前言
解释器模式是用来解释和执行特定的语法或表达式。它将一种表达式的规则和语义进行抽象和封装,然后通过解释器来解析和执行这些规则,将其转化为可执行的操作。
代码
csharp
//抽象表达式
public interface Expression
{
int Interpret(Context context); //解释方法
}
//终止符表达式
public class NumberExpression : Expression
{
private int number;
public NumberExpression(int number)
{
this.number = number;
}
public int Interpret(Context context)
{
return number;
}
}
//非终结符表达式
public class AddExpression : Expression
{
private Expression Left;
private Expression Right;
public AddExpression(Expression left, Expression right)
{
this.Left = left;
this.Right = right;
}
public int Interpret(Context context)
{
return Left.Interpret(context)+Right.Interpret(context);
}
}
//非终结符表达式
public class MultiplyExpression:Expression
{
private Expression Left;
private Expression Right;
public MultiplyExpression(Expression left, Expression right)
{
Left = left;
Right = right;
}
public int Interpret(Context context)
{
return Right.Interpret(context)*Left.Interpret(context);
}
}
// 上下文
public class Context
{
private int variable;
public int Variable { get { return variable; } set { variable = value; } }
}
/*
* 行为型模式:Behavioral Pattern
* 解释器模式:Interpreter Pattern
*/
internal class Program
{
static void Main(string[] args)
{
//解释器语法术
Expression expression = new AddExpression(new NumberExpression(120),
new AddExpression(
new NumberExpression(5),
new NumberExpression(2))
);
Context context = new Context();
int result = expression.Interpret(context);
Console.WriteLine($"{result}");
//
Expression multiflyExpression = new MultiplyExpression(new NumberExpression(2),new NumberExpression(6));
Context context2 = new Context();
context2.Variable = 5;
int result2 = multiflyExpression.Interpret(context2);
Console.WriteLine($"{result2}{Environment.NewLine}{context2.Variable}");
Console.ReadLine();
}
}
运行结果
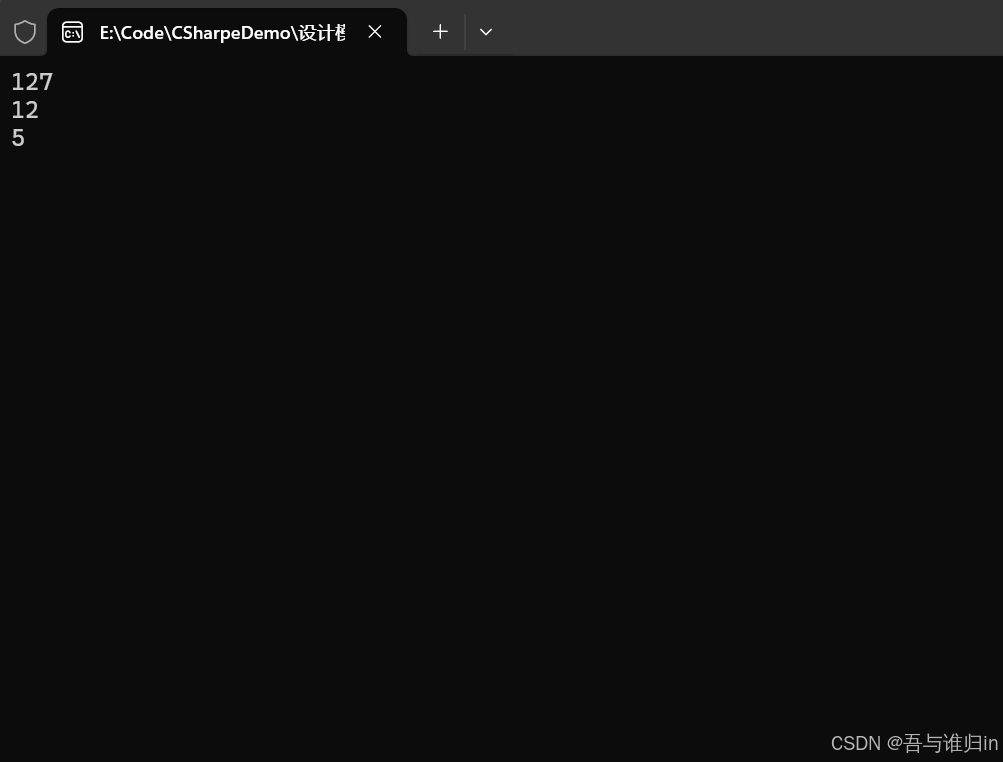