javascript
const { Web3 } = require('web3')
let apiKeyOnServer = process.env.apiKeyOnServer
const web3 = new Web3(
`https://bsc-mainnet.infura.io/v3/${process.env.metamaskApiKey}`
)
const getWeb3Instance = () => {
let endpoints = [
{
url: 'https://polygon-mainnet.infura.io/v3/',
name: 'polygon',
},
// {
// url: 'https://mainnet.infura.io/v3/',
// name: 'mainnet',
// },
{
url: 'https://bsc-mainnet.infura.io/v3/',
name: 'bscMainnet',
},
]
let web3InstanceObj = {}
endpoints.forEach((item) => {
let instance = new Web3(item.url + process.env.metamaskApiKey)
web3InstanceObj[item.name] = {
...item,
instance,
}
})
return web3InstanceObj
}
let web3InstanceObj = getWeb3Instance()
//查询
const web3OnAzure = async (req, res, type) => {
const { pageNum = 1, pageSize = 10, apiKey = 'sk-xxx' } = req.body
if (apiKey === apiKeyOnServer) {
// let getBlockNumberRes = await web3.eth.getBlockNumber()
// console.log(getBlockNumberRes)
// const chainId = await web3.eth.getChainId()
console.log('binance钱包')
for (let key in web3InstanceObj) {
let bBalance = await web3InstanceObj[key].instance.eth.getBalance(
process.env.binanceWallet1
)
// console.log(web3InstanceObj[key].name, bBalance)
bBalance = web3.utils.fromWei(bBalance, 'ether')
console.log(web3InstanceObj[key].name + ' ether', bBalance)
}
console.log('币安交易账号地址')
for (let key in web3InstanceObj) {
let bBalance = await web3InstanceObj[key].instance.eth.getBalance(
process.env.binanceMarketWallet1
)
// console.log(web3InstanceObj[key].name, bBalance)
bBalance = web3.utils.fromWei(bBalance, 'ether')
console.log(web3InstanceObj[key].name + ' ether', bBalance)
}
console.log('onekey钱包')
for (let key in web3InstanceObj) {
let bBalance = await web3InstanceObj[key].instance.eth.getBalance(
process.env.onkeyWallet1
)
// console.log(web3InstanceObj[key].name, bBalance)
bBalance = web3.utils.fromWei(bBalance, 'ether')
console.log(web3InstanceObj[key].name + ' ether', bBalance)
}
console.log('公开的钱包')
for (let key in web3InstanceObj) {
let bBalance = await web3InstanceObj[key].instance.eth.getBalance(
'0xd8dA6BF26964aF9D7eEd9e03E53415D37aA96045'
)
// console.log(web3InstanceObj[key].name, bBalance)
bBalance = web3.utils.fromWei(bBalance, 'ether')
console.log(web3InstanceObj[key].name + ' ether', bBalance)
}
// const gasPrice = await web3.eth.getGasPrice()
// console.log('gasPrice', gasPrice)
// get the balance of an address
// let wallet1 = web3.eth.accounts.wallet.create(1)
// console.log('wallet1', wallet1)
// const account = web3.eth.accounts.create()
// console.log(account)
// // use the account to sign a message
// const signature = account.sign('Hello, Web3.js!')
// console.log(signature)
res.send({
code: 200,
data: {
// getBlockNumberRes: getBlockNumberRes + '',
// chainId: chainId + '',
},
message: '成功',
})
} else {
res.send({
code: 400,
message: '失败:参数apiKey',
})
}
}
module.exports = {
web3OnAzure,
}
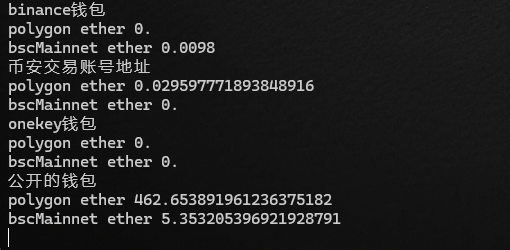
人工智能学习网站