思维导图
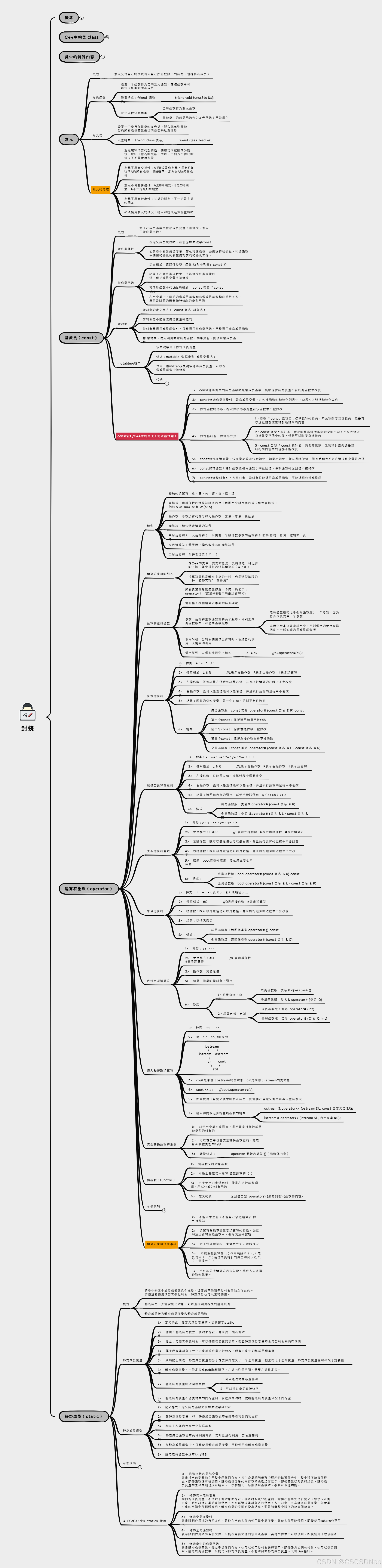
将My_string类中的所有能重载的运算符全部进行重载
+、[] 、>、<、==、>=、<=、!= 、+=(可以加等一个字符串,也可以加等一个字符)、输入输出(<< 、 >>)
My_string
my_string.h
cpp
#ifndef MY_STRING_H
#define MY_STRING_H
#include <iostream>
#include <cstring>
using namespace std;
class My_string
{
private:
char *ptr; //指向字符数组的指针
int size; //字符串的最大容量
int len; //字符串当前容量
public:
//无参构造
My_string():size(20)
{
cout<<"****************无参构造***********"<<endl;
this->ptr = new char[size];
this->ptr[0] = '\0'; //表示串为空串
this->len = 0;
}
//有参构造
My_string(const char* src){
cout<<"****************一个参数有参构造***********"<<endl;
this->len = strlen(src);
this->size = len + 1;
this->ptr = new char[size];
strcpy(this->ptr,src);
}
My_string(int num,char value){
cout<<"****************两个参数有参构造***********"<<endl;
this->len = num;
this->size = len + 1;
this->ptr = new char[len+1];
int i=0;
while(i<num){
this->ptr[i]=value;
i++;
}
this->ptr[num]='\0';
}
//拷贝构造
My_string (const My_string &other){
cout<<"****************拷贝构造***********"<<endl;
len = other.len;
size = other.size;
ptr = new char[size];
strcpy(ptr, other.ptr);
}
//拷贝赋值
My_string &operator=(const My_string &other){
cout<<"****************拷贝赋值***********"<<endl;
if (this == &other) {
return *this; // 直接返回当前对象
}
delete[] ptr;
this->len = other.len;
this->size = other.size;
this->ptr = new char[size];
strcpy(ptr, other.ptr);
return *this;
}
//析构函数
~My_string(){
cout<<"****************析构函数***********"<<endl;
delete []this->ptr;
}
//显示内容
void show();
//判空
void isempty();
//判满
void isfull();
//尾插
void push_back(char value);
//尾删
void pop_back();
//at函数实现
char &at(int index);
//清空函数
void clear();
//返回C风格字符串
char* data();
//返回实际长度
int get_length();
//返回当前最大容量
int get_size();
//君子函数:二倍扩容
void resize();
//自定义运算符重载 +
const My_string operator+(const My_string &R){
My_string temp;
while(this->len+R.len>this->size){
resize();
}
strcpy(temp.ptr,this->ptr);
temp.ptr = strcat(temp.ptr,R.ptr);
this->len = R.len+this->len;
return temp;
}
//自定义运算符重载 []
