一、装饰器模式
1、意图
动态增加功能,相比于继承更加灵活
2、类图
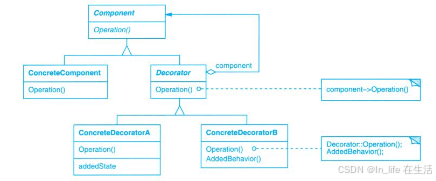
Component(VisualComponent):定义一个对象接口,可以给这些对象动态地添加职责。ConcreteComponent(TextView):定义一个对象,可以给这个对象添加一些职责。
Decorator:维持一个指向Component对象的指针,并定义一个与Component接口一致的接口。ConcreteDecorator(BorderDecorator、ScrollDecorator):向组件添加职责。
3、实现
java
public interface Component {
void draw();
}
java
public class ConcreteComponent implements Component{
@Override
public void draw() {
System.out.println("展示一个窗口");
}
}
抽象类实现接口
java
public abstract class Decorator implements Component{
public Component component;
Decorator(Component component){
this.component=component;
}
@Override
public void draw() {
component.draw();
}
}
java
public class ConcreteDecorator1 extends Decorator{
ConcreteDecorator1(Component component) {
super(component);
}
@Override
public void draw() {
System.out.println("给窗口加边框");
super.draw();
}
}
java
public class ConcreteDecorator2 extends Decorator{
ConcreteDecorator2(Component component) {
super(component);
}
@Override
public void draw() {
System.out.println("给窗口加滚动条");
super.draw();
}
}
java
public class test {
public static void main(String[] args) {
Component concreteComponent=new ConcreteComponent();
Decorator decorator1=new ConcreteDecorator1(concreteComponent);
decorator1.draw();
System.out.println("------------------------------");
Decorator decorator2=new ConcreteDecorator2(concreteComponent);
decorator2.draw();
}
}
4、效果
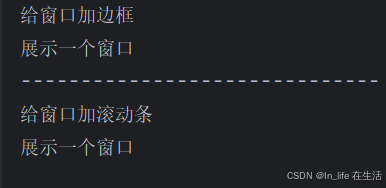
二、命令模式
1、意图
例子:
可以将开关理解成一个请求的发送者,用户通过它来发送一个"开灯"请求,而电灯是"开灯"请求的最终接收者和处理者。
使用不同的电线可以连接不同的请求接收者,只需更换一根电线。相同的发送者(开关)即可对应不同的接收者(电器)。
含义:
将请求发送者和接收者解耦
