1.cmakelist.txt
cmake_minimum_required(VERSION 3.16)
project(pthread_linux_test LANGUAGES C)
# 查找Threads库(包含pthread支持)
find_package(Threads REQUIRED)
if (Threads_FOUND)
message(STATUS "成功找到Threads库")
endif()
add_executable(pthread_linux_test main.c
threadpool.c threadpool.h
)
# 链接Threads库到可执行文件
target_link_libraries(pthread_linux_test Threads::Threads)
include(GNUInstallDirs)
install(TARGETS pthread_linux_test
LIBRARY DESTINATION ${CMAKE_INSTALL_LIBDIR}
RUNTIME DESTINATION ${CMAKE_INSTALL_BINDIR}
)
2.测试代码
#include <stdio.h>
#include "threadpool.h"
void taskfunc(void* arg) {
int num = *(int*)arg;
printf("thread %ld is working, number=%d\n", pthread_self(), num);
sleep(1);
}
int main()
{
//创建线程池
ThreadPool* pool = threadPoolCreate(3, 10, 100);
for (int i = 0; i < 100; i++) {
int* num = (int*)malloc(sizeof(int));
*num = i + 100;
threadPoolAdd(pool, taskfunc, num);
}
sleep(30);
threadPoolDestroy(pool);
printf("Hello World!\n");
return 0;
}
3.结果
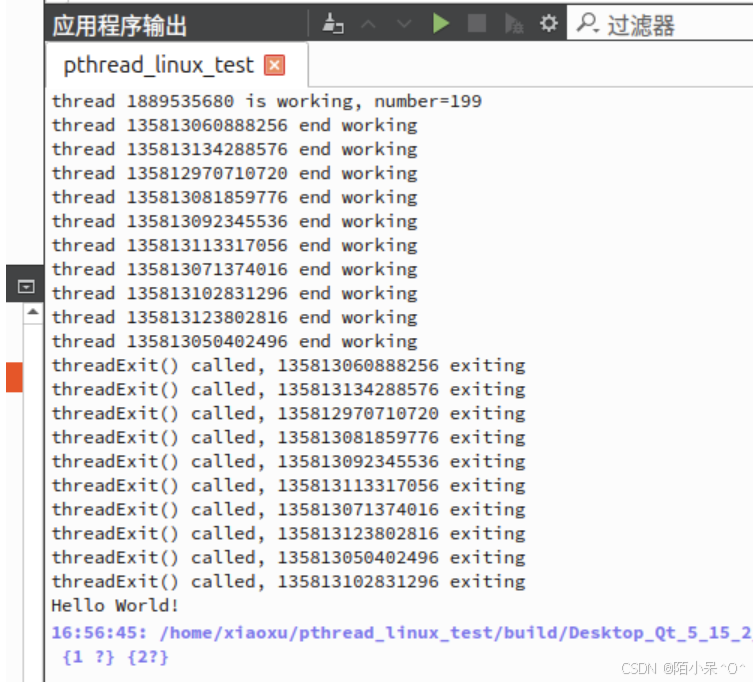