数据代理Object.defineproperty
ler person = {
name:'张三',
sex:'男',
age:18
}
console.log(Object.keys(person))
Object.keys是把对象的属性变成数组

let person = {
name: '张三',
sex: '男',
// age: 18
}
Object.defineProperty(person, 'age', {
value: 18
})
console.log(Object.keys(person));

数据代理写的不参与枚举。
在Object.defineProperty中加上enumerable:true;就可以枚举了。
不通过数据代理,可以改变值
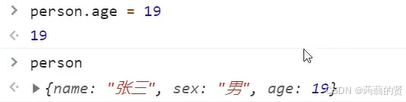
同样,代理中加上writable:true;就可以改变值。
configurable:true;控制删除
html
<script>
let number = 18
let person = {
name: '张三',
sex: '男',
// age: 18
}
Object.defineProperty(person, 'age', {
get: function () {
return number;
}
})
console.log(person);
// console.log(Object.keys(person));
</script>
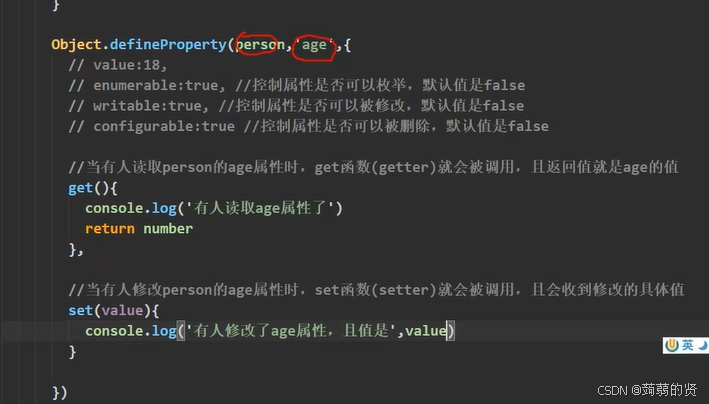
数据代理:通过一个对象代理对另一个对象中属性的操作
html
<script>
let obj = {x:100}
let obj2 = {y:100}
Object.defineProperty(obj2,'x',{
get() {
return obj.x
},
set(value) {
obj.x = value
}
})
</script>
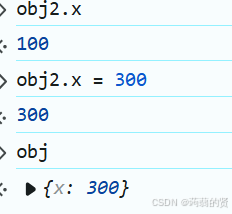
Vue中的数据代理
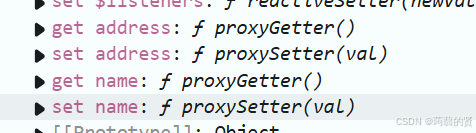
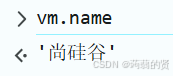
这个就是通过getter方法,调用的data的name
vm把data存储到了vm_data
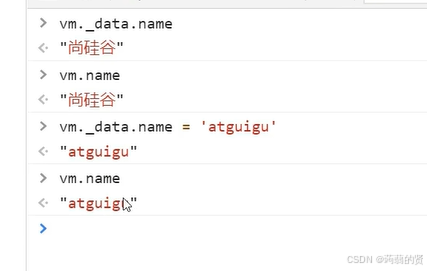
这个触发了setter
总结:
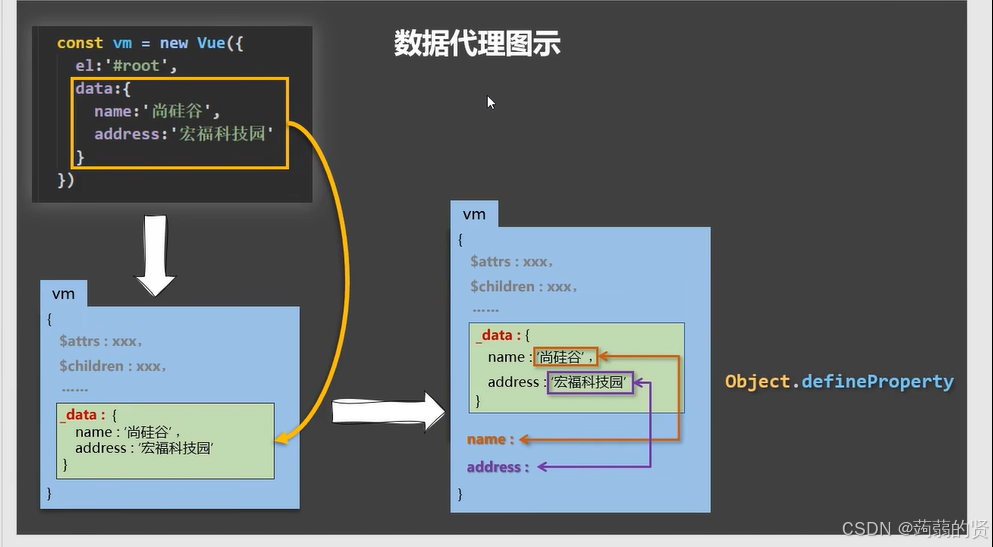
