文章目录
-
-
- 0.写在前面(对mybatis,spring的理解)(不看可跳过)
-
- [0.1 为什么需要mybatis](#0.1 为什么需要mybatis)
- [0.2 为什么需要spring](#0.2 为什么需要spring)
- 0.3为什么需要springmvc
- 1.新建ssmbuild数据库
- 2.新建Maven项目
- 3.初始化步骤
- [3.1 配置下载maven依赖,构建资源导出](#3.1 配置下载maven依赖,构建资源导出)
-
- [3.2 连接数据库](#3.2 连接数据库)
- 3.3建包,建mybatis,数据库和spring配置文件
- 4.配置dao,service,pojo包下的文件
- 5.配置spring-dao.xml和spring-service.xml文件
- 6.spring-mvc相关配置
- 7.编写Controller文件
- 8.前端界面渲染
-
0.写在前面(对mybatis,spring的理解)(不看可跳过)
0.1 为什么需要mybatis
1.传统的jdbc代码,有很多重复的代码块。比如:数据取出时候的封装,数据库的建立连接等,操作非常繁琐...,通过框架可以减少重复代码,提高开发效率
2.为什么需要编写一个工具类,获得sqlSession对象?答:用于执行 SQL 语句、管理数据库连接、事务以及缓存
3.mybatis通过xml语句实现数据的增删改查
4.使用流程:4.1写好映射接口,UserMapper.java,4.2.写好对应的映射文件(增删改查语句,结果集)usermapper.xml 4.3mybatis-config.xml引用该配置文件 4.3 sqlsesion访问usermapper接口调用方法
0.2 为什么需要spring
1.对于一个实体类,无需创建对象,通过简单的bean配置就能实现对象的创建
2.支持注解。直接再某个类上面使用@Component,对应的Bean就不需要写了,简化代码
3.支持自动装配,某个类里面引用了其他类,再其他类上面加一个@Autowired,就需要再bean里面用ref引用了
4.配置数据源,帮mybatis完成这项工作
0.3为什么需要springmvc
1.后端的数据方便直接封装和返回给前端界面
1.新建ssmbuild数据库
1.打开Navicat
2.点击新建查询
3.输入一下语句代码
shell
CREATE DATABASE `ssmbuild`;
USE `ssmbuild`;
DROP TABLE IF EXISTS `books`;
CREATE TABLE `books` (
`bookID` INT(10) NOT NULL AUTO_INCREMENT COMMENT '书id',
`bookName` VARCHAR(100) NOT NULL COMMENT '书名',
`bookCounts` INT(11) NOT NULL COMMENT '数量',
`detail` VARCHAR(200) NOT NULL COMMENT '描述',
KEY `bookID` (`bookID`)
) ENGINE=INNODB DEFAULT CHARSET=utf8
INSERT INTO `books` (`bookID`, `bookName`, `bookCounts`, `detail`) VALUES
(1, 'Java', 1, '从入门到放弃'),
(1, 'MySQL', 10, '从删库到跑路'),
(3, 'Linux', 5, '从入门到坐牢');
2.新建Maven项目
2.1 操作步骤
2.2 初始项目结构
3.初始化步骤
3.1 配置下载maven依赖,构建资源导出
xml
<!--依赖, junit, 连接池,servlet,jsp,mybatis,mybatis-spring,spring,-->
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
</dependency>
<!--数据库驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.24</version>
</dependency>
<!--数据库连接池-->
<dependency>
<groupId>com.mchange</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.5.2</version>
</dependency>
<!--Servlet JSP-->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!--Mybatis-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.2</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.2</version>
</dependency>
<!--Spring-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
</dependencies>
<!--静态资源导出问题-->
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
</build>
3.2 连接数据库
3.3建包,建mybatis,数据库和spring配置文件
