目录
open函数
man 2 open
打开已有文件
cpp
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
int main(){
int fd = open("a.txt",O_RDONLY);
if(fd==-1){
perror("open");
}
//关闭
close(fd);
return 0;
}
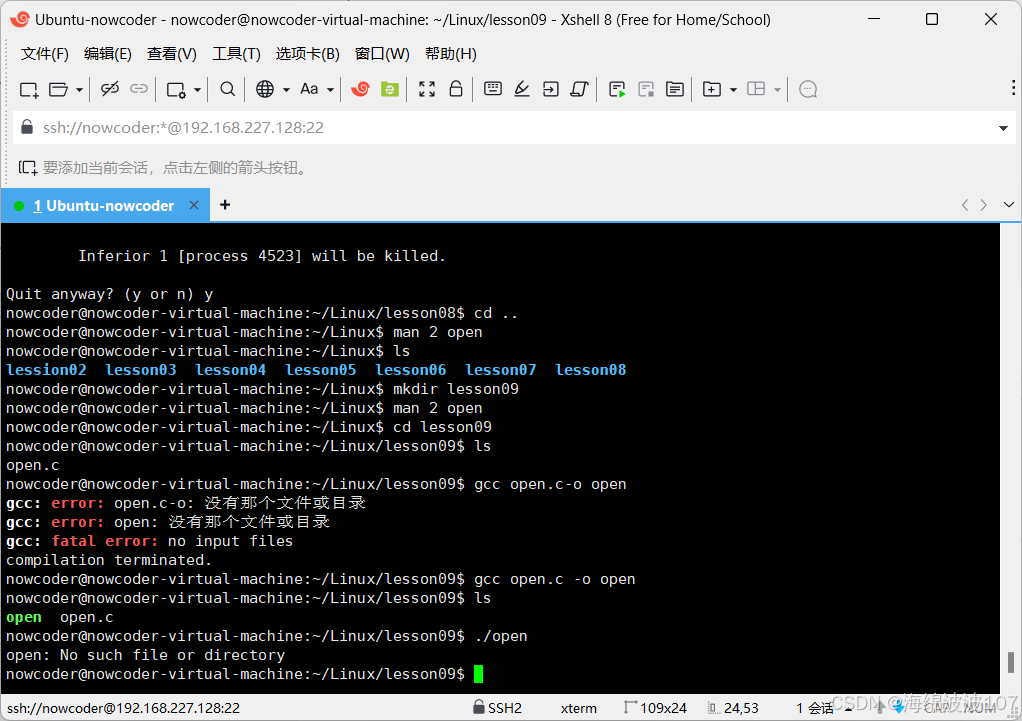
创建新文件
cpp
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <stdio.h>
int main(){
//创建一个新文件
int fd=open("create.txt",O_RDWR | O_CREAT,0777);
if(fd==-1){
perror("open");
}
//关闭
close(fd);
return 0;
}
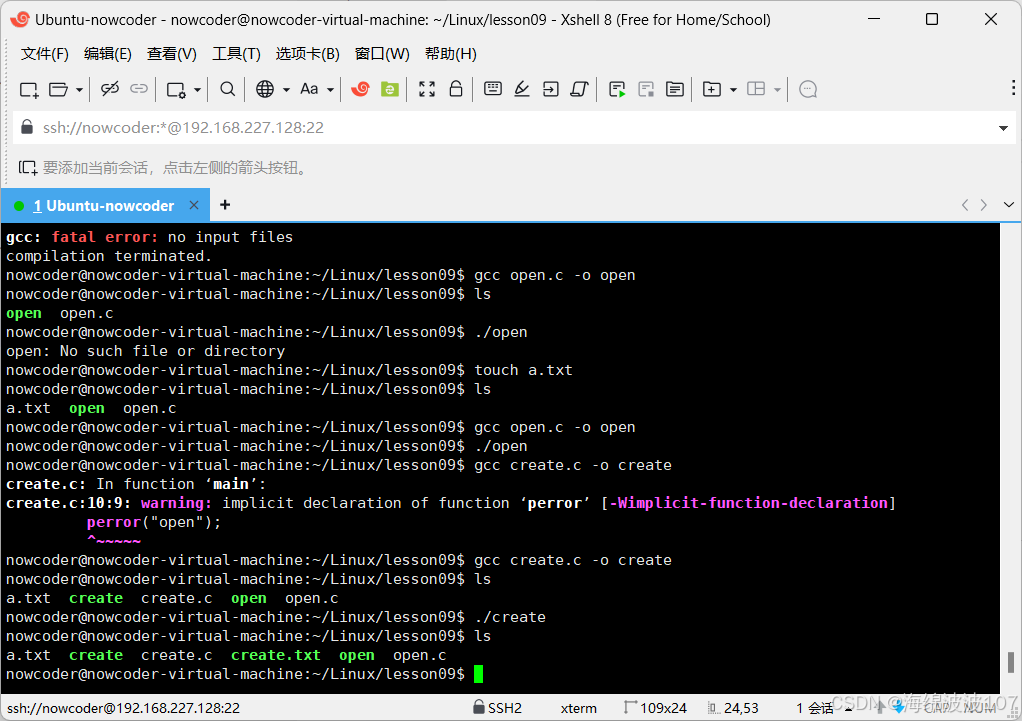
read和write函数
把文件全部读取并全部写到另一个文件中,拷贝操作
cpp
#include<unistd.h>
#include<stdio.h>
#include<sys/types.h>
#include<sys/stat.h>
#include<fcntl.h>
int main(){
//open打开english.txt文件
int srcfd=open("english.txt",O_RDONLY);
if(srcfd==-1){
perror("open");
return -1;
}
//创建一个新的文件(拷贝文件)
int destfd = open("cpy.txt",O_WRONLY | O_CREAT,0664);
if(destfd==-1){
perror("open");
return -1;
}
//频繁的读写操作
char buf[1024]={0};
int len=0;
while((len=read(srcfd,buf,sizeof(buf)))>0){
write(destfd,buf,len);
