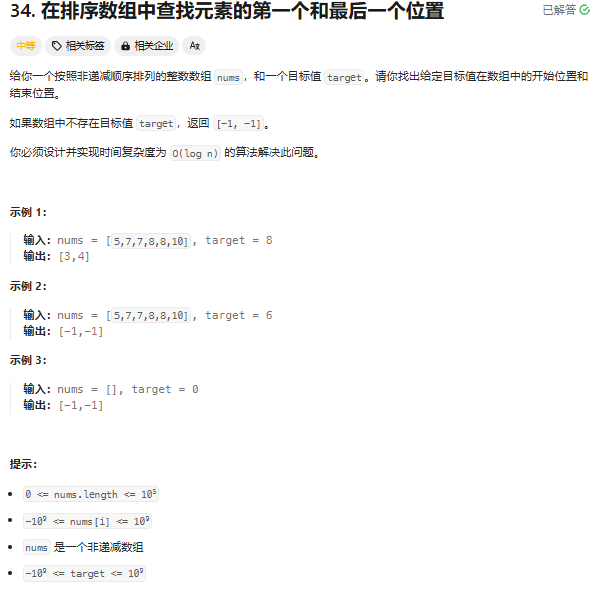
class Solution {
public:
int binarySearch(vector<int>& nums, int target, bool lower) {
int left = 0, right = (int)nums.size() - 1, ans = (int)nums.size();
while (left <= right) {
int mid = (left + right) / 2;
if (nums[mid] > target || (lower && nums[mid] >= target)) {
right = mid - 1;
ans = mid;
} else {
left = mid + 1;
}
}
return ans;
}
vector<int> searchRange(vector<int>& nums, int target) {
int leftIdx = binarySearch(nums, target, true);
int rightIdx = binarySearch(nums, target, false) - 1;
if (leftIdx <= rightIdx && rightIdx < nums.size() && nums[leftIdx] == target && nums[rightIdx] == target) {
return vector<int>{leftIdx, rightIdx};
}
return vector<int>{-1, -1};
}
};
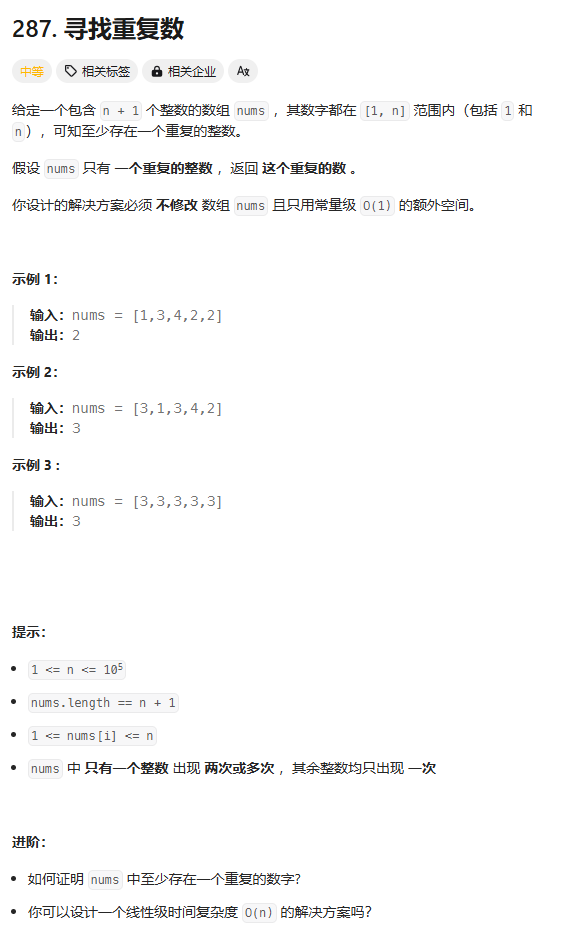
巧妙的使用二分查找法可以
class Solution {
public:
int findDuplicate(vector<int>& nums) {
int n = nums.size();
int l = 1, r = n - 1, ans = -1;
while (l <= r) {
int mid = (l + r) >> 1;
