前言
SpringMVC本身提高了便捷的文件上传方式,接下来我将演示如何在SpringMVC中实现文件上传的相关操作。以下案例是基于SSM框架整合的基础上实现的文件上传功能,如何进行SSM整合,可以参考我以往的博客。
第一步:添加依赖
pom.xml
XML
<!-- 文件上传与下载-->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.4</version>
</dependency>
第二步:创建springmvc配置文件,添加文件上传配置
spring-mvc.xml
XML
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.1.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.1.xsd
http://www.springframework.org/schema/util
http://www.springframework.org/schema/util/spring-util-4.1.xsd">
<context:component-scan base-package="com.csx"/>
<mvc:annotation-driven/>
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" p:prefix="/" p:suffix=".jsp"/>
<!--文件上传 2.加配置,注意id-->
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="maxUploadSize" value="104857600"/>
<property name="defaultEncoding" value="UTF-8"/>
</bean>
</beans>
开启扫描
开启注解
配置视图解析器
配置文件上传相关配置
- 一定要配置id,不能省略,否则报错(底层根据id加载这个bean)
第三步:创建文件上传页面
up.html
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<!--文件上传 3.写页面-->
<form action="/user/up.do" method="post" enctype="multipart/form-data">
文件:<input type="file" name="file"> <br/>
<input type="submit" value="提交">
</form>
</body>
</html>
- 请求方式只能是post
- 注意添加enctype属性
- 文件上传的type为file,注意name属性值要与controller层的参数名一致
第四步:写controller,上传到指定文件夹
UserController
java
package com.csx.controller;
import com.csx.entity.User;
import com.csx.service.UserService;
import com.csx.util.PageUtil;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import java.io.File;
import java.io.IOException;
import java.util.List;
import java.util.UUID;
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
/*文件上传 4.写controller,上传至指定文件夹*/
@RequestMapping("/up")
public String fileUpload(MultipartFile file, HttpServletRequest request) throws IOException {
//创建文件夹,上传文件
//获得完整路径
String realPath = request.getSession().getServletContext().getRealPath("/upload");
System.out.println("realPath===" + realPath);
//创建文件夹
File f = new File(realPath);
//如果文件夹不存在,就创建
if (!f.exists()) {
f.mkdirs();
}
//拿到上传的文件名
String fileName = file.getOriginalFilename();
//将UUID和文件名拼接,防止文件名重名而覆盖
fileName = UUID.randomUUID() + "_" + fileName;
//创建空文件
File newFile = new File(f, fileName);
//将文件内容写入
file.transferTo(newFile);
return "index";
}
}
代码解析
- 文件上传,controller使用MultipartFile 类型进行接收,并且参数名与页面的属性值保持一致
String realPath = request.getSession().getServletContext().getRealPath("/upload"); System.out.println("realPath===" + realPath);
- 获取完整路径,指定是绝对路径,包括盘符的字符串
//创建文件夹 File f = new File(realPath); //如果文件夹不存在,就创建 if (!f.exists()) { f.mkdirs(); }
- 如果文件夹不存在,则创建文件夹upload
//拿到上传的文件名 String fileName = file.getOriginalFilename(); //将UUID和文件名拼接,防止文件名重名而覆盖 fileName = UUID.randomUUID() + "_" + fileName;
- 拿到原始文件名,并且使用UUID进行覆盖,防止上传同名文件导致文件覆盖
//创建空文件 File newFile = new File(f, fileName); //将文件内容写入 file.transferTo(newFile); return "index";
- 创建空文件,写入内容,返回Json格式数据
演示效果
地址栏输入http://localhost:8080/up.html
点击选择文件,选择第5.jpg
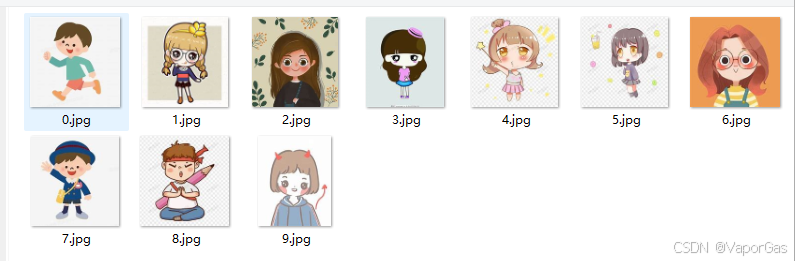
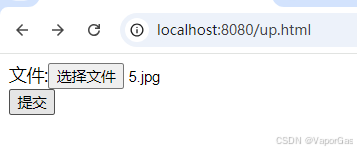
点击提交
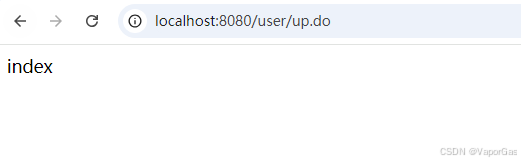
返回index,就是我们return的String数据,表示上传成功,查看upload文件夹
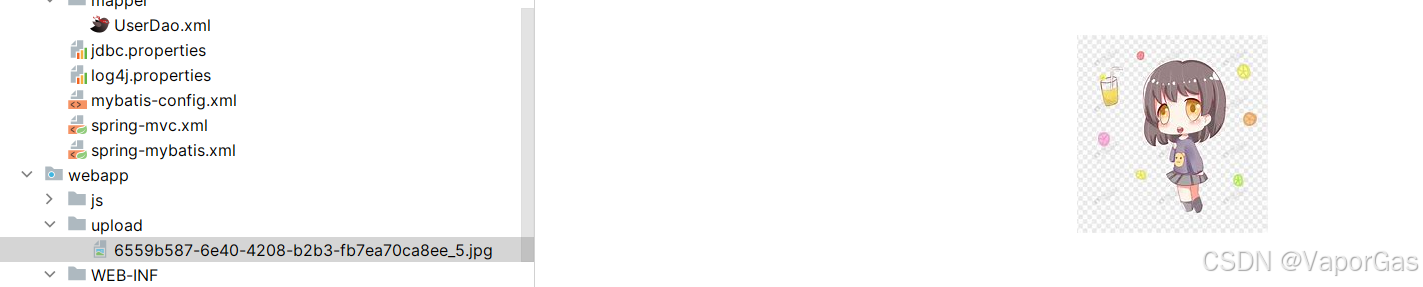
文件上传成功!