Redis是什么
一种数据库 ,但是不是mysql那样的表格,而是key-value 的形式存储,而且它存在内存里,所以读写速度更快。
Redis常用数据类型
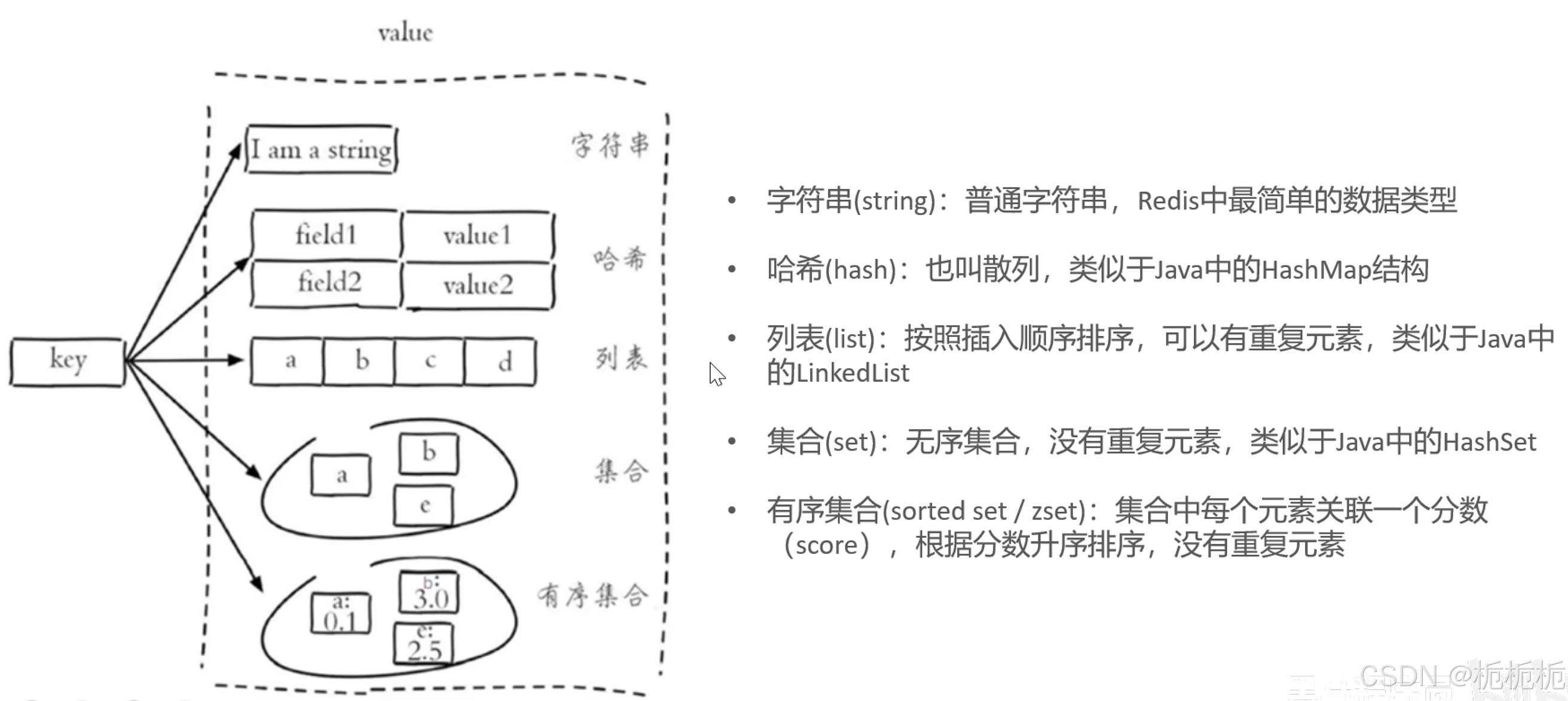
Redis常用命令简单使用
字符串操作
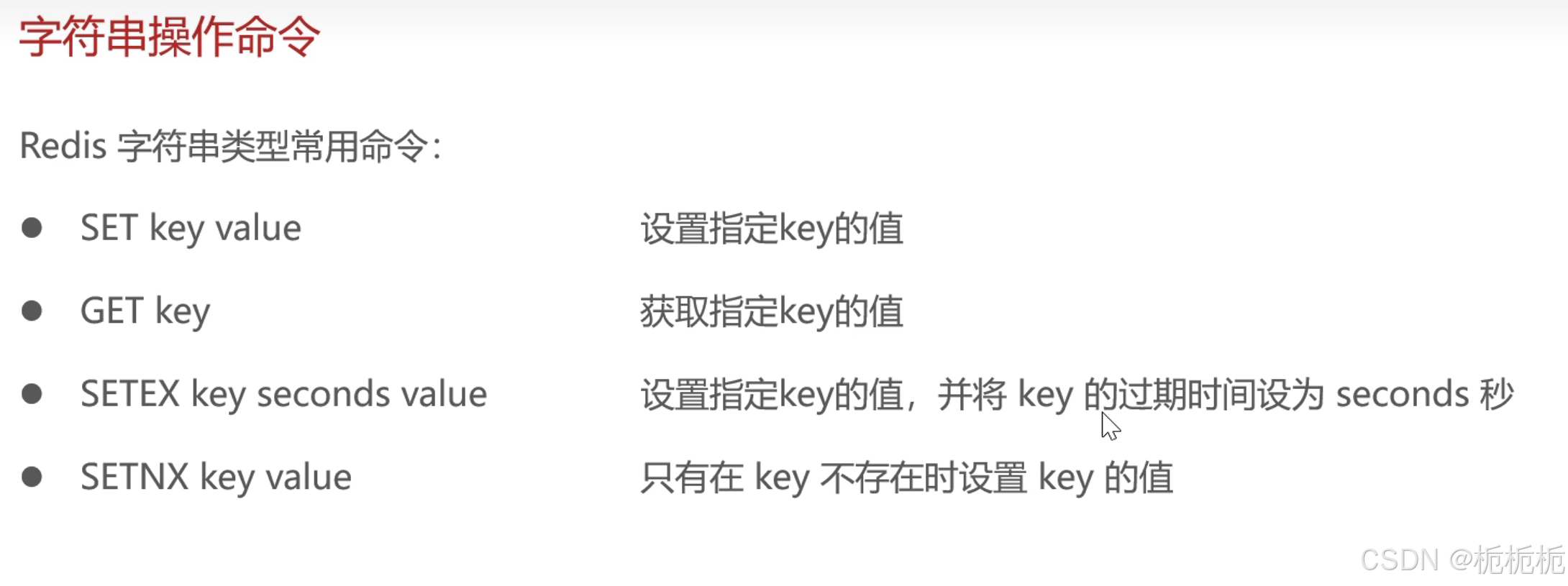
set name x
get name


哈希操作
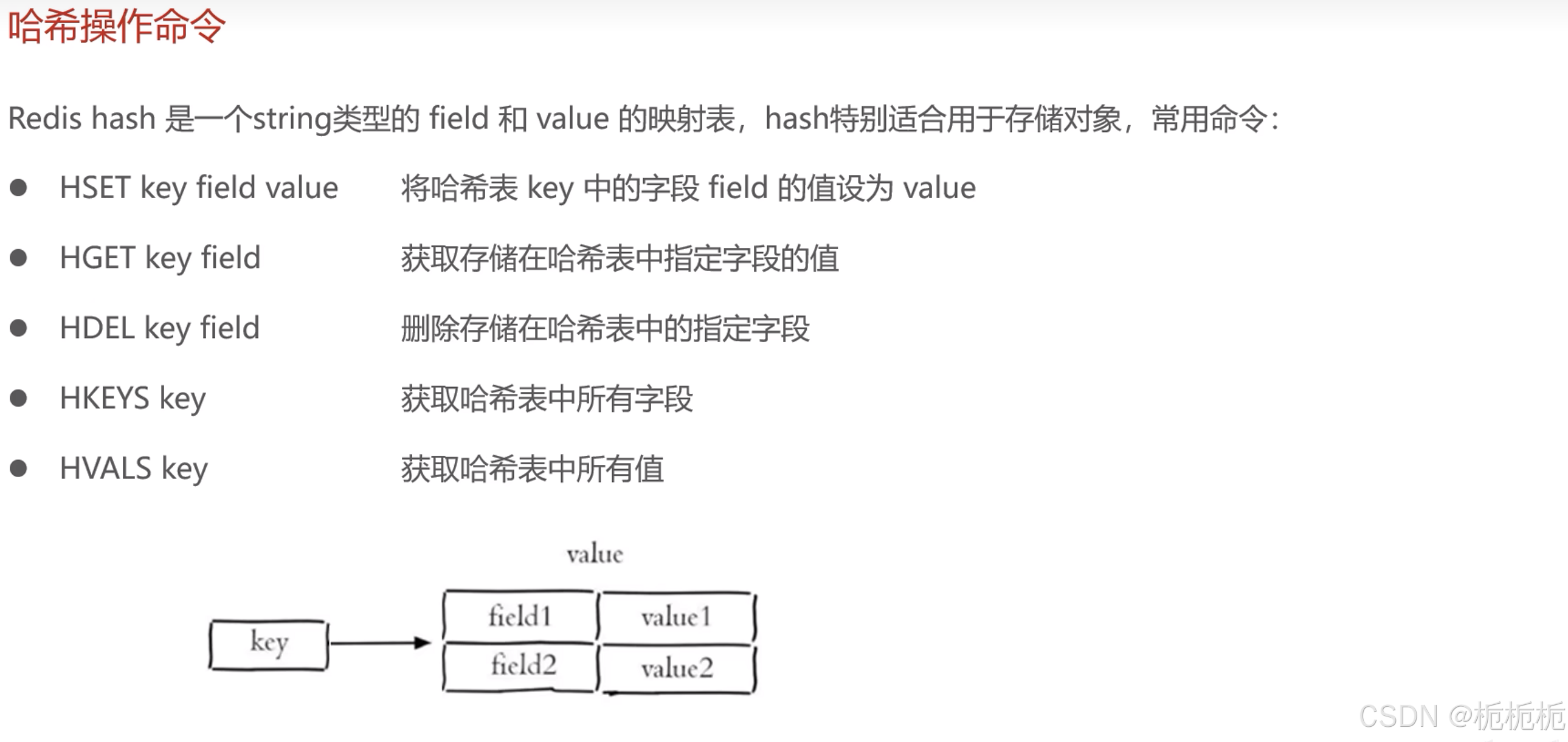
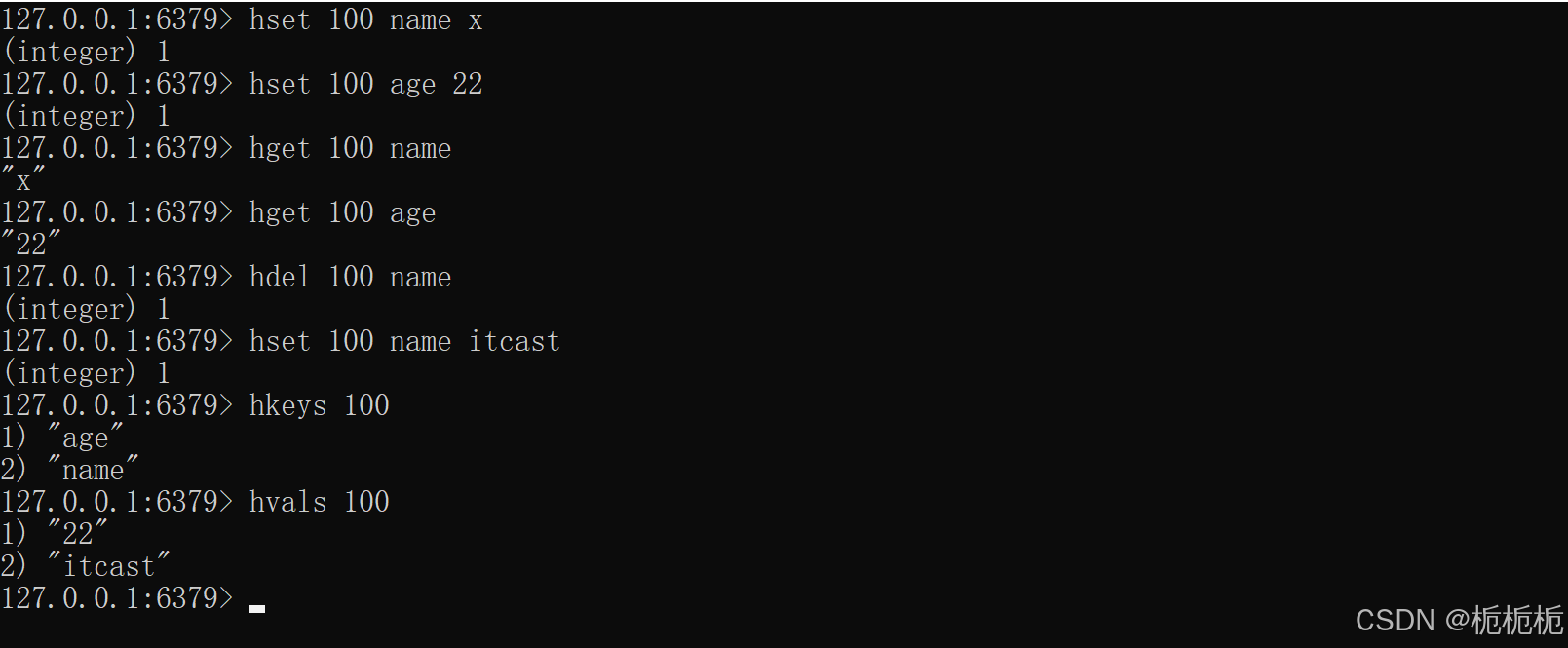
列表操作
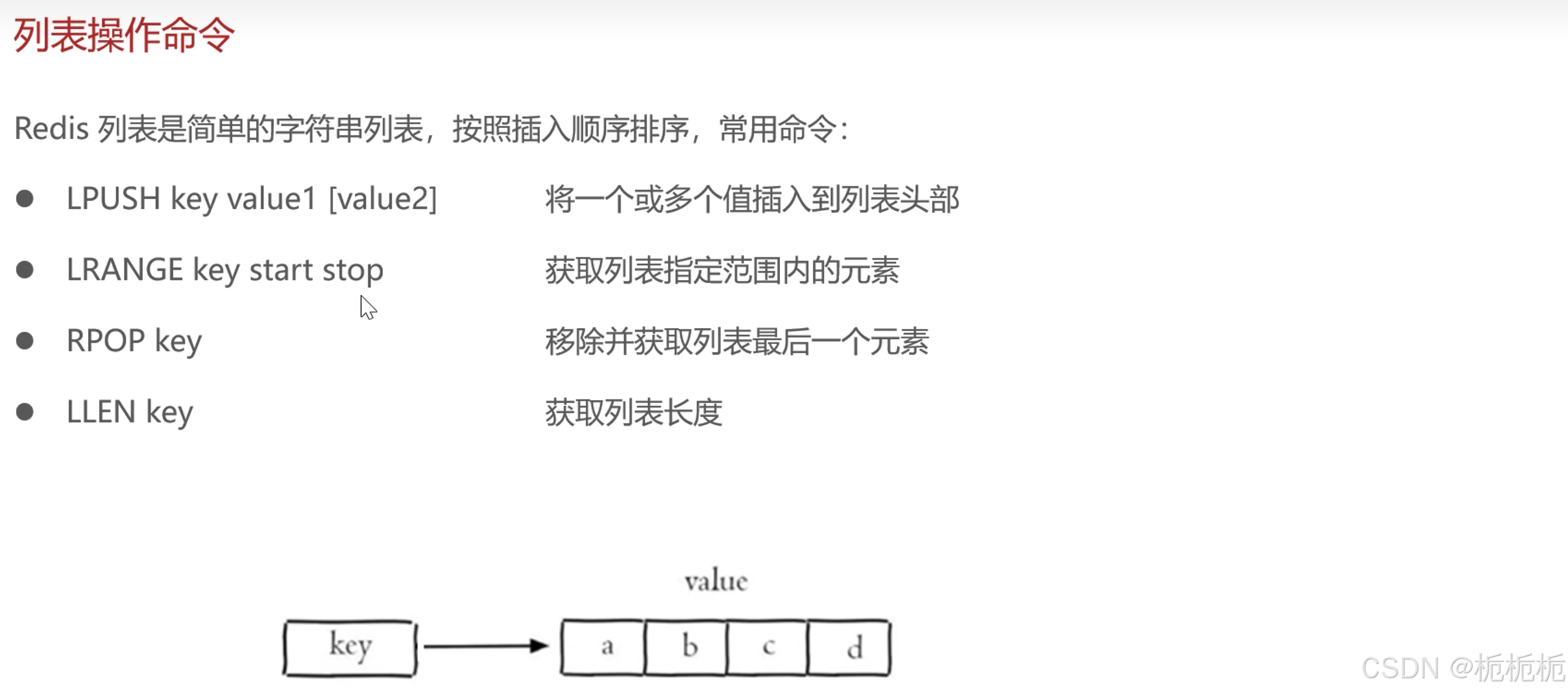
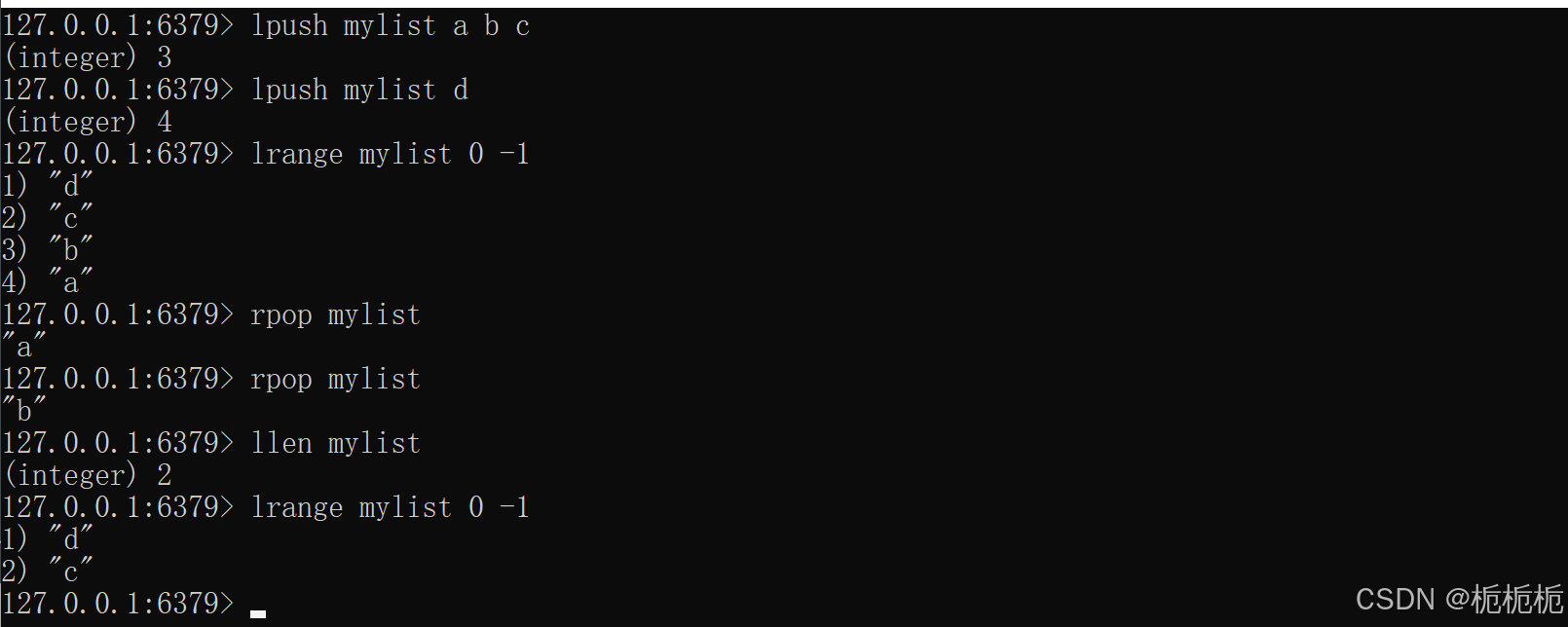
集合操作
有序集合操作
