1.思维导图
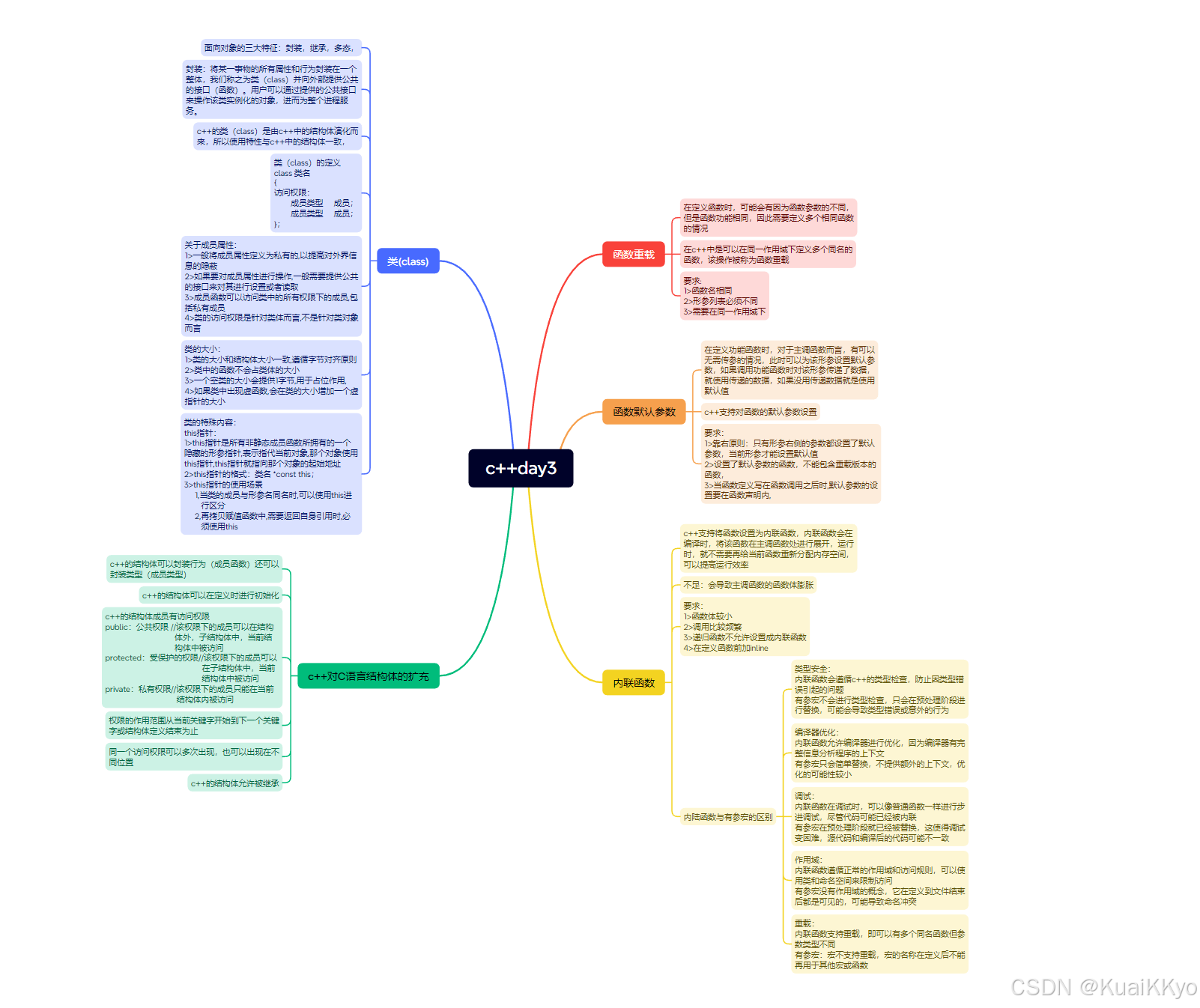
2.顺序表
头文件
#ifndef RECTANGLE_H
#define RECTANGLE_H
#include <iostream>
using namespace std;
using datatype = int ;//类型重定义
class Seqlist
{
private://私有权限
datatype *ptr; //指向堆区申请空间的起始地址
int size;//堆区空间的长度
int len = 0;//顺序表的实际长度
public://公共权限
void init(int );
void menu();
bool menu1();
bool empty();
bool full();
void push_back(datatype);
void show();
void insert();
void erase();
void pop_back();
void Size();
void sort(bool);
void at();
};
#endif // RECTANGLE_H
功能函数
#include"rectangle.h"
void Seqlist::init(int n)
{
this->ptr = new datatype[n];//在堆区申请n个datatype类型的空间
this->len = 0;//对Seqlist的实际长度初始化
this->size = n;//确定Seqlist的最大长度
}
void Seqlist::menu()
{
cout<<"****1.插入***************"<<endl;
cout<<"****2.删除***************"<<endl;
cout<<"****3.尾删***************"<<endl;
cout<<"****4.求长度*************"<<endl;
cout<<"****5.获取任意位置元素***"<<endl;
cout<<"****6.排序***************"<<endl;
cout<<"****7.查看所有元素*******"<<endl;
cout<<"****8.退出***************"<<endl;
int n;
cout<<"请输入:";
cin>>n;
bool flag;
switch(n)
{
case 1:insert();
break;
case 2:erase();
break;
case 3:pop_back();
break;
case 4:Size();
break;
case 5:at();
break;
case 6:flag = menu1();sort(flag);
break;
case 7:show();
break;
case 8:exit(0);
break;
}
}
bool Seqlist::empty()//判空
{
return this->len == 0;//Seqlistd的实际长度为0时返回一个false(假)
}
bool Seqlist::full()//判满
{
return this->len == this->size;//Seqlist的实际长度为size时返回一个true(真)
}
void Seqlist::push_back(datatype e)//尾插
{
if(this->full())//判满
{
cout<<"顺序表已满"<<endl;
return;
}
this->ptr[len++] = e;
}
void Seqlist::show()//查看顺序表内的数据
{
if(this->empty())// 判空
{
cout<<"顺序表为空"<<endl;
return;
}
for(int i = 0;i<this->len;i++)
{
