7.5 本章练习
- 创建一个Spring Boot项目,使用Thymeleaf页面模板引擎和JdbcTemplate数据持久化框架实现完整的学生信息管理模块。
答案:
-
application.properties 配置数据库
spring.datasource.primary.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.primary.jdbc-url=jdbc:mysql://127.0.0.1:3306/test?useSSL=false&serverTimezone=UTC
spring.datasource.primary.username=root
spring.datasource.primary.password=123456
spring.datasource.secondary.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.secondary.jdbc-url=jdbc:mysql://127.0.0.1:3306/dp_database?useSSL=false&serverTimezone=UTC
spring.datasource.secondary.username=root
spring.datasource.secondary.password=123456 -
- JdbcTemplate 配置
package com.example.demo.config;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.jdbc.core.JdbcTemplate;import javax.sql.DataSource;
@Configuration
public class DataSourceConfig {@Primary @Bean(name = "primaryDataSource") @Qualifier("primaryDataSource") @ConfigurationProperties("spring.datasource.primary") public DataSource primaryDataSource() { return DataSourceBuilder.create().build(); } @Bean(name = "secondaryDataSource") @Qualifier("secondaryDataSource") @ConfigurationProperties("spring.datasource.secondary") public DataSource secondaryDataSource() { return DataSourceBuilder.create().build(); } @Bean(name = "primaryJdbcTemplate") public JdbcTemplate primaryJdbcTemplate(@Qualifier("primaryDataSource") DataSource dataSource) { return new JdbcTemplate(dataSource); } @Bean(name = "secondaryJdbcTemplate") public JdbcTemplate secondaryJdbcTemplate(@Qualifier("secondaryDataSource") DataSource dataSource) { return new JdbcTemplate(dataSource); }
}
-
学生实体类 Student.java
package com.example.demo.bean;
public class Student {
private Long id; private String name; private Integer sex; private Integer age; public Student() { } public Student(String name, int sex, int age) { this.name = name; this.sex = sex; this.age = age; } @Override public String toString() { return "Student{" + "id=" + id + ", name='" + name + '\'' + ", sex=" + sex + ", age=" + age + '}'; } public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getSex() { return sex; } public void setSex(int sex) { this.sex = sex; } public Integer getAge() { return age; } public void setAge(int age) { this.age = age; }
}
-
StudentController 控制器 创建 StudentController 类,处理学生管理的 HTTP 请求。
package com.example.demo.controller;
import com.example.demo.bean.Student;
import com.example.demo.service.StudentService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;@Controller
@RequestMapping("/students")
public class StudentController {@Autowired private StudentService studentService; @GetMapping("/") public String getAllStudents(Model model) { model.addAttribute("students", studentService.listAllStudent()); return "students/list"; } @GetMapping("/add") public String showAddForm(Model model) { model.addAttribute("student", new Student()); return "students/add"; } @PostMapping("/add") public String addStudent(@ModelAttribute Student student) { studentService.insertStudent(student); return "redirect:/students/"; } @GetMapping("/edit/{id}") public String showEditForm(@PathVariable Long id, Model model) { Student student = studentService.getStudentById(id); model.addAttribute("student", student); return "students/edit"; } @PostMapping("/edit/{id}") public String updateStudent(@PathVariable Long id, @ModelAttribute Student student) { student.setId(id); studentService.updateStudent(student); return "redirect:/students/"; } @GetMapping("/delete/{id}") public String deleteStudent(@PathVariable Long id) { studentService.deleteStudent(id); return "redirect:/students/"; }
