题目:手机信息管理系统
- 语言环境
- 实现语言
Java
- 环境要求
IDEA ,mysql8
- 功能要求
使用 Java语言实现手机信息管理的功能,mysql8作为数据库,主菜单包括菜单项:如图效果(1,2,3,4,5分别 实现功能,0的时候退出系统,其它的输入提示输入错误,请重新输入)
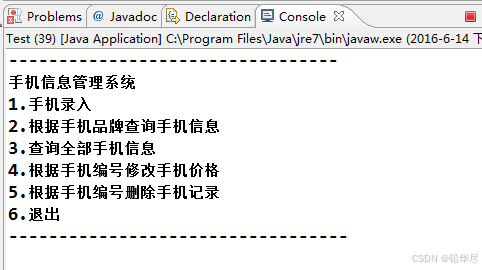
(图1)
- 数据库设计
|----|---------|------|-------------|----|----|-------|
| 表名 | Mobile || 中文表名称 | 手机信息表 |||
| 序号 | 字段名称 | 字段说明 | 类型 | 长度 | 属性 | 备注 |
| 1 | ID | 序号 | Int | 4 | 序列 | 主键,非空 |
| 2 | Brand | 品牌 | Varchar | 50 | | 非空 |
| 3 | Model | 型号 | Varchar | 50 | | 非空 |
| 4 | Price | 价格 | Double(9,2) | | | 非空 |
| 5 | Count | 数量 | Int | | | 非空 |
| 6 | Version | 版本 | Varchar | 50 | | 非空 |
- 具体实现步骤
- 创建数据库表Mobile,并输入至少3条测试数据:
- 在IDEA中创建Java项目
- 完成信息查询功能,如下图所示
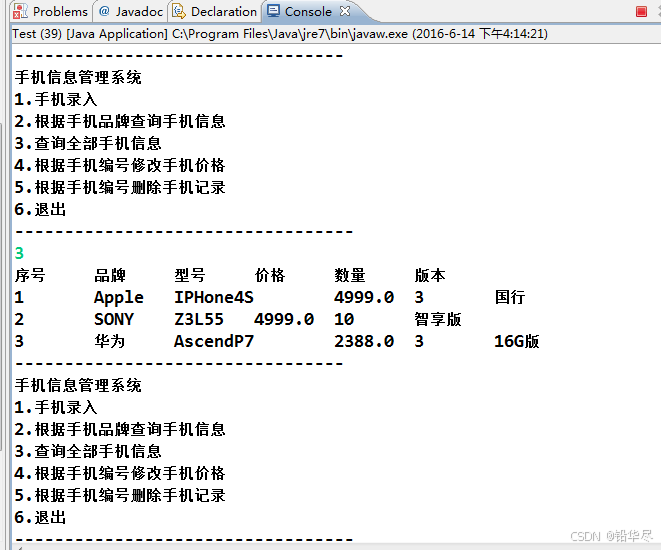
4、完成信息录入的功能。效果如下图
5完成删除的功能,效果图如下所示
6、要删除的手机编号不存在
7、要删除的手机编号存在
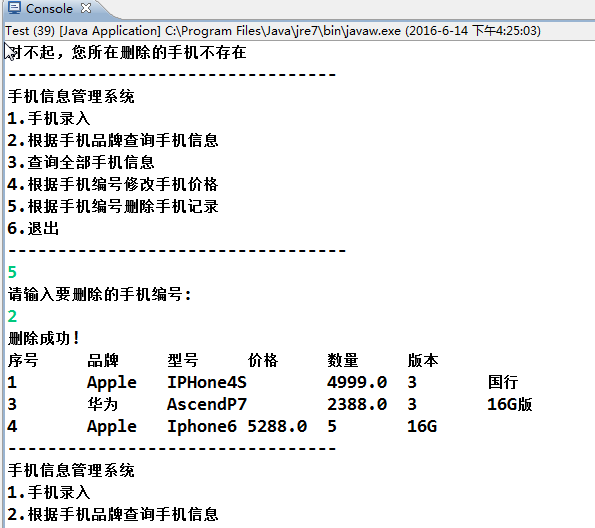
8、完成根据手机品牌查询手机信息,要求使用模糊查询,效果如图所示
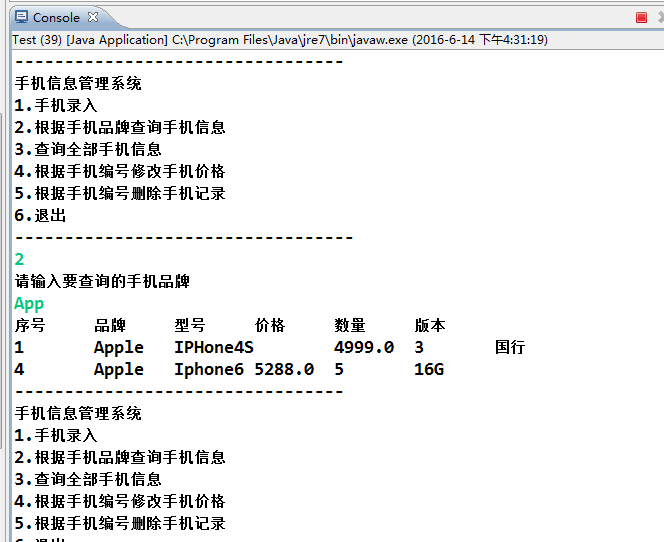
9、完成根据手机编号修改手机价格功能,效图所下图所示
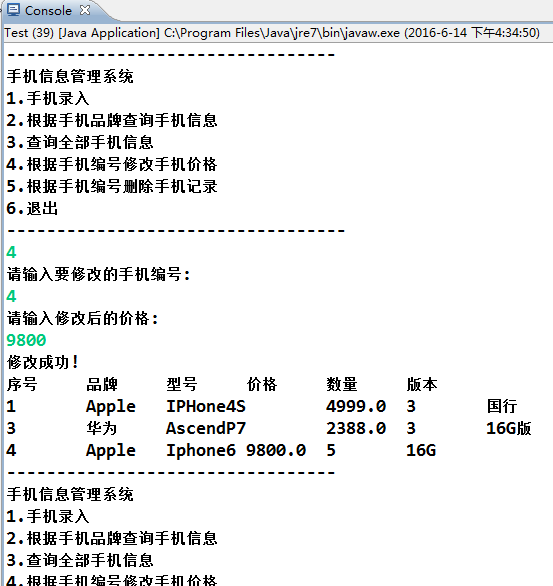
10、退出
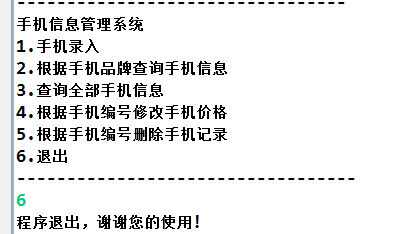
11、用户录入的选择不正确
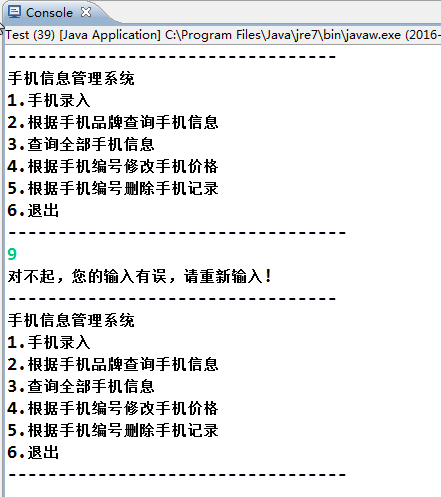
项目结构:
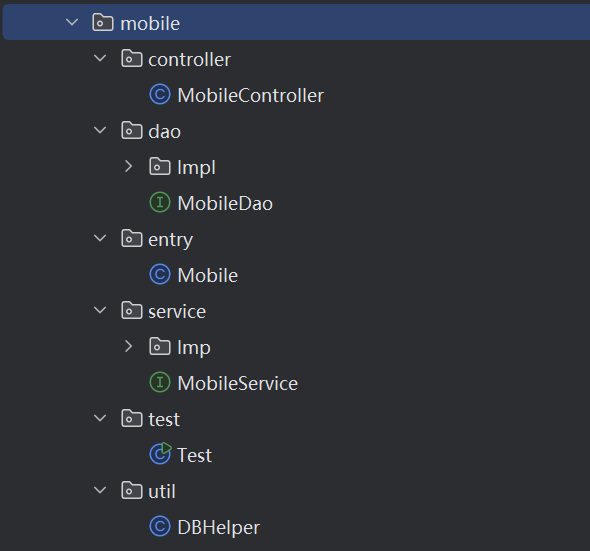
controller层:
MobileController类
java
package mobile.controller;
import mobile.entry.Mobile;
import mobile.service.Imp.MobileServiceImpl;
import mobile.service.MobileService;
import java.util.List;
import java.util.Scanner;
public class MobileController {
Scanner sc = new Scanner(System.in);
MobileService ms = new MobileServiceImpl();
public void menu() {
System.out.println("------------------------------------");
System.out.println("手机信息管理系统");
System.out.println("1、手机录入:");
System.out.println("2、根据手机品牌查询手机信息");
System.out.println("3、查询手机全部信息:");
System.out.println("4、根据手机编号修改手机价格;");
System.out.println("5、根据手机编号删除手机记录");
System.out.println("6、退出");
System.out.println("请选择:");
int index = sc.nextInt();
switch (index){
case 1:
methodadd();
break;
case 2:
methodselect();
break;
case 3:
metholdselectAll();
break;
case 4:
methodupdate();
break;
case 5:
deleteMobileById();
break;
case 6:
System.out.println("谢谢使用!");