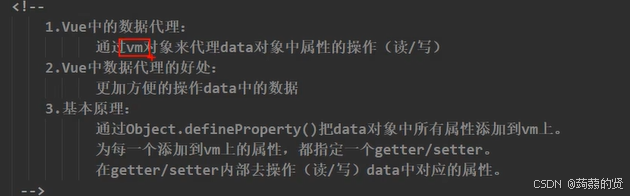
vm._data中除了data,还有一些数据劫持,用来响应页面的变化值。
事件处理:
v-on: 语法糖@
html
<body>
<div id="app">
<h1>欢迎来到{{ name }}学习</h1>
<button v-on:click="showInfo">点我提示信息</button>
</div>
</body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el: '#app',
data: {
name: '尚硅谷',
address: '北京'
},
methods: {
showInfo: () => {
alert('同学你好')
}
}
})
</script>
showInfo(event){}中 的this是vm(vue实例化对象)
showInfo()=>{ }这里面的this是window
传参时直接加小括号
<button v-on:click="showInfo2(66)">点我提示信息2</button>
showInfo2(number) {
console.log(number);
}
但是用上number就把自带的event弄丢了,
解决方法: <button v-on:click="showInfo2(66,$event)">点我提示信息2</button>
showInfo2(number,a)
a用来接收event
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div id="app">
<h1>欢迎来到{{ name }}学习</h1>
<button v-on:click="showInfo">点我提示信息</button>
<button v-on:click="showInfo2(66)">点我提示信息2</button>
</div>
</body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el: '#app',
data: {
name: '尚硅谷',
address: '北京'
},
methods: {
showInfo: () => {
alert('同学你好')
},
showInfo2(number) {
console.log(number);
}
}
})
</script>
</html>
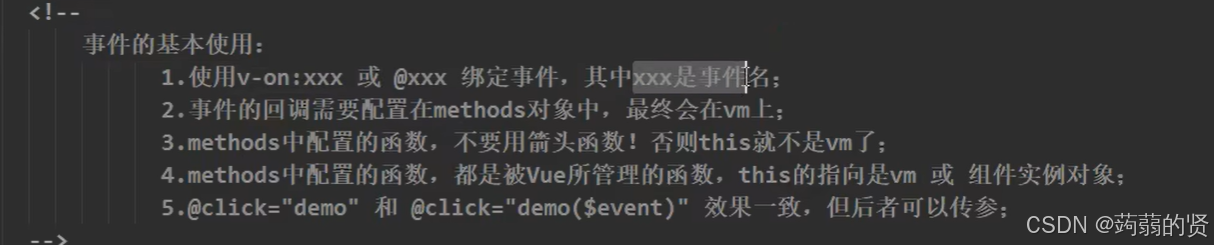