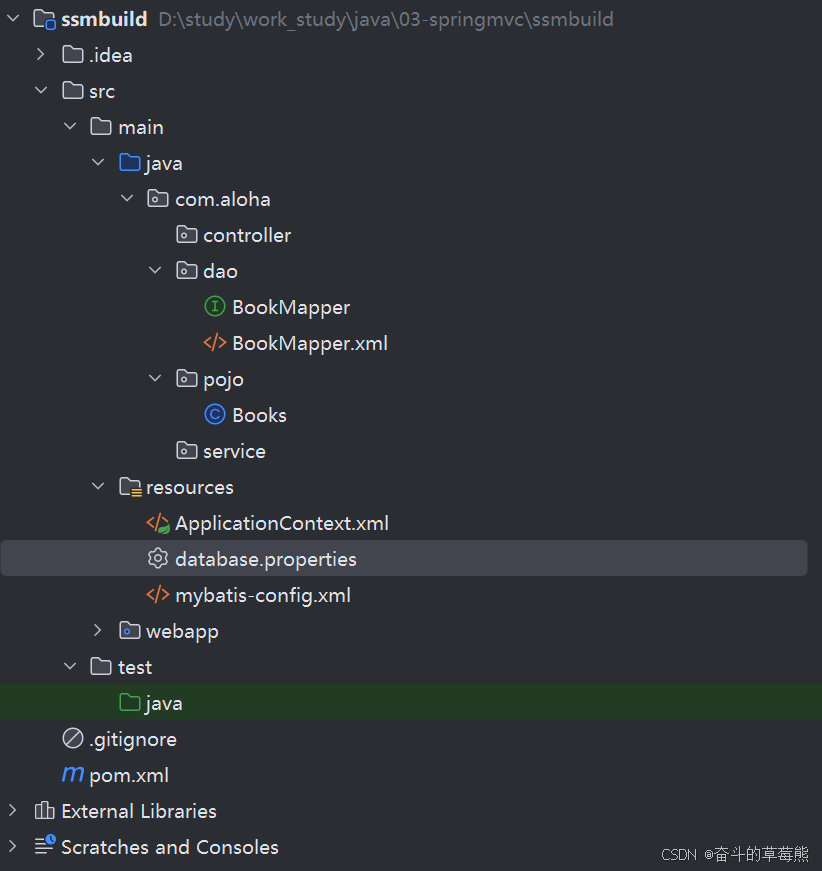
mybatis-config.xml文件代码
xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!--配置数据源,交给Spring去做-->
<typeAliases>
<package name="com.aloha.pojo"/>
</typeAliases>
</configuration>
applicationContext.xml文件代码
xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
</beans>
database.properties代码文件
xml
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/ssmbuild?useSSL=false&useUnicode=true&characterEncoding=utf8&serverTimezone=UTC
jdbc.username=root
jdbc.password=123456
4.配置dao,service,pojo包下的文件
结构如下
4.1新建实体类Books
新建pojo中Books实体类
java
package com.aloha.pojo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class Books {
private int bookID;
private String bookName;
private int bookCounts;
private String detail;
}
4.2新建dao接口和对应xml文件
BookMapper
java
package com.aloha.dao;
import com.aloha.pojo.Books;
import org.apache.ibatis.annotations.Param;
import java.awt.print.Book;
import java.util.List;
public interface BookMapper {
// 增加一本书
int addBook(Books books);
// 删除一本书
int deleteBookById(@Param("bookId") int id);
// 更新一本书
int updateBook(Books books);
// 查询一本书
Books queryBookById(@Param("bookId") int id);
// 查询全部的书
List<Books> queryAllBooks();
}
BookMapper.xml
xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.aloha.dao.BookMapper">
<insert id="addBook" parameterType="Books">
insert into ssmbuild.books (bookName, bookCounts, detail)
values (#{bookName}, #{bookCounts}, #{detail})
</insert>
<delete id="deleteBookById" parameterType="int">
delete from ssmbuild.books where bookID = #{bookId}
</delete>
<update id="updateBook" parameterType="Books">
update ssmbuild.books
set bookName=#{bookName}, bookCounts=#{bookCounts}, detail=#{detail}
where bookID=#{bookID}
</update>
<select id="queryBookById" resultType="Books">
select * from ssmbuild.books where bookID = #{bookId}
</select>
<select id="queryAllBooks" resultType="Books">
select * from ssmbuild.books
</select>
</mapper>
4.3新建service接口和实现类
BookService
java
package com.aloha.service;
import com.aloha.pojo.Books;
import org.apache.ibatis.annotations.Param;
import java.util.List;
public interface BookService {
// 增加一本书
int addBook(Books books);
// 删除一本书
int deleteBookById(int id);
// 更新一本书
int updateBook(Books books);
// 查询一本书
Books queryBookById(int id);
// 查询全部的书
List<Books> queryAllBooks();
}
BookServiceImpl
java
package com.aloha.service;
