单值转换器
1.创建项目后下载两个NuGet程序包
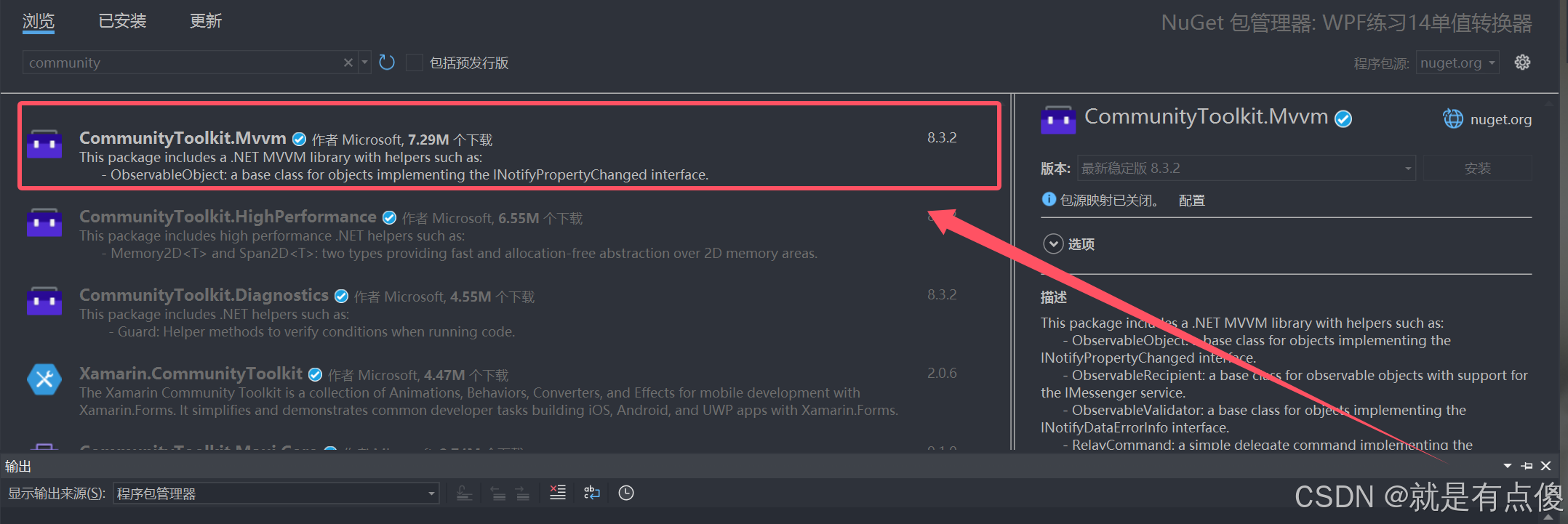
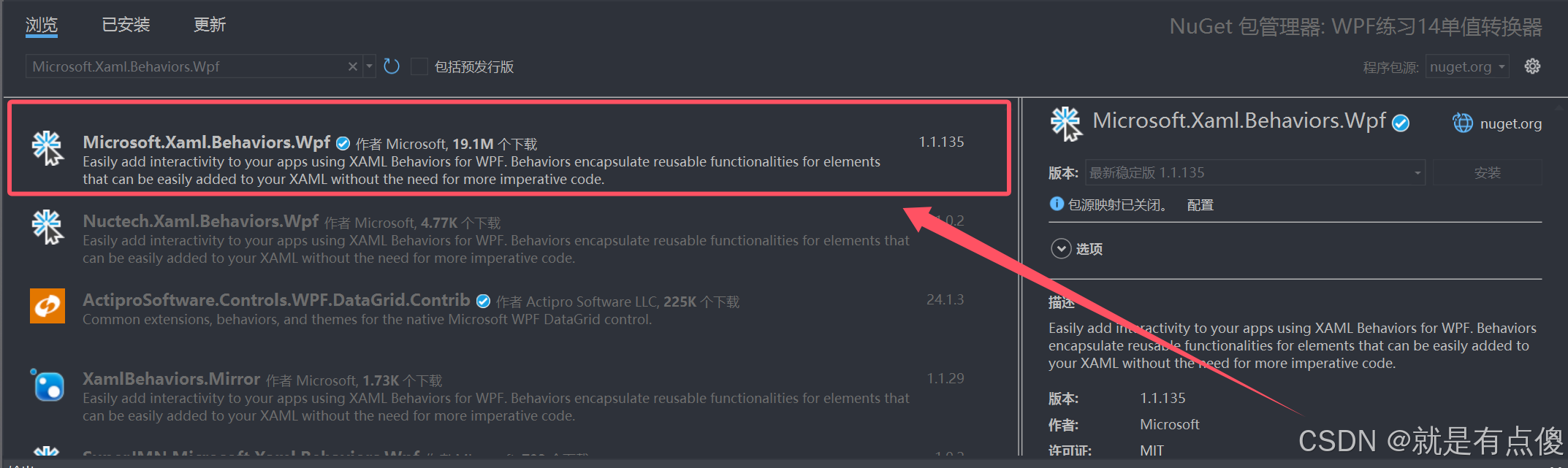
2.先定义一个转换器实现IValueConverter接口
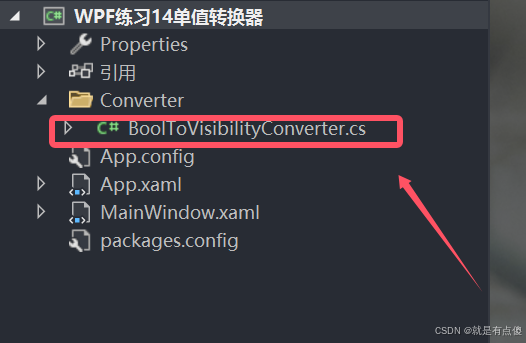
cs
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Data;
namespace WPF练习14单值转换器.Converter
{
public class BoolToVisibilityConverter : IValueConverter
{
// Convert负责把源数据类型转换成目标数据类型
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
bool oldType = (bool)value; // 源数据类型
Visibility newType = oldType ? Visibility.Visible : Visibility.Collapsed; // 目标类型
return newType;
}
// ConvertBack负责目标数据类型转换成源数据类型
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