System.exit(0);
default:
System.out.println("无效选项,请重新选择。");
}
System.out.println("------------------------------------");
System.out.println("请输入0返回");
int num = sc.nextInt();
if (num == 0) {
menu();
}
}
public void methodadd(){
System.out.println("--------------------------");
System.out.println("请输入手机品牌:");
String brand = sc.next();
System.out.println("请输入手机型号:");
String model = sc.next();
System.out.println("请输入手机价格:");
double price = sc.nextDouble();
System.out.println("请输入手机数量:");
int count = sc.nextInt();
System.out.println("请输入手机版本:");
String version = sc.next();
Mobile mobile = new Mobile();
mobile.setBrand(brand);
mobile.setModel(model);
mobile.setPrice(price);
mobile.setCount(count);
mobile.setVersion(version);
Mobile insertmobbile = ms.insertmobbile(mobile);
System.out.println("添加成功");
}
public void methodselect(){
System.out.println("请输入要查询的手机品牌:");
String brand = sc.next();
List<Mobile> mobile = ms.selectMobile(brand);
// 输出查询结果
if (mobile.isEmpty()) {
System.out.println("没有找到该品牌的手机。");
} else {
System.out.println("查询到以下手机:");
for (Mobile mobiles : mobile) {
System.out.println(mobiles);
}
}
menu();
}
public void metholdselectAll(){
List<Mobile> mb = ms.selectMobileAll();
System.out.println("序号\t品牌\t型号\t价格\t数量\t版本\t");
for(Object o : mb){
System.out.println(o.toString());
}
menu();
}
public void methodupdate(){
System.out.print("请输入手机编号:");
int id = sc.nextInt();
System.out.print("请输入新的价格:");
double price = sc.nextDouble();
boolean success = ms.updatePrice(id, price);
if (success) {
System.out.println("手机价格修改成功!");
} else {
System.out.println("手机价格修改失败,可能编号不存在。");
}
menu();
}
public void deleteMobileById() {
System.out.print("请输入要删除的手机编号:");
int id = sc.nextInt();
boolean success = ms.deleteMobile(id);
if (success) {
System.out.println("手机记录删除成功!");
} else {
System.out.println("删除失败,可能该编号不存在。");
}
menu();
}
}
dao层:
MobileDao接口
java
package mobile.dao;
import mobile.entry.Mobile;
import java.util.List;
public interface MobileDao {
int insertMobile(Mobile mobile);