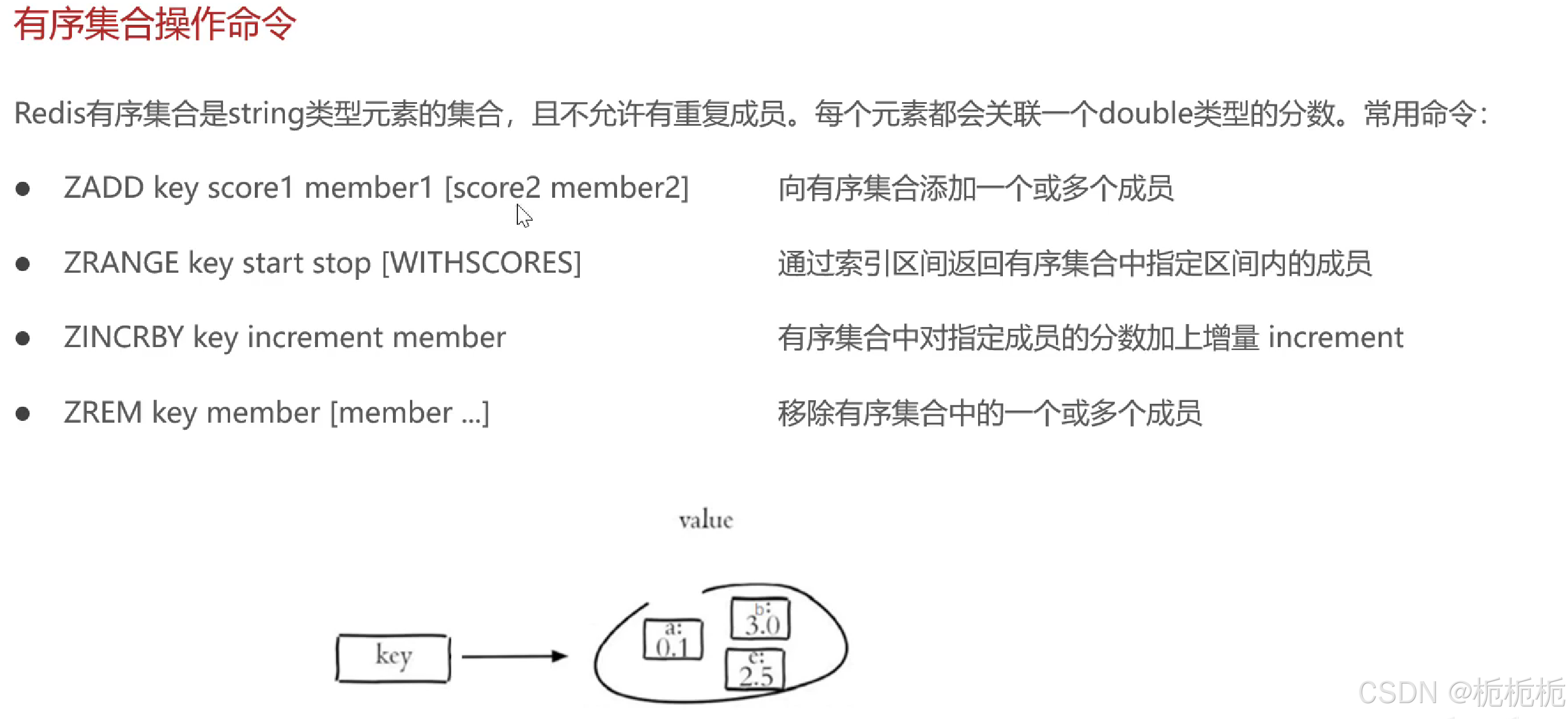
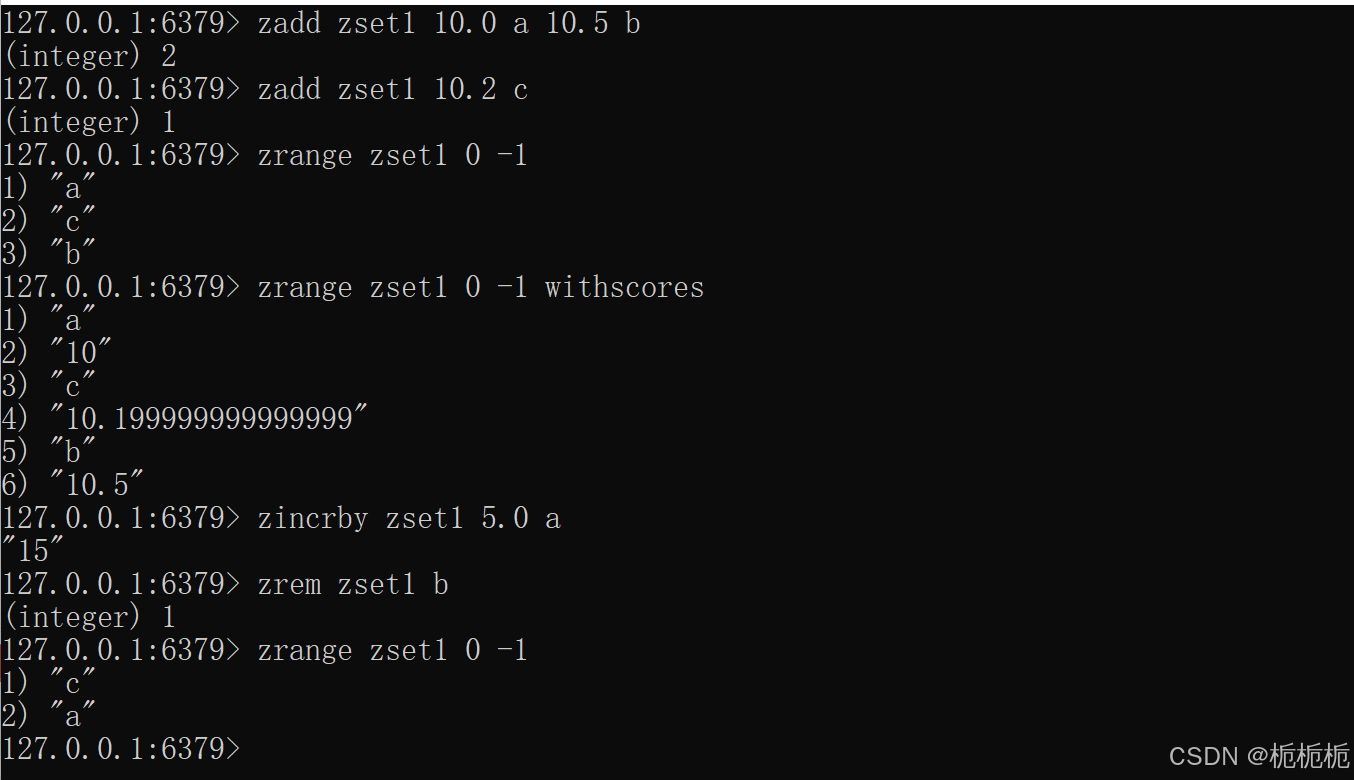
通用操作
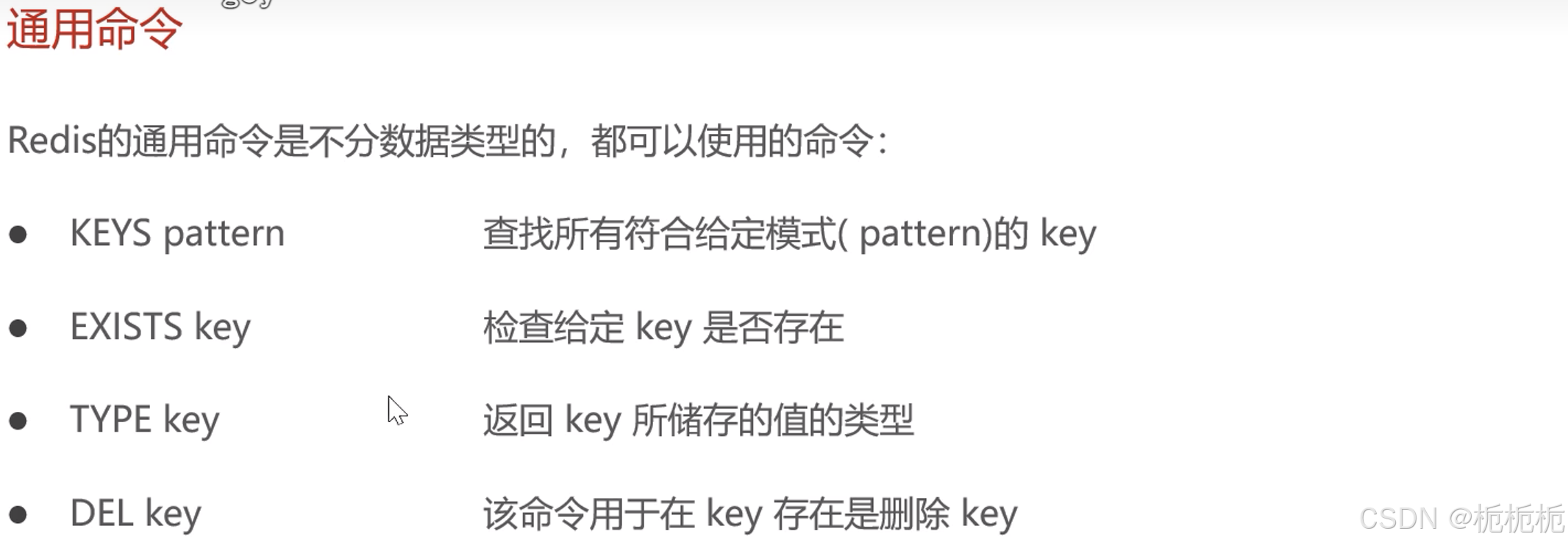
在Java中操作Redis
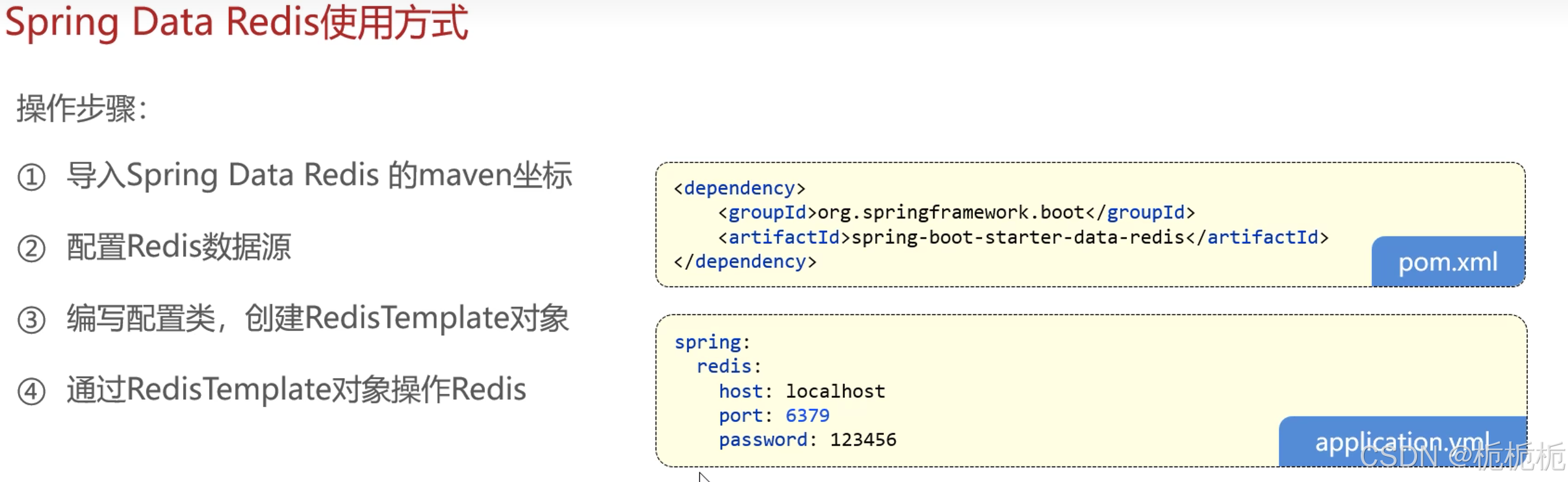
配置Redis数据源
方法1
application.yml下的datasource
java
redis:
host: localhost
port: 6379
database: 0
方法2(推荐)
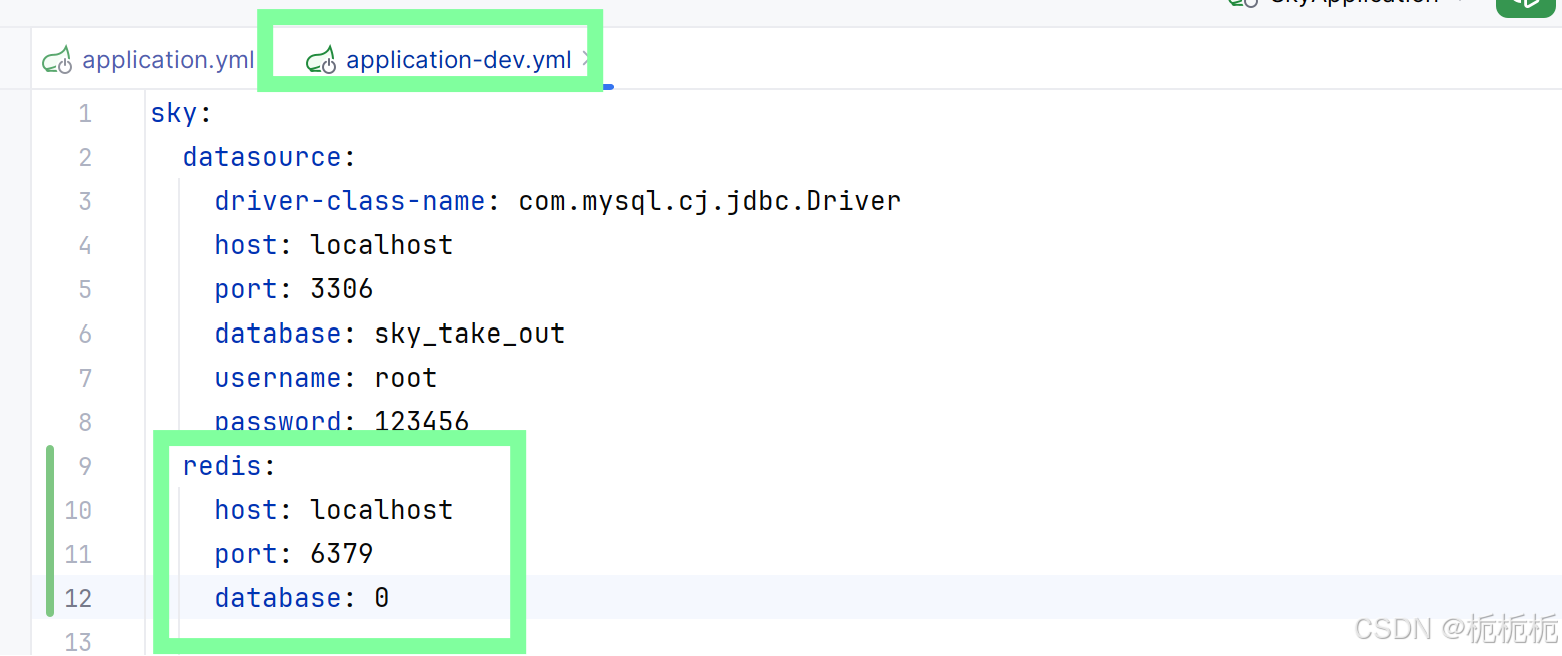
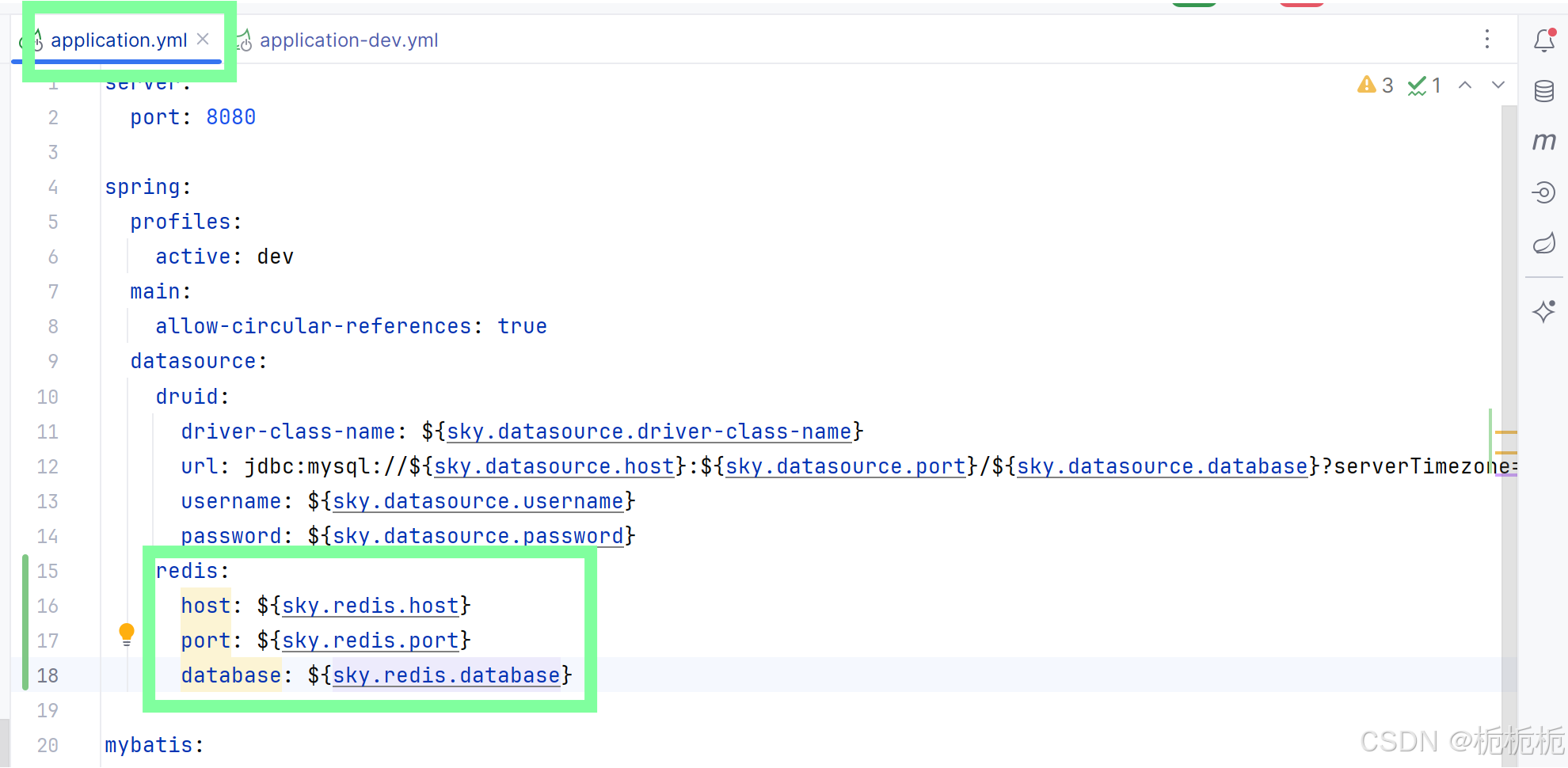
配置类
java
package com.sky.config;