char operator[](int n){
return ptr[n];
}
// 重载大于运算符 (>)
// 用于比较两个 My_string 对象,逐字符比较
bool operator>(const My_string &R) const {
int i = 0;
// 逐字符比较当前对象和传入的对象,直到遇到字符串结束符 '\0'
while (this->ptr[i] != '\0' && R.ptr[i] != '\0') {
if (this->ptr[i] > R.ptr[i]) {// 如果当前对象的字符大于传入对象的字符,则返回 true
return true;
} else if (this->ptr[i] < R.ptr[i]) { // 如果当前对象的字符小于传入对象的字符,则返回 false
return false;
}
i++;
}
if (this->ptr[i] != '\0' && R.ptr[i] == '\0') {// 如果当前对象的字符串未结束,而传入对象的字符串已结束,返回 true
return true;
}
return false;
}
// 重载小于运算符 (<)
bool operator<(const My_string &R) const {
return strcmp(this->ptr, R.ptr) < 0;
}
// 重载小于等于运算符 (<=)
bool operator<=(const My_string &R) const {
return strcmp(this->ptr, R.ptr) <= 0;
}
// 重载大于等于运算符 (>=)
bool operator>=(const My_string &R) const {
return strcmp(this->ptr, R.ptr) >= 0;
}
// 重载相等运算符 (==)
bool operator==(const My_string &R) const {
return strcmp(this->ptr, R.ptr) == 0;
}
// 重载不等运算符 (!=)
bool operator!=(const My_string &R) const {
return strcmp(this->ptr, R.ptr) != 0;
}
// 重载 += 运算符,用于将传入的字符串附加到当前字符串后
My_string& operator+=(const My_string &R) {
// 检查当前字符串的容量是否足够容纳新字符串,如果不够则调用 resize 扩展容量
while (this->len + R.len >= this->size) {
this->resize();
}
// 使用 strcat 将传入对象的字符串附加到当前对象的字符串后
strcat(this->ptr, R.ptr);
// 更新当前字符串的实际长度
this->len = this->len + R.len;
// 返回当前对象
return *this;
}
// 重载输出运算符 <<
friend ostream& operator<<(ostream &L, const My_string &R);
};
#endif // MY_STRING_H
my_string.cpp
cpp
#include "my_string.h"
#include <cstring>
void My_string::show(){
cout<<ptr<<endl;
}
//判空
void My_string::isempty(){
if(this->len==0){
cout<<"字符串为空"<<endl;
}
return;
}
//判满
void My_string::isfull(){
if(this->len>=this->size){
cout<<"字符串满"<<endl;
resize();
cout<<"重新分配空间"<<endl;
}else{
cout<<"字符串未满"<<endl;
}
return;
}
//尾插
void My_string::push_back(char value){
this->isfull();
this->ptr[len]=value;
len++;
ptr[len]='\0';
}
//尾删
void My_string::pop_back(){
this->len=this->len-1;
ptr[len]='\0';
}
//at函数实现
char & My_string::at(int index){
return ptr[index];