}
//关闭文件
close(destfd);
close(srcfd);
return 0;
}
写的文件和读取的文件大小一样,在vscode中查看内容一样,拷贝成功
lseek函数
移动文件头
lseek(fd,0,SEEK_SET)
获取当前文件指针位置
lseek(fd,0,SEEK_CUR)
获取文件长度
lseek(fd,0,SEEK_END)
拓展文件长度
lseek(fd,100,SEEK_END)
下面介绍拓展文件长度的用法
cpp
#include<sys/types.h>
#include<sys/stat.h>
#include<fcntl.h>
#include<unistd.h>
#include<stdio.h>
int main(){
int fd=open("hello.txt",O_RDWR);
if(fd==-1){
perror("open");
return -1;
}
//拓展文件的长度
int ret=lseek(fd,100,SEEK_END);
if(ret==-1){
perror("lseek");
return -1;
}
write(fd,"",1);
close(fd);
return 0;
}
拓展文件长度的时候,需要写一次数据,可以加入一个空的字符串
stat和lstat函数
stat直接获取指向的文件的信息(哪怕前面有软链接,也会指向最终那个文件信息)
查看文件状态
cpp
#include<sys/types.h>
#include<sys/stat.h>
#include<fcntl.h>
#include<unistd.h>
#include<stdio.h>
int main(){
struct stat statbuf;
int ret=stat("a.txt",&statbuf);
if(ret==-1){
perror("stat");
return -1;
}
printf("size:%ld\n",statbuf.st_size);
return 0;
}
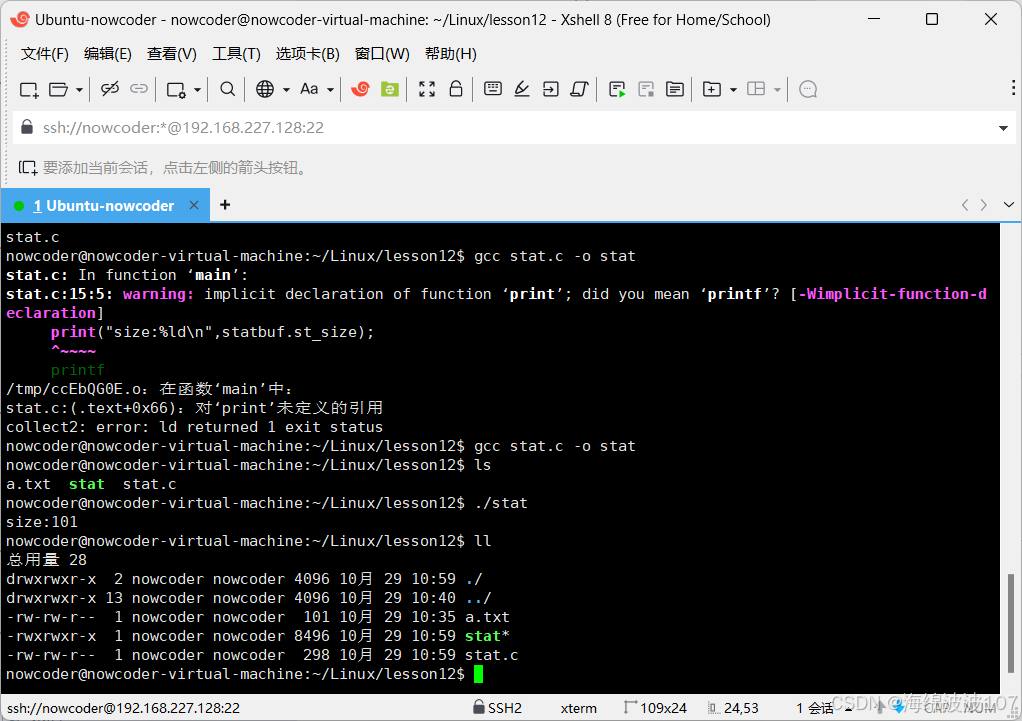
lstat用于获取指向该文件的软链接的信息