yarn add monaco-editor @monaco-editor/loader
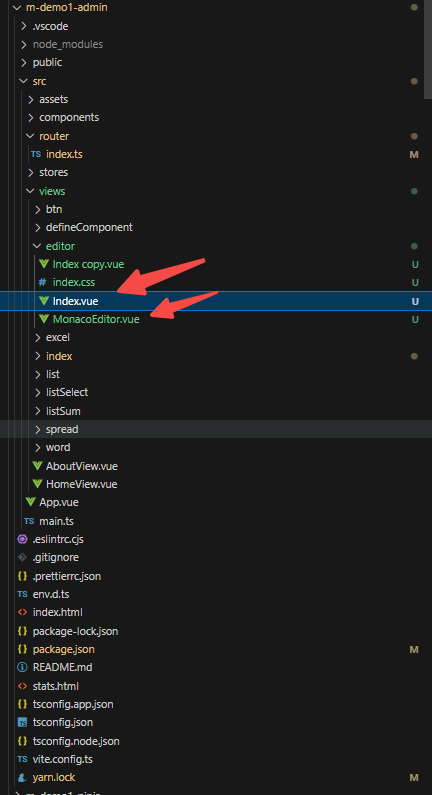
Index.vue:
javascript
<script setup>
import { ref, onMounted } from 'vue'
import MonacoEditor from './MonacoEditor.vue'
const code = ref('// Hello World')
const language = ref('java')
const theme = ref('default-theme') // default-theme vs-dark
const logValue = () => {
console.log(code.value)
}
onMounted(() => {
code.value =
`
Here is the chat histories between human and assistant, inside <histories></histories> XML tags.
\`\`\`java
示例代码
package com.cool.modules.base.entity.sys;
import com.cool.core.base.BaseEntity;
import com.tangzc.autotable.annotation.enums.IndexTypeEnum;
import com.tangzc.mybatisflex.autotable.annotation.ColumnDefine;
import com.mybatisflex.annotation.Table;
import com.tangzc.autotable.annotation.Index;
import lombok.Getter;
import lombok.Setter;
/**
* 系统配置
*/
@Getter
@Setter
@Table(value = "base_sys_conf", comment = "系统配置表")
public class BaseSysConfEntity extends BaseEntity<BaseSysConfEntity> {
@Index(type = IndexTypeEnum.UNIQUE)
@ColumnDefine(comment = "配置键", notNull = true)
private String cKey;
@ColumnDefine(comment = "值", notNull = true, type = "text")
private String cValue;
}
\`\`\`
<histories>
{{#histories#}}
</histories>
Human: {{#sys.query#}}
Assistant:
`
})
</script>
<template>
<div id="editor" style="height: 400px">
<MonacoEditor
v-model:value="code"
:language="language"
:theme="theme"
/>
</div>
<div class="controls">
<label for="language">Select Language: </label>
<select id="language" v-model="language">
<option value="java">Java</option>
<option value="javascript">Javascript</option>
</select>
</div>
<button @click="logValue">Log Value</button>
</template>
MonacoEditor.vue:
javascript
<script setup>
import { ref, onMounted, onBeforeUnmount, watch, defineProps, defineEmits } from 'vue'
import loader from '@monaco-editor/loader'
const props = defineProps({
value: String,
language: {
type: String,
default: 'java'
},
theme: {
type: String,
default: 'vs-dark'
}
})
const emits = defineEmits(['update:value'])
const editorContainer = ref(null)
let editorInstance = null
onMounted(() => {
loader.init().then((monaco) => {
editorInstance = monaco.editor.create(editorContainer.value, {
value: props.value || '',
language: props.language,
theme: props.theme,
readOnly: true,
domReadOnly: true,
quickSuggestions: false,
minimap: { enabled: false },
lineNumbersMinChars: 1,
lineNumbers: 'off',
wordWrap: 'on',
unicodeHighlight: {
ambiguousCharacters: false
}
})
editorInstance.onDidChangeModelContent(() => {
emits('update:value', editorInstance.getValue())
})
})
})
onBeforeUnmount(() => {
if (editorInstance) {
editorInstance.dispose()
}
})
watch(
() => props.language,
(newLanguage) => {
if (editorInstance) {
loader.init().then((monaco) => {
monaco.editor.setModelLanguage(editorInstance.getModel(), newLanguage)
})
}
}
)
watch(
() => props.value,
(newValue) => {
if (editorInstance && editorInstance.getValue() !== newValue) {
editorInstance.setValue(newValue)
}
}
)
</script>
<template>
<div ref="editorContainer" class="editor-container"></div>
</template>
<style>
.editor-container {
width: 100%;
height: 100%;
}
</style>
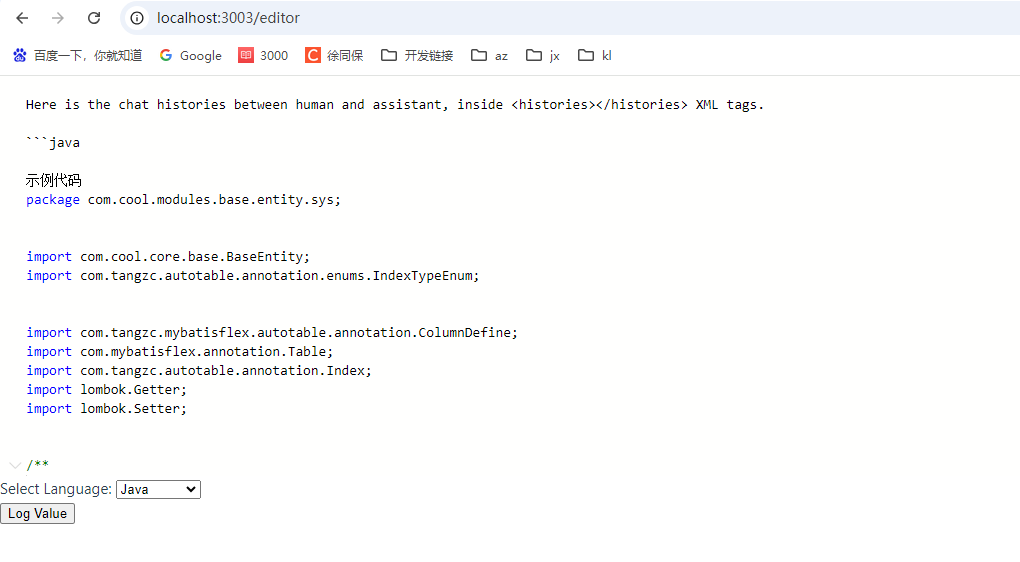
参考链接
https://blog.csdn.net/weixin_67711401/article/details/140729746
https://microsoft.github.io/monaco-editor/
人工智能学习网站