EasyExcel好处是什么?
EasyExcel 是一个基于 Apache POI 的 Java Excel 处理库,主要用于高效地读写 Excel 文件。它的主要好处包括:
-
高性能:EasyExcel 在内存管理和读取速度上进行了优化,适合处理大规模 Excel 文件。
-
简洁易用:提供了简单的 API,减少了复杂的配置和代码量,开发者可以快速上手。
-
低内存消耗:支持流式读取和写入,可以逐行处理数据,避免将整个文件加载到内存中,适合大数据量的处理。
-
支持多种格式 :可以处理
.xls
和.xlsx
格式的文件,满足不同的需求。 -
注解支持:通过注解定义 Excel 的结构,使得代码更加清晰,易于维护。
-
良好的文档和社区支持:提供了丰富的示例和文档,社区活跃,可以得到及时的支持和反馈。
-
支持复杂数据类型:能够处理自定义对象的读写,支持多种数据类型的转换。
这些特点使得 EasyExcel 在 Java 开发中成为处理 Excel 文件的优选库。
常用注解
1.@ExcelProperty:用于指定 Excel 列的名称和顺序。
@ExcelProperty("姓名")
private String name;
2.@ExcelIgnore:用于忽略某个字段,不在 Excel 中读写。
@ExcelIgnore
private String password;
3.@ExcelList:用于处理 Excel 中的集合类型。
@ExcelList("成绩")
private List<Integer> scores;
4.@ExcelConverter:用于自定义字段的转换逻辑。
@ExcelProperty("状态")
@ExcelConverter(converter = StatusConverter.class)
private Status status;
5.@DateTimeFormat:用于指定日期时间的格式。
@ExcelProperty("出生日期")
@DateTimeFormat("yyyy-MM-dd")
private LocalDate birthDate;
6.@ExcelIgnoreUnannotated:在读取时忽略未标注的字段。