}
-
StudentService 服务类 创建 StudentService 类,用于业务逻辑处理。
package com.example.demo.service;
import com.example.demo.bean.Student;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.jdbc.core.BeanPropertyRowMapper;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Service;import java.util.List;
@Service
public class StudentService {@Autowired @Qualifier("primaryJdbcTemplate") private JdbcTemplate jdbcTemplate; public List<Student> listAllStudent(){ String sql = "select * from student"; return jdbcTemplate.query(sql, new BeanPropertyRowMapper<Student>(Student.class)); } public Student getStudentById(Long id){ String sql = "select * from student where id=?"; return jdbcTemplate.queryForObject(sql, new Object[]{id}, new BeanPropertyRowMapper<>(Student.class)); } public void insertStudent(Student student){ String sql = "insert into student(id, name, age, sex) values(?,?,?,?)"; jdbcTemplate.update(sql,student.getId(),student.getName(),student.getAge(),student.getSex()); } public void updateStudent(Student student){ String sql = "update student set name=?,age=?,sex=? where id=?"; jdbcTemplate.update(sql,student.getName(),student.getAge(),student.getSex(),student.getId()); } public void deleteStudent(Long id){ String sql = "delete from student where id=?"; jdbcTemplate.update(sql,id); }
}
-
Thymeleaf 页面 创建页面模板来展示学生列表、添加、编辑学生等功能。
list.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>学生信息管理</title>
</head>
<body>
<h1>学生信息</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="student : ${students}">
<td th:text="${student.id}"></td>
<td th:text="${student.name}"></td>
<td th:text="${student.age}"></td>
<td th:text="${student.sex}"></td>
<td>
<a th:href="@{/students/edit/{id}(id=${student.id})}">编辑</a> |
<a th:href="@{/students/delete/{id}(id=${student.id})}">删除</a>
</td>
</tr>
</tbody>
</table>
<a href="/students/add">添加学生</a>
</body>
</html>
add.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>添加学生</title>
</head>
<body>
<h1>添加学生</h1>
<form action="#" th:action="@{/students/add}" th:object="${student}" method="post">
<label>姓名:</label>
<input type="text" th:field="*{name}"/><br/>
<label>年龄:</label>
<input type="number" th:field="*{age}"/><br/>
<label>性别:</label>
<input type="number" th:field="*{sex}"/><br/>
<button type="submit">提交</button>
</form>
<a href="/students/">返回</a>
</body>
</html>
edit.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>编辑学生</title>
</head>
<body>
<h1>编辑学生</h1>
<form action="#" th:action="@{/students/edit/{id}(id=${student.id})}" th:object="${student}" method="post">
<label>姓名:</label>
<input type="text" th:field="*{name}"/><br/>
<label>年龄:</label>
<input type="number" th:field="*{age}"/><br/>
<label>性别:</label>
<input type="number" th:field="*{sex}"/><br/>
<button type="submit">提交</button>
</form>
<a href="/students/">返回</a>
</body>
</html>
- 测试 启动 Spring Boot 应用,访问:
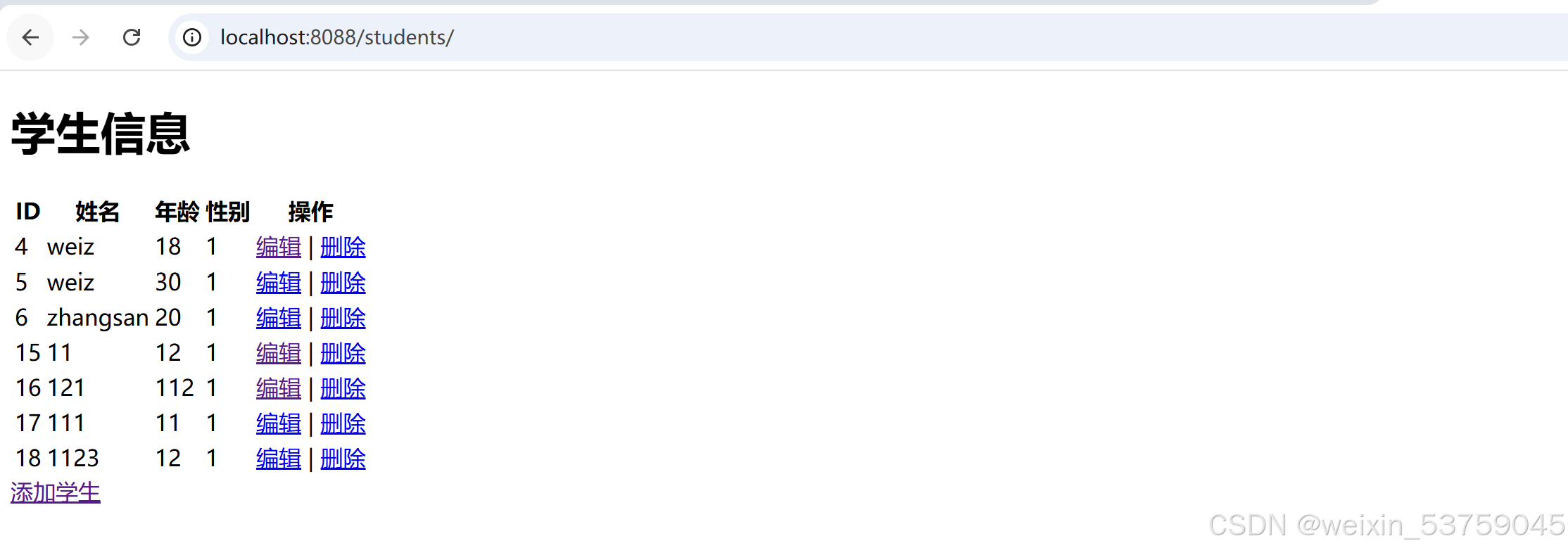
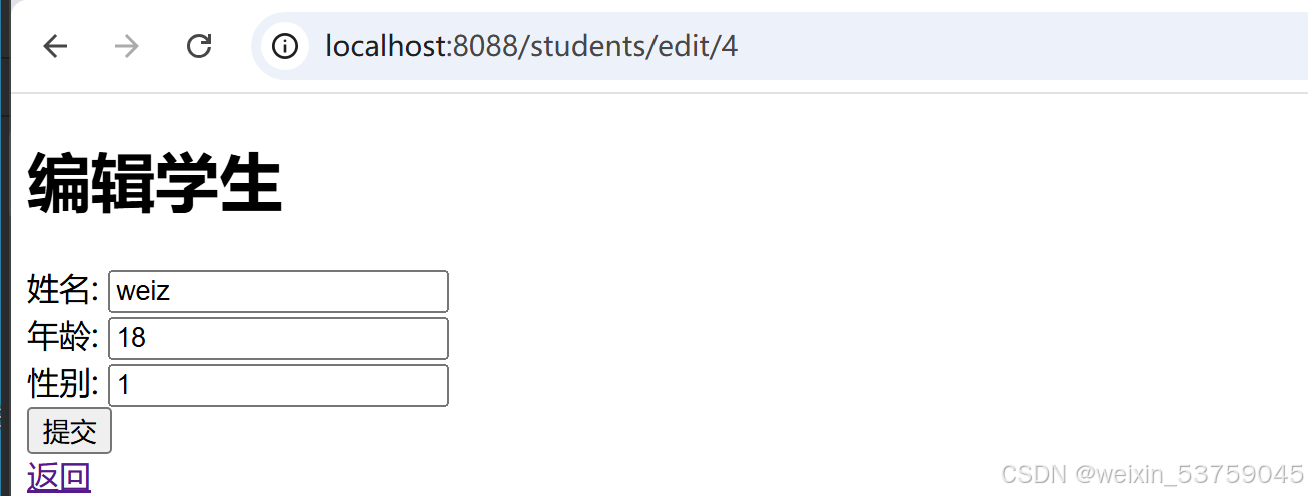