效果:鼠标按下Tab建可以选选择标签或者方块之间的切换
这段代码使用了 QtQuick 框架,创建了一个包含两个 Text
元素和两个嵌套 Rectangle
的用户界面。以下是对代码中涉及的主要知识点和实现细节的介绍:
知识点及代码细节介绍
-
导入 QtQuick
qmlimport QtQuick
QtQuick
是一个用于创建现代图形用户界面的核心模块,包含布局、动画、用户交互等功能。
-
根容器 (
Rectangle
)qmlRectangle { id: root width: 600 height: 300 } - `Rectangle` 作为整个界面的根容器。 - 设置 `width` 和 `height` 为 600 和 300 像素,定义了窗口的大小。 - `id: root` 用于标识该矩形,其他元素可以通过 `root` 来引用该对象。
-
Text 元素 -
thisLabel
qmlText { id: thisLabel width: 150 height: 100 x: 24 y: 100 ... }
Text
元素用于显示文本内容。- 设置
id: thisLabel
,标识符用于唯一标识该元素,方便在代码中引用。 width
和height
设置了文本框的大小为 150x100 像素。x: 24
和y: 100
设置了文本框在root
容器内的相对位置。
-
嵌套的
Rectangle
qmlRectangle { anchors.fill: parent color: "yellow" z: parent.z - 1 }
Rectangle
作为嵌套在Text
内部的背景元素。- 使用
anchors.fill: parent
使得背景矩形填满Text
元素的区域。 color: "yellow"
设置了背景颜色为黄色。z: parent.z - 1
设置了z
值,以控制元素的层次顺序。z
值越小,越靠后显示,因此该矩形会在文本内容的背后显示。
-
自定义属性 (
property
) 和别名属性 (property alias
)qmlproperty int times: 24 property alias anotherTimes: thisLabel.times
property int times: 24
定义了一个自定义整数属性times
,初始值为 24。该属性可以用于存储和管理与该对象相关的数据。property alias anotherTimes: thisLabel.times
定义了一个属性别名,将thisLabel
的times
属性作为一个引用,这样其他组件可以通过anotherTimes
来访问或修改thisLabel.times
的值。
-
设置文本内容 (
text
)qmltext: "thisLabel " + anotherTimes
text
属性用于设置Text
元素显示的内容。- 这里使用字符串连接的方式将
"thisLabel "
和anotherTimes
(即thisLabel.times
)连接在一起,用于显示动态内容。
-
字体属性 (
font
)qmlfont.family: "Ubuntu" font.pixelSize: 16
font
是一个分组属性,包含了字体设置。font.family: "Ubuntu"
设置字体为 Ubuntu 字体。font.pixelSize: 16
设置字体的大小为 16 像素。
-
键盘导航 (
KeyNavigation
)qmlKeyNavigation.tab: thatLabel
KeyNavigation
是一个附加属性,用于处理键盘事件,例如焦点的移动。KeyNavigation.tab: thatLabel
表示按下 Tab 键时,将焦点移到thatLabel
元素。
-
信号处理程序 (
onHeightChanged
)qmlonHeightChanged: console.log('height:', height)
onHeightChanged
是一个信号处理程序,当Text
元素的height
属性发生变化时会被触发。- 使用
console.log('height:', height)
打印当前的高度值,便于调试。
-
焦点管理 (
focus
)qmlfocus: true
focus: true
表示该元素有焦点,能够接收键盘输入。focus
也用来控制文本颜色,如果当前元素有焦点,则文本为红色 (color: focus ? "red" : "black"
),否则为黑色。
-
第二个
Text
元素 -thatLabel
qmlText { id: thatLabel width: thisLabel.width height: thisLabel.height x: thisLabel.x + thisLabel.width + 20 y: thisLabel.y ... }
- 定义了另一个
Text
元素,标识符为thatLabel
