目录
- 前言
- [1. 基本知识](#1. 基本知识)
- [2. Demo](#2. Demo)
前言
基本的Java知识推荐阅读:
从实战中学习启发

1. 基本知识
@ConditionalOnProperty 是 Spring Framework 中的一个注解,用于根据特定的属性值条件性地加载 Spring 组件
通常用于配置类或组件中,以便在满足特定条件时激活某个 Bean
基本的概念如下:
- 用途:@ConditionalOnProperty 用于条件性地创建和配置 Spring Bean,当某个特定的配置属性存在并且符合特定值时,才会创建该 Bean
- 属性:
name
:需要检查的属性名,可以是一个或多个属性名
havingValue
:属性的值,只有当属性值与此相等时,条件才会成立。默认值为空字符串
matchIfMissing
:当指定的属性不存在时,是否匹配。默认值为 false,表示如果属性缺失,则条件不成立
基本的作用 有如下:
- 灵活配置:可以通过外部配置(如 application.properties 或 application.yml)来控制 Bean 的加载,支持不同环境下的配置
- 减少启动时间:只在需要时加载特定的 Bean,从而减少应用程序的启动时间和内存占用
- 增强模块化:通过条件性加载,可以实现功能模块的分离和组合,使得应用更具可扩展性
2. Demo
整体架构分别如下:
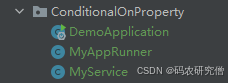
对应的代码如下:
java
// DemoApplication.java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
// MyService.java
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.stereotype.Service;
@Service
@ConditionalOnProperty(name = "app.feature.enabled", havingValue = "true", matchIfMissing = false)
public class MyService {
public void execute() {
System.out.println("MyService is active!");
}
}
// MyAppRunner.java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class MyAppRunner implements CommandLineRunner {
@Autowired(required = false) // 使得该注入为可选
private MyService myService;
@Override
public void run(String... args) {
if (myService != null) {
myService.execute();
} else {
System.out.println("MyService is not active!");
}
}
}
在 src/main/resources/application.properties 中添加以下配置:
xml
# 启用特性
app.feature.enabled=true
如果将 app.feature.enabled 的值设置为 false 或不设置该属性,则 MyService 不会被激活,控制台将输出 MyService is not active!
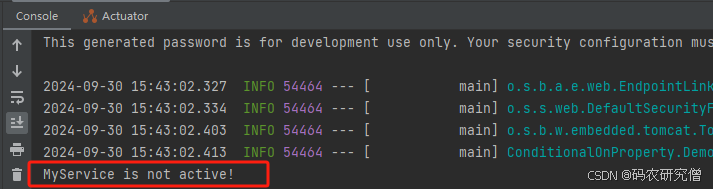
设置之后,将会被激活
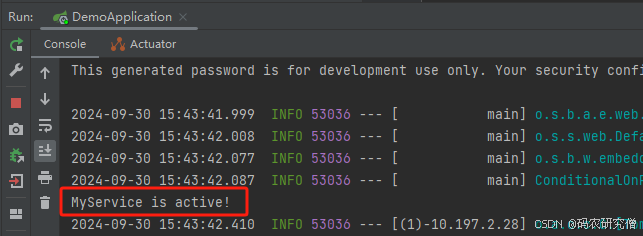