一、新建项目KafakDemo
<ItemGroup>
<PackageReference Include="Confluent.Kafka" Version="2.6.0" />
</ItemGroup>
二、Program.cs
cs
using Confluent.Kafka;
using System;
using System.Threading;
using System.Threading.Tasks;
namespace KafakDemo
{
internal class Program
{
static void Main(string[] args)
{
Task.Run(() =>
{
Publish();
});
Task.Run(() =>
{
Consumer();
});
Console.ReadKey();
}
static void Publish() {
var conf = new ProducerConfig { BootstrapServers = "192.168.31.135:9092" };
Action<DeliveryReport<Null, string>> handler = r =>
Console.WriteLine(!r.Error.IsError
? $"Delivered message to {r.TopicPartitionOffset}"
: $"Delivery Error: {r.Error.Reason}");
while (true)
{
using (var p = new ProducerBuilder<Null, string>(conf).Build())
{
for (int i = 0; i < 1; ++i)
{
p.Produce("my-topic", new Message<Null, string> { Value = i.ToString() }, handler);
}
p.Flush();
}
Thread.Sleep(TimeSpan.FromSeconds(2));
}
}
static void Consumer()
{
var conf = new ConsumerConfig
{
GroupId = "test-consumer-group",
BootstrapServers = "192.168.31.135:9092"
// Note: The AutoOffsetReset property determines the start offset in the event