。 - 它的宽度和高度与
thisLabel
相同,确保两个文本框大小一致。 - 通过
x
设置,使thatLabel
位于thisLabel
的右侧,并有 20 像素的间距。 - 该元素也有一个嵌套的
Rectangle
,颜色设置为粉色 (color: "pink"
),并位于文本的背后 (z: parent.z - 1
)。
- 定义了另一个
-
键盘焦点切换
qmlfocus: !thisLabel.focus KeyNavigation.tab: thisLabel
focus: !thisLabel.focus
表示当thisLabel
没有焦点时,thatLabel
会获得焦点。- 通过
KeyNavigation.tab
属性,按下 Tab 键可以将焦点切换回thisLabel
。
需要注意的知识点
-
嵌套组件及相对布局:
- 代码中使用了嵌套的
Rectangle
元素作为背景,同时通过anchors.fill: parent
来填满父组件。 - 使用
x
和y
设置了元素的绝对位置,也可以考虑使用anchors
来实现更灵活的相对布局,适应不同尺寸的窗口。
- 代码中使用了嵌套的
-
属性系统:
property
和property alias
是 QML 中非常重要的概念,用于定义和引用属性。property alias
可以让不同组件共享属性,方便数据的访问和管理。
-
键盘事件和焦点管理:
KeyNavigation
和focus
用于处理键盘焦点的切换。- 焦点管理在交互式界面设计中非常重要,确保用户可以通过键盘顺利地切换和控制元素。
-
z 值控制元素层次:
- 使用
z
控制元素的前后显示顺序,z
值越大,元素越靠前显示。 - 可以通过设置
z
值调整文本和背景矩形之间的显示关系。
- 使用
-
动态文本和信号处理:
- 通过连接字符串的方式实现动态文本更新。
- 信号处理器 (
onHeightChanged
) 用于监测属性的变化,这在调试和动态界面更新中非常有用。
这段代码展示了如何使用 QtQuick 实现一个具有动态属性、键盘导航、和简单动画效果的用户界面。掌握这些基础知识是创建复杂和交互式 QML 应用的关键。
c
import QtQuick
Rectangle {
id: root
width: 600
height: 300
Text {
// (1) identifier
id: thisLabel
// 设置宽度和高度
width: 150
height: 100
// (2) set x- and y-position
x: 24
y: 100
Rectangle {
anchors.fill: parent
color: "yellow"
z: parent.z - 1
}
// (4) custom property
property int times: 24
// (5) property alias
property alias anotherTimes: thisLabel.times
// (6) set text appended by value
text: "thisLabel " + anotherTimes
// (7) Font is a grouped property
font.family: "Ubuntu"
font.pixelSize: 16
// (8) KeyNavigation is an attached property
KeyNavigation.tab: thatLabel
// (9) Signal handler for property changes
onHeightChanged: console.log('height:', height)
// Focus is needed to receive key events
focus: true
color: focus ? "red" : "black"
}
Text {
id: thatLabel
// 设置与 thisLabel 相同的宽度和高度
width: thisLabel.width
height: thisLabel.height
// 设置位置在 thisLabel 右边,并有 20 像素的间距
x: thisLabel.x + thisLabel.width + 20
y: thisLabel.y
Rectangle {
anchors.fill: parent
color: "pink"
z: parent.z - 1
}
text: "thatLabel"
font.family: "Ubuntu"
font.pixelSize: 16
focus: !thisLabel.focus
KeyNavigation.tab: thisLabel
color: focus ? "red" : "black"
}
}
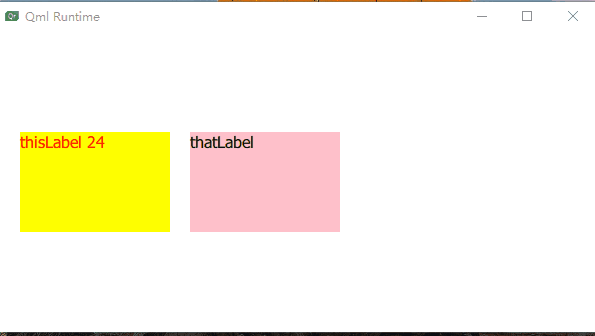