import lombok.extern.slf4j.Slf4j;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
@Slf4j
public class RedisConfiguration {
@Bean
public RedisTemplate redisTemplate(RedisConnectionFactory redisConnectionFactory){
RedisTemplate redisTemplate = new RedisTemplate();
//设置redis的连接工厂对象
redisTemplate.setConnectionFactory(redisConnectionFactory);
//设置redis key的序列化器
redisTemplate.setKeySerializer(new StringRedisSerializer());
return redisTemplate;
}
}
写一个测试类看看是否好使
java
package com.sky.test;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.core.RedisTemplate;
@SpringBootTest
public class SpringDataRedisTest {
@Autowired
private RedisTemplate redisTemplate;
@Test
public void testRedisTemplate(){
System.out.println(redisTemplate);
}
}
在测试类里的运行结果
在java中操作字符串类型
java
package com.sky.test;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.core.*;
import java.util.concurrent.TimeUnit;
@SpringBootTest
public class SpringDataRedisTest {
@Autowired
private RedisTemplate redisTemplate;
@Test
public void testRedisTemplate(){
System.out.println(redisTemplate);
ValueOperations valueOperations=redisTemplate.opsForValue();
HashOperations hashOperations = redisTemplate.opsForHash();
ListOperations listOperations = redisTemplate.opsForList();