事件修饰符
prevent:
当写下面:
<a href="http://www.sdau.edu.cn" @click="showInfo"></a>
showInfo(){
alert('hello')
}
提示完之后,就自动跳转,但是不想让他跳转,
加一个preventDefault
showInfo(e) {
e.preventDefault()
alert('hello')
}
或者使用vue的@click.prevent来阻止默认行为
stop:
<div class="demo1" @click="showInfo">
<button @click="showInfo">点我跳转</button>
</div>
上面的导致事件冒泡
使用e.stopPropagation
或给button的@click.stop
once:
只触发一次
@click.once
capture:
使用事件的捕获模式,
正常下面的代码,点击div2的时候会输出2,1
这是因为从外向内捕,然后从内向外冒泡。
<div class="box1" @click="showMsg(1)">div1
<div class="box2" @clcik="showMsg(22)">div2
</div>
</div>
showMsg(msg) {
console.log(msg);
}
但是想输出1,2
使用@click.capture
self
wheel
passive
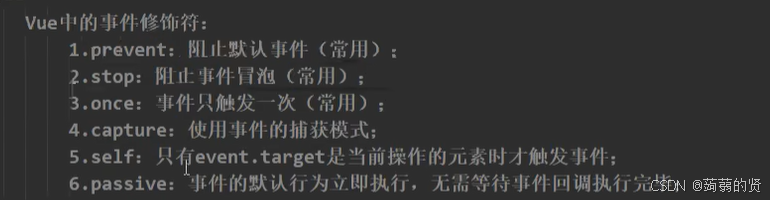
键盘事件
keydown:按下按键
keyup:按下去弹起来的时候
e.target.value显示按下的键位
e.keyCode显示ascall码,e.key显示名字
showInfo(e) {
if (e.keyCode != 13) return
console.log(e.target.value);
}
回车ascall是13
在vue中,把事件后面加上.enter就可以了.
@keyup.enter="showInfo
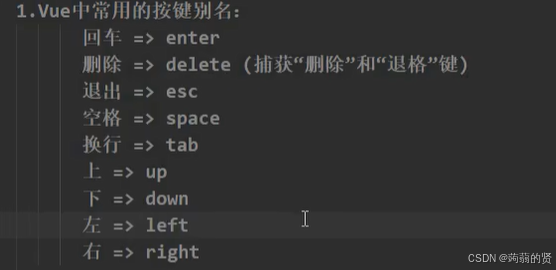
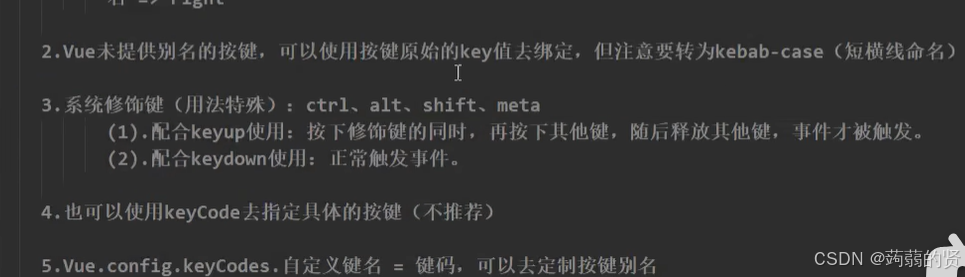
如绑定CapsLock,得写成.caps-lock
特殊;tab键得用keydown,因为keydown自动切走光标,然后再用keyup显示的时候就失效了。
所以在keydown的时候实现两个功能。
系统修饰键ctrl,alt,shift,meat如果用keyup,得是这个按键加其他被抬起才生效,