import com.aloha.dao.BookMapper;
import com.aloha.pojo.Books;
import java.util.Collections;
import java.util.List;
public class BookServiceImpl implements BookMapper {
// service调dao层,业务层调用dao层就对了:组合Dao
private BookMapper bookMapper;
public void setBookMapper(BookMapper bookMapper) {
this.bookMapper = bookMapper;
}
@Override
public int addBook(Books books) {
return bookMapper.addBook(books);
}
@Override
public int deleteBookById(int id) {
return bookMapper.deleteBookById(id);
}
@Override
public int updateBook(Books books) {
return bookMapper.updateBook(books);
}
@Override
public Books queryBookById(int id) {
return bookMapper.queryBookById(id);
}
@Override
public List<Books> queryAllBooks() {
return bookMapper.queryAllBooks();
}
}
5.配置spring-dao.xml和spring-service.xml文件
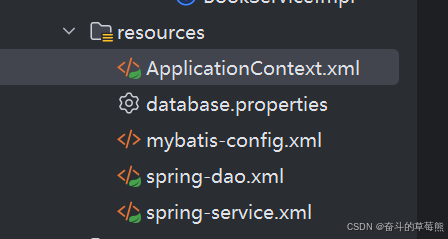
resources文件目录下的spring-dao.xml
xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.0.xsd">
<!--1.关联数据库配置文件-->
<context:property-placeholder location="classpath:database.properties"/>
<!--2.连接池
dbcp: 半自动化,不能自动链接
c3p0:自动化操作(自动化加载配置文件,并且可以自动设置到对象中)
druid
hikari
-->
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driver}"/>
<property name="jdbcUrl" value="${jdbc.url}"/>
<property name="user" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
<!-- c3p0连接池的私有属性 -->
<property name="maxPoolSize" value="30"/>
<property name="minPoolSize" value="10"/>
<!-- 关闭连接后不自动commit -->
<property name="autoCommitOnClose" value="false"/>
<!-- 获取连接超时时间 -->
<property name="checkoutTimeout" value="10000"/>
<!-- 当获取连接失败重试次数 -->
<property name="acquireRetryAttempts" value="2"/>
</bean>
<!--3.sqlSessionFactory-->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<!--绑定Mybatis配置文件-->
<property name="configLocation" value="classpath:mybatis-config.xml"/>
</bean>
<!--配置dao接口扫描包,动态实现Dao接口可以注入到Spring容器中-->
<!--意思是这个配置文件,mapper接口就不需要使用bean注入了-->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<!--注入sqlSessionFactory-->
<property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"/>
<!--要扫描的dao包-->
<property name="basePackage" value="com.aloha.dao"/>
</bean>
</beans>
spring-service.xml
xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.0.xsd">
<!--1.扫描service下的包,将它们自动注册为 Spring 容器的 Bean-->
<context:component-scan base-package="com.aloha.service"/>
<!--2.将我们所有的业务类,注入到Spring,可以通过配置或注解实现-->
<bean id="bookServiceImpl" class="com.aloha.service.BookServiceImpl">
