注释很详细,直接上代码
三层架构
- 项目结构
源码:
HelloController
java
package com.amoorzheyu.controller;
import com.amoorzheyu.pojo.User;
import com.amoorzheyu.service.HelloService;
import com.amoorzheyu.service.impl.HelloServiceA;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.*;
import java.time.LocalDateTime;
import java.util.Arrays;
import java.util.List;
/* 注解标识请求处理类 */
@RestController
public class HelloController {
private HelloService helloService = new HelloServiceA();
/* 标识请求路径 */
//test
@RequestMapping( "/hello")
public String hello(){
List<User> list =helloService.getUserList() ;
return list.toString();
}
}
HelloDao
java
package com.amoorzheyu.dao;
import com.amoorzheyu.pojo.User;
import java.util.List;
public interface HelloDao {
//获取用户信息列表
public List<User> getUserList();
}
HelloDaoA
java
package com.amoorzheyu.dao.impl;
import com.amoorzheyu.dao.HelloDao;
import com.amoorzheyu.pojo.User;
import java.util.ArrayList;
import java.util.List;
public class HelloDaoA implements HelloDao {
@Override
public List<User> getUserList() {
//调用dao获取数据
List<User> ReturnList = new ArrayList<User>();
//模拟数据
ReturnList.add(new User("Amoor", 18,null));
ReturnList.add(new User("Bob", 19,null));
ReturnList.add(new User("Candy", 20,null));
return ReturnList;
}
}
HelloService
java
package com.amoorzheyu.service;
