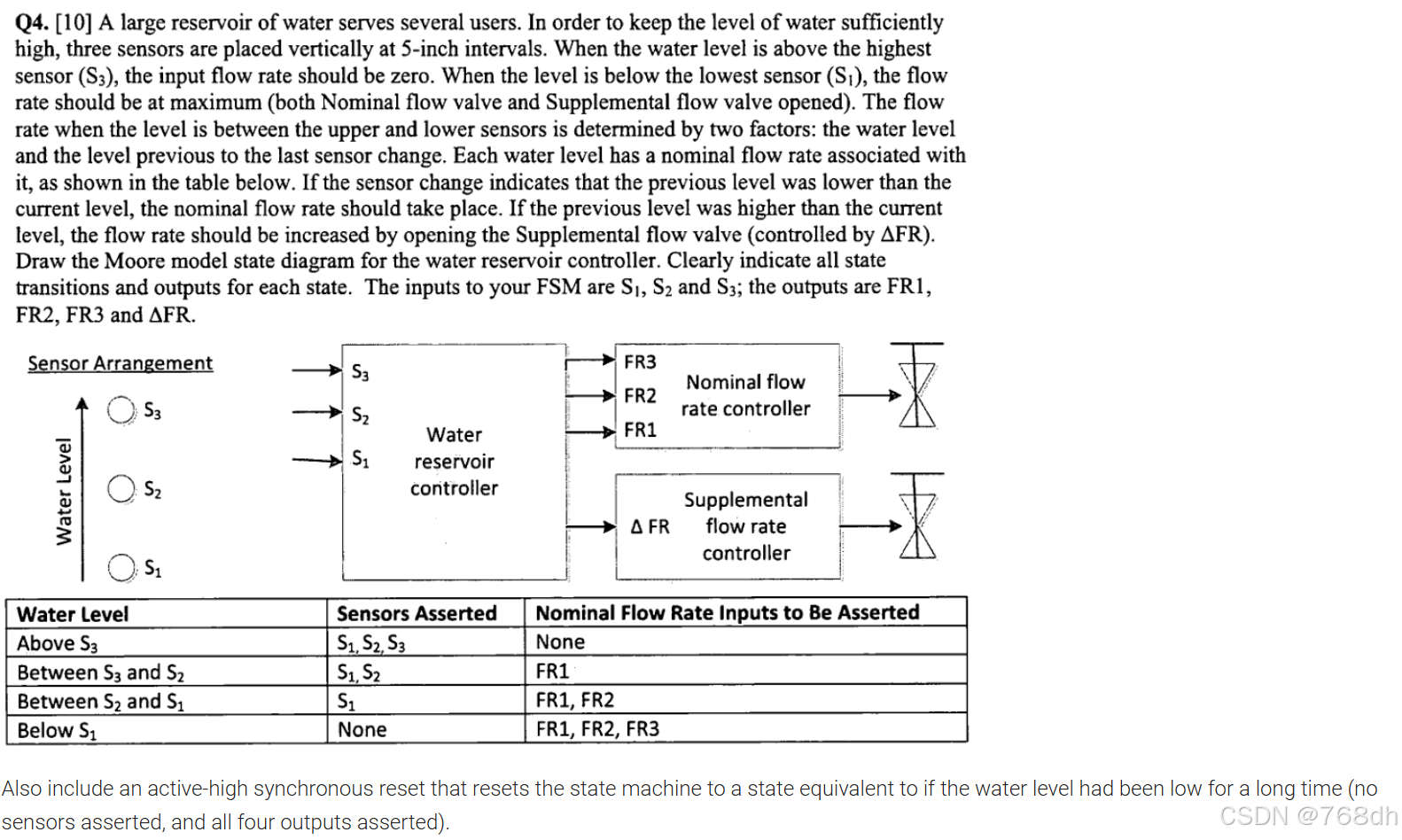
module top_module (
input clk,
input reset,
input [3:1] s,
output reg fr3,
output reg fr2,
output reg fr1,
output reg dfr
);
// Give state names and assignments. I'm lazy, so I like to use decimal numbers.
// It doesn't really matter what assignment is used, as long as they're unique.