int cnt = 0;
for (int i = 0; i < n; ++i) {
cnt += nums[i] <= mid;
}
if (cnt <= mid) {
l = mid + 1;
} else {
r = mid - 1;
ans = mid;
}
}
return ans;
}
};
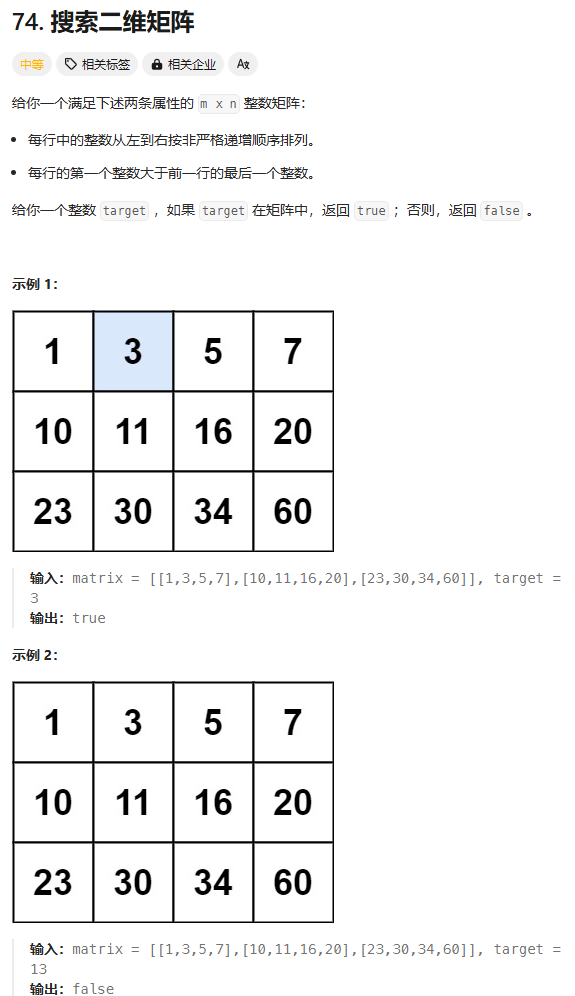
class Solution {
public:
bool searchMatrix(vector<vector<int>>& matrix, int target) {
int m = matrix.size(), n = matrix[0].size();
int low = 0, high = m * n - 1;
while (low <= high) {
int mid = (high - low) / 2 + low;
int x = matrix[mid / n][mid % n];
if (x < target) {
low = mid + 1;
} else if (x > target) {
high = mid - 1;
} else {
return true;
}
}
return false;
}
};
滑动窗口
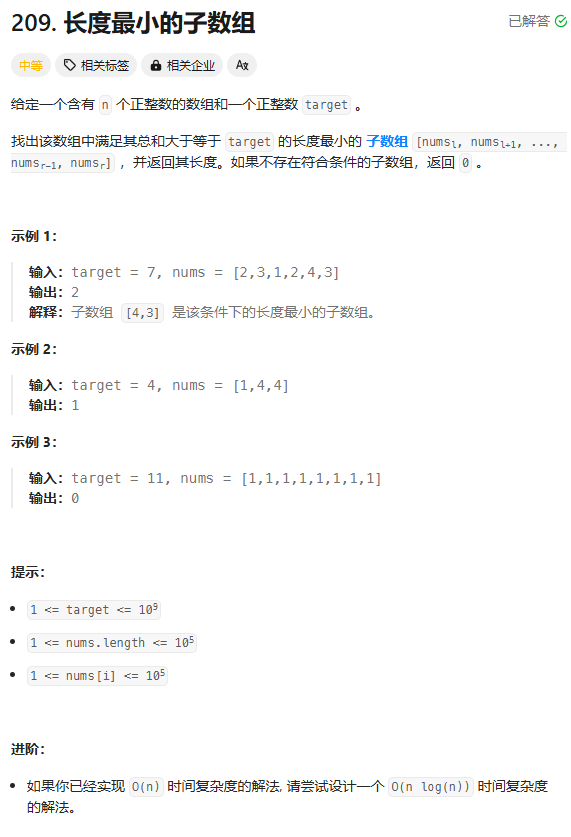
class Solution {
public:
int minSubArrayLen(int target, vector<int>& nums) {
int result = INT32_MAX;
int i=0;
int sum=0;
int subLenth =0;
for(int j=0;j<nums.size();j++)
{
sum+= nums[j];
while(sum>=target)
{
subLenth = (j-i+1);
result = result<subLenth?result :subLenth;
sum-=nums[i++];
}
}
return result == INT32_MAX ? 0 :result;
}
};