MT1331·用函数求π的近似值
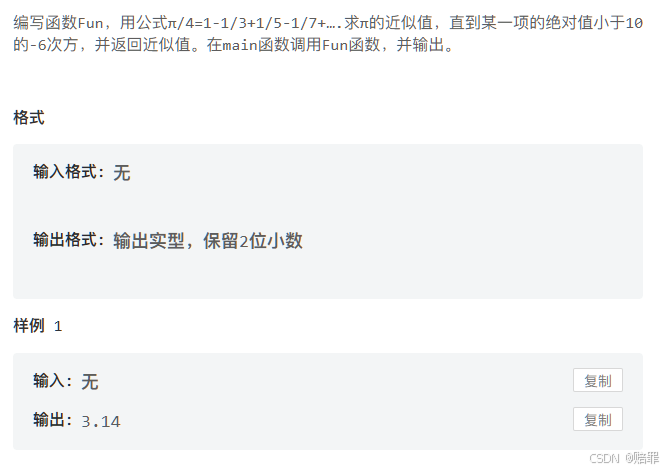
c 语言代码实现
#include <math.h>
#include <stdio.h>
double Fun() {
double pi_over_4 = 0.0; // Π / 4 的近似值
double current; // 当前项
int i = 0; // 项的索引
do {
current = (i % 2 == 0 ? 1.0 : -1.0) / (2.0 * i + 1.0); // 计算当前项
pi_over_4 += current; // 累加当前项
i++;
} while (fabs(current) >= 1e-6); // 小于等于 10^-6
return pi_over_4 * 4;
}
int main() {
double pi_approx = Fun();
printf("%.2f", pi_approx);
return 0;
}
MT1332·用函数求最大值
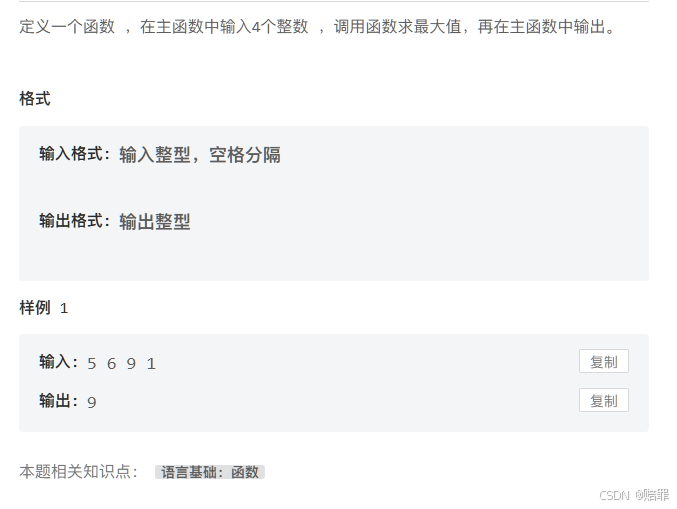
c 语言代码实现
#include <stdio.h>
int max_1(int a, int b) {
if (a > b)
return a;
return b;
}
int max(int a, int b, int c, int d) {
if (max_1(a, b) > max_1(c, d))
return max_1(a, b);
return max_1(c, d);
}
int main() {
int a, b, c, d;
scanf("%d %d %d %d", &a, &b, &c, &d);
printf("%d",max(a, b, c, d));
return 0;
}
MT1333·用函数求最小值
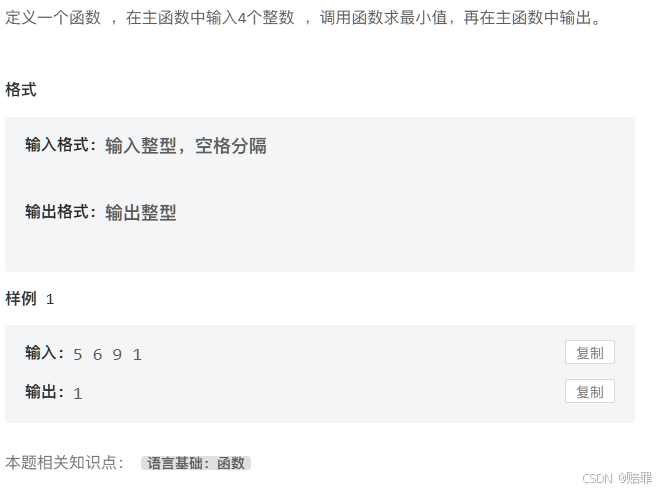
c 语言实现代码
#include <stdio.h>
int min_1(int a, int b) {
if (a > b)
return b;
return a;
}
int min(int a, int b, int c, int d) {
if (min_1(a, b) > min_1(c, d))
return min_1(c, d);
return min_1(a, b);
}
int main() {
int a, b, c, d;
scanf("%d %d %d %d", &a, &b, &c, &d);
printf("%d", min(a, b, c, d));
return 0;
}
MT1334·最小整数
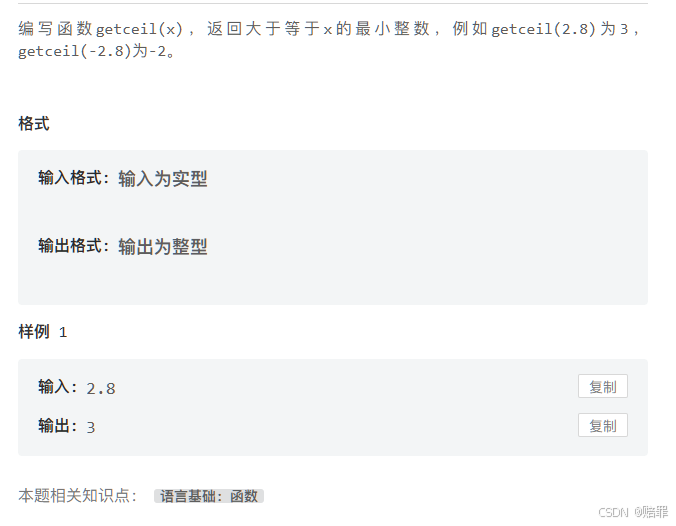
c 语言代码实现
#include <math.h>
#include <stdio.h>
double getceil(double x) { return ceil(x); }
int main() {
double x;
scanf("%lf", &x);
printf("%.0lf", getceil(x));
return 0;
}
MT1335·最大整数
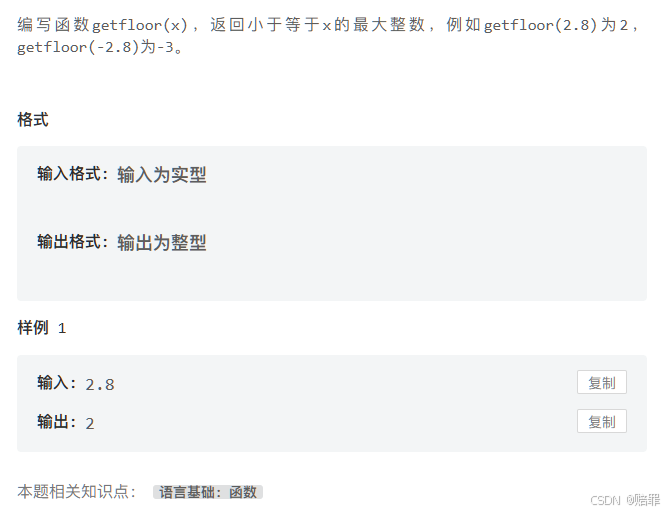
c 语言代码实现
#include <math.h>
#include <stdio.h>
double getfloor(double x) { return floor(x); }
int main() {
double x;
scanf("%lf", &x);
printf("%.0lf", getfloor(x));
return 0;
}
MT1336·用函数求阶乘