// We have 6 states here.
parameter A2=0, B1=1, B2=2, C1=3, C2=4, D1=5;
reg [2:0] state, next; // Make sure these are big enough to hold the state encodings.
// Edge-triggered always block (DFFs) for state flip-flops. Synchronous reset.
always @(posedge clk) begin
if (reset) state <= A2;
else state <= next;
end
// Combinational always block for state transition logic. Given the current state and inputs,
// what should be next state be?
// Combinational always block: Use blocking assignments.
always@(*) begin
case (state)
A2: next = s[1] ? B1 : A2;
B1: next = s[2] ? C1 : (s[1] ? B1 : A2);
B2: next = s[2] ? C1 : (s[1] ? B2 : A2);
C1: next = s[3] ? D1 : (s[2] ? C1 : B2);
C2: next = s[3] ? D1 : (s[2] ? C2 : B2);
D1: next = s[3] ? D1 : C2;
default: next = 'x;
endcase
end
// Combinational output logic. In this problem, a procedural block (combinational always block)
// is more convenient. Be careful not to create a latch.
always@(*) begin
case (state)
A2: {fr3, fr2, fr1, dfr} = 4'b1111;
B1: {fr3, fr2, fr1, dfr} = 4'b0110;
B2: {fr3, fr2, fr1, dfr} = 4'b0111;
C1: {fr3, fr2, fr1, dfr} = 4'b0010;
C2: {fr3, fr2, fr1, dfr} = 4'b0011;
D1: {fr3, fr2, fr1, dfr} = 4'b0000;
default: {fr3, fr2, fr1, dfr} = 'x;
endcase
end
endmodule
错误代码 在研究ing
module top_module (
input clk,
input reset,
input [3:1] s,
output reg fr3,
output reg fr2,
output reg fr1,
output reg dfr
);
parameter A = 0, B=1,C=2,D=3;
reg[2:0] state, next;
reg[1:0] previous;
//注意只有这里的always是用的clk,其他的都是星号。
//这里就是状态转换,注意B1,B2,C1,C2的区别
always@(*)begin
case(state)
A:next <= s[1]?B:A;
B:next <= s[2]? C: (s[1]?previous:A);
C:next <= s[3]? D:(s[2]?previous:A);
D:next <= s[3]? D:C;
default:next <= A;
endcase
end
always @(posedge clk) begin
if (reset)
state <= A;
else begin
state <= next;
previous <= state;
end
end
//这里是输出结果,根据不同的状态开不同的阀门和赋值不同的dfr
always@(*)begin
case(state)
A: {fr3,fr2,fr1,dfr} = 4'b1111;
B: {fr3,fr2,fr1,dfr} = 4'b0110;
C: {fr3,fr2,fr1,dfr} = 4'b0010;
D: {fr3,fr2,fr1,dfr} = 4'b0000;
default: {fr3, fr2, fr1, dfr} = 'x;
endcase
end
endmodule
另一种
module top_module (
input clk,
input reset,
input [3:1] s,
output fr3,
output fr2,
output fr1,
output dfr
);
reg [1:0] state , nstate;
reg [2:0] outfr;
parameter
t1 = 2'b00,
t2 = 2'b01,
t3 = 2'b10,
t4 = 2'b11;
assign {fr3,fr2,fr1} = outfr;
always@(posedge clk)begin
if(reset)
state <= t1;
else
state <= nstate;
end
always@(*)begin
nstate = t1;
case(s)
3'b000:nstate = t1;
3'b001:nstate = t2;
3'b011:nstate = t3;
3'b111:nstate = t4;
default:nstate = t1;
endcase
end
always@(posedge clk)begin
if(reset)
outfr <= 3'b111;
else begin
case(nstate)
t1:outfr <= 3'b111;
t2:outfr <= 3'b011;
t3:outfr <= 3'b001;
t4:outfr <= 3'b000;
endcase
end
end
always@(posedge clk)begin
if(reset)
dfr <= 1;
else begin
if(nstate < state)
dfr <= 1;
else if(nstate > state)
dfr <= 0;
else
dfr <= dfr;
end
end
endmodule