Visual Studio C# 编写加密火星坐标转换
- 1、WGS84坐标转GCJ02火星坐标
- 2、GCJ02火星坐标转WGS84坐标(回归计算)
- 3、GCJ02火星坐标转BD09百度坐标
- 4、BD09百度坐标转GCJ02火星坐标(回归计算)
- 5、坐标公共转换类
- 6、地图显示
- 7、程序简单界面
- 8、完整源代码工程
1、WGS84坐标转GCJ02火星坐标
csharp
/// <summary>WGS84坐标转GCJ02火星坐标</summary>
/// <param name="Position">坐标结构体</param>
/// <returns>返回GCJ02火星坐标</returns>
public coord WGS84ToGCJ02(coord Position)
{
Double lon = Position.lon;
Double lat = Position.lat;
if (!isInChina(lon, lat)) return Position;
coord Pos = offset(lon, lat);
Pos.lon = lon + Pos.lon;
Pos.lat = lat + Pos.lat;
return Pos;
}
2、GCJ02火星坐标转WGS84坐标(回归计算)
csharp
/// <summary>GCJ02火星坐标转WGS84坐标</summary>
/// <param name="Position">坐标结构体</param>
/// <returns>返回WGS84火星坐标</returns>
public coord GCJ02ToWGS84(coord Position)
{
Double lon = Position.lon;
Double lat = Position.lat;
if (!isInChina(lon, lat)) return Position;
coord Pos = Position;
coord tempPoint = WGS84ToGCJ02(Pos);
Double dx = tempPoint.lon - lon;
Double dy = tempPoint.lat - lat;
while (Math.Abs(dx) > 1e-14 || Math.Abs(dy) > 1e-14)//回归
{
Pos.lon -= dx;
Pos.lat -= dy;
tempPoint = WGS84ToGCJ02(Pos);
dx = tempPoint.lon - lon;
dy = tempPoint.lat - lat;
}
return Pos;
}
3、GCJ02火星坐标转BD09百度坐标
csharp
/// <summary>GCJ02火星坐标转BD09百度坐标</summary>
/// <param name="Position">坐标结构体</param>
/// <returns>返回BD09百度坐标</returns>
public coord GCJ02ToBD09(coord Position)
{
Double lon = Position.lon;
Double lat = Position.lat;
Double x = lon;
Double y = lat;
Double z = Math.Sqrt(x * x + y * y) + 0.00002 * Math.Sin(y * baiduFactor);
Double theta = Math.Atan2(y, x) + 0.000003 * Math.Cos(x * baiduFactor);
coord outPos;
outPos.lon = z * Math.Cos(theta) + 0.0065;
outPos.lat = z * Math.Sin(theta) + 0.006;
return outPos;
}
4、BD09百度坐标转GCJ02火星坐标(回归计算)
csharp
/// <summary>BD09百度坐标转GCJ02火星坐标</summary>
/// <param name="Position">坐标结构体</param>
/// <returns>返回GCJ02火星坐标</returns>
public coord BD09ToGCJ02(coord Position)
{
Double lon = Position.lon;
Double lat = Position.lat;
Double x = lon - 0.0065;
Double y = lat - 0.006;
coord Pos;
Pos.lon = x;
Pos.lat = y;
coord tempPoint = GCJ02ToBD09(Pos);
Double dx = tempPoint.lon - lon;