}
//清空函数
void My_string::clear(){
ptr[0]='\0';
this->len=0;
}
//返回C风格字符串
char* My_string::data(){
return this->ptr; // 返回指向字符串的指针
}
//返回实际长度
int My_string::get_length(){
return this->len;
}
//返回当前最大容量
int My_string::get_size(){
return this->size;
}
//君子函数:二倍扩容
void My_string::resize() {
size *= 2;
char* new_ptr = new char[size];
strcpy(new_ptr, ptr);
delete[] ptr;
ptr = new_ptr;
}
ostream & operator<<(ostream &L,const My_string &R){
L<<R.ptr;
return L;
}
main.cpp
cpp
#include "my_string.h"
int main(){
My_string s1("ABCDEF");
My_string s2("GHIJK");
//重构 + 运算符
My_string s3 = s1+s2;
s3.show();
//重构 [] 运算符
cout<<s3[2]<<endl;
cout<<"s1:"<<s1<<" s2:"<<s2<<endl;
//重构 > 运算符
cout<<"s1"<<">"<<"s2?"<<endl;
if(s1>s2){
cout<<"Yes"<<endl;
}else{
cout<<"No"<<endl;
}
//重构 < 运算符
cout<<"s1"<<"<"<<"s2?"<<endl;
if(s1<s2){
cout<<"Yes"<<endl;
}else{
cout<<"No"<<endl;
}
//重构 <= 运算符
cout<<"s1"<<"<="<<"s2?"<<endl;
if(s1<=s2){
cout<<"Yes"<<endl;
}else{
cout<<"No"<<endl;
}
//重构 >= 运算符
cout<<"s1"<<">="<<"s2?"<<endl;
if(s1>=s2){
cout<<"Yes"<<endl;
}else{
cout<<"No"<<endl;
}
//重构 == 运算符
cout<<"s1"<<"=="<<"s2?"<<endl;
if(s1==s2){
cout<<"Yes"<<endl;
}else{
cout<<"No"<<endl;
}
//重构 != 运算符
cout<<"s1"<<"!="<<"s2?"<<endl;
if(s1!=s2){
cout<<"Yes"<<endl;
}else{
cout<<"No"<<endl;
}
//重构 += 运算符
cout<<"************************"<<endl;
s1.show();
s2.show();
s1+=s2;
s1.show();
//重构<<运算符
cout<<s1<<endl;
}
仿照stack类实现my_stack,实现一个栈的操作
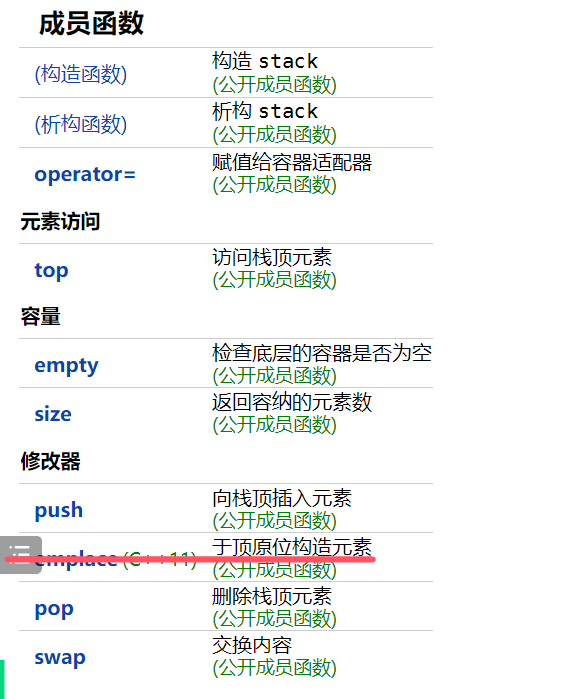
Mystack
My_stack.cpp
cpp
#include"My_stack.h"
using namespace std;
// 入栈操作
void My_Stack::push(int value) {
if (top>=MAX_SIZE - 1) {
cout<<"栈满!"<< value<<endl;
return;
}
arr[++top] = value; // 增加栈顶并赋值
cout<<"入栈: "<<value<<endl;
}
// 出栈操作
int My_Stack::pop() {
if (isEmpty()) {
cout << "栈空!" << endl;
return -1; // 返回 -1 作为错误指示
}
int pop = arr[top--]; // 返回栈顶值并减少栈顶索引
cout << "出栈: " << pop << endl;
return pop;
}
// 获取栈顶元素
int My_Stack::peek() {
if (isEmpty()) {
cout << "栈空!" << endl;
return -1;
}
return arr[top]; // 返回栈顶值