所以基本上用keydown
自定义按键名:
@keydown.huiche="showInfo"
Vue.config.keyCodes.huiche = 13;
补充:
@click.stop.prevent修饰符可以连用,先阻止冒泡,再阻止弹窗
@keyup.ctrl.y,按下ctrl+y的时候实现功能
姓名案例:
html
<!-- 法一:插值 -->
<body>
<div id="app">
<input type="text" v-model="firstname">
<input type="text" v-model="lastname">
<h1>姓名:{{ firstname.slice(0,3) }}-{{ lastname }}</h1>
</div>
</body>
<script>
const vm = new Vue({
el: '#app',
data: {
firstname: '张',
lastname: '三'
},
methods: {
}
})
</script>
当要处理的步骤很多,{{ firstname.slice(0,3) }}就得写一大串,
这时应该放到计算属性中。
法二:函数
methods: {
fullName() {
return this.firstname + '--' + this.lastname
}
}
<h1>姓名:{{ fullName() }}</h1>
法三:计算属性
html
computed: {
fullName: {
get() {
return this.firstname + '-' + this.lastname
}
}
}
当有人读取fullName时,get就会被调用,返回值作为fullName的值
computed比method调用省时间,比如显示四次姓名,method要访问四次,computed有缓存,
只需要计算一次。
计算属性还有set()
当fullName被修改时,调用set
html
set(value) {
const arr = value.split('-')
this.firstname = arr[0]
this.lastname = arr[1]
}
}
简写(只考虑读取,不考虑修改才可以简写)
html
fullName:function(){
return this.firstname + '-' + this.lastname
}
fullName(){
return this.firstname + '-' + this.lastname
}
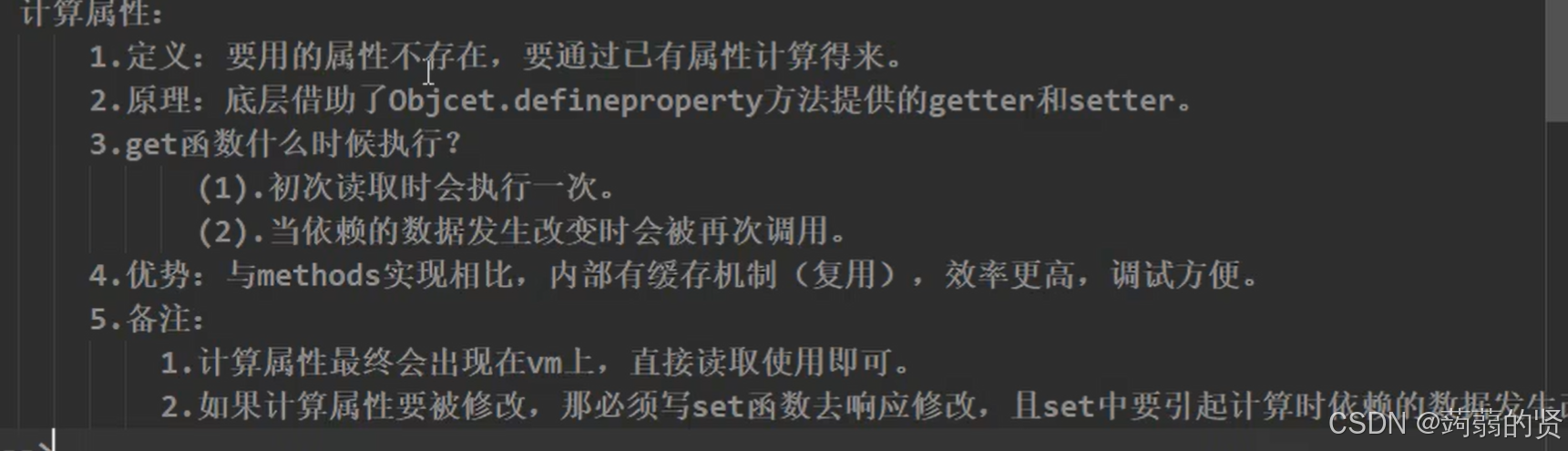