在WPF(Windows Presentation Foundation)中,文件选择通常是通过使用 OpenFileDialog
和 SaveFileDialog
类来实现的。这些类位于 Microsoft.Win32
命名空间中。
一、安装相关依赖包
1、点击"工具-NuGet包管理-管理解决方案的NuGet程序包"
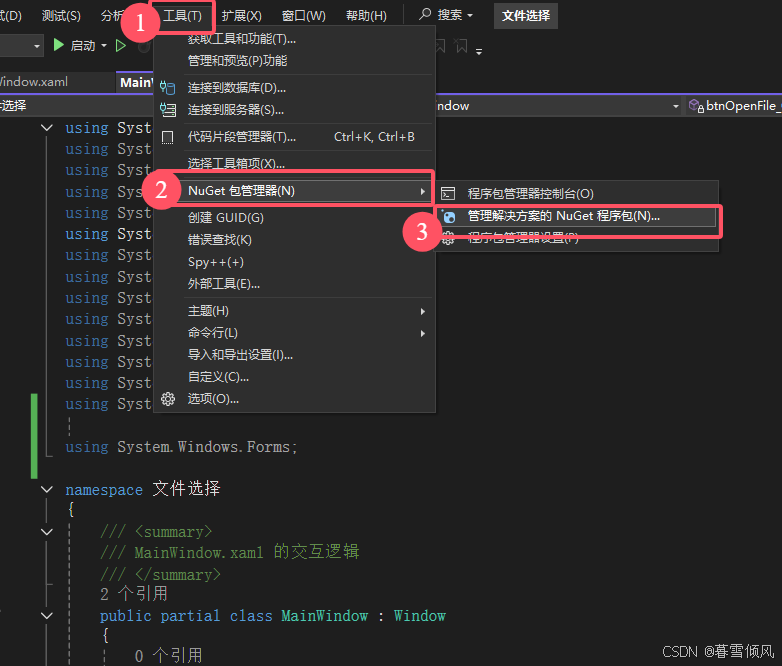
2、搜索"form",并安装到自己的项目中
一定要选择微软官方的那个包
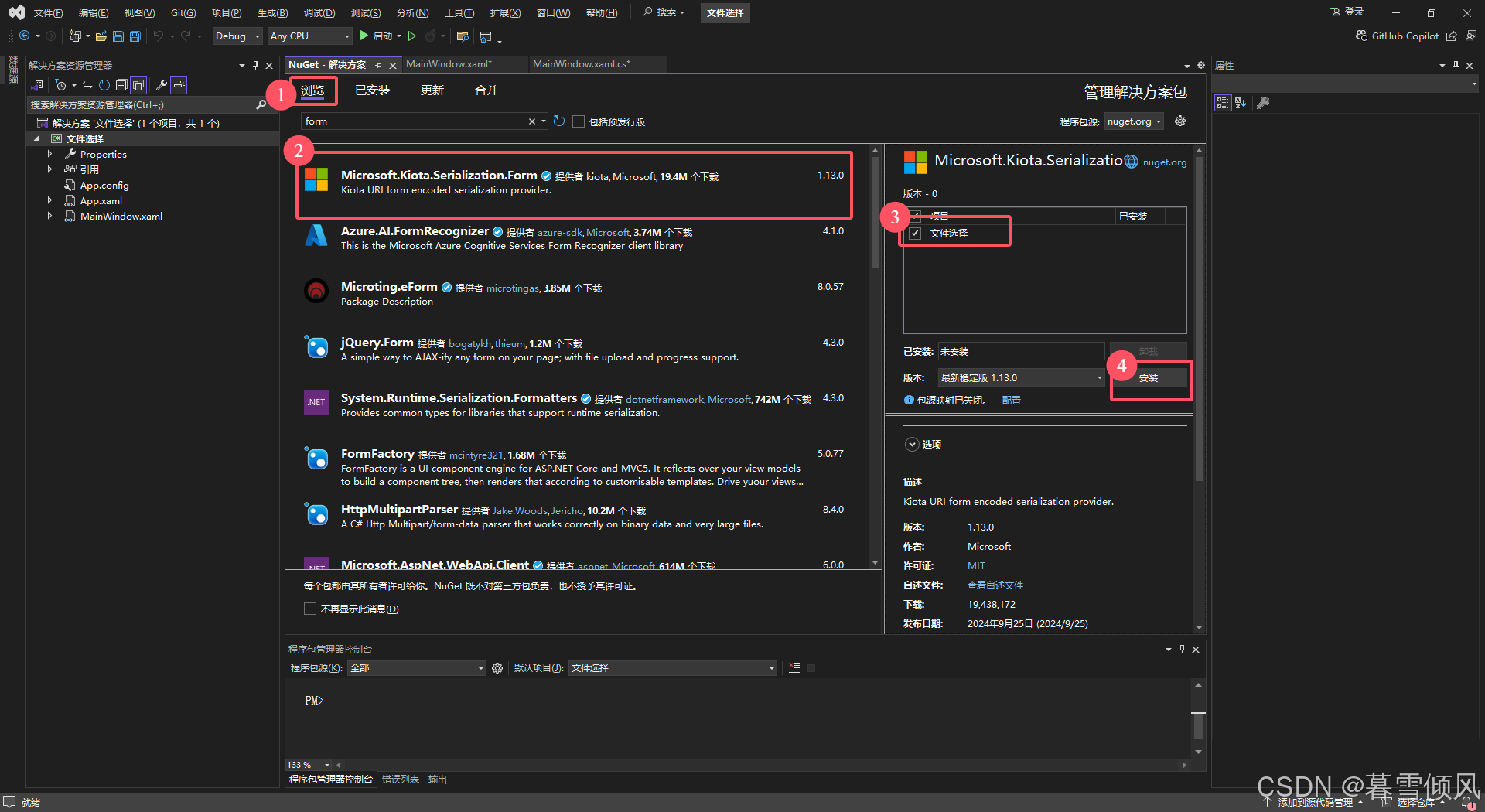
3、等待安装
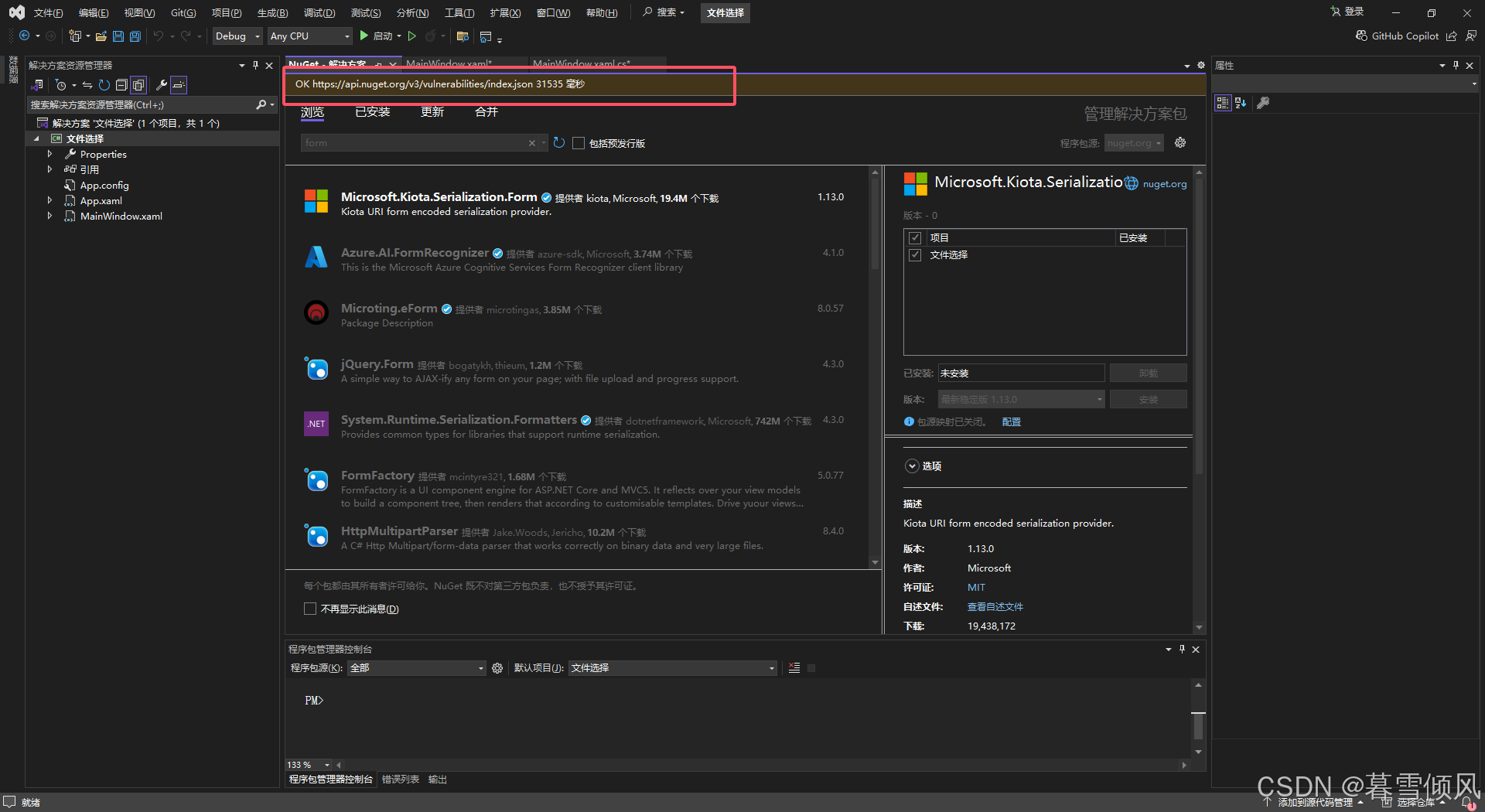
4、安装完成
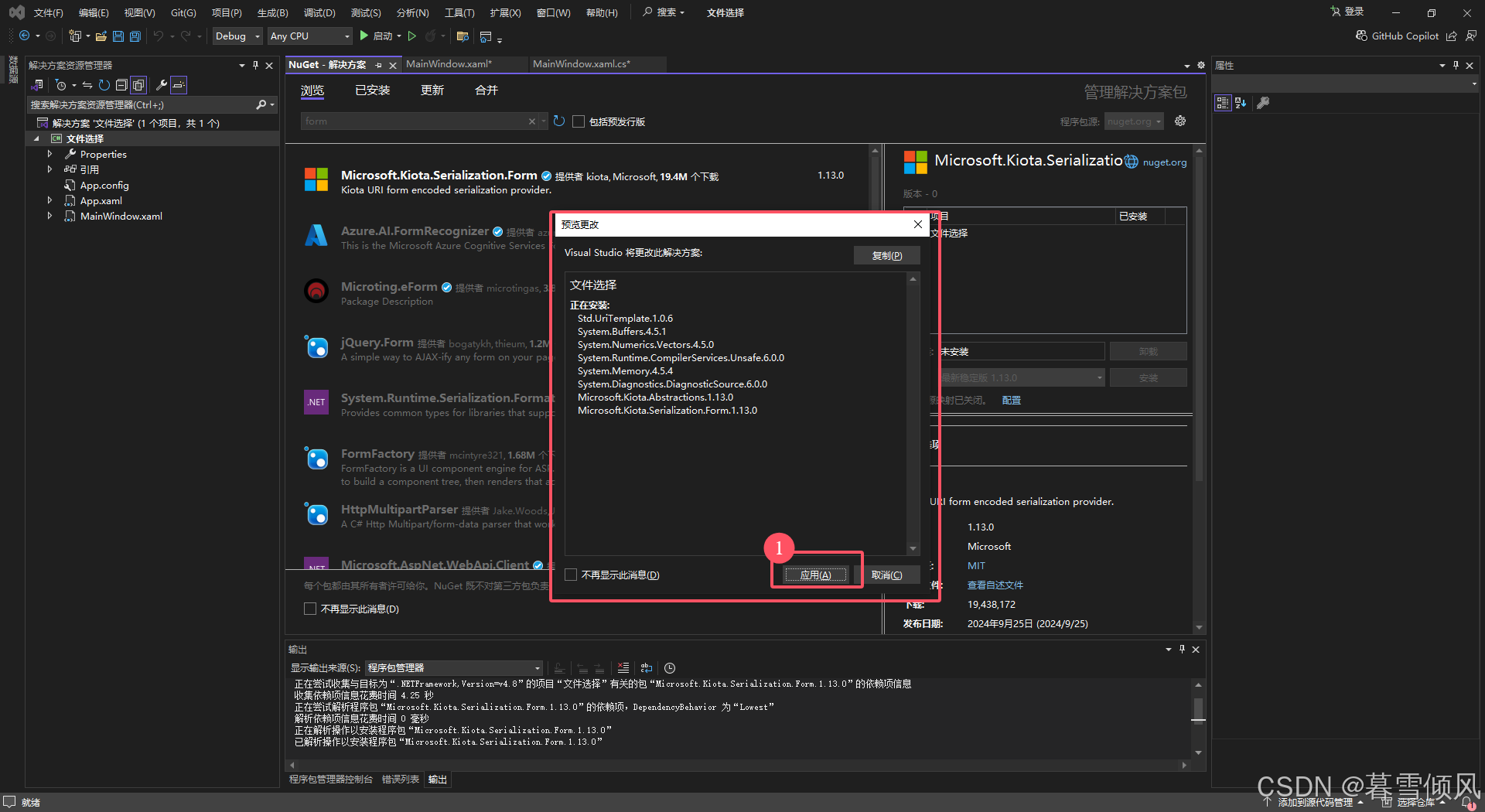
5、接收协议
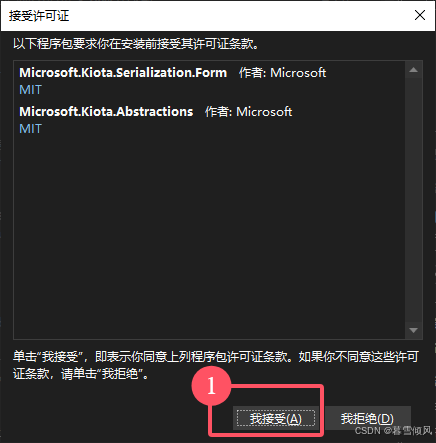
二、代码介绍
OpenFileDialog 属性
基础属性
Filter
:定义在对话框中显示的文件类型过滤器。FileName
:获取或设置在对话框中选定的文件的名称。FilterIndex
:获取或设置选定文件过滤器索引。
进阶属性
Multiselect
:获取或设置一个值,该值指示对话框是否允许选择多个文件。InitialDirectory
:获取或设置对话框显示的初始目录。Title
:获取或设置对话框标题。AddExtension
:获取或设置一个值,该值指示在用户输入文件名但没有指定扩展名时,对话框是否自动添加扩展名。
高级属性
CheckFileExists
:获取或设置一个值,该值指示对话框是否检查选定文件是否存在。CheckPathExists