SetOperations setOperations = redisTemplate.opsForSet();
ZSetOperations zSetOperations = redisTemplate.opsForZSet();
}
/**
* 操作字符串类型的数据
*/
@Test
public void testString(){
redisTemplate.opsForValue().set("city","北京");
String city = (String) redisTemplate.opsForValue().get("city");
System.out.println(city);
//setex
redisTemplate.opsForValue().set("code","1234",3, TimeUnit.MINUTES);//3分钟
//setnx
redisTemplate.opsForValue().setIfAbsent("lock",1);//成功
redisTemplate.opsForValue().setIfAbsent("lock",2);//不成功
}
}
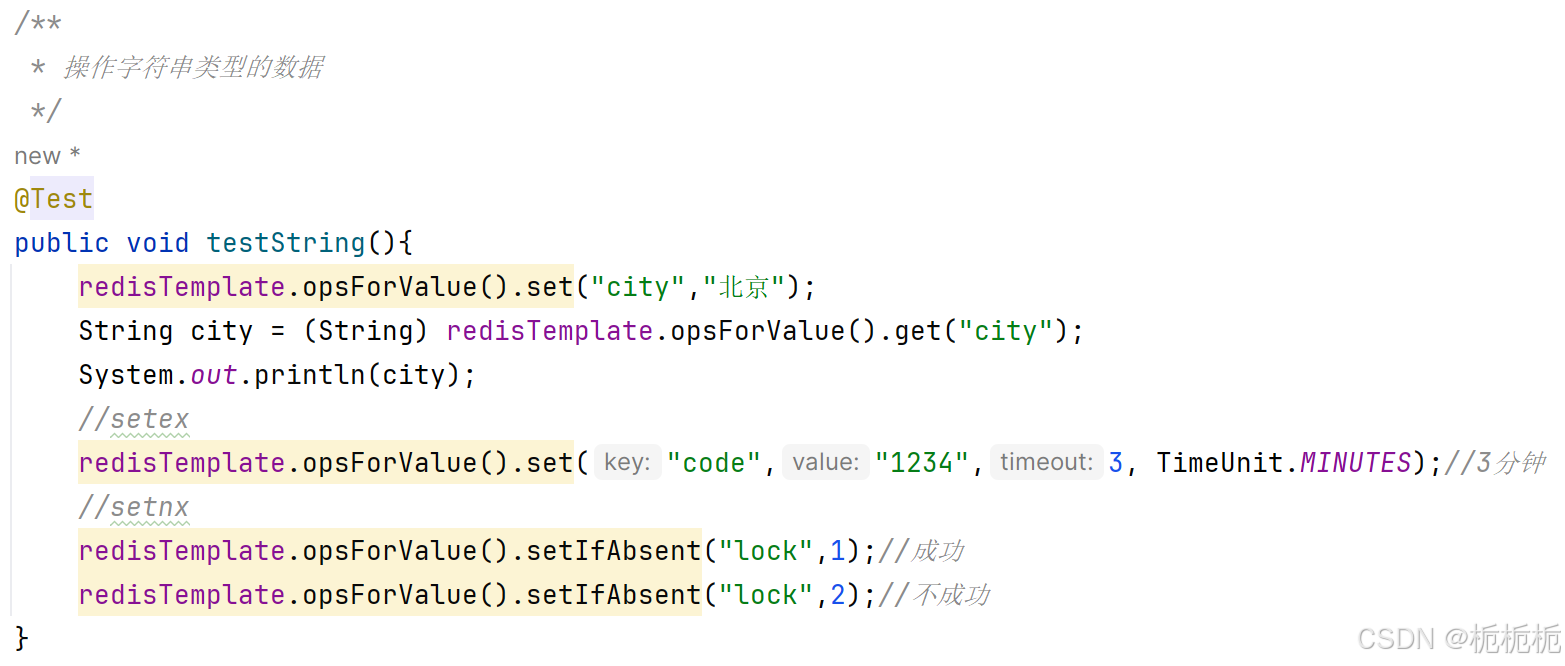

在java中操作哈希类型
java
/**
* 哈希
*/
@Test
public void testHash(){
HashOperations hashOperations = redisTemplate.opsForHash();
//hset
hashOperations.put("100","name","tom");
hashOperations.put("100","age","20");
//hget
String name = (String) hashOperations.get("100", "name");