import com.amoorzheyu.pojo.User;
import java.util.List;
public interface HelloService {
//获取信息列表
public List<User> getUserList();
}
HelloServiceA
java
package com.amoorzheyu.service.impl;
import com.amoorzheyu.dao.HelloDao;
import com.amoorzheyu.dao.impl.HelloDaoA;
import com.amoorzheyu.pojo.User;
import com.amoorzheyu.service.HelloService;
import java.util.List;
public class HelloServiceA implements HelloService {
//newDao实现类
private HelloDao helloDao = new HelloDaoA();
@Override
public List<User> getUserList() {
List<User> ReturnList = helloDao.getUserList();
return ReturnList;
}
}
User
java
package com.amoorzheyu.pojo;
public class User {
private Integer age;
private String name;
private Address address;
public User( String name,Integer age, Address address) {
this.age = age;
this.name = name;
this.address = address;
}
public Address getAddres() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "User{" +
"age=" + age +
", name='" + name + '\'' +
", address=" + address +
'}';
}
}
我们可以发现层级之间在new时耦合了,也就是说改了此处会影响其他地方,其他位置也需要改动,为了解决这些问题我们可以引入以下思想
控制反转
:Inversion Of Control,简称ioc,对象的创建控制权由程序自身转移到外部(容器),这种思想称为控制反转;
依赖注入
:Dependency Injection,简称Dl,容器为应用程序提供运行时,所依赖的资源,称之为依赖注入;
Bean对象
:Oc容器中创建、管理的对象,称之为bean;
解耦
以下修改为去除new的内容+添加控制反转与依赖注入注解
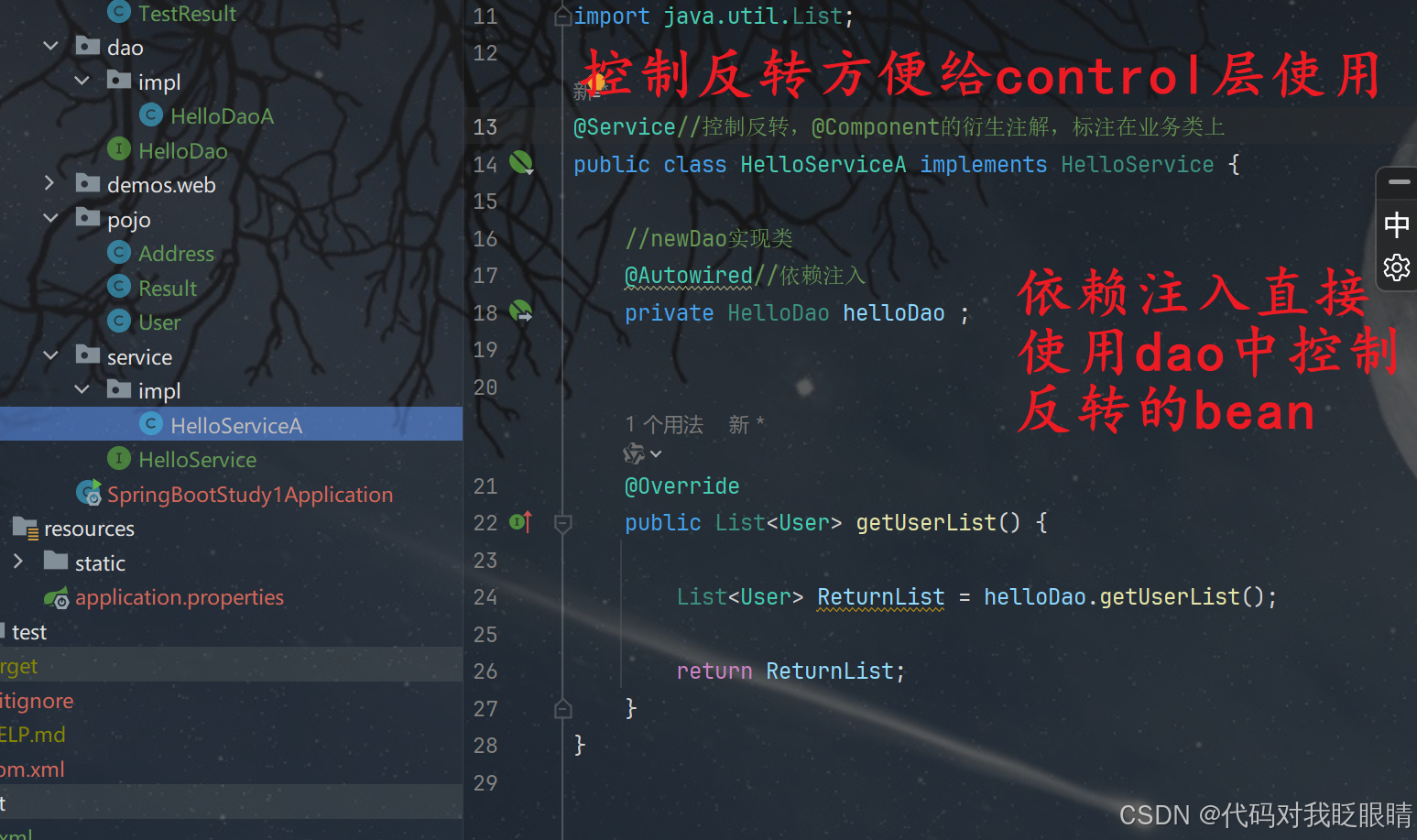
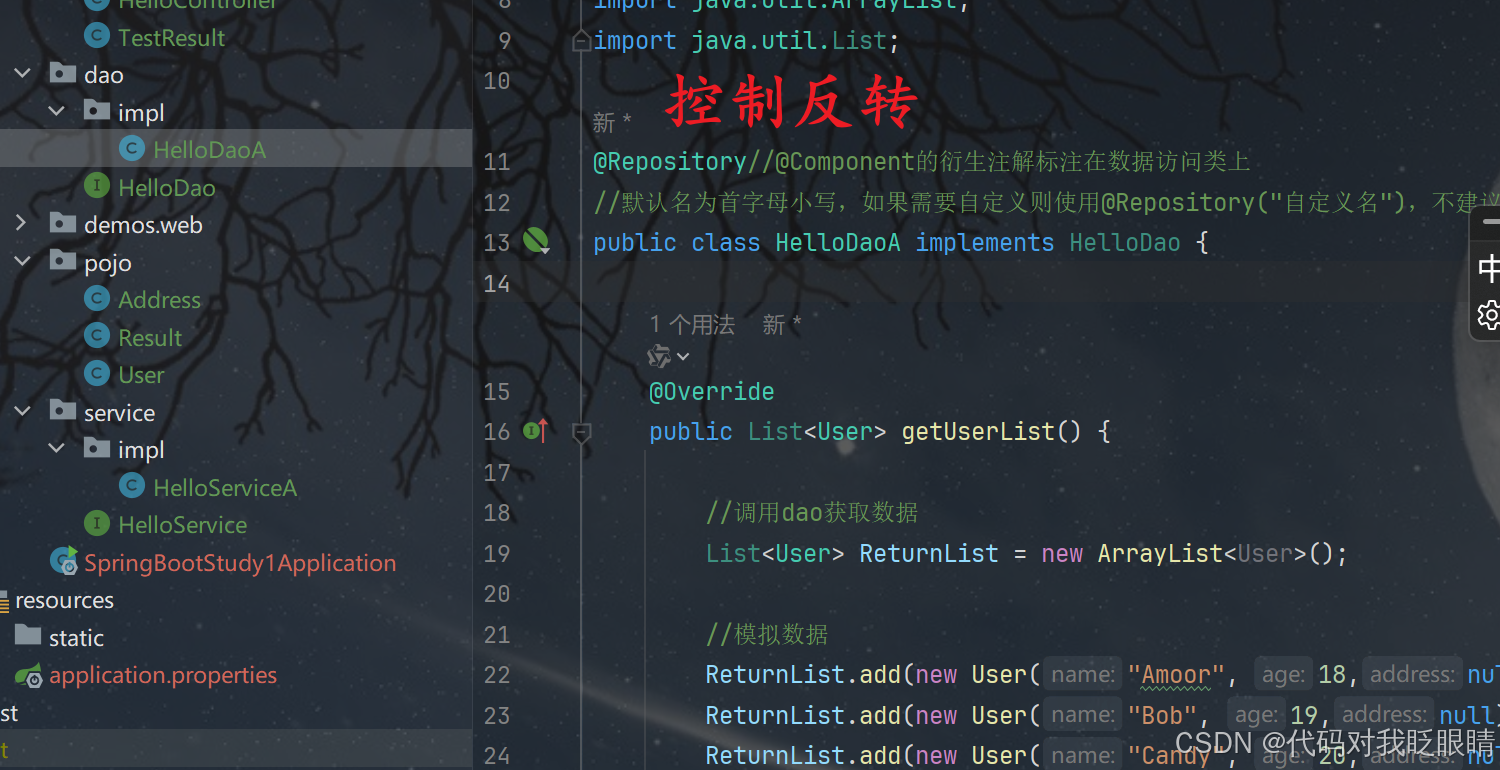