List<Mobile> searchByBrand(String brand);//根据品牌查询
List<Mobile> selectMobileAll();//查询所有
boolean updatePriceById(int id, double price);//根据编号修改
boolean deleteMobileById(int id);//删除功能
}
Impl层
MobileDaoImpl类
java
package mobile.dao.Impl;
import com.util.DBHelper;
import mobile.dao.MobileDao;
import mobile.entry.Mobile;
import java.util.List;
public class MobileDaoImpl implements MobileDao {
DBHelper db = new DBHelper();
@Override
public int insertMobile(Mobile mobile) {
String sql = "INSERT INTO mobile (brand, model, price, count, version) VALUES (?, ?, ?, ?, ?)";
Object[] params = {mobile.getBrand(), mobile.getModel(), mobile.getPrice(), mobile.getCount(), mobile.getVersion()};
return db.update(sql, params);
}
@Override
public List<Mobile> searchByBrand(String brand) {
String sql = "SELECT * FROM Mobile WHERE Brand LIKE ?";
Object[] params ={"%" + brand + "%"};
return db.qurty(sql, Mobile.class, params);
}
@Override
public List<Mobile> selectMobileAll() {
String sql ="select * from mobile";
return db.qurty(sql, Mobile.class);
}
@Override
public boolean updatePriceById(int id, double price) {
String sql = "UPDATE Mobile SET Price = ? WHERE Id = ?";
Object[] params = {price, id};
int rows = db.update(sql, params);
return rows > 0;
}
@Override
public boolean deleteMobileById(int id) {
String sql = "DELETE FROM Mobile WHERE Id = ?";
Object[] params = {id};
int rows = db.update(sql, params);
return rows > 0;
}
}
entry层
Mobile类
java
package mobile.entry;
public class Mobile {
private Integer ID;
private String Brand;
private String Model;
private Double price;
private Integer Count;
private String Version;
public Mobile() {
}
public Mobile(Integer ID, String brand, String model, Double price, Integer count, String version) {
this.ID = ID;
Brand = brand;
Model = model;
this.price = price;
Count = count;
Version = version;
}
public Integer getID() {
return ID;
}
public void setID(Integer ID) {
this.ID = ID;
}
public String getBrand() {
return Brand;
}
public void setBrand(String brand) {