:获取或设置一个值,该值指示对话框是否检查路径是否存在。DefaultExt
:获取或设置默认文件扩展名。RestoreDirectory
:获取或设置一个值,该值指示对话框关闭后,是否恢复当前目录到对话框打开前的状态。ShowHelp
:获取或设置一个值,该值指示对话框是否显示帮助按钮。
SaveFileDialog 属性
SaveFileDialog
类具有与 OpenFileDialog
类相似的大多数属性,以下是 SaveFileDialog
特有的属性:
基础属性
CreatePrompt
:获取或设置一个值,该值指示如果用户指定不存在的文件,对话框是否提示创建文件。OverwritePrompt
:获取或设置一个值,该值指示如果用户指定已存在的文件,对话框是否提示覆盖文件。
进阶属性
ValidateNames
:获取或设置一个值,该值指示对话框是否验证文件名。
高级属性
AddExtension
:与OpenFileDialog
相同,但在保存文件时,如果用户没有输入扩展名,对话框会自动添加默认扩展名。
三、代码编写
1、添加依赖
在cs文件头部添加代码
cs
using System.Windows.Forms;
2、添加布局
XML
<StackPanel HorizontalAlignment="Stretch" VerticalAlignment="Center" Orientation="horizontal">
<Border Background="#ffffff" Padding="5" CornerRadius="15" Width="800">
<Grid HorizontalAlignment="Stretch" Height="60">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="9*" />
