当前大部分照片尺寸大于5MB,而50MB限制的PHP通常上传4MB左右
于是就需要压缩后上传,上5+代码使用后筛选的代码
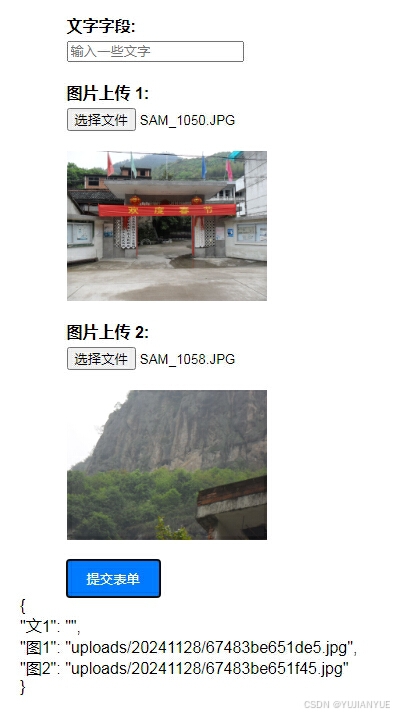
html
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$uploadDir = 'uploads/' . date('Ymd') . '/';
if (!is_dir($uploadDir)) {
mkdir($uploadDir, 0777, true);
}
$results = [];
foreach ($_FILES as $key => $file) {
$targetFile = $uploadDir . uniqid() . '.jpg';
if (move_uploaded_file($file['tmp_name'], $targetFile)) {
$_POST[$key] = $targetFile;
} else {
$_POST[$key] = '上传失败';
}
}
header('Content-Type: application/json');
echo json_encode($_POST); exit();
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>图片压缩上传</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
form {
max-width: 600px;
margin: auto;
}
.form-group {
margin-bottom: 20px;
}
label {
display: block;
margin-bottom: 5px;
font-weight: bold;
}
input[type="file"] {
margin-bottom: 10px;
}
img.preview {
display: block;
max-width: 200px;
max-height: 200px;
margin-top: 10px;
}
button {
padding: 10px 20px;
background-color: #007BFF;
color: #FFF;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:disabled {
background-color: #CCC;
cursor: not-allowed;
}
</style>
</head>
<body>
<h1>表单图片上传</h1>
<form id="data-form">
<div class="form-group">
<label for="text-input">文字字段:</label>
<input type="text" id="text-input" name="文1" placeholder="输入一些文字">
</div>
<div class="form-group">
<label for="image1">图片上传 1:</label>
<input type="file" id="image1" name="图1" accept="image/*">
<img id="preview1" class="preview" src="" alt="预览图片" style="display: none;">
</div>
<div class="form-group">
<label for="image2">图片上传 2:</label>
<input type="file" id="image2" name="图2" accept="image/*">
<img id="preview2" class="preview" src="" alt="预览图片" style="display: none;">
</div>
<button type="button" id="submit-button" disabled>提交表单</button>
</form>
<div id="result"></div>
<script>
const form = document.getElementById('data-form');
const submitButton = document.getElementById('submit-button');
let compressedImages = {}; // 用于存储压缩后的图片 Blob
// 监听文件选择
form.addEventListener('change', async (event) => {
if (event.target.type === 'file') {
const input = event.target;
const preview = document.getElementById(`preview${input.id.match(/\d+/)[0]}`);
if (input.files && input.files[0]) {
const file = input.files[0];
if (!file.type.startsWith('image/')) {
alert('请选择图片文件!');
return;
}
try {
const compressedBlob = await compressImage(file, 1024, 0.8); // 压缩图片
compressedImages[input.name] = compressedBlob; // 存储压缩后的 Blob
// 预览压缩后的图片
const previewURL = URL.createObjectURL(compressedBlob);
preview.src = previewURL;
preview.style.display = 'block';
// 激活提交按钮
submitButton.disabled = false;
} catch (err) {
console.error('图片压缩失败:', err);
}
}
}
});
// 压缩图片函数
function compressImage(file, maxWidth, quality) {
return new Promise((resolve, reject) => {
const reader = new FileReader();
reader.onload = function (event) {
const img = new Image();
img.onload = function () {
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
// 计算缩放比例
let width = img.width;
let height = img.height;
if (width > maxWidth) {
height = height * (maxWidth / width);
width = maxWidth;
}
// 绘制到 Canvas
canvas.width = width;
canvas.height = height;
ctx.drawImage(img, 0, 0, width, height);
// 导出压缩后的图片 Blob
canvas.toBlob(
(blob) => {
if (blob) resolve(blob);
else reject(new Error('图片压缩失败'));
},
file.type || 'image/jpeg',
quality
);
};
img.onerror = function () {
reject(new Error('图片加载失败'));
};
img.src = event.target.result;
};
reader.onerror = function () {
reject(new Error('文件读取失败'));
};
reader.readAsDataURL(file);
});
}
// 提交表单
submitButton.addEventListener('click', () => {
const formData = new FormData(form);
// 替换图片字段为压缩后的 Blob
Object.keys(compressedImages).forEach((name) => {
formData.delete(name); // 删除原始图片
formData.append(name, compressedImages[name], `${name}.jpg`); // 添加压缩后的图片
});
// 使用 AJAX 提交表单
fetch('?act=upload', {
method: 'POST',
body: formData,
})
.then((response) => response.json())
.then((data) => {
console.log(data);
document.getElementById('result').innerText = JSON.stringify(data, null, 2);
alert('上传成功');
})
.catch((error) => {
console.error(error);
alert('上传失败');
});
});
</script>
</body>
</html>