}
3.在ViewModel文件夹中创建MainWindowViewModel
cs
using CommunityToolkit.Mvvm.ComponentModel;
using CommunityToolkit.Mvvm.Input;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WPF练习14单值转换器.ViewModel
{
public class MainWindowViewModel : ObservableObject
{
private bool isShow=true;
public bool IsShow
{
get => isShow;
set => SetProperty(ref isShow, value);
}
public RelayCommand UpdateIsShowCommand
{
get
{
return new RelayCommand(() =>
{
IsShow = !IsShow;
});
}
}
}
}
4.把转换器当成一个资源导入到XAML文件中 并且将MainWindowViewModel导入DataContext上下文中
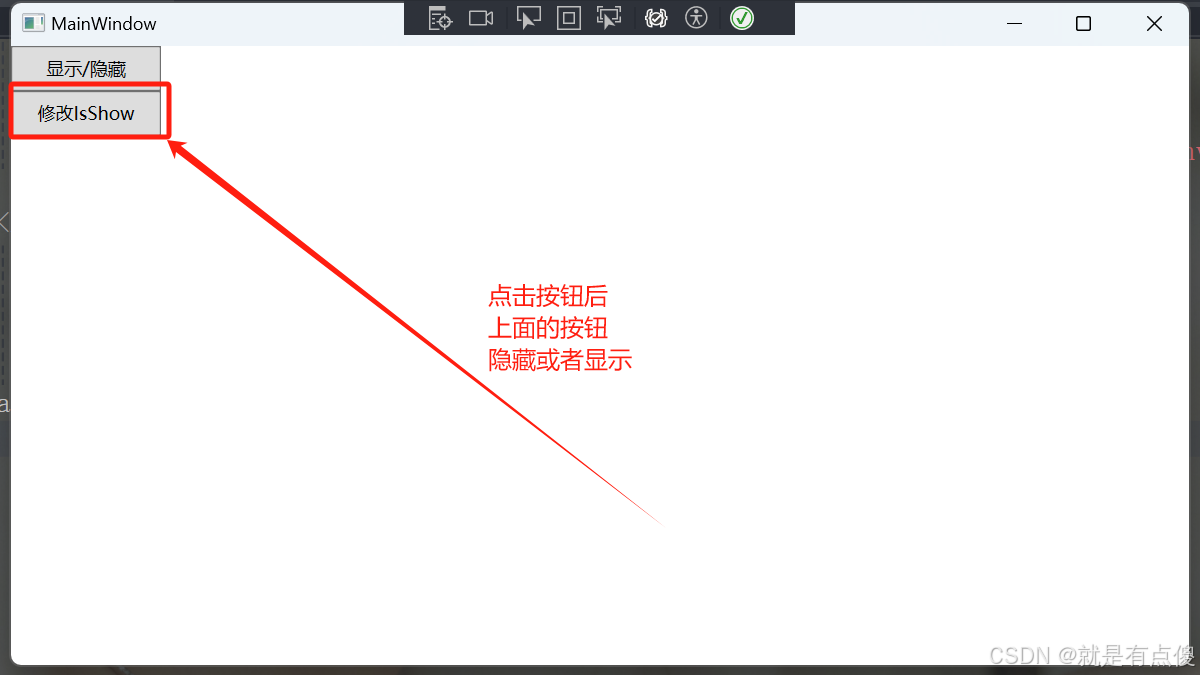
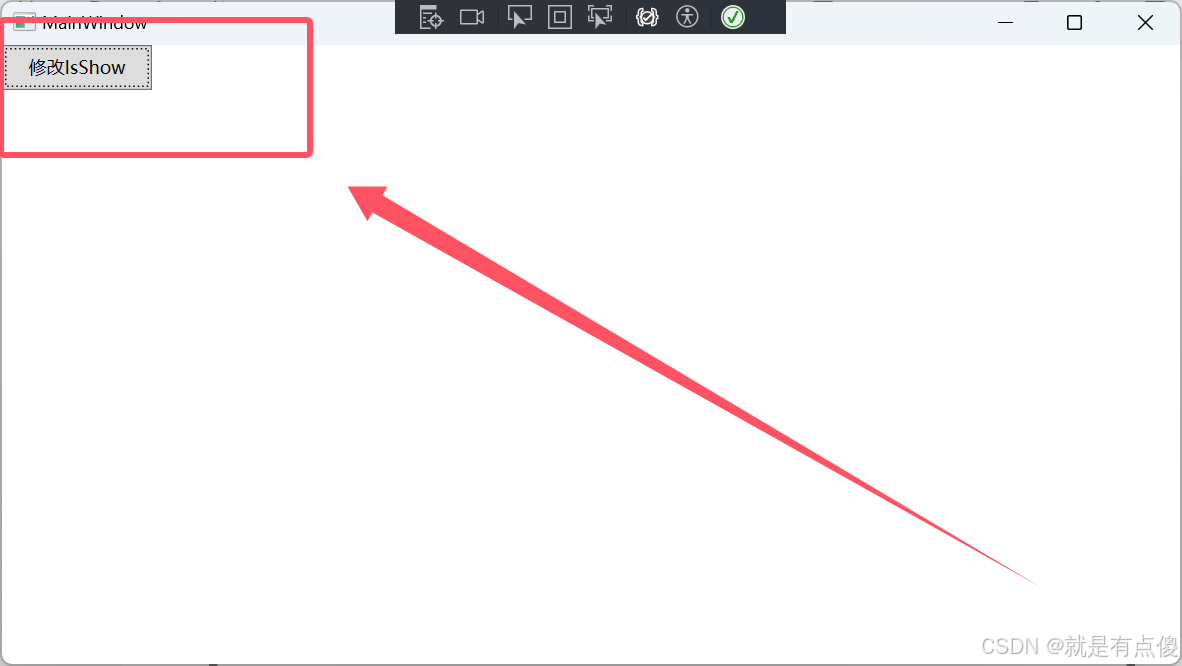
XML
