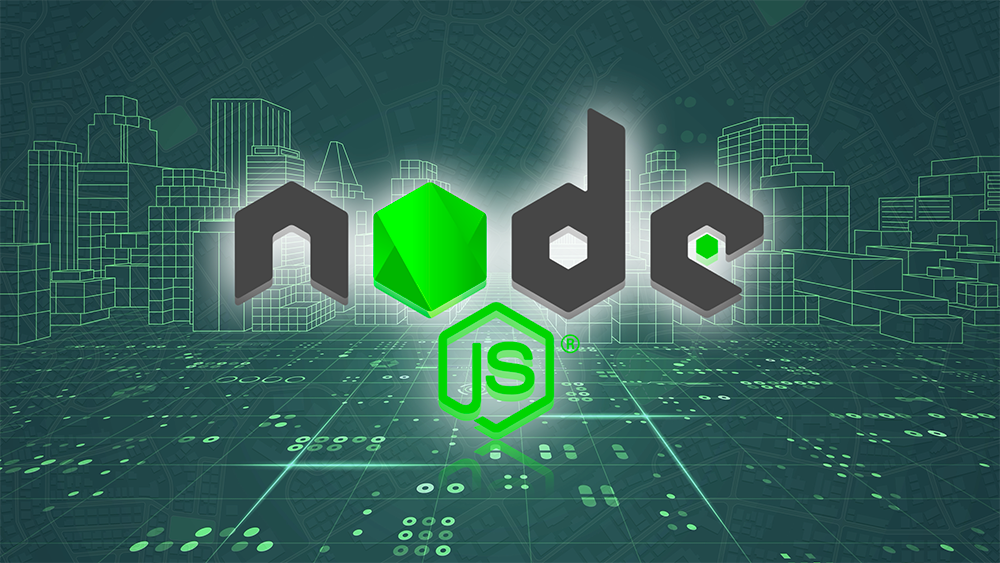
在快节奏的 web 开发世界中,管理操作的时间对于创建高效、用户友好的应用程序至关重要。Node.js 是一个功能强大的 JavaScript 运行时,它提供了几种通过延迟和超时来控制执行流的方法。本指南将引导你完成在 Node.js 中实现延迟和超时的要点,确保你的应用程序平稳高效地运行。
理解 Delays 和 Timeouts
Delays
用于将代码的执行暂停一段指定的时间。这对于模拟异步操作、管理 API 请求计时或在执行代码块之前添加暂停非常有用。
Timeouts
是在指定延迟后执行一段代码的一种方法。与简单的延迟不同,如果需要,可以清除超时,从而提供对何时执行某些操作的更多控制。
实现 Delays
Node.js 不像其他语言那样有内置的 sleep 函数,但是你可以使用 setTimeout() 函数或创建一个自定义的基于 promise 的延迟函数来实现延迟。
Using setTimeout() for Delays
setTimeout() 函数是实现延迟的最直接的方法。它接受两个参数:回调函数和以毫秒为单位的延迟。
javascript
console.log('Delay start');
setTimeout(() => {
console.log('Executed after 2 seconds');
}, 2000);
在本例中,消息 Executed after 2 seconds 将在 2 秒延迟后打印到控制台。
Creating a Promise-Based Delay Function
对于要使用 async/await 语法的场景,可以将 setTimeout() 封装在 promise 中。
javascript
function delay(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function run() {
console.log('Delay start');
await delay(2000);
console.log('Executed after 2 seconds');
}
run();
这种方法提供了一种更优雅的方式来处理延迟,特别是在异步函数中。
实现 Timeouts
Timeouts 通常用于限制操作的执行时间。在 Node.js 中,你可以使用 setTimeout() 来实现超时,如果操作在限制内完成,可以使用 clearartimeout() 来取消超时。
javascript
const timeoutId = setTimeout(() => {
console.log('This operation took too long and was terminated');
}, 3000);
someLongRunningOperation().then(() => {
clearTimeout(timeoutId);
console.log('Operation completed within the time limit');
});
在本例中,如果 someLongRunningOperation() 在 3 秒内完成,则清除超时,并且不打印终止消息。如果操作时间超过 3 秒,则打印操作结束的消息。
处理异步操作中的 Timeouts
当使用异步操作时,特别是在现代 Node.js 应用程序中,有效地集成超时以防止挂起操作或无响应行为是很重要的。
Using Promise.race() for Timeouts
javascript
function timeout(ms) {
return new Promise((_, reject) => setTimeout(() => reject(new Error('Operation timed out')), ms));
}
Promise.race([
someLongRunningOperation(),
timeout(3000)
]).then(() => {
console.log('Operation completed successfully');
}).catch(error => {
console.error(error.message);
});
这种方法确保如果操作没有在指定的时间限制内完成,则将其视为失败,并可以执行适当的错误处理。
我的开源项目
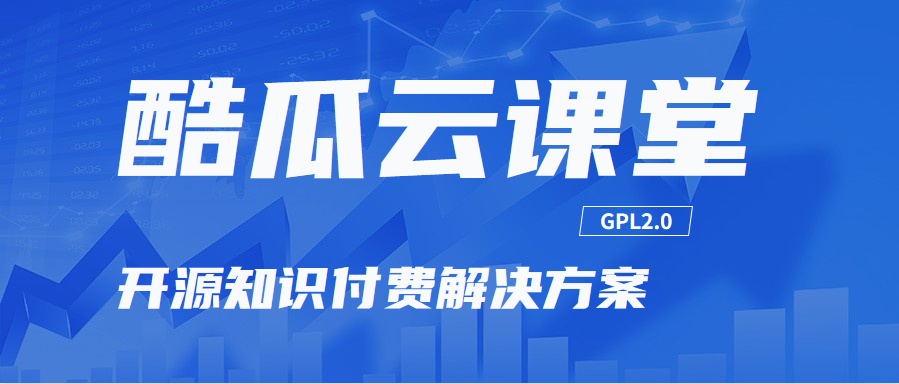