@ExcelIgnoreUnannotated
7.@ExcelPropertyOrder:用于指定属性的顺序。
@ExcelPropertyOrder({"name", "age", "email"})
依赖
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>3.1.0</version> <!-- 请根据需要选择版本 -->
</dependency>
创建实体类
@Data
public class PmsItemExcel {
@ExcelProperty("物品名称")
private String itemName;
@ExcelProperty("物品别名")
private String itemAlias;
@ExcelProperty("物品编号")
private String itemCarId;
@ExcelProperty("物品价格")
private BigDecimal itemPrice;
@ExcelProperty("物品状态")
private Long itemStatus;
@ExcelProperty("物品类型名称")
private String typeName;
@ExcelProperty("物品数量")
private Integer itemCount;
@ExcelProperty("物品图片")
private String itemPic;
/**
* 转换为物品对象
* @return PmsItem
*/
public PmsItem toItem() {
PmsItem pmsItem = new PmsItem();
BeanUtils.copyProperties(this, pmsItem);
return pmsItem;
}
}
导出
控制层
@Operation(summary = "导出物品Excel")
@RequestMapping(value = "/export", method = RequestMethod.GET)
@ResponseBody
public void export(HttpServletResponse response) {
response.setContentType("application/vnd.openxmlformats-officedocument.spreadsheetml.sheet");
response.setHeader("Content-Disposition", "attachment; filename=pms_item_export.xlsx");
// 获取数据
List<PmsItemExcel> list = pmsItemService.list();
try {
EasyExcel.write(response.getOutputStream(), PmsItemExcel.class)
.sheet("物品数据")
.doWrite(list);
} catch (IOException e) {
throw new RuntimeException("导出失败", e);
}
}
测试1
使用测试工具直接发送,出现一堆乱码没关系,这不是报错,这是编码问题
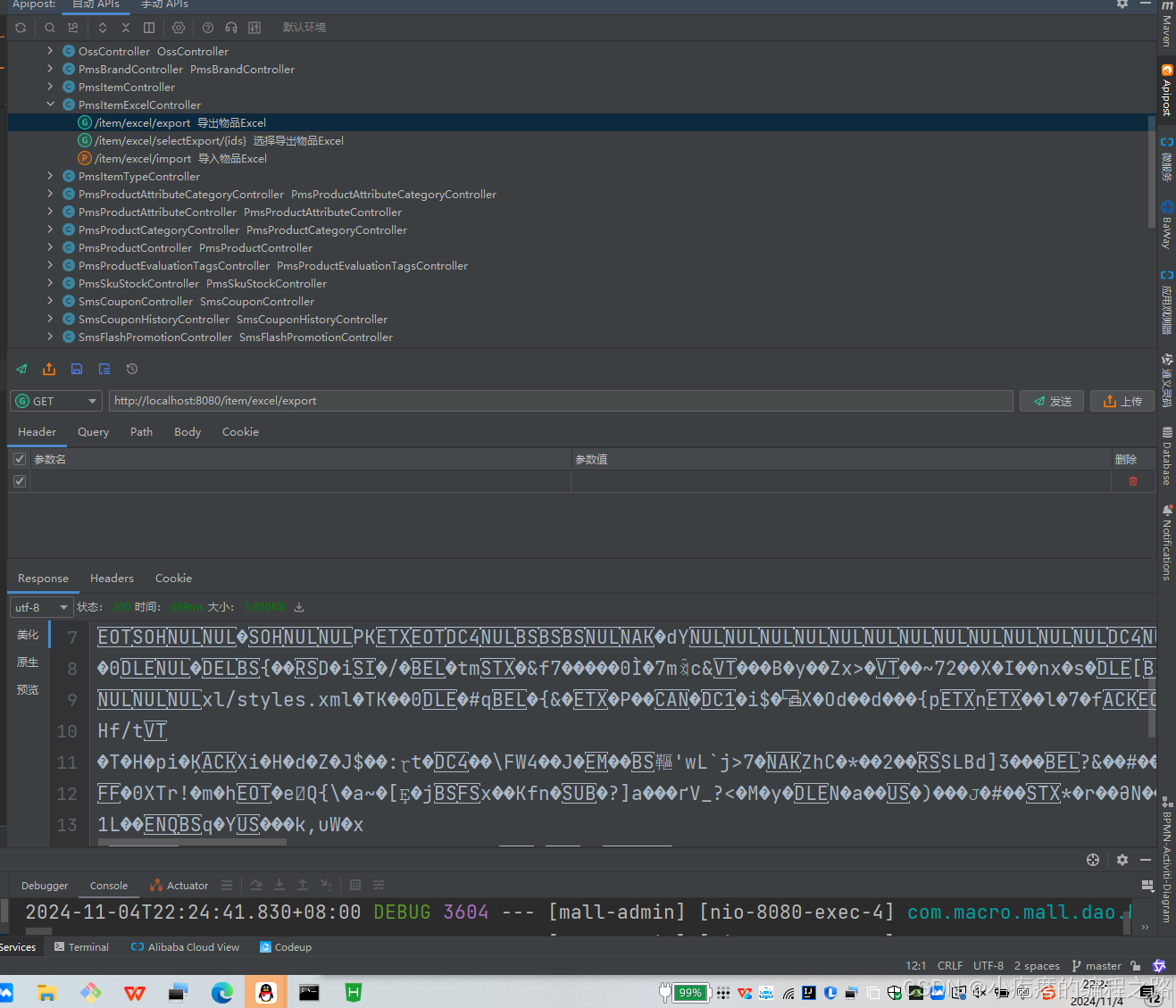
点击下载就可以导出了
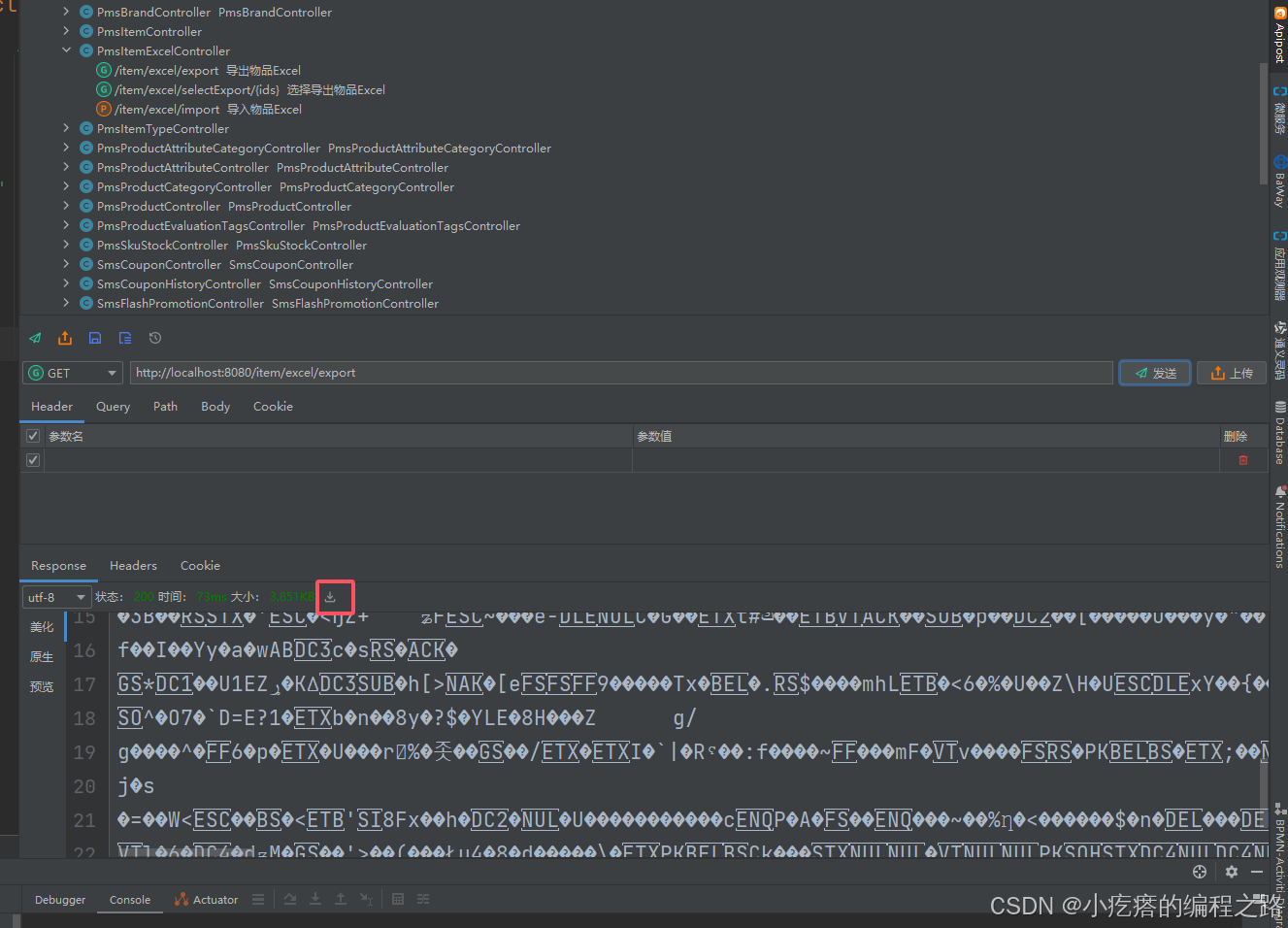
测试2
我这边写的前台是vue2写的
<div>
<el-button type="primary" @click="exportFile">导出 Excel</el-button>
</div>
async exportFile() {
try {
const response = await fetch('http://localhost:8201/mall-admin/item/excel/export', {
method: 'GET',
});
if (response.ok) {
const blob = await response.blob();
const url = window.URL.createObjectURL(blob);
const link = document.createElement('a');
link.href = url;
link.setAttribute('download', '物品管理数据.xlsx'); // 设置下载文件名
