string的基本操作
一.与C语言中字符串的区别
C语言中的字符串是以'\0'为结束标志的字符的数组,但字符串函数与字符串是分离的,这与OOP(面向对象编程)的思想不符,所以C++提供了支持自动扩容且用类域封装的------string类
二.标准库中的string
官方文档中对string类的介绍如下:
String class
Strings are objects that represent sequences of characters.
( 字符串是表示字符序列的类)The standard string class provides support for such objects with an interface similar to that of a standard container of bytes, but adding features specifically designed to operate with strings of single-byte characters.
( 标准的字符串类提供了对此类对象的支持,其接口类似于标准字符容器的接口,但添加了专门用于操作单字节字符字符串的设计特性。)The string class is an instantiation of the basic_string class template that uses char (i.e., bytes) as its character type, with its default char_traits and allocator types (see basic_string for more info on the template).
(string类是basic_string模板类的一个实例,它使用char来实例化basic_string模板类,并用char_traits和allocator作为basic_string的默认参数(根于更多的模板信息请参考basic_string)。)Note that this class handles bytes independently of the encoding used: If used to handle sequences of multi-byte or variable-length characters (such as UTF-8), all members of this class (such as length or size), as well as its iterators, will still operate in terms of bytes (not actual encoded characters).
(注意,这个类独立于所使用的编码来处理字节:如果用来处理多字节或变长字符(如UTF-8)的序列,这个类的所有成员(如长度或大小)以及它的迭代器,将仍然按照字节(而不是实际编码的字符)来操作。)
三.string中常用接口的介绍
1.string中常用的构造函数
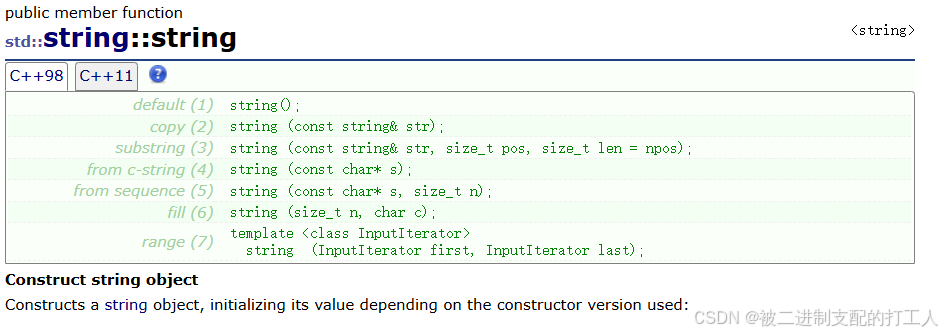
constructor函数 | 功能说明 |
---|---|
string() | 默认构造,构造空字符串的string类 |
string (const string& str) (重点) | 用一个string对象来构造新的string对象(拷贝构造) |
string (const string& str, size_t pos, size_t len = npos) | 用一个string对象pos位置开始的len个字符构造,如果len太大或者len==npos就直到末尾 |
string (const char* s)(重点) | 用C-string来构造string类对象 |
string (const char* s, size_t n) | 用C-string的前n个字符构造 |
string (size_t n, char c) | 用n个c字符构造初始化 |
template <class InputIterator>string (InputIterator first, InputIterator last)(重点) | 用一段迭代器区间构造 |
测试用例:
cpp
int main()
{
string s1;
string s2("hello world");
string s3(s2);
string s4("hello world", 5);
string s5(5, 'x');
cout << "s1:" << s1 << endl;
cout << "s2:" << s2 << endl;
cout << "s3:" << s3 << endl;
cout << "s4:" << s4 << endl;
cout << "s5:" << s5 << endl;
return 0;
}
运行结果:
s1:
s2:hello world
s3:hello world
s4:hello
s5:xxxxx
2.string类对象的容量操作函数
函数名称 | 功能说明 |
---|---|
size | 返回字符串有效字符(不包括'\0')的长度(重点) |
length | 返回字符串有效字符(不包括'\0')的长度(同size) |
capacity | 返回空间总大小 |
empty | 判断是否为空串,如果是返回true,不是返回false |
clear(重点) | 清除有效字符 |
reserve(重点) | 预留空间(扩容) |
resize(重点) | == 将有效字符个数变为n个,多出的空间用字符c填充== |
注意:
1.size()与length()方法底层实现原理完全相同,引入size()的原因是为了与其他容器的接口保持一 致,一般情况下基本都是用size()。
clear()只是将string中有效字符清空,不改变底层空间大小。