c语言代码实现
#include <stdio.h>
int fact(int x) {
if (x == 1)
return 1;
return x * fact(x - 1);
}
int main() {
int x;
scanf("%d", &x);
printf("%d", fact(x));
return 0;
}
MT1337·n次方
c 语言代码实现
#include <math.h>
#include <stdio.h>
int fun(int m, int n) { return pow(m, n); }
int main() {
int m, n;
scanf("%d %d", &m, &n);
printf("%d", fun(m, n));
return 0;
}
MT1338·开n次方
c 语言代码实现
#include <math.h>
#include <stdio.h>
double S(double x, int n) { return pow(x, 1.0 / n); }
int main() {
double x;
int n;
scanf("%lf %d", &x, &n);
printf("%lf", S(x, n));
return 0;
}
MT1339·平均数
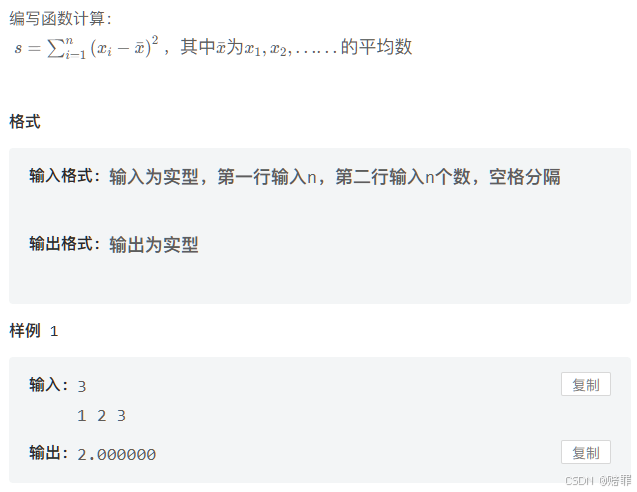
c 代码实现
#include <math.h>
#include <stdio.h>
// 函数声明
double calculate_sum_of_squares(double arr[], int n);
int main() {
// 示例数组
double data[100];
int n;
scanf("%d", &n);
for (int i = 0; i < n; i++) {
scanf("%lf", &data[i]);
}
// 计算平方差和
double result = calculate_sum_of_squares(data, n);
printf("%lf", result);
return 0;
}
// 计算平方差和的函数
double calculate_sum_of_squares(double arr[], int n) {
if (n <= 0)
return 0; // 如果数组长度为0,返回0
// 计算平均数
double sum = 0;
for (int i = 0; i < n; i++) {
sum += arr[i];
}
double mean = sum / n;
// 计算平方差和
double sum_of_squares = 0;
for (int i = 0; i < n; i++) {
sum_of_squares += pow(arr[i] - mean, 2);
}
return sum_of_squares;
}
MT1340·平均值函数
c 语言实现代码
#include <stdio.h>
double avg(int arr[100], int begin, int end) {
double sum = 0, mean = 0;
for (int i = begin; i <= end; i++) {
sum += arr[i];
}
mean = sum / (end - begin + 1);
return mean;
}
int main() {
int n, begin, end, a[100];
scanf("%d %d %d", &n, &begin, &end);
for (int i = 0; i < n; i++) {
scanf("%d", &a[i]);
}
printf("%lf", avg(a, begin, end));
return 0;
}