document.body.appendChild(link);
link.click();
link.remove();
} else {
alert('导出失败,请重试');
}
} catch (error) {
console.error('导出错误:', error);
alert('导出时发生错误');
}
},
导入
控制层
@Autowired
private PmsItemExcelListener pimItemExcelListener;
@Operation(summary = "导入物品Excel")
@RequestMapping(value = "/import", method = RequestMethod.POST)
@ResponseBody
public void importExcel(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
throw new RuntimeException("上传的文件为空");
}
try {
EasyExcel.read(file.getInputStream(), PmsItemExcel.class,pimItemExcelListener).sheet().doRead();
} catch (Exception e) {
throw new RuntimeException("导入失败", e);
}
}
监听器
/**
* 物品Excel导入监听器
*/
@Component
@Slf4j
public class PmsItemExcelListener extends AnalysisEventListener<PmsItemExcel> {
@Autowired
private PmsItemService pmsItemService;
@Override
public void invoke(PmsItemExcel item, AnalysisContext context) {
// 处理每一行数据,比如保存到数据库
// 在这里可以调用服务保存数据到数据库
pmsItemService.create(item.toItem().toReq());
}
@Override
public void doAfterAllAnalysed(AnalysisContext context) {
// 完成后的处理
//打印出成功的数据
log.info("成功读取数据:{}", context.readRowHolder().getRowIndex());
}
}
测试1
表示成功了
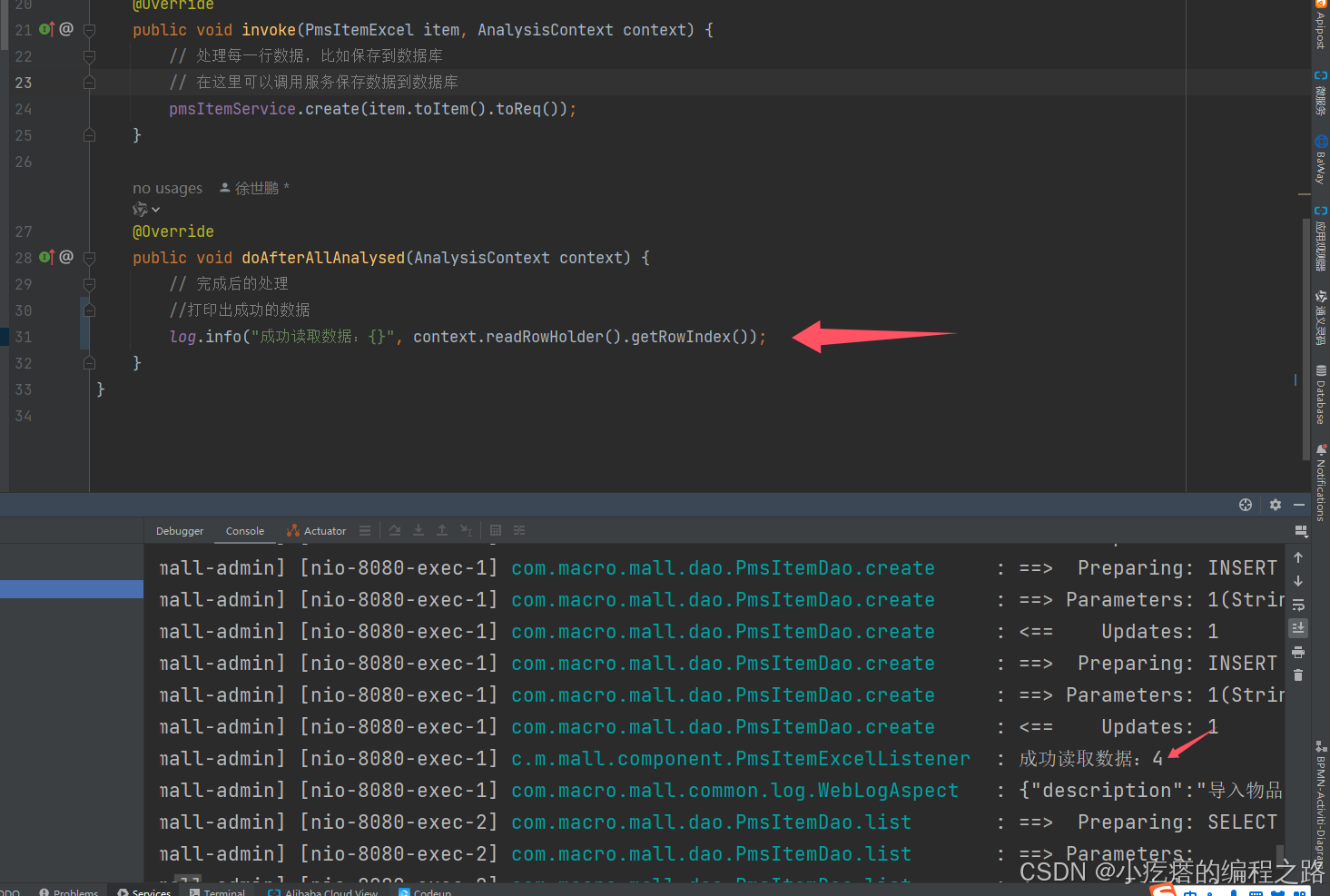
测试2
写的vue测试导入
<div>
<input type="file" @change="onFileChange" />
<el-button type="primary" @click="uploadFile">上传 Excel</el-button>
</div>
data() {
return {
selectedFile: null,
}
},
onFileChange(event) {
this.selectedFile = event.target.files[0];
},
async uploadFile() {
if (!this.selectedFile) {
alert("请先选择一个文件");
return;
}
const formData = new FormData();
formData.append("file", this.selectedFile);
try {
const response = await fetch("http://localhost:8201/mall-admin/item/excel/import", {
method: "POST",
body: formData,
});
if (response.ok) {
this.getList();
alert("文件上传成功");
} else {
alert("文件上传失败");
}
} catch (error) {
console.error("上传错误:", error);
alert("文件上传出错");
}
},
测试成功
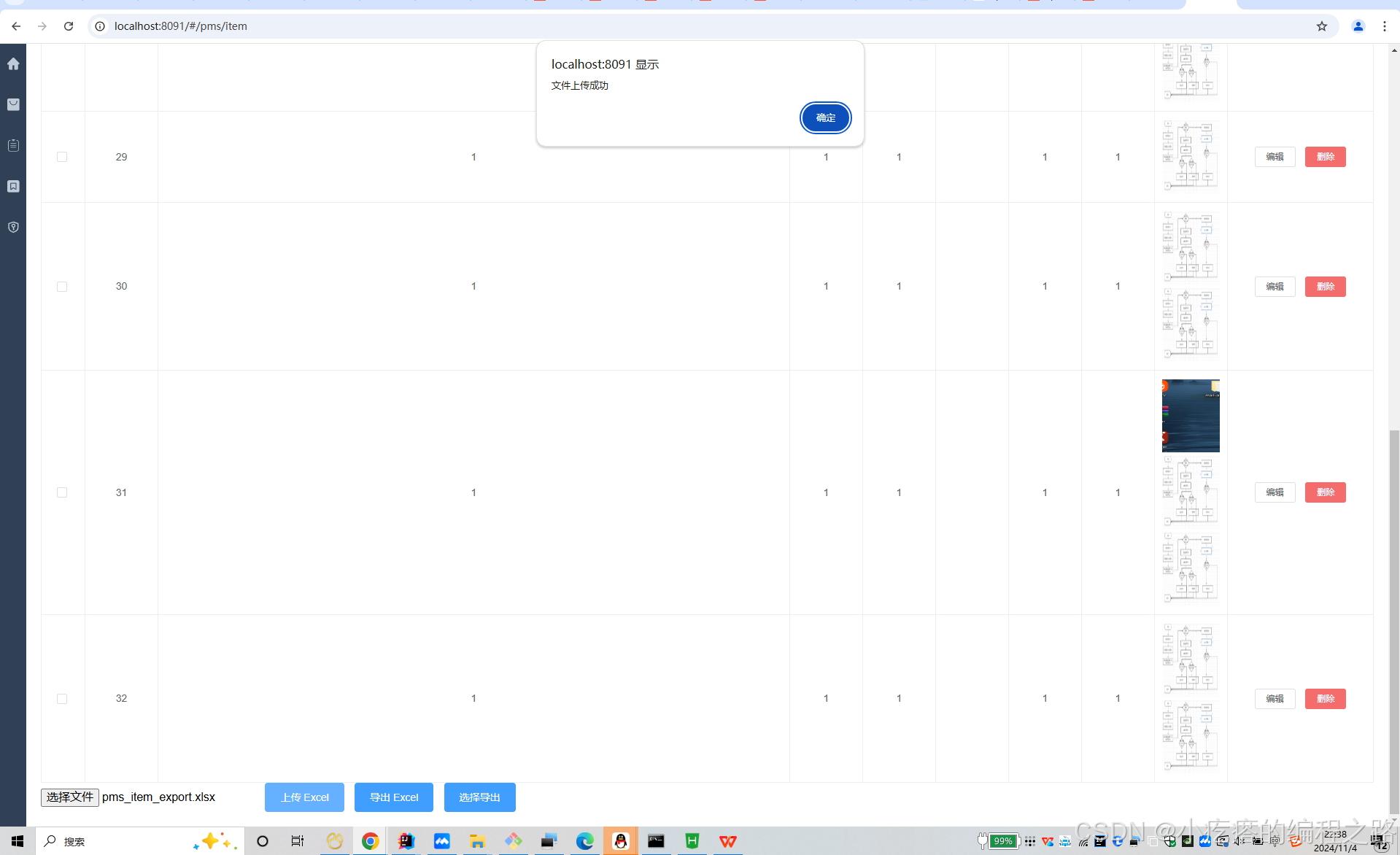