resize(size_t n) 与 resize(size_t n, char c)都是将字符串中有效字符个数改变到n个,不同的是当字 符个数增多时:resize(n)用0来填充多出的元素空间,resize(size_t n, char c)用字符c来填充多出的 元素空间。注意:resize在改变元素个数时,如果是将元素个数增多,可能会改变底层容量的大小,如果是将元素个数减少,底层空间总大小不变。
reserve(size_t res_arg=0):为string预留空间,不改变有效元素个数,当reserve的参数小于 string的底层空间总大小时,reserver不会改变容量大小。
测试用例:
cpp
int main()
{
string s1("hello world");
cout << s1.size() << endl;
cout << s1.capacity() << endl;
s1.resize(20,'x');
cout << s1.size() << endl;
cout << s1 << endl;
s1.reserve(100);
cout << s1.capacity() << endl;
cout << s1.empty() << endl;
s1.clear();
cout << s1.empty() << endl;
return 0;
}
运行结果:
11
15
20
hello worldxxxxxxxxx
111
0
1
3.string类对象的访问及遍历操作
函数名称 | 功能说明 |
---|---|
operator[] | 返回pos位置的字符 |
begin+end | begin返回第一个位置的迭代器,end返回最后一个字符的下一个位置的迭代器 |
rbegin+rend | 返回反向迭代器,这样可以支持从后往前遍历 |
范围for | C++11支持更加简洁的范围for遍历 |
测试用例:
cpp
int main()
{
string s1("hello world");
//1.operator[]遍历
for (int i = 0; i < s1.size(); ++i)
{
cout << s1[i] << " ";
}
cout << endl;
//2.迭代器遍历
string::iterator it = s1.begin();
while (it != s1.end())
{
cout << *it << " ";
++it;
}
cout << endl;
//3.反向迭代器
string::reverse_iterator rit = s1.rbegin();
while (rit != s1.rend())
{
cout << *rit << " ";
++rit;
}
cout << endl;
//4.范围for
for (auto& e : s1)
{
cout << e << " ";
}
cout << endl;
return 0;
}
运行结果:
h e l l o w o r l d
h e l l o w o r l d
d l r o w o l l e h
h e l l o w o r l d
4.string类对象的修改操作
函数名称 | 功能说明 |
---|---|
push_back | 在字符串后尾插一个字符 |
append | 在字符串后追加一个字符串 |
operator+=(重点) | 在字符串末尾追加一个字符串 |
c_str | 返回C语言格式的字符串 |
find | 从字符串pos位置开始往后找字符c,返回该字符在字符串中的位置,找不到返回npos |
rfind | rfind 从字符串pos位置开始往前找字符c,返回该字符在字符串中的位置,找不到返回npos |
substr | 在str中从pos位置开始,截取n个字符,然后将其返回 |
注意:
1. 在string尾部追加字符时,s.push_back© / s.append(1, c) / s += 'c'三种的实现方式差不多,一般 情况下string类的+=操作用的比较多,+=操作不仅可以连接单个字符,还可以连接字符串。
2. 对string操作时,如果能够大概预估到放多少字符,可以先通过reserve把空间预留好。
代码演示:
cpp
int main()
{
string s1("hello world");
s1.push_back('x');
s1.append("yyyyyy");
s1 += 'z';
s1 += "+++++++++++";
cout << s1<<endl;
return 0;
}
运行结果:
hello worldxyyyyyyz+++++++++++
5.string类的非成员函数
函数名称 | 功能说明 |
---|---|
operator+ | 尽量少用,传值返回效率低 |
operator>> | 流插入运算符重载 |
operator<<流提取运算符重载 | |
getline(重点) | 获取一行字符串 |
relational operators(重点) | 各种大小比较 |
这些操作比较好理解,这里就不演示代码了,如果需要就去文档中看看测试用例:
链接: link
6.string中的其他一些操作
函数名称 | 功能说明 |
---|---|
shrink_to_fit | 将容量和大小适配 |
assign | 为字符串分配一个新值,替换其当前内容。 |
replace | 将字符串中从字符 pos 开始并跨越 len 字符的部分(或 [i1,i2) 之间范围内的字符串部分)替换为新内容 |
find_first_of | 在字符串中搜索与其参数中指定的任何字符匹配的第一个字符。 |
find_last_of | 在字符串中搜索与参数中指定的任何字符匹配的最后一个字符。 |
find_first_not_of | 在字符串中搜索与其参数中指定的任何字符不匹配的第一个字符。 |
find_last_not_of | 在字符串中搜索与参数中指定的任何字符不匹配的最后一个字符。 |
copy | 将字符串对象当前值的子字符串复制到 s 指向的数组中。此子字符串包含从位置 pos 开始的 len 字符。该函数不会在复制内容的末尾附加 '\0'字符。 |
关于string类的使用就这么多,感谢您的观看!!!