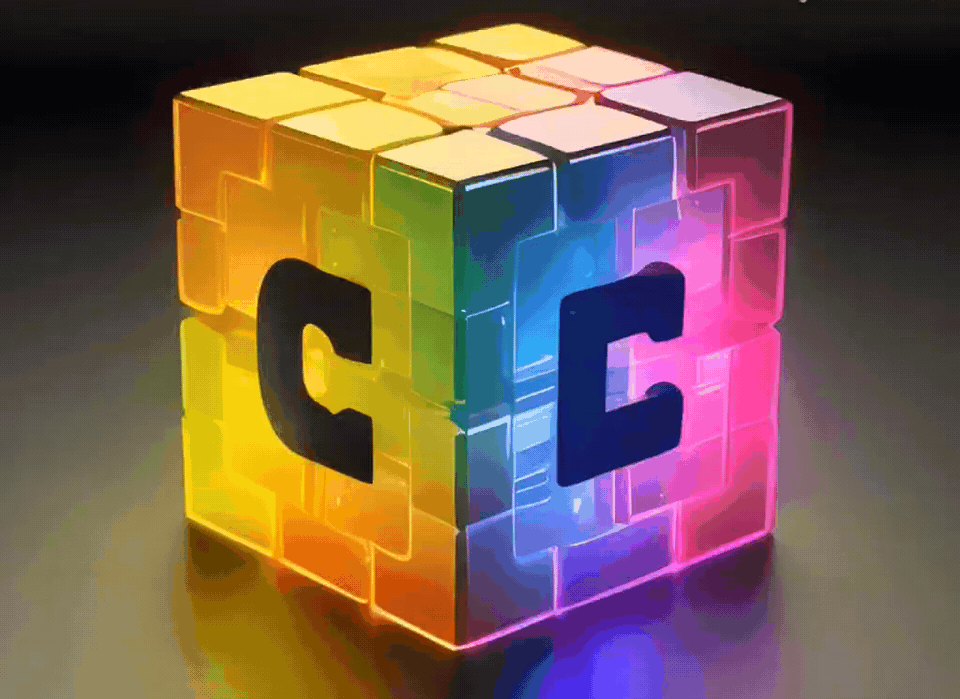
1. 引言
在计算机科学中,理解和测量程序的运行时间对于优化性能至关重要。C语言提供了多种工具来帮助开发者进行时间统计。本文旨在提供一个全面而深入的理解框架,使读者能够有效地利用这些工具。
2. 时间表示与获取
C语言中使用time_t
类型来表示时间戳,通常是自1970年1月1日午夜以来的秒数(不包括闰秒)。通过time()
函数可以轻松获取当前的时间戳。
c
#include <time.h>
int main(void) {
time_t t;
time(&t);
printf("Current time: %s", ctime(&t));
return 0;
}
3. 精确计时
3.1 clock()
函数
clock()
函数提供了一种简单的方法来测量程序或部分代码块的执行时间。需要注意的是,它测量的是用户态下的CPU时间,而不是实际经过的时间。CLOCKS_PER_SEC
宏定义了每秒钟的clock tick
数量,这个数值依赖于具体的系统实现。
c
#include <time.h>
#include <stdio.h>
int main(void) {
clock_t start, end;
double cpu_time_used;
start = clock();
// Code to measure
end = clock();
cpu_time_used = ((double) (end - start)) / CLOCKS_PER_SEC;
printf("Time used: %f\n", cpu_time_used);
return 0;
}
3.2 gettimeofday()
对于需要更高精度的情况,可以使用gettimeofday()
函数。它返回的是相对于某个固定点的时间,通常精度达到微秒级别。struct timeval
结构体包含两个字段:tv_sec
(秒数)和tv_usec
(微秒数)。
c
#include <sys/time.h>
#include <stdio.h>
int main(void) {
struct timeval tv;
gettimeofday(&tv, NULL);
printf("Microseconds since the epoch: %ld.%06ld\n",
(long) tv.tv_sec, tv.tv_usec);
return 0;
}
4. 进程时间统计
除了测量程序的执行时间外,我们还经常需要了解程序消耗了多少CPU时间。这可以通过times()
函数来实现。times()
函数返回自进程启动以来的用户时间和系统时间,单位是tms tick
。
c
#include <sys/times.h>
#include <stdio.h>
int main(void) {
struct tms tms;
clock_t ticks;
ticks = times(&tms);
printf("User time: %ld\nSystem time: %ld\nTotal time: %ld\n",
tms.tms_utime, tms.tms_stime, ticks / sysconf(_SC_CLK_TCK));
return 0;
}
5. 高级话题
5.1 多线程下的时间统计
在多线程环境中,我们需要更加细致地管理时间统计。可以使用clock_gettime()
函数来获取更精确的时间信息。该函数允许指定不同的时钟源,如CLOCK_MONOTONIC
,它提供了单调递增的时间值,非常适合用于时间测量。
c
#include <time.h>
#include <stdio.h>
#include <pthread.h>
void *thread_func(void *arg) {
struct timespec ts;
clock_gettime(CLOCK_MONOTONIC, &ts);
printf("Thread started at %ld.%ld\n", ts.tv_sec, ts.tv_nsec);
return NULL;
}
int main(void) {
pthread_t thread;
pthread_create(&thread, NULL, thread_func, NULL);
pthread_join(thread, NULL);
return 0;
}
5.2 实时性考虑
在实时系统中,时间的准确性尤为重要。开发者需要确保时间测量不受外部因素的影响,例如系统负载或中断。使用单调时钟如CLOCK_MONOTONIC
可以避免因系统时间调整导致的时间跳跃问题。
6. 实践案例
假设我们要编写一个程序来测试排序算法的效率,可以使用前面介绍的技术来测量其执行时间。
c
#include <stdlib.h>
#include <time.h>
#include <stdio.h>
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
void bubble_sort(int arr[], size_t n) {
for (size_t i = 0; i < n - 1; i++) {
for (size_t j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(&arr[j], &arr[j + 1]);
}
}
}
}
int main(void) {
int *arr = malloc(10000 * sizeof(int));
srand(time(NULL));
for (size_t i = 0; i < 10000; i++) {
arr[i] = rand();
}
clock_t start = clock();
bubble_sort(arr, 10000);
clock_t end = clock();
double cpu_time_used = ((double) (end - start)) / CLOCKS_PER_SEC;
printf("Bubble sort took %f seconds.\n", cpu_time_used);
free(arr);
return 0;
}
7. 总结
本文详细介绍了C语言中用于时间统计的各种工具和技术,从基本的时间表示到高级的多线程时间测量。通过对这些技术的掌握,开发者可以更好地优化自己的程序,并确保它们在各种环境下都能高效运行。