<Window x:Class="WPF练习14单值转换器.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPF练习14单值转换器"
xmlns:c="clr-namespace:WPF练习14单值转换器.Converter"
xmlns:vm="clr-namespace:WPF练习14单值转换器.ViewModel"
mc:Ignorable="d"
Title="MainWindow"
Height="450"
Width="800">
<Window.DataContext>
<vm:MainWindowViewModel />
</Window.DataContext>
<Window.Resources>
<c:BoolToVisibilityConverter x:Key="boolToVisibilityConverter" />
</Window.Resources>
<Grid>
<WrapPanel Orientation="Vertical">
<Button Width="100"
Height="30"
HorizontalAlignment="Left"
Content="显示/隐藏"
Visibility="{Binding IsShow, Converter={StaticResource boolToVisibilityConverter}}" />
<Button Width="100"
Height="30"
HorizontalAlignment="Left"
Command="{Binding UpdateIsShowCommand}"
Content="修改IsShow" />
</WrapPanel>
</Grid>
</Window>
多值转换器(结构同上)
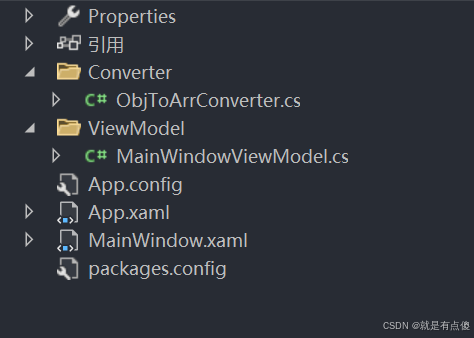
ObjToArrConverter如下:
cs
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Data;
namespace WPF练习14单值转换器.Converter
{
public class ObjToArrConverter : IMultiValueConverter
{
public object Convert(object[] values, Type targetType, object parameter, CultureInfo culture)
{
return values.ToArray();
}
public object[] ConvertBack(object value, Type[] targetTypes, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
}