Brand = brand;
}
public String getModel() {
return Model;
}
public void setModel(String model) {
Model = model;
}
public Double getPrice() {
return price;
}
public void setPrice(Double price) {
this.price = price;
}
public Integer getCount() {
return Count;
}
public void setCount(Integer count) {
Count = count;
}
public String getVersion() {
return Version;
}
public void setVersion(String version) {
Version = version;
}
@Override
public String toString() {
return "Mobile{" +
"ID=" + ID +
", Brand='" + Brand + '\'' +
", Model='" + Model + '\'' +
", price=" + price +
", Count=" + Count +
", Version='" + Version + '\'' +
'}';
}
}
service层
MobileService接口
java
package mobile.service;
import mobile.entry.Mobile;
import java.util.List;
public interface MobileService {
Mobile insertmobbile(Mobile mobile);
List<Mobile> selectMobile(String brand);
List<Mobile> selectMobileAll();
boolean updatePrice(int id, double price);
boolean deleteMobile(int id);
}
Impl层
MobileServiceImpl类
java
package mobile.service.Imp;
import mobile.dao.Impl.MobileDaoImpl;
import mobile.dao.MobileDao;
import mobile.entry.Mobile;
import mobile.service.MobileService;
import java.util.List;
public class MobileServiceImpl implements MobileService {
MobileDao md = new MobileDaoImpl();
@Override
public Mobile insertmobbile(Mobile mobile) {
int result = md.insertMobile(mobile);
if (result > 0) {
return mobile;
} else {
throw new RuntimeException("工单添加失败!");
}
}
@Override
public List<Mobile> selectMobile(String brand) {
List<Mobile> mobiles = md.searchByBrand(brand);
return mobiles;
}
@Override
public List<Mobile> selectMobileAll() {
return md.selectMobileAll();
}
@Override
public boolean updatePrice(int id, double price) {
if (id <= 0) {
throw new IllegalArgumentException("手机编号必须大于 0!");
}
if (price < 0) {
throw new IllegalArgumentException("价格不能为负数!");
}
return md.updatePriceById(id, price);
}
@Override
public boolean deleteMobile(int id) {
if (id <= 0) {
throw new IllegalArgumentException("手机编号必须大于 0!");
