这是我记录Qt学习过程心得文章的第二篇,主要是为了方便QMessageBox弹出框的使用,通过自定义的方式,将其常用的功能,统一封装成一个函数,还是写在了Skysonya类里面。
实现代码:
cpp
//中文提示对话框
bool Skysonya::messageBox(QString msgType, QString dlgTitle, QString strInfo)
{
QMessageBox *msgBox;
int result = -1;
bool value = false;
if (msgType.toLower() == "critical")
{
//创建一个Critical弹出对话框,添加按钮:"Ok"
msgBox = new QMessageBox(QMessageBox::Critical, dlgTitle, strInfo, QMessageBox::Ok);
msgBox->button(QMessageBox::Ok)->setText("确定"); //将"Ok"按钮改为显示"确定"
result = msgBox->exec(); //显示Critical弹出对话框
if (result == QMessageBox::Ok)
{
value = true;
}
}
else if (msgType.toLower() == "warning")
{
//创建一个Warning弹出对话框,添加按钮:"Ok"
msgBox = new QMessageBox(QMessageBox::Warning, dlgTitle, strInfo, QMessageBox::Ok);
msgBox->button(QMessageBox::Ok)->setText("确定"); //将"Ok"按钮改为显示"确定"
result = msgBox->exec(); //显示Warning弹出对话框
if (result == QMessageBox::Ok)
{
value = true;
}
}
else if (msgType.toLower() == "warningyes")
{
//创建一个Warning弹出对话框,添加按钮:"Yes"、"No"
msgBox = new QMessageBox(QMessageBox::Warning, dlgTitle, strInfo, QMessageBox::Yes | QMessageBox::No);
msgBox->button(QMessageBox::Yes)->setText("是"); //将"Yes"按钮改为显示"是"
msgBox->button(QMessageBox::No)->setText("否"); //将"No"按钮改为显示"否"
result = msgBox->exec(); //显示Warning弹出对话框
if (result == QMessageBox::Yes)
{
value = true;
}
}
else if (msgType.toLower() == "information")
{
//创建一个Information弹出对话框,添加按钮:"Ok"
msgBox = new QMessageBox(QMessageBox::Information, dlgTitle, strInfo, QMessageBox::Ok);
msgBox->button(QMessageBox::Ok)->setText("确定"); //将"Ok"按钮改为显示"确定"
result = msgBox->exec(); //显示Information弹出对话框
if (result == QMessageBox::Ok)
{
value = true;
}
}
else if (msgType.toLower() == "informationyes")
{
//创建一个Information弹出对话框,添加按钮:"Ok"
msgBox = new QMessageBox(QMessageBox::Information, dlgTitle, strInfo, QMessageBox::Yes | QMessageBox::No);
msgBox->button(QMessageBox::Yes)->setText("是"); //将"Yes"按钮改为显示"是"
msgBox->button(QMessageBox::No)->setText("否"); //将"No"按钮改为显示"否"
result = msgBox->exec(); //显示Information弹出对话框
if (result == QMessageBox::Yes)
{
value = true;
}
}
return value;
}
具体使用:
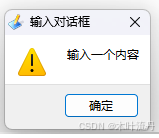
cpp
//文件
void MainWindow::slot_file_triggered()
{
//输入对话框
QString strInfo = skysonya.inputDialog("对话框", "请输入:");
skysonya.messageBox("warning", "输入对话框", strInfo);
}
自定义类:
cpp
#ifndef SKYSONYA_H
#define SKYSONYA_H
#include <QDebug>
#include <QFile>
#include <QInputDialog>
#include <QMessageBox>
#include <QObject>
#include <QPushButton>
#include <QString>
#include <QTextCodec>
enum EncodingFormat
{
ANSI,
UTF16LE,
UTF16BE,
UTF8,
UTF8BOM,
};
class Skysonya : public QObject
{
Q_OBJECT
Q_ENUM(EncodingFormat)
public:
explicit Skysonya(QObject *parent = nullptr);
~Skysonya();
QString doAppAbout(QString appName); //程序关于信息
bool messageBox(QString msgType, QString dlgTitle, QString strInfo); //中文提示对话框
QString inputDialog(QString dlgTitle, QString labelText, QString textValue = ""); //中文按钮文本输入对话框
QTextCodec *getFileCharacterEncoding(const QString &fileName); //获取文件编码格式函数
QString openFileByIOWhole(const QString &fileName); //用QFile打开文件,整体读取
QString openFileByIOLines(const QString &fileName); //用QFile打开文件,逐行读取
QString openFileByStreamWhole(const QString &fileName); //用QTextStream读取文件,整体读取
QString openFileByStreamLines(const QString &fileName); //用QTextStream读取文件,逐行读取
bool saveFileByIOWhole(const QString &fileName, QString text); //用QFile保存文件,整体保存
bool saveFileByStreamWhole(const QString &fileName, QString text); //用QTextStream保存文件,整体保存
private:
QString appVersion; //软件版本号
QString buildTime; //程序构建时间
QString qtVersion; // QT版本号
QString fun_buildTime(); //获取程序构建时间
};
#endif // SKYSONYA_H
完整的示例地址:https://download.csdn.net/download/skysonya_shisy/89861254