MainWindowViewModel如下:
cs
using CommunityToolkit.Mvvm.ComponentModel;
using CommunityToolkit.Mvvm.Input;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
namespace WPF练习14单值转换器.ViewModel
{
public class MainWindowViewModel : ObservableObject
{
public RelayCommand<object[]> Command3
{
get
{
return new RelayCommand<object[]>((arr) =>
{
MessageBox.Show(arr[0].ToString());
MessageBox.Show(arr[1].ToString());
MessageBox.Show(arr[2].ToString());
});
}
}
}
}
MainWindow.XAML如下:
cs
<Window x:Class="WPF练习14单值转换器.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPF练习14单值转换器"
xmlns:c="clr-namespace:WPF练习14单值转换器.Converter"
xmlns:vm="clr-namespace:WPF练习14单值转换器.ViewModel"
xmlns:i="http://schemas.microsoft.com/xaml/behaviors"
mc:Ignorable="d"
Title="MainWindow"
Height="450"
Width="800">
<Window.DataContext>
<vm:MainWindowViewModel />
</Window.DataContext>
<Window.Resources>
<c:ObjToArrConverter x:Key="objToArrConverter" />
</Window.Resources>
<Grid>
<WrapPanel Orientation="Vertical">
<TextBox x:Name="Id" />
<TextBox x:Name="Name" />
<TextBox x:Name="Status" />
<TextBlock Width="150"
Height="30"
HorizontalAlignment="Left"
Text="传递多个参数">
<i:Interaction.Triggers>
<i:EventTrigger EventName="MouseLeftButtonUp">
<i:InvokeCommandAction Command="{Binding Command3}">
