1、使用slot站位,不传内容,显示默认值
javascript
//父组件
import SlotChild from './projectConstruction-child/SlotChild.vue'
<div>
<SlotChild></SlotChild>
</div>
//子组件
<template>
<div>下面是插槽内容</div>
<div><slot>我是默认值</slot></div>
</template>

2、使用slot站位,传内容,不显示默认值
javascript
//父组件
import SlotChild from './projectConstruction-child/SlotChild.vue'
<div>
<SlotChild>text</SlotChild>
</div>
//子组件
<template>
<div>下面是插槽内容</div>
<div><slot>我是默认值</slot></div>
</template>
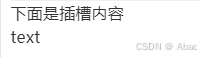
3、多个插槽时为了分清每一个插槽 所以需要 具名插槽 传值显示值,不传显示默认值
javascript
//父组件
<SlotChild>
<template #header>
<h3>text</h3>
</template>
<template>
<h3>foot</h3>
</template>
</SlotChild>
//子组件
<template>
<div>下面是插槽内容</div>
<div><slot name="header">我是默认值</slot></div>
<div><slot name="footer">我是默认值</slot></div>
</template>
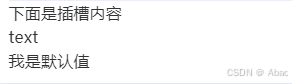
4、作用域插槽
javascript
//父组件 #main="data" 接收slot传来的值
<SlotChild>
<template #header>
<h3>text</h3>
</template>
<template #main="data">
<h3>{{ data }}</h3>
</template>
<template>
<h3>foot</h3>
</template>
</SlotChild>
//子组件 传递数据slotArr
<template>
<div>下面是插槽内容</div>
<div><slot name="header">我是默认值</slot></div>
<div><slot name="main" :arr="slotArr"></slot></div>
<div><slot name="footer">我是默认值</slot></div>
</template>
<script setup lang="ts">
import { ref } from 'vue'
const slotArr = ref(['aaa', 'bbb', 'ccc', 'ddd'])
</script>
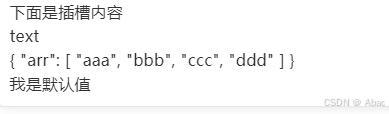
5、遍历数据
javascript
//父组件
<SlotChild>
<template #header>
<h3>text</h3>
</template>
<template #main="data">
<ul>
<li v-for="e in data.arr" :key="e">{{ e }}</li>
</ul>
</template>
<template>
<h3>foot</h3>
</template>
</SlotChild>
//子组件
<template>
<div>下面是插槽内容</div>
<div><slot name="header">我是默认值</slot></div>
<div><slot name="main" :arr="slotArr"></slot></div>
<div><slot name="footer">我是默认值</slot></div>
</template>
<script setup lang="ts">
import { ref } from 'vue'
const slotArr = ref(['aaa', 'bbb', 'ccc', 'ddd'])
</script>
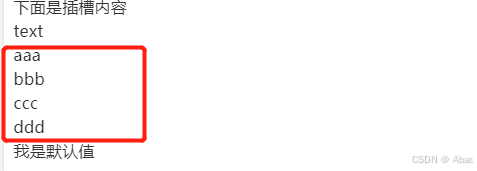