Double dy = tempPoint.lat - lat;
while (Math.Abs(dx) > 1e-14 || Math.Abs(dy) > 1e-14)//回归
{
Pos.lon -= dx;
Pos.lat -= dy;
tempPoint = GCJ02ToBD09(Pos);
dx = tempPoint.lon - lon;
dy = tempPoint.lat - lat;
}
return Pos;
}
5、坐标公共转换类
csharp
/// <summary>长半轴</summary>
public const Double a = 6378245.0;
/// <summary>第一偏心率</summary>
public const Double ee = 0.00669342162296594322796213968775;
/// <summary>百度坐标转换因子</summary>
public const Double baiduFactor = (Math.PI * 3000.0) / 180.0;
// 定义坐标结构体
public struct coord
{
public Double lon;
public Double lat;
};
/// <summary>是否中国坐标</summary>
/// <param name="lon">经度</param>
/// <param name="lat">纬度</param>
/// <returns>返回布尔值</returns>
public Boolean isInChina(Double lon, Double lat)
{
return lon >= 72.004 && lon <= 137.8347 && lat >= 0.8293 && lat <= 55.8271;
}
/// <summary>偏移量</summary>
/// <param name="lon">经度</param>
/// <param name="lat">纬度</param>
/// <returns></returns>
public coord offset(Double lon, Double lat)
{
Double dLon = transformLon(lon - 105.0, lat - 35.0);
Double dLat = transformLat(lon - 105.0, lat - 35.0);
Double radLat = (lat / 180.0) * Math.PI;
Double magic = Math.Sin(radLat);
magic = 1 - ee * magic * magic;
Double sqrtMagic = Math.Sqrt(magic);
coord outPos;
outPos.lon = (dLon * 180.0) / (a / sqrtMagic * Math.Cos(radLat) * Math.PI);
outPos.lat = (dLat * 180.0) / ((a * (1 - ee)) / (magic * sqrtMagic) * Math.PI);
return outPos;
}
/// <summary>纬度偏移量</summary>
/// <param name="lon">经度</param>
/// <param name="lat">纬度</param>
/// <returns>返回纬度</returns>
public Double transformLat(Double lon, Double lat)
{
Double ret = -100.0 + 2.0 * lon + 3.0 * lat + 0.2 * lat * lat + 0.1 * lon * lat + 0.2 * Math.Sqrt(Math.Abs(lon));
ret += ((20.0 * Math.Sin(6.0 * lon * Math.PI) + 20.0 * Math.Sin(2.0 * lon * Math.PI)) * 2.0) / 3.0;
ret += ((20.0 * Math.Sin(lat * Math.PI) + 40.0 * Math.Sin((lat / 3.0) * Math.PI)) * 2.0) / 3.0;
ret += ((160.0 * Math.Sin((lat / 12.0) * Math.PI) + 320.0 * Math.Sin((lat * Math.PI) / 30.0)) * 2.0) / 3.0;
return ret;
}
/// <summary>经度偏移量</summary>
/// <param name="lon">经度</param>
