1. 异常的概念
C语言主要通过错误码的方式处理错误,错误码本质上就是对错误信息进行分类编号,拿到错误码以后还要去查询错误信息,比较麻烦。异常时抛出一个对象,这个对象可以涵盖更全面的信息。
异常处理机制允许程序中独立开发的部分能在运行时就出现的问题进行通信并做出相应的处理,异常使得我们能够将问题的检测与解决问题的过程分开,程序的一部分负责检测问题的出现,然后解决问题的任务传递给程序的另一部分,检测环节无需知道问题的处理模块的所有细节。
2. 异常的抛出和捕获
程序出现问题时,我们通过抛出(throw)一个对象来引发一个异常,该对象的类型以及当前的调用链决定了应该由哪个catch的处理代码来处理该异常。
被选中的处理代码是调用链中与该对象类型匹配且离抛出异常位置最近的那一个。根据抛出对象的类型和内容,程序的抛出异常部分告知异常处理部分到底发生了什么错误。当throw执行的时候throw后面的语句就不会执行了。程序就从throw的位置直接跳到与之匹配的catch模块(可能是在同一函数中,还可能是在调用链中的另一个函数中)
1.沿着调用链的函数可能提早退出。
2.一旦程序开始执行异常处理程序,沿着调用链创建的对象都将销毁。
抛出异常对象后,会生成一个异常对象的拷贝,因为抛出的异常对象可能是一个局部对象,所以会生成一个拷贝对象,这个拷贝对象会在catch子句后销毁。(类似函数的传值返回)
3. 栈展开
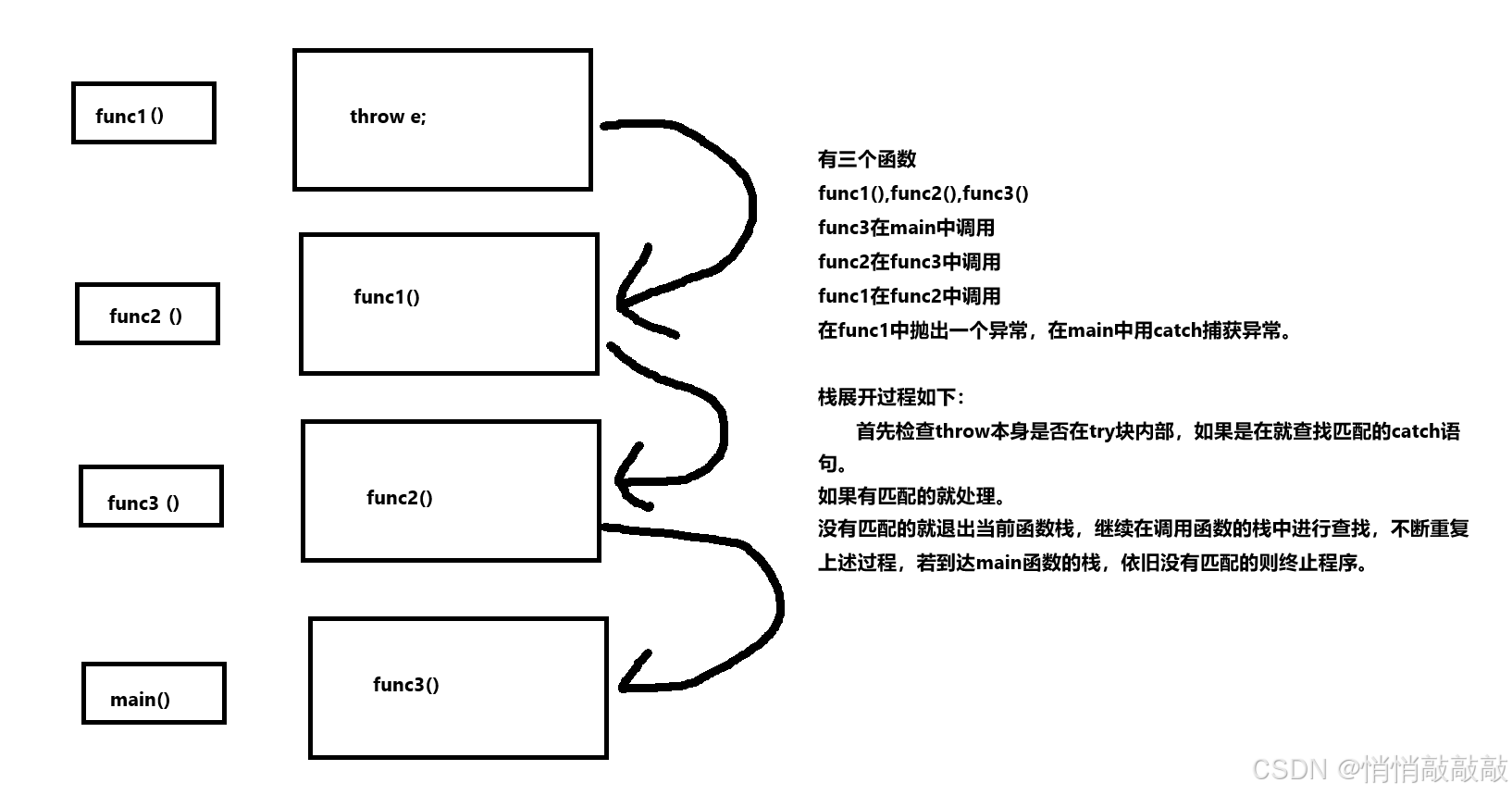
抛出异常后,程序暂停当前函数的执行,开始寻找与之匹配的catch子句,首先检查throw本身是否在try块内部,如果在则查找匹配的catch语句,如果有匹配的,则跳到catch的地方进行处理。
如果当前函数中没有try/catch子句,或者有try/catch子句但是类型不匹配,则退出当前函数,继续 在外层调用函数链中查找,上述查找的catch过程被称为栈展开。
如果到达main函数,依旧没有找到匹配的catch⼦句,程序会调用标准库的 terminate 函数终止程序。
如果找到匹配的catch子句处理后,catch子句代码会继续执行。
cpp
#include<iostream>
#include<string>
using namespace std;
double Divide(int a, int b)
{
try
{
if (b == 0)
{
string s("Divide 除数不能为0");
throw s;
}
else
{
return (double)a / (double)b;
}
}
catch(int errid)
{
cout << errid << endl;
}
return 0;
}
void Func1()
{
int a, b;
cin >> a >> b;
try
{
cout<<Divide(a, b) << endl;
}
catch (const char* errmsg)
{
cout << errmsg << endl;
}
int main()
{
while (1)
{
try
{
Func1();
}
catch (const string& errmsg)
{
cout << errmsg << endl;
}
}
return 0;
}
如上代码抛出异常后先查看Divide中的catch,不匹配,再沿调用链就会匹配到Func1里的catch还是不匹配,最后匹配到main里的catch语句。
4. 查找匹配的处理代码
如果抛出对象有多个catch类型匹配,就选择相距最近的那一个。
但是也有一些例外:
允许从非常量向常量进行类型转换,也就是权限缩小
允许数组转换成指向数组元素类型的指针,函数被转换成指向函数的指针
允许从派生类向基类类型的转换,实际中的继承体系基本都使用此方式设计
如果到main函数,异常仍旧没有被匹配就会终止程序,不是发生严重错误的情况下,我们是不期望程序终止的,所以一般main函数中最后都会使用catch(...),他可以捕获任意类型的异常,但是并不知道异常错误是什么。
通过以下代码来验证
cpp
#include<thread>
class Exception
{
public:
Exception(const string& errmsg,int id)
:_errmsg(errmsg)
,_id(id)
{
}
virtual string what() const
{
return _errmsg;
}
int getid() const
{
return _id;
}
protected:
string _errmsg;
int _id;
};
class SqlException :public Exception
{
public:
SqlException(const string& errmsg, int id, const string& sql)
:Exception(errmsg,id)//父类初始化
,_sql(sql)
{
}
virtual string what() const
{
string str = "SqlException";
str += _errmsg;
str += "->";
str += _sql;
return str;
