常见错误总结
错误1:对象const问题
#include <iostream>
class Man {
public:
void walk() {
std::cout << "I am walking." << std::endl;
}
};
int main() {
const Man man;
man.walk();
return 0;
}
原因是Man man是const对象 但是调用了非const的成员函数
解决办法1:去掉man前面的const
解决办法2:walk后面加上const
错误2:vector加入的成员是拷贝新成员
cpp
#include <iostream>
#include <vector>
using namespace std;
class Man {
public:
Man(){}
void play() {
count += 10;
cout << "Man is playing" << endl;
}
int getCount() const {
return count;
}
private:
int count = 0;
};
int main() {
vector<Man> mans;
Man man;
man.play();
mans.push_back(man);
man.play();
cout << "Total count: " << man.getCount() << endl;
cout << "vector Total count: " << mans[0].getCount() << endl;
return 0;
}
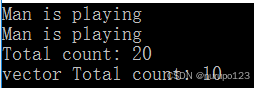
错误3:const引用问题
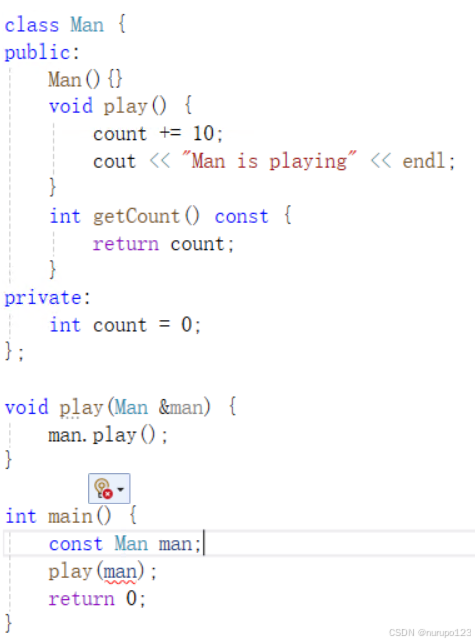
原因:非const引用,不能对const变量进行引用
注意:const引用,可以对非const变量进行引用
错误4:static错误
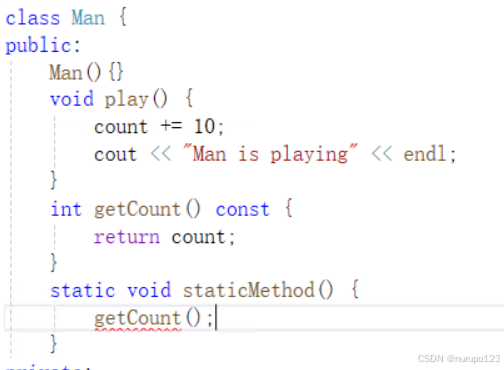
static方法不能访问实例方法和实例数据成员
Linux学习
1..需要研究 Linux的那些方面?
1)基本命令操作(<50 条命令)
2)Linux 系统编程-服务器开发
2.应该选择 Linux 的哪些发行版本?
Ubuntu
Centos (Redhat 的社区版)
3.使用虚拟机方式还是硬盘安装?
VMware workstation (vmware)
项目练习一
创建一个类,用来表示"玩具 "
文具,有以下数据 :
名称,价格,产地 。
在使用中,需要获取它的名称,价格,产地。
注意:根据自己当前的优惠情况,有一个对外的价格
Toy.h
cpp
#pragma once
#include <string>
class Toy {
public:
Toy();
Toy(std::string name, double price, std::string manufacturerLocation);
~Toy();
std::string getName() const;
double getPrice() const;
std::string getManufacturerLocation() const;
void setDisCount(double discount);
private:
std::string name;
double price;
std::string manufacturerLocation;
double discount = 1.0;
};
Toy.cpp
cpp
#include "toy.h"
Toy::Toy() {
}
Toy::~Toy() {
}
Toy::Toy(std::string name, double price, std::string maufacturerLocation) {
this->name = name;
this->price = price;
this->manufacturerLocation = maufacturerLocation;
}
std::string Toy::getName() const {
return this->name;
}
double Toy::getPrice() const {
return this->price * this->discount;
}
std::string Toy::getManufacturerLocation() const {
return this->manufacturerLocation;
}
void Toy::setDisCount(double disCount) {
this->discount = disCount;
}
main.cpp
cpp
#include <iostream>
#include "toy.h"
using namespace std;
int main() {
Toy t("car", 1002.123, "中国");
cout << t.getName() << t.getPrice() << t.getManufacturerLocation() << endl;
t.setDisCount(0.5);
cout << t.getPrice();
return 0;
}
项目练习二
定义一个或多个类,来描述以下需求:
定义一个类,来表示某模拟养成游戏中人物:
每个人物,有昵称,年龄,性别,配偶,朋友
支持的活动有:结婚,离婚,交友,断交,询问昵称,询问性别,询问年龄,简介等
Human.h
cpp
#pragma once
#include <string>
#include <iostream>
#include <vector>
enum Gender {
Male,
Female
};
class Human {
public:
int getAge() const;
std::string Description() const;
Gender getGender() const;
std::string getName() const;
Human* getMarried() const;
std::vector<Human*> getFriend() const;
void marry(Human& partner);
void divorce();
void addFriend(Human& friend_);
void removeFriend(Human& friend_);
Human();
Human(std::string, int, Gender);
~Human();
private:
std::string name;
int age;
Gender gender;
Human* lover = nullptr;
std::vector<Human*> friends;
};
Human.cpp
cpp
#include "Human.h"
#include <sstream>
Human::Human(){}
Human::Human(std::string name , int age , Gender gender) : age(age), name(name), gender(gender) {}
Human::~Human(){}
int Human::getAge() const{
return age;
}
std::string Human::Description() const {
std::stringstream ss;
ss << "Age: " << age;
ss << " Name: " << name;
ss << " Gender: " << (gender == Male)? "男" : "女";
return ss.str();
}
Gender Human::getGender() const{
return gender;
}
std::string Human::getName() const{
return name;
}
Human* Human::getMarried() const {
return lover;
}
std::vector<Human*> Human::getFriend() const {
return friends;
}
void Human::marry(Human& partner) {
if (gender == partner.gender) return;
this->lover = &partner;
partner.lover = this;
}
void Human::divorce() {
if (this->lover!= nullptr) {
this->lover->lover = nullptr;
this->lover = nullptr;
}
}
void Human::addFriend(Human& friend_) {
friends.push_back(&friend_);
}
void Human::removeFriend(Human& friend_) {
for (auto it = friends.begin(); it != friends.end(); ++it) {
if (*it == &friend_) {
friends.erase(it);
}
else {
it++;
}
}
}
main.cpp
cpp
#include <iostream>
#include "Human.h"
using namespace std;
int main() {
Human man1("John", 25, Male);
Human women1("Mary", 30, Female);
Human man2("Tom", 20, Male);
Human women2("Lily", 25, Female);
man1.marry(women1);
Human* who = man1.getMarried();
if (who == NULL) {
cout << "Man1 is not married" << endl;
}
else {
cout << "Man1 is married to " << who->getName() << endl;
}
man1.divorce();
who = man1.getMarried();
if (who == NULL) {
cout << "Man1 is not married" << endl;
}
else {
cout << "Man1 is married to " << who->getName() << endl;
}
man1.addFriend(man2);
man1.addFriend(women2);
/*cout << "Woman1 is married to " << who->getMarried()->Description() << endl;
*/return 0;
}