2.1基于django
python
#settings.py
urlpatterns = [
path('admin/', admin.site.urls),
path('auth',views.auth) #创建一个路由
]
python
#views.py
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
# Create your views here.
@csrf_exempt
def auth(request):
return JsonResponse({'status':True,'message':'success'})
使用postman测试,可以看到有返回数据
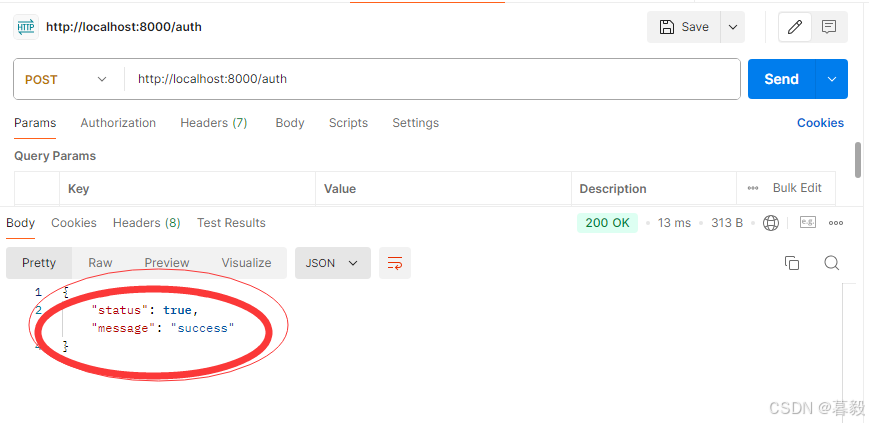
2.2基于drf
1.安装drf
shell
pip3 install djangorestframework
2.注册drf
python
#settings.py
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'app1.apps.App1Config', # 添加应用名称(appName.apps.className)
'rest_framework', #注册drf
]
3、注册路由
python
#url.py
urlpatterns = [
path('admin/', admin.site.urls),
path('auth/',views.auth),
path('login1/',views.login), #drf视图路由(方法1:FBV)
path('login2/',views.LoginView.as_view()) #drf视图路由(方法2:CBV)
]
4、创建drf视图函数
python
#方法1:FBV: function basic views(基于函数的视图)
from rest_framework.response import Response
from rest_framework.views import APIView
from rest_framework.decorators import api_view
@api_view(['GET']) #视图函数前面需要加上一个装饰器
def login(request):
return Response({'status':True,'message':'success'})
python
#方法2:CBV: class basic views(基于类的视图)
class LoginView(APIView):
def get(self,request):
return Response({'status': True, 'message': 'success'})
5、查看效果
用浏览器访问login,这里可以看到,Response返回的信息,被drf的基本页面包裹(以上两种方法效果相同)
6、CBV示例1(jdango)
python
from django.http import JsonResponse
from django.views import View
class UserView(View):
#不同的请求,执行不同的值
def get(self,request):
#get请求执行的函数
return JsonResponse({'status': True, 'message': 'GET'})
def post(self,request):
#post请求执行的函数
return JsonResponse({'status': True, 'message': 'POST'})
def put(self,request):
#put请求执行的函数
return JsonResponse({'status': True, 'message': 'PUT'})
def delete(self,request):
#delete请求执行的函数
return JsonResponse({'status': True, 'message': 'DELETE'})
7、CBV示例2(drf)
python
from rest_framework.response import Response
from rest_framework.views import APIView
class UserView1(APIView): #drf中的函数自动套用了免除CRSF认证,相当于会自动给函数加一个@csrf_exempt装饰器
def get(self,request):
return Response({'status': True, 'message': 'GET'})
def post(self,request):
return Response({'status': True, 'message': 'POST'})
def put(self,request):
return Response({'status': True, 'message': 'PUT'})
def delete(self,request):
return Response({'status': True, 'message': 'DELETE'})
python
#APIView底层代码
def as_view(cls, **initkwargs):
"""
用于免除CRSF认证
Store the original class on the view function.
This allows us to discover information about the view when we do URL
reverse lookups. Used for breadcrumb generation.
"""
def dispatch(self, request, *args, **kwargs):
#视图执行前、反射执行视图,视图后处理
#判断用户请求类型,以执行相对应的函数
# Try to dispatch to the right method; if a method doesn't exist,
# defer to the error handler. Also defer to the error handler if the
# request method isn't on the approved list.