}
private:
const string _sql;
};
class CacheException :public Exception
{
public:
CacheException(const string& errmsg, int id)
:Exception(errmsg,id)
{
}
virtual string what() const
{
string str = "CacheException:";
str += _errmsg;
return str;
}
};
class HttpException :public Exception
{
public:
HttpException(const string& errmsg,int id,const string& type)
:Exception(errmsg,id)
,_type(type)
{}
virtual string what() const
{
string str = "HttpException:";
str += _type;
str += ":";
str += _errmsg;
return str;
}
private:
string _type;
};
void SQLMgr()
{
if (rand() % 7 == 0)
{
throw SqlException("权限不足", 100, "select * from name = '张三'");
}
else
{
cout << "SQLMgr 调用成功" << endl;
}
}
void CacheMgr()
{
if (rand() % 5 == 0)
{
throw CacheException("权限不足", 100);
}
else if (rand() % 6 == 0)
{
throw CacheException("数据不存在", 101);
}
else
{
cout << "CacheMgr 调用成功" << endl;
}
SQLMgr();
}
void HttpServer()
{
if (rand() % 3 == 0)
{
throw HttpException("请求资源不存在", 100, "get");
}
else if (rand() % 4 == 0)
{
throw HttpException("权限不足", 100, "post");
}
else
{
cout << "HttpException调用成功" << endl;
}
CacheMgr();
}
void HttpSever()
{
if(rand() % 3 == 0)
{
throw HttpException("请求资源不存在", 100, "get");
}
else if (rand() % 4 == 0)
{
throw HttpException("权限不足", 101, "post");
}
else
{
cout << "HttpServer调用成功" << endl;
}
CacheMgr();
}
int main()
{
srand(time(0));
while (1)
{
this_thread::sleep_for(chrono::seconds(1));
try
{
HttpSever();
}
catch (const Exception& e)//这里捕获基类,基类对象和派生对象都可以被捕捉
{
cout << e.what() << endl;
}
catch (...)//三个点表示其余情况
{
cout << "Unkown Exception" << endl;
}
}
return 0;
}
派生类和基类都可以被捕获基类的catch给捕获到。
5. 重新抛出异常
有时候我们不会因为一次尝试失败就直接放弃,要多尝试几次。
在catch到一个对象时,需要对错误进行分类,其中的某种异常错误需要进行特殊的处理,其他错误则重新抛出异常给外层调用链处理。捕获异常后需要重新抛出,直接throw就可以把捕获的对象直接给抛出去。
我们类利用上面实现的
cpp
void _SeedMsg(const string& s)
{
if (rand() % 2 == 0)
{
throw HttpException("网络不稳定,发送失败", 102, "put");
}
else if (rand() % 7 == 0)
{
throw HttpException("你不是对方好友,发送失败", 103, "put");
}
else
{
cout << "发送成功" << endl;
}
}
void SendMsg(const string& s)
{
for (size_t i = 0; i < 4; i++)
{
try
{
_SeedMsg(s);
break;
}
catch (const Exception& e)
{
//如果是102号错误,网=网络不稳定,将重新发送
//捕获异常,else中不是102号错误,将异常重新抛出
if (e.getid() == 102)
{
if (i == 3)
throw;
cout << "开始第" << i + 1 << "次重试" << endl;
}
else
throw;
}
}
}
int main()
{
srand(time(0));
while (1)
{
this_thread::sleep_for(chrono::seconds(1));
string str;
try
{
SendMsg(str);
}
catch (const Exception& e)//这里捕获基类,基类对象和派生对象都可以被捕捉
{
cout << e.what() << endl;
}
catch (...)//三个点表示其余情况
{
cout << "Unkown Exception" << endl;
}
}
return 0;
}
上述代码中,第一次如果成功了就会走break跳出循环,反之被捕获,如果是102号错误并且没有尝试三次就重新尝试(抛出),如果不是102号错误就将异常重新抛出。main函数捕捉这些抛出的异常。
6. 异常安全问题
异常抛出后,后面的代码就不再执行了,前面申请了资源(内存、锁等),后面进行释放,但是中间可能会抛异常就会导致资源没有释放,这里由于异常就引发了内存泄漏,产生安全性的问题。中间我们需要捕获异常,释放资源后面再重新抛出,对于这个问题智能指针有更好的解决方式。
并且在析构函数中,如果要抛出异常也要谨慎处理,比如析构函数要释放10个资源,释放到第五个抛出异常,则也需要捕获处理,否则后面的5个资源就没释放,也会造成内存泄漏。
cpp
double Divide(int a, int b)
{
if (b == 0)
{
throw "Divide 除数不能为0";
}
return (double)a / (double)b;
}
void Func1()
{
int* array = new int[10];
try
{
int a, b;
cin >> a>> b;
cout<<Divide(a, b)<<endl;
}
catch (...)
{
cout << "delete []" << array << endl;
delete[] array;
throw;//将异常重新抛出
}
cout << "delete []" << array << endl;
delete[] array;
}
int main()
{
try
{
Func1();
}
catch (const char* errmsg)
{
cout << errmsg << endl;
}
catch (const exception& e)
{
cout << e.what() << endl;
}
catch (...)
{
cout << "Unkown Exception" << endl;
}
return 0;
}
7. 异常规范
对于用户与编译器来说,提前知道某个程序会不会抛出异常大有裨益,知道某个函数是否会抛出异常有助于简化和调用函数的代码。
C++98中函数参数列表的后面接throw(),表示函数不抛异常,函数参数列表后面接throw(type1,type2...)表示可能会抛出多种类型的异常,可能会抛出的类型用逗号进行分割。
cpp
//C++98
//表示这个函数只会抛出bad_alloc的异常
void Func1(std::size_t a,std::size_t b) throw(std::bad_alloc);
//表示这个函数不会抛出异常
void Func2(std::size_t size, void* ptr) throw();
C++11对此进行了简化,函数参数列表后面加noexcept表示不会抛出异常,啥都不加表示可能会抛出异常。
但是编译器并不会在编译时检查noexcept,也就是说如果一个函数用noexecpt修饰了,但是同时又包含了throw语句或者调用的函数可能会抛出异常,编译器函数会顺利编译通过(有些编译器可能会报警告)。但是如果一个声明了noexcept的函数抛出了异常,程序会调用terminate来终止程序。
cpp
double Divide(int a, int b) noexcept
{
if (b == 0)
{
throw "Divide 除数不能为0";
}
return (double)a / (double)b;
}
int main()
{
try
{
int a, b;
cin >> a >> b;
cout << Divide(a, b) << endl;
}
catch(const char* errmsg)
{
cout << errmsg << endl;
}
catch (...)
{
cout << "Unkown Exception" << endl;
}
}
noexcept(expression)还可以作为一个运算符来检查一个表达式是否会抛出异常,可能会返回true,不会就返回false。
cpp
int i = 0;
cout << noexcept(Divide(1, 2)) << endl;
cout << noexcept(Divide(1, 0)) << endl;
cout << noexcept(i++) << endl;
由于上面的Divide后面加了 noexcept 所以都不会抛异常,输出如下
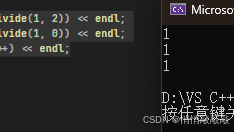