System.out.println(name);
//hkeys
Set keys = hashOperations.keys("100");
System.out.println(keys);
//hvals
List values = hashOperations.values("100");
System.out.println(values);
//hdel
hashOperations.delete("100","age");
}

在java中操作列表类型数据
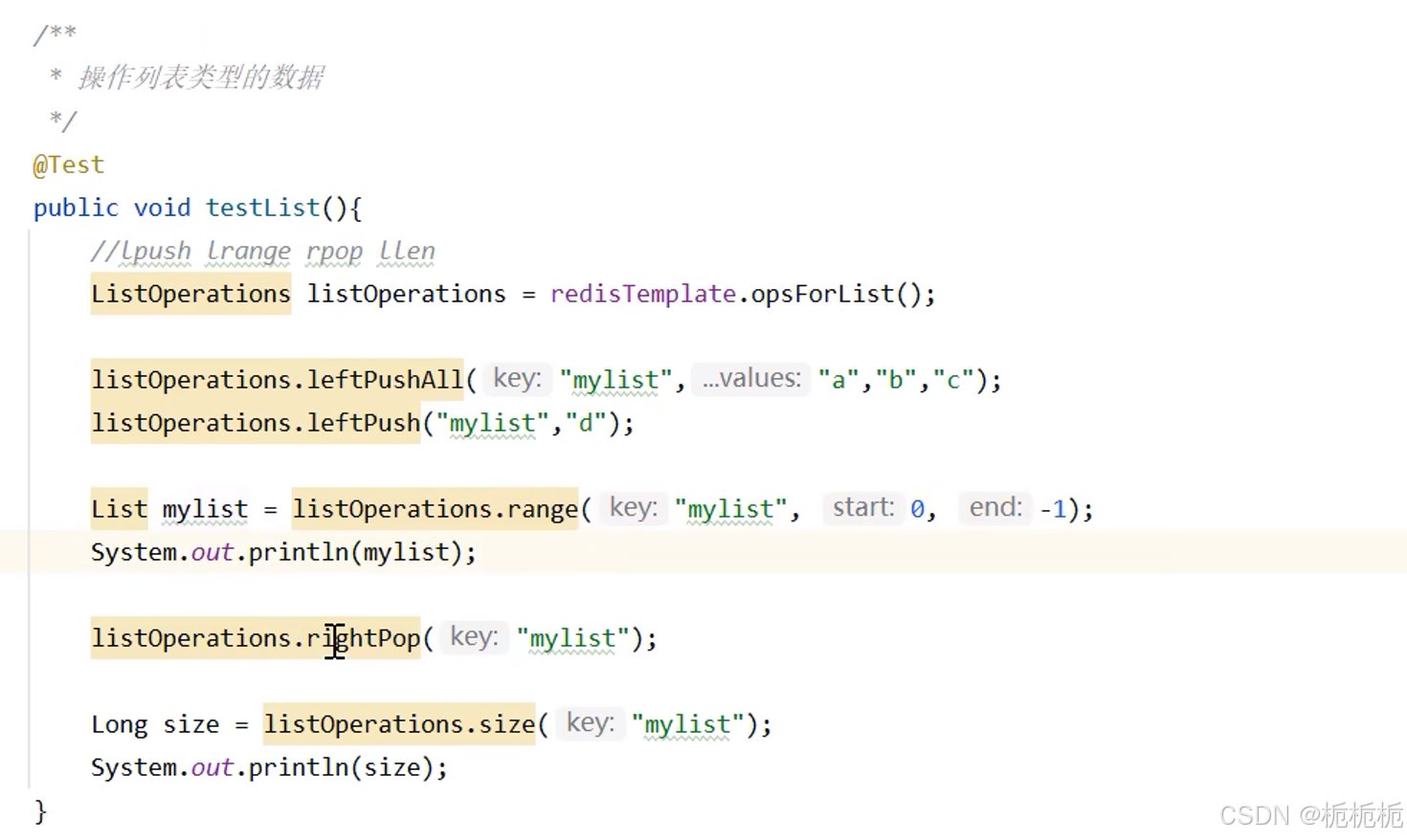
在java中操作集合类型数据
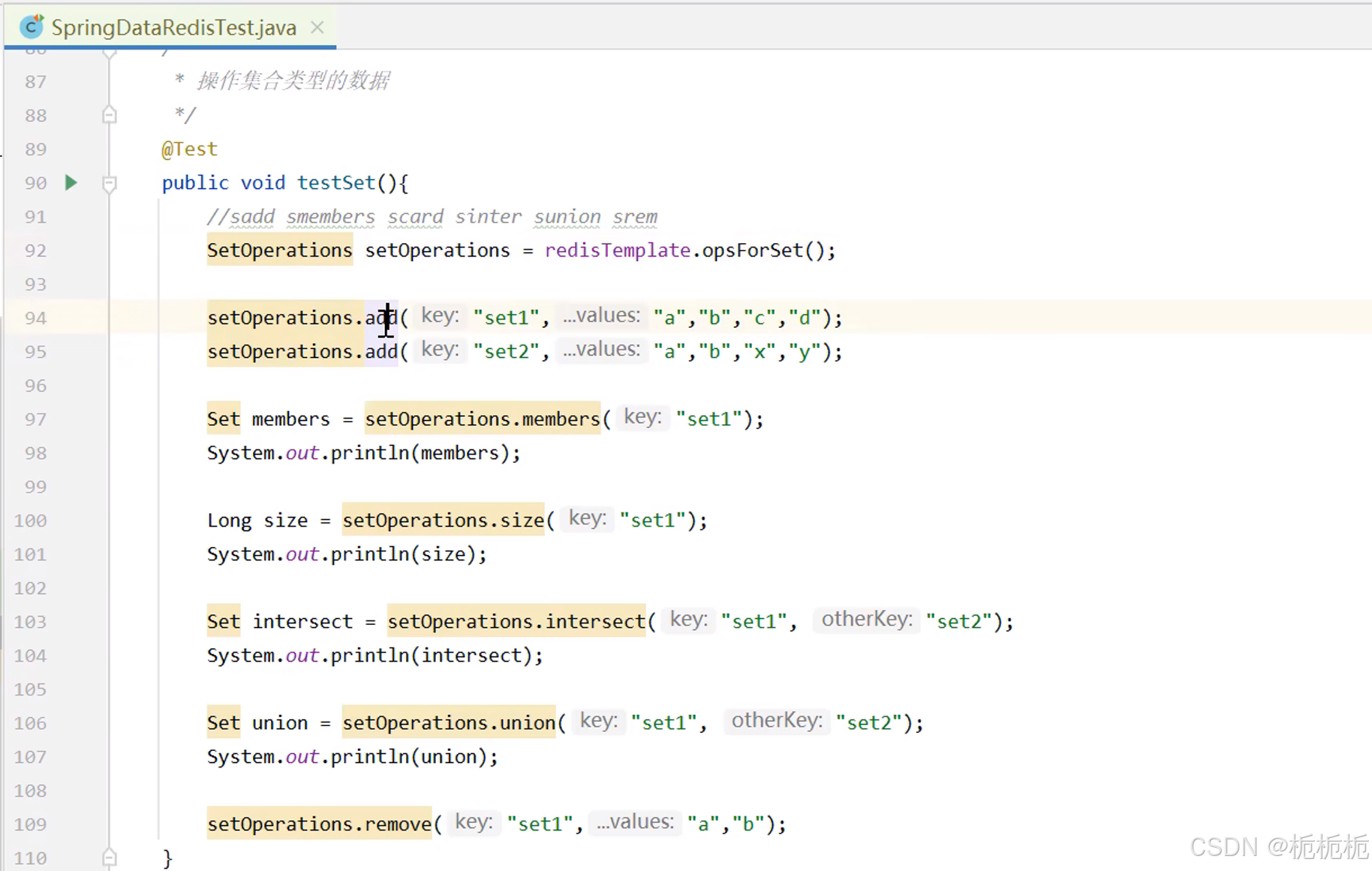
在java中操作有序集合类型数据
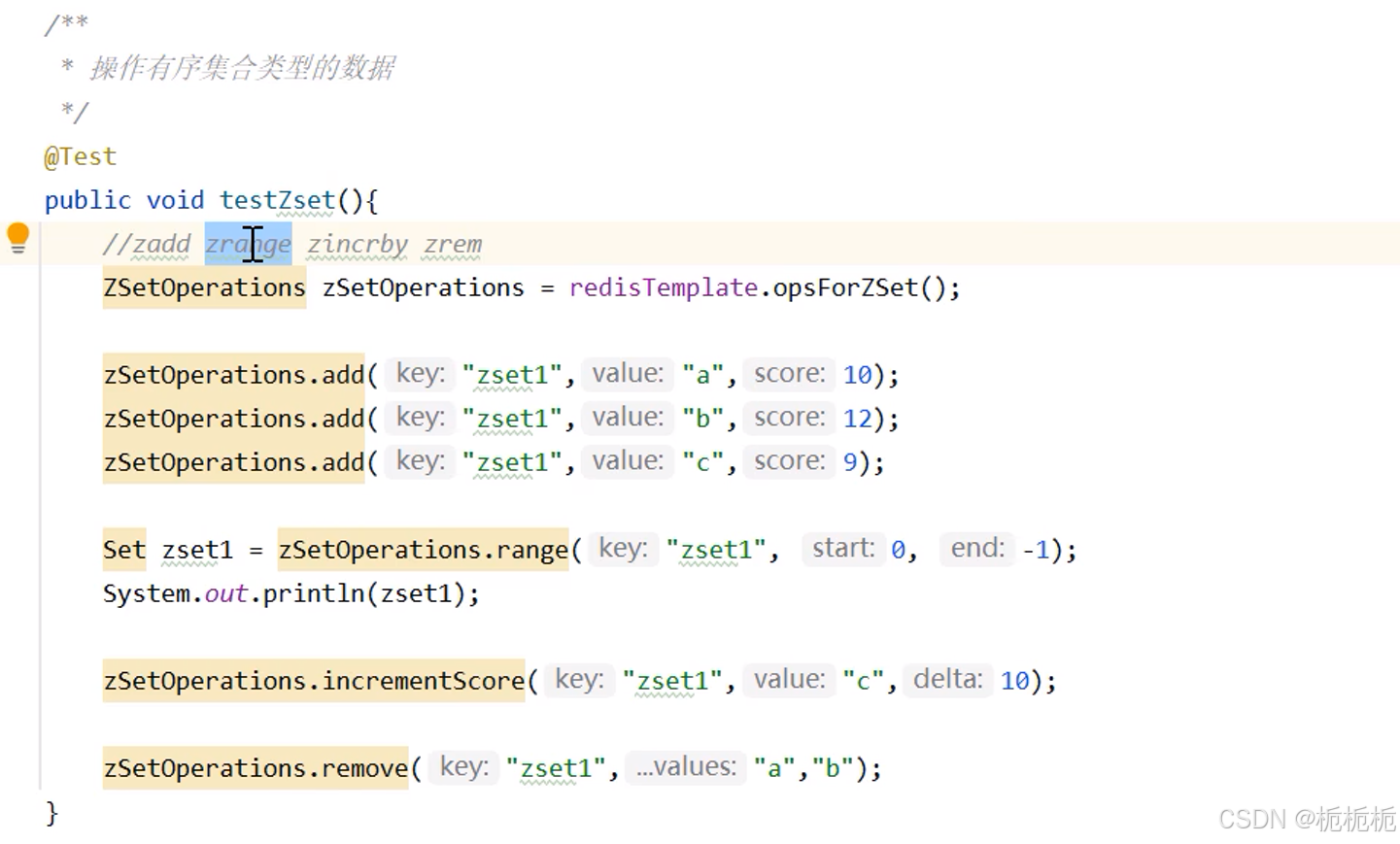