<i:InvokeCommandAction.CommandParameter>
<MultiBinding Converter="{StaticResource objToArrConverter}">
<Binding ElementName="Id"
Path="Text" />
<Binding ElementName="Name"
Path="Text" />
<Binding ElementName="Status"
Path="Text" />
</MultiBinding>
</i:InvokeCommandAction.CommandParameter>
</i:InvokeCommandAction>
</i:EventTrigger>
</i:Interaction.Triggers>
</TextBlock>
</WrapPanel>
</Grid>
</Window>
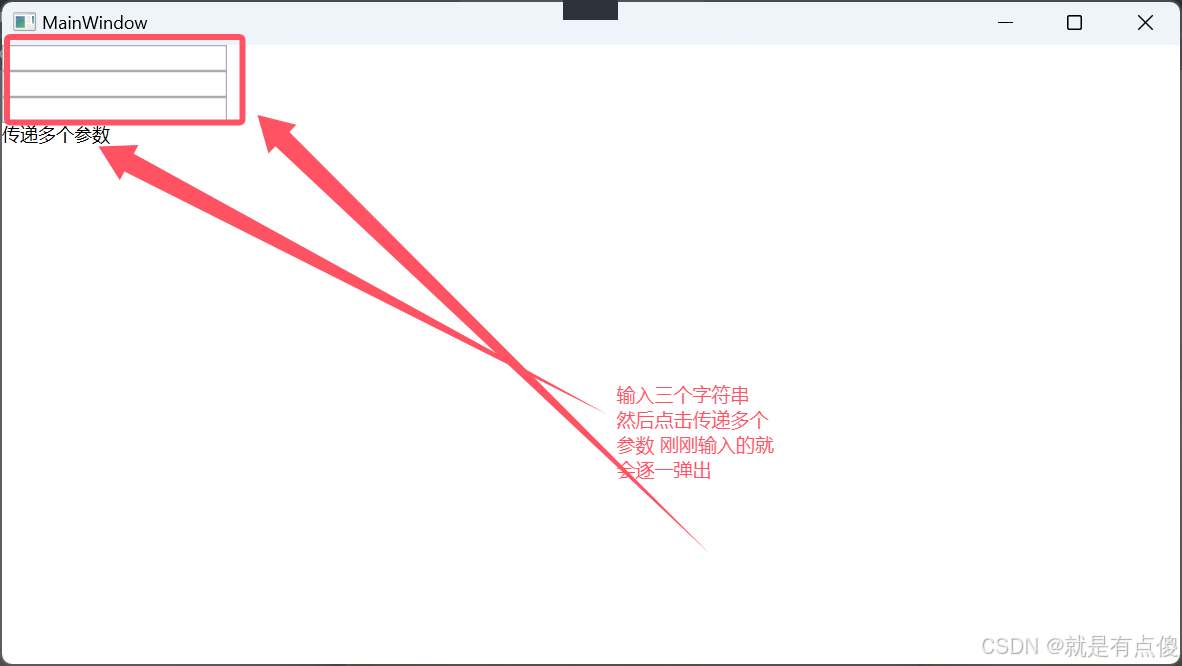
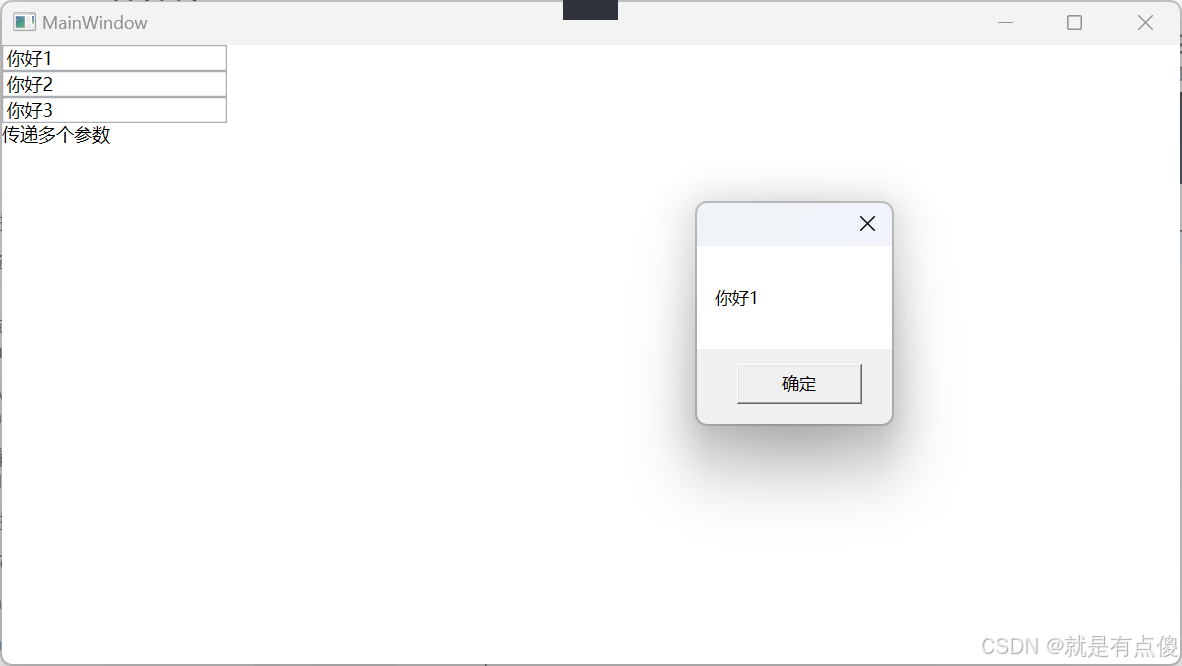
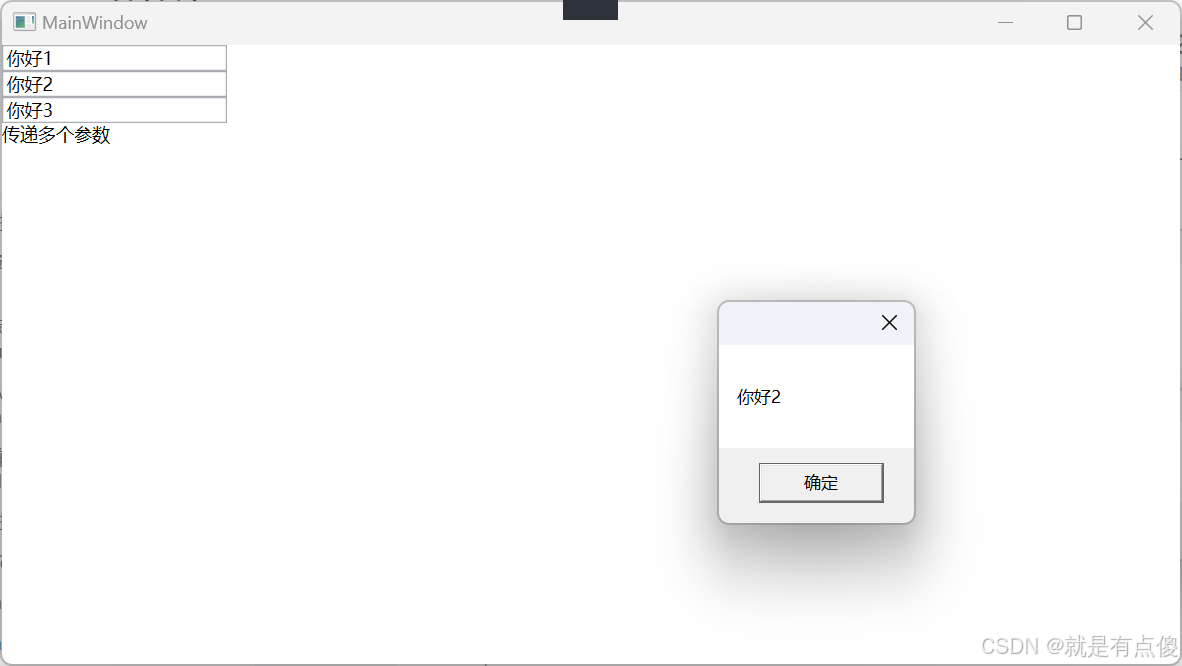
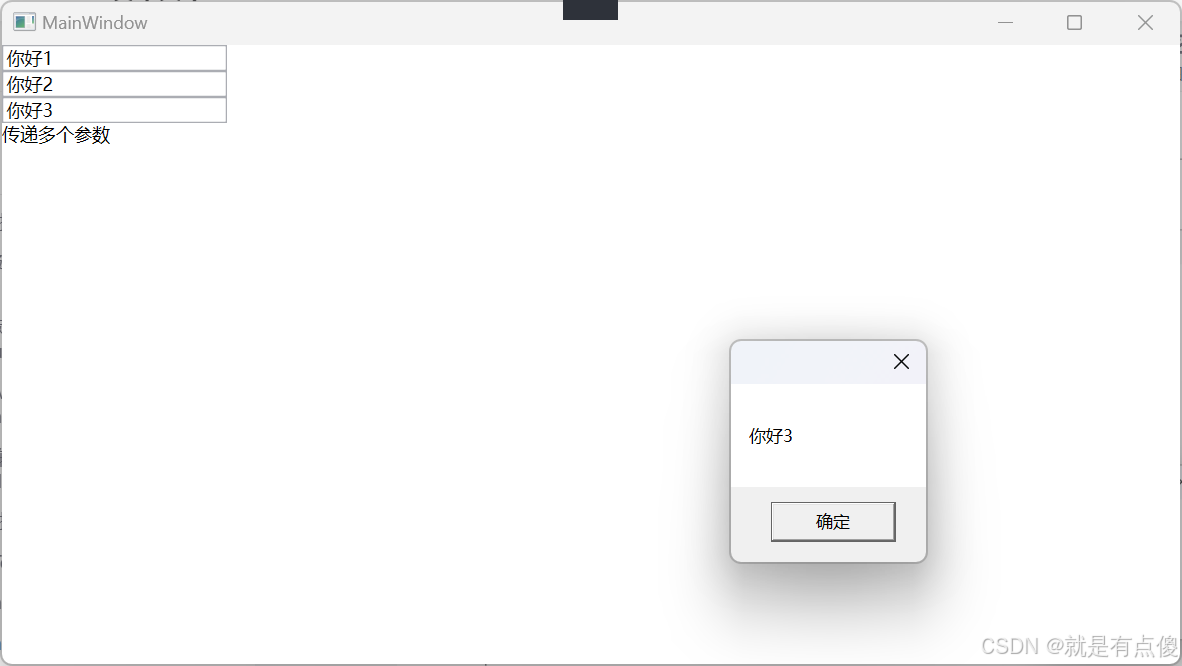
按钮传递多个参数和这个类似
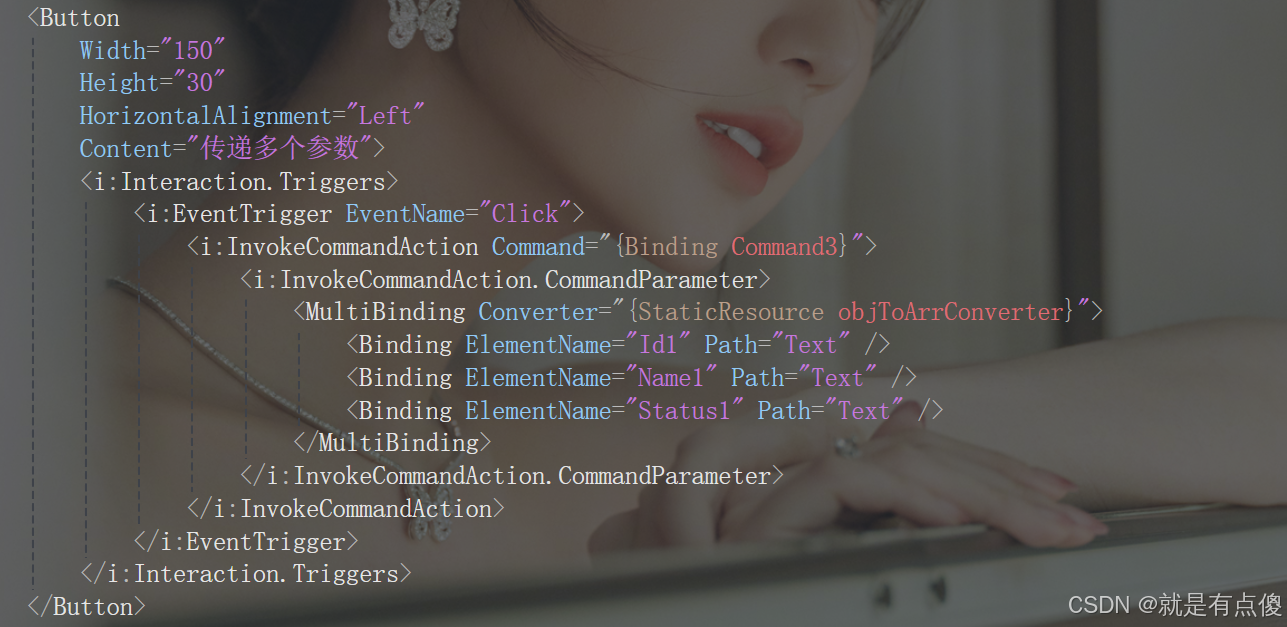