// there are not yet any committed offsets for the consumer group for the
// topic/partitions of interest. By default, offsets are committed
// automatically, so in this example, consumption will only start from the
// earliest message in the topic 'my-topic' the first time you run the program.
//AutoOffsetReset = AutoOffsetReset.Earliest
};
using (var c = new ConsumerBuilder<Ignore, string>(conf).Build())
{
c.Subscribe("my-topic");
CancellationTokenSource cts = new CancellationTokenSource();
Console.CancelKeyPress += (_, e) => {
// Prevent the process from terminating.
e.Cancel = true;
cts.Cancel();
};
try
{
while (true)
{
try
{
var cr = c.Consume(cts.Token);
Console.WriteLine($"Consumed message '{cr.Message.Value}' at: '{cr.TopicPartitionOffset}'.");
}
catch (ConsumeException e)
{
Console.WriteLine($"Error occured: {e.Error.Reason}");
}
}
}
catch (OperationCanceledException)
{
// Ensure the consumer leaves the group cleanly and final offsets are committed.
c.Close();
}
}
}
}
}
运行效果:
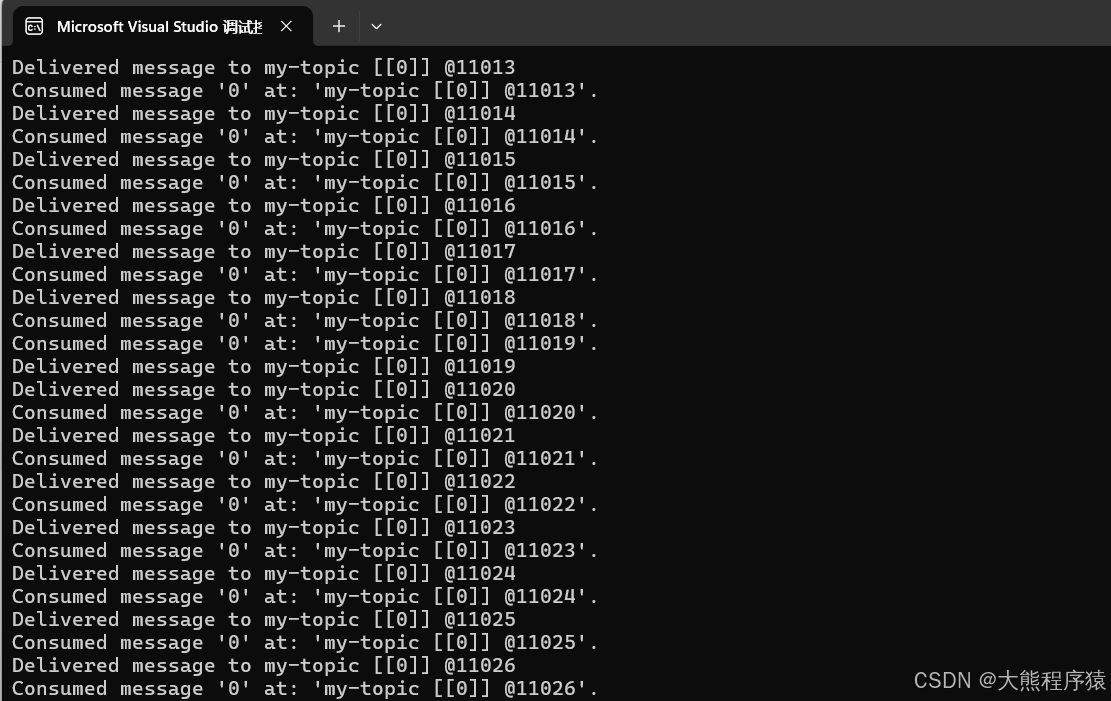
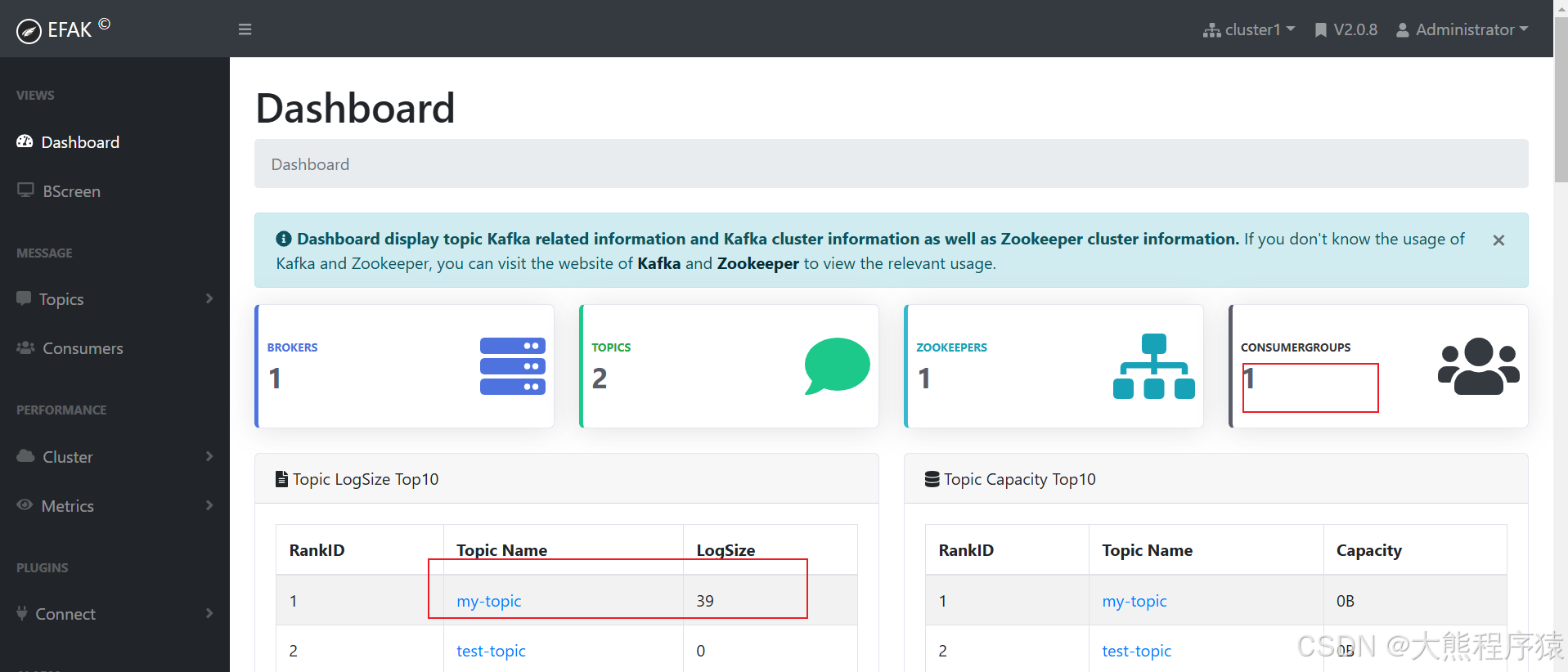