发送者与接收者之间没有直接引用关系,发送请求的对象只需要知道如何发送请求,而不必知道如何完成请求
2、类图
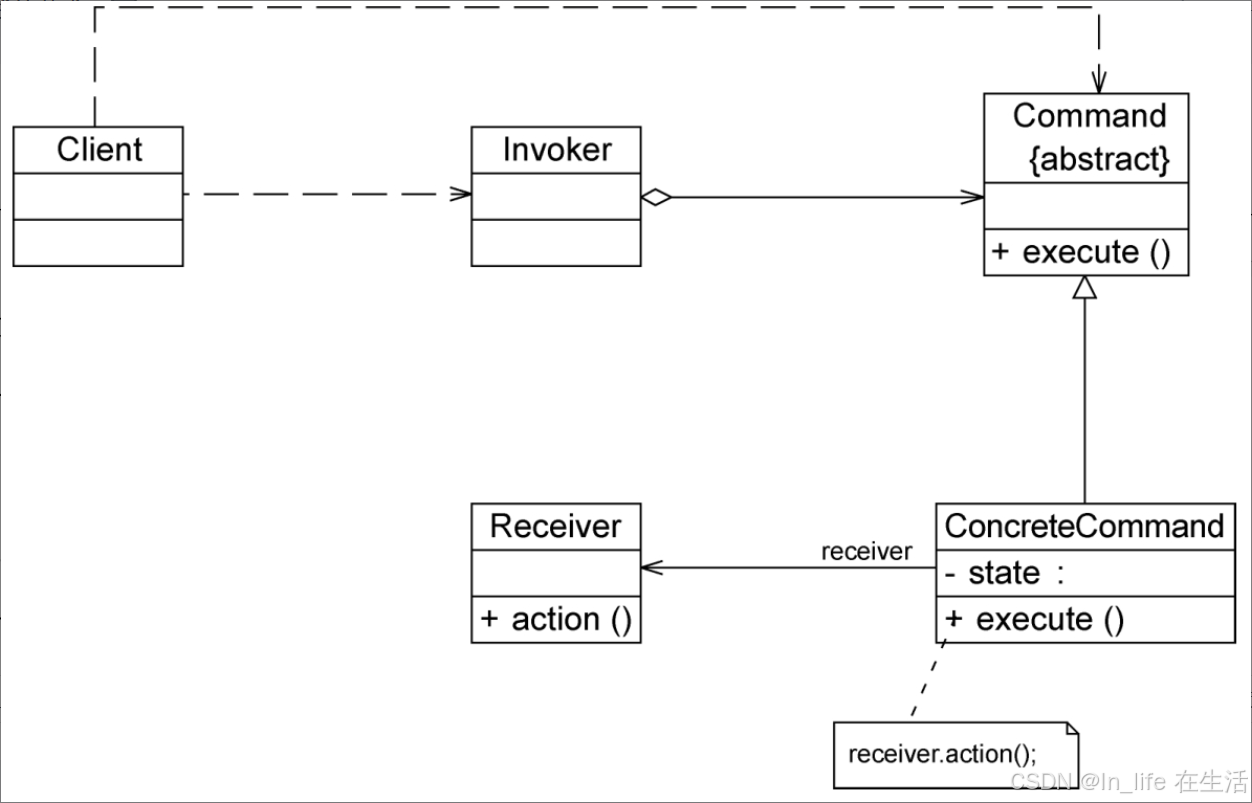
Invoker(调用者):调用者即请求发送者,一个调用者并不需要在设计时确定其接收者
Receiver(接收者):接收者执行与请求相关的操作
Command(抽象命令类):抽象命令类一般是一个抽象类或接口
ConcreteCommand(具体命令类):具体命令类是抽象命令类的子类,实现了在抽象命令类中声明的方法。它对应具体的接收者对象,将接收者对象的动作绑定其中
命令模式的关键在于引入了抽象命令类。请求发送者针对抽象命令类编程,只有实现了抽象命令类的具体命令才与请求接收者相关联
3、实现
一个请求发送者对应多个请求接收者
java
/**
* 开关
*/
public class Invoker {
Command command;
public Invoker(Command command) {
this.command = command;
}
void call(){
command.execute();
}
}
java
public interface Command {
void execute();
}
java
public class ConcreteCommand1 implements Command{
Receiver1 receiver1;
public ConcreteCommand1(Receiver1 receiver1) {
this.receiver1 = receiver1;
}
@Override
public void execute() {
receiver1.action();
}
}
java
public class ConcreteCommand2 implements Command{
Receiver2 receiver2;
public ConcreteCommand2(Receiver2 receiver2) {
this.receiver2 = receiver2;
}
@Override
public void execute() {
receiver2.action();
}
}
java
public class Receiver1 {
void action(){
System.out.println("打开电灯");
}
}
java
public class Receiver2 {
void action(){
System.out.println("打开电风扇");
}
}
java
public class test {
public static void main(String[] args) {
Receiver1 receiver1=new Receiver1();
Command command1=new ConcreteCommand1(receiver1);
Invoker invoker1=new Invoker(command1);
invoker1.call();
System.out.println("---------------------------");
Receiver2 receiver2=new Receiver2();
Command command2=new ConcreteCommand2(receiver2);
Invoker invoker2=new Invoker(command2);
invoker2.call();
}
}
4、效果
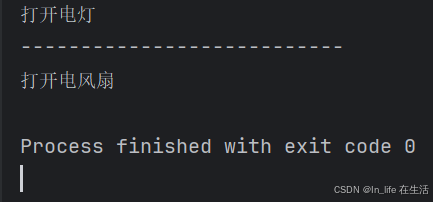