}
// 判断栈是否为空
bool My_Stack::isEmpty() {
return top == -1; //则栈为空,栈顶索引为 -1
}
// 获取栈的当前大小
int My_Stack::size() {
return top + 1; // 返回栈中元素的个数
}
void My_Stack::swap_t(My_Stack& other) {
// 交换栈顶索引
swap(top, other.top);
// 交换栈中的内容
for (int i = 0; i < MAX_SIZE; ++i) {
swap(arr[i], other.arr[i]);
}
}
// 显示栈中的内容
void My_Stack::show() {
if (isEmpty()) {
cout << "栈空!" << endl;
return;
}
cout << "栈中的元素: ";
for (int i = 0; i <= top; ++i) {
cout << arr[i] << " "; // 打印每个元素
}
cout << endl;
}
My_stack.h
cpp
#ifndef MY_STACK_H
#define MY_STACK_H
#include <iostream>
using namespace std;
class My_Stack {
private:
static const int MAX_SIZE = 100; // 定义栈的最大容量
int arr[MAX_SIZE]; // 数组用于存储栈元素
int top; // 栈顶索引
public:
My_Stack():top(-1){} // 构造函数
My_Stack(const My_Stack& other):top(other.top) {//拷贝构造函数
for (int i = 0; i <= top; ++i) {
arr[i] = other.arr[i]; // 深拷贝
}
}
~My_Stack(){}
void push(int value); // 入栈操作
int pop(); // 出栈操作
int peek(); // 获取栈顶元素
bool isEmpty(); // 判断栈是否为空
int size(); // 获取栈的当前大小
void swap_t(My_Stack& other);
void show(); // 显示栈中的内容
My_Stack& operator=(const My_Stack& other){ // 赋值操作符重载
if (this != &other) { // 自我赋值检查
top = other.top; // 复制栈顶索引
for (int i = 0; i <= top; ++i) {
arr[i] = other.arr[i]; // 深拷贝
}
}
return *this; // 返回当前对象的引用
}
};
#endif // MY_STACK_H
main.cpp
cpp
#include "My_stack.h"
int main() {
// 创建第一个栈并进行入栈操作
My_Stack s1;
s1.push(10);
s1.push(20);
s1.push(30);
// 创建第二个栈并进行入栈操作
My_Stack s2;
s2.push(40);
s2.push(50);
My_Stack s3 = s2;
cout<<"s3:";
s3.show();
cout <<"交换前:"<< endl;
cout<<"s1:";
s1.show();
cout<<"s2:";
s2.show();
// 交换s1和s2的内容
s1.swap_t(s2);
cout << "交换后:" << endl;
cout<<"s1:";
s1.show();
cout<<"s2:";
s2.show();
// 出栈
cout<<"从 s1 弹出元素: "<<s1.pop()<<endl;
cout<<"从 s2 弹出元素: "<<s2.pop()<<endl;
// 检查栈的大小
cout<<"s1 当前大小: "<<s1.size()<<endl;
cout<<"s2 当前大小: "<<s2.size()<<endl;
// 再次弹出元素
s1.pop();
s2.pop();
cout << "交换后再次弹出后的大小:" << endl;
cout << "s1 当前大小: " << s1.size() << endl;
cout << "s2 当前大小: " << s2.size() << endl;
return 0;
}