cout<<this->ptr[i]<<" ";
}
cout<<endl;
}
void Seqlist::insert()//任意位置插入
{
if(this->full())//判满
{
cout<<"顺序表已满"<<endl;
return;
}
cout<<"输入插入位置:";
int index;
cin>>index;
cout<<"输入插入数字:";
datatype e;
cin>>e;
this->len++;
for(int i=this->len;i>=index;i--)
{
this->ptr[i]=this->ptr[i-1];
}
this->ptr[index-1] = e;
}
void Seqlist::erase()//任意位置删除
{
if(this->empty())// 判空
{
cout<<"顺序表为空"<<endl;
return;
}
cout<<"输入删除位置:";
int index;
cin>>index;
for(int i=index-1;i<this->len;i++)
{
this->ptr[i]=this->ptr[i+1];
}
this->len--;
}
void Seqlist::pop_back()//尾删
{
if(this->empty())// 判空
{
cout<<"顺序表为空"<<endl;
return;
}
this->ptr[len]=0;
this->len--;
}
void Seqlist::Size()//顺序表长度
{
if(this->empty())// 判空
{
cout<<"顺序表为空"<<endl;
return;
}
cout<<"长度为:"<<this->len<<endl;
}
bool Seqlist::menu1()
{
cout<<"1.升序"<<endl;
cout<<"2.降序"<<endl;
bool flag;
int n;
cin>>n;
if(n==1)
{
flag=true;
}
else
{
flag=false;
}
return flag;
}
void Seqlist::sort(bool flag)//排序
{
if(this->empty())// 判空
{
cout<<"顺序表为空"<<endl;
return;
}
int i,j;
for(i=1;i<this->len;i++)
{
for(j=0;j<this->len-i;j++)
{
if(flag!=0&&this->ptr[j]>this->ptr[j+1])
{
int temp = this->ptr[j];
this->ptr[j]=this->ptr[j+1];
this->ptr[j+1]=temp;
}
else if(flag==0&&this->ptr[j]<this->ptr[j+1])
{
int temp = this->ptr[j];
this->ptr[j]=this->ptr[j+1];
this->ptr[j+1]=temp;
}
}
}
}
void Seqlist::at()//获得数据
{
if(this->empty())// 判空
{
cout<<"顺序表为空"<<endl;
return;
}
int inex;
cout<<"输入获取位置:";
cin>>inex;
if(inex>this->len)
{
cout<<"该位置为空";
}
else
{
cout<<"该位置为"<<this->ptr[inex-1]<<endl;
}
}
主函数
#include"rectangle.h"
int main()
{
Seqlist s1;
s1.init(5);
int arr[3]={1,3,5};
for(int i=0;i<3;i++)
{
s1.push_back(arr[i]);
}
while(1)
{
s1.menu();
}
return 0;
}