}
return md.deleteMobileById(id);
}
}
util层
DBHelper类
java
package mobile.util;
import java.lang.reflect.Field;
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class DBHelper {
public Connection getCon(){
Connection con = null;
try{
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://127.0.0.1:3306/数据库名?useSSL=false&useUnicode=true&characterEncoding=UTF-8&serverTimezone=Asia/Shanghai&allowPublicKeyRetrieval=true";
String userName = "root";
String password = "连接密码";
con = DriverManager.getConnection(url,userName,password);
}catch(Exception e){
e.printStackTrace();
}
return con;
}
public static void Close(Connection con, PreparedStatement ps, ResultSet rs){
try {
if (con != null) {
con.close();
}
if(ps != null){
ps.close();
}
if(rs != null){
rs.close();
}
}catch(SQLException e){
e.printStackTrace();
}
}
public int update(String sql,Object...arrs){
Connection con = getCon();
PreparedStatement ps = null;
int count = 0;
try{
ps = con.prepareStatement(sql);
for (int i = 0; i <arrs.length; i++) {
ps.setObject((i+1),arrs[i]);
}
count = ps.executeUpdate();
}catch(Exception e){
e.printStackTrace();
}finally {
Close(con,ps,null);
}
return count;
}
public List qurty(String sql, Class cla , Object...arrs){
Connection con = getCon();
PreparedStatement ps = null;
ResultSet rs = null;
List list = new ArrayList<>();
try{
ps = con.prepareStatement(sql);
for (int i = 0; i <arrs.length ; i++) {
ps.setObject((i+1),arrs[i]);
}
rs = ps.executeQuery();
while(rs.next()){
Object obj = cla.newInstance();
Field[] fl = cla.getDeclaredFields();
for(Field f: fl){
f.setAccessible(true);
f.set(obj,rs.getObject(f.getName()));
}
list.add(obj);
}
}catch(Exception e){
e.printStackTrace();
}finally{
Close(con,ps,rs);
}
return list;
}
}
test层
test类
java
package mobile.test;
import mobile.controller.MobileController;
public class Test {
public static void main(String[] args) {
MobileController mc = new MobileController();
mc.menu();
}
}
运行结果:
截图太麻烦了,自己运行试一试
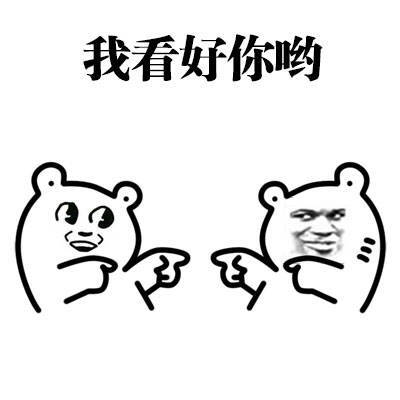