/// <param name="lat">纬度</param>
/// <returns>返回经度</returns>
public Double transformLon(Double lon, Double lat)
{
Double ret = 300.0 + lon + 2.0 * lat + 0.1 * lon * lon + 0.1 * lon * lat + 0.1 * Math.Sqrt(Math.Abs(lon));
ret += ((20.0 * Math.Sin(6.0 * lon * Math.PI) + 20.0 * Math.Sin(2.0 * lon * Math.PI)) * 2.0) / 3.0;
ret += ((20.0 * Math.Sin(lon * Math.PI) + 40.0 * Math.Sin((lon / 3.0) * Math.PI)) * 2.0) / 3.0;
ret += ((150.0 * Math.Sin((lon / 12.0) * Math.PI) + 300.0 * Math.Sin((lon / 30.0) * Math.PI)) * 2.0) / 3.0;
return ret;
}
6、地图显示
csharp
/// <summary>坐标值改变,地图改变</summary>
private void ChangeVal()
{
Double longitude = 0;
Double latitude = 0;
switch (comboBox1.SelectedIndex)
{
case 0://高德
if (string.IsNullOrEmpty(textBox3.Text) || string.IsNullOrEmpty(textBox4.Text))
{
longitude = 112.960235035495;
latitude = 28.1661659723607;
}
else
{
longitude = Convert.ToDouble(textBox4.Text);
latitude = Convert.ToDouble(textBox3.Text);
}
//string amapUrl = "http://ditu.amap.com/?longitude=" + longitude + "&latitude=" + latitude;
string amapUrl = "http://ditu.amap.com";// "http://map.gaode.com/";
webBrowser1.Navigate(amapUrl);
break;
case 1://百度
if (string.IsNullOrEmpty(textBox5.Text) || string.IsNullOrEmpty(textBox6.Text))
{
longitude = 112.966759165825;
latitude = 28.1719881916543;
}
else
{
longitude = Convert.ToDouble(textBox6.Text);
latitude = Convert.ToDouble(textBox5.Text);
}
//string url = "http://map.baidu.com/";
string url = "http://api.map.baidu.com/marker?location=" + latitude + "," + longitude + "&title=&content=我在这里&output=html";
webBrowser1.Navigate(url);
break;
case 2://腾讯
if (string.IsNullOrEmpty(textBox3.Text) || string.IsNullOrEmpty(textBox4.Text))
{
longitude = 112.960235035495;
latitude = 28.1661659723607;
}
else
{
longitude = Convert.ToDouble(textBox4.Text);
latitude = Convert.ToDouble(textBox3.Text);
}
// string qqMapUrl= "http://map.qq.com/?referer=ts.qq.com&type=ms&lat=" + latitude + "&lng=" +longitude;
string qqMapUrl = "http://map.qq.com/";
webBrowser1.Navigate(qqMapUrl);
break;
}
}
7、程序简单界面
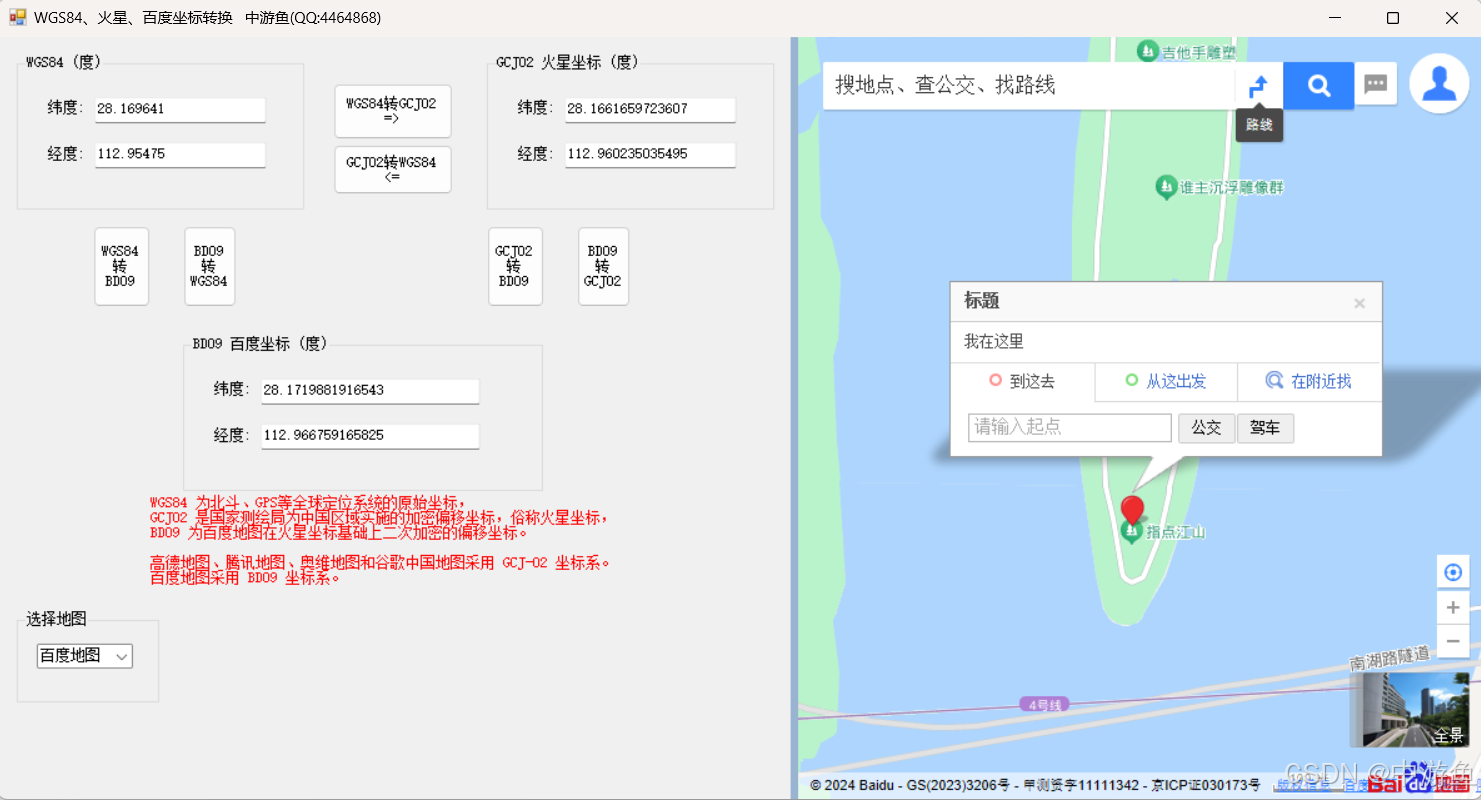
8、完整源代码工程
CSDN 资源下载地址: