迭代器模式(Iterator Pattern)是一种行为型设计模式,它提供了一种顺序访问聚合对象元素的方法,而又不暴露该对象的内部表示。简单来说,迭代器模式的目的是将集合对象(例如列表、数组等)的遍历过程与集合的内部结构解耦,使得访问这些元素的方式更加灵活和通用。
迭代器模式提供了一种统一的方式来遍历不同的数据集合,而不需要关心集合的具体实现。这样,客户端代码不需要知道集合的具体类型或如何存储其元素,只需要使用迭代器进行遍历。
一、迭代器模式的结构
迭代器模式通常由以下几个角色组成:
- Iterator(迭代器接口) :定义访问和遍历元素的方法,如
next()
,hasNext()
,remove()
等。 - ConcreteIterator(具体迭代器):实现迭代器接口,负责具体的元素遍历逻辑。它通常保存一个指向当前遍历元素的位置。
- Aggregate(聚合接口):定义创建迭代器的方法,即提供一个方法来获取一个迭代器实例。
- ConcreteAggregate(具体聚合类):实现聚合接口,表示一个具体的集合。它可以是一个列表、数组、树或其他类型的集合类。这个类负责创建迭代器实例,并将自己的数据提供给迭代器。
二、迭代器模式的工作原理
迭代器模式的工作原理非常简单:通过使用一个迭代器对象来访问集合中的元素,客户端代码通过该迭代器进行遍历,而不需要直接访问集合对象。这使得不同类型的集合能够使用相同的接口进行遍历,而无需关心它们的内部结构。
迭代器的核心在于 hasNext()
和 next()
方法:
hasNext()
方法用于检查是否还有下一个元素可以遍历。next()
方法用于返回下一个元素并将迭代器的位置向前移动。
具体来说,迭代器负责遍历一个聚合对象(例如数组或列表),并保持遍历状态。用户可以通过迭代器逐个访问元素,而不需要关心如何遍历集合或其实现方式。
三、示例代码
以下是一个简单的迭代器模式的示例,展示了如何使用迭代器模式来遍历一个集合。
定义迭代器接口
java
public interface Iterator {
boolean hasNext();
Student next();
}
定义聚合接口
java
public interface StudentAggregate {
void addStudent(Student student);
void removeStudent(Student student);
Iterator getIterator();
}
定义具体迭代器
java
public class ConcreteIterator implements Iterator{
List<Student> students = new ArrayList<>();
int index = 0;
public ConcreteIterator(List<Student> students) {
this.students = students;
}
@Override
public boolean hasNext() {
return index < students.size();
}
@Override
public Student next() {
Student student = students.get(index);
index++;
return student;
}
}
定义具体聚合类
java
public class StudentAggregateImpl implements StudentAggregate{
List<Student> students = new ArrayList<>();
@Override
public void addStudent(Student student) {
students.add(student);
}
@Override
public void removeStudent(Student student) {
students.remove(student);
}
@Override
public Iterator getIterator() {
ConcreteIterator concreteIterator = new ConcreteIterator(students);
return concreteIterator;
}
}
实体类
java
public class Student {
private String name;
private String number;
public Student(String name, String number) {
this.name = name;
this.number = number;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", number='" + number + '\'' +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
}
使用迭代器模式的客户端代码
java
public class Client {
public static void main(String[] args) {
StudentAggregateImpl studentAggregate = new StudentAggregateImpl();
studentAggregate.addStudent(new Student("张三", "001"));
studentAggregate.addStudent(new Student("李四", "002"));
studentAggregate.addStudent(new Student("王五", "003"));
studentAggregate.addStudent(new Student("周六", "004"));
Iterator iterator = studentAggregate.getIterator();
while (iterator.hasNext()){
System.out.println(iterator.next());
}
}
}
输出结果
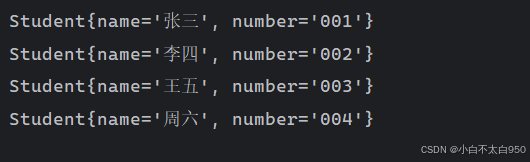
四、迭代器模式的优缺点
优点:
- 统一访问接口:迭代器模式通过提供一个统一的接口,使得不同集合类的遍历方式一致,无论是数组、列表还是其他数据结构,客户端代码都可以通过相同的迭代器进行访问。
- 减少了集合类的复杂性:通过迭代器分离了集合对象的遍历逻辑,使得集合对象的代码更加简洁,只关注元素的存储,而不需要关心如何遍历。
- 支持多重遍历:迭代器模式允许多个迭代器同时遍历同一个集合,而不需要干扰彼此的遍历过程。
缺点:
- 可能会增加类的数量:为了实现迭代器模式,可能需要为每个集合类提供一个迭代器类,这会增加类的数量,尤其是在集合类比较复杂时。
- 性能问题:对于某些复杂数据结构,迭代器的实现可能会稍微影响性能,尤其是在需要进行多次遍历的情况下。
五、应用场景
迭代器模式适用于以下几种场景:
- 集合对象的遍历:当需要遍历一个聚合对象中的元素时,迭代器模式可以提供一种标准的访问方式,而无需暴露集合的内部结构。
- 复杂数据结构的访问:对于具有复杂内部结构的对象(如树、图、链表等),可以通过迭代器模式统一访问方式,而不需要考虑数据结构的复杂性。
- 多个遍历方式的需求:当需要对同一个集合进行多种不同方式的遍历时,可以通过使用不同的迭代器来实现。例如,正向遍历、反向遍历、深度优先遍历等。