<ColumnDefinition Width="1*" />
</Grid.ColumnDefinitions>
<TextBox Grid.Column="0" x:Name="textboxFilePath"/>
<Button Grid.Column="1" x:Name="botOpenFile" Content="打开文件" Click="botOpenFile_Click"/>
</Grid>
</Border>
</StackPanel>
3、添加事件
cs
private void botOpenFile_Click(object sender, RoutedEventArgs e)
{
System.Windows.Forms.OpenFileDialog openFileDialog = new System.Windows.Forms.OpenFileDialog();
openFileDialog.Filter = "文本文件|*.mxl;*.doc;*.html;*.txt|视频文件|*.mp4;*.avi|所有文件|*.*";
openFileDialog.InitialDirectory = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments);
openFileDialog.Title = "打开文本文件";
openFileDialog.Multiselect = false;
// 显示文件对话框
if (openFileDialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
// 获取用户选择的文件路径
textboxFilePath.Text = openFileDialog.FileName;
}
}
四、完整代码
xaml代码
XML
<Window x:Class="文件选择.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:文件选择"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800" Background="#838383" >
<StackPanel HorizontalAlignment="Stretch" VerticalAlignment="Center" Orientation="horizontal">
<Border Background="#ffffff" Padding="5" CornerRadius="15" Width="800">
<Grid HorizontalAlignment="Stretch" Height="60">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="9*" />
<ColumnDefinition Width="1*" />
</Grid.ColumnDefinitions>
<TextBox Grid.Column="0" x:Name="textboxFilePath"/>
<Button Grid.Column="1" x:Name="botOpenFile" Content="打开文件" Click="botOpenFile_Click"/>
</Grid>
</Border>
</StackPanel>
</Window>
cs代码
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Windows.Forms;
namespace 文件选择
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void botOpenFile_Click(object sender, RoutedEventArgs e)
{
System.Windows.Forms.OpenFileDialog openFileDialog = new System.Windows.Forms.OpenFileDialog();
openFileDialog.Filter = "文本文件|*.mxl;*.doc;*.html;*.txt|视频文件|*.mp4;*.avi|所有文件|*.*";
openFileDialog.InitialDirectory = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments);
openFileDialog.Title = "打开文本文件";
openFileDialog.Multiselect = false;
// 显示文件对话框
if (openFileDialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
// 获取用户选择的文件路径
textboxFilePath.Text = openFileDialog.FileName;
}
}
}
}
五、效果演示
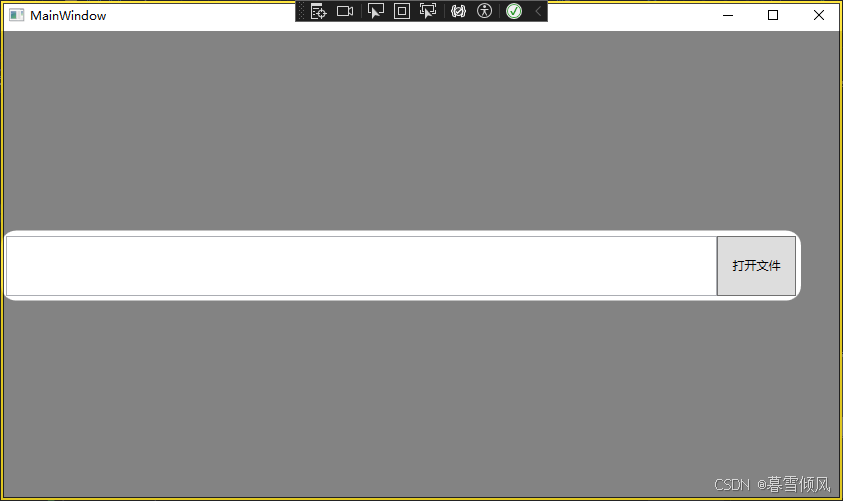
六、工程下载
通过百度网盘分享的文件:文件选择.ziphttps://pan.baidu.com/s/1-Wb0RlPJse-EfVID25JujQ