<property name="bookMapper" ref="bookMapper"/>
</bean>
<!--3.声明式事务配置-->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<!--注入数据源-->
<property name="dataSource" ref="dataSource"/>
</bean>
<!--4.aop事务支持-->
</beans>
ApplicationContext进行关联
xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<import resource="classpath:spring-dao.xml"/>
<import resource="classpath:spring-service.xml"/>
</beans>
6.spring-mvc相关配置
6.1配置web.xml
xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!--DispatchServlet-->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<!--乱码过滤-->
<filter>
<filter-name>encondingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encondingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!--Session-->
<session-config>
<session-timeout>15</session-timeout>
</session-config>
</web-app>
6.2配置spring-mvc.xml
xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.0.xsd">
<!--1.注解驱动,这些功能涉及 Web 层的请求映射、数据绑定和响应处理。处理映射器,处理器适配器-->
<mvc:annotation-driven/>
<!--2.静态资源过滤-->
<mvc:default-servlet-handler/>
<!--3.扫描包controller,将该类自动注册为 Spring 容器的 Bean-->
<context:component-scan base-package="com.aloha.controller"/>
<!--4.视图解析器-->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/jsp/"/>
<property name="suffix" value=".jsp"/>
</bean>
</beans>
最后
7.编写Controller文件
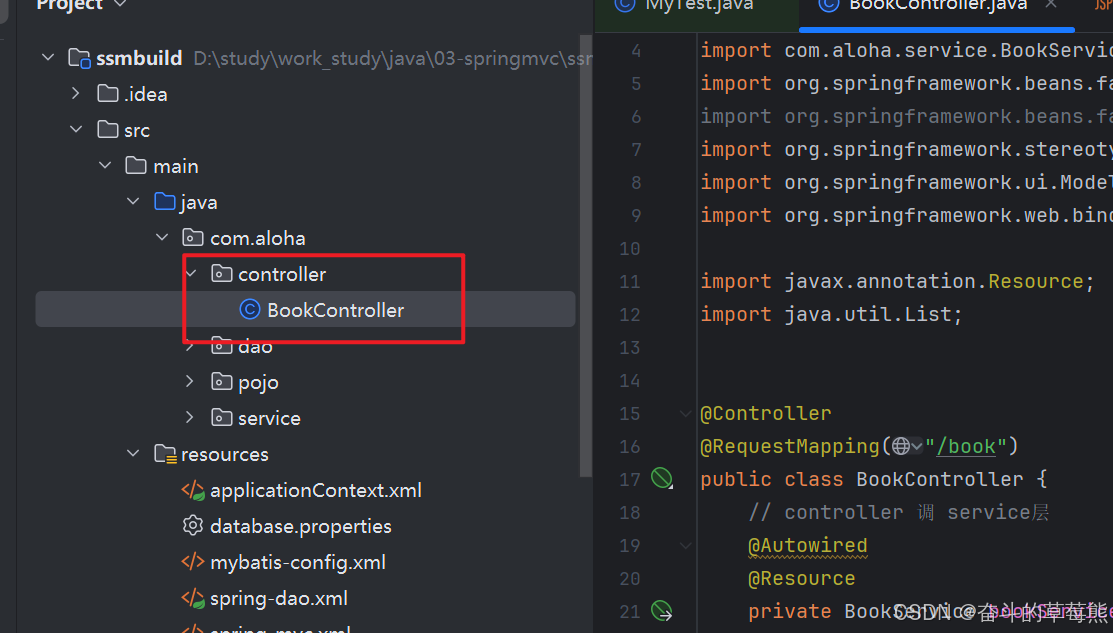
java
package com.aloha.controller;
import com.aloha.pojo.Books;
import com.aloha.service.BookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.annotation.Resource;
import java.util.List;
@Controller
@RequestMapping("/book")
public class BookController {
// controller 调 service层
@Autowired
@Resource
private BookService bookService; // 属性自动注入
@RequestMapping("/allBook")
public String list(Model model) {
List<Books> list = bookService.queryAllBooks();
model.addAttribute("list", list);
return "allBook"; // 返回的jsp界面
}
}
8.前端界面渲染
index.jsp
html
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<body>
<h2>Hello World!</h2>
<style>
a {
text-decoration: none;
color: black;
}
h3 {
width: 180px;
height: 38px;
margin: 100px auto;
text-align: center;
line-height: 38px;
background: deepskyblue;
border-radius: 5px;
}
</style>
</body>
<h3>
<a href="${pageContext.request.contextPath}/book/allBook">进入书籍界面</a>
</h3>
</html>
jsp/allBook.jsp
html
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%--
Created by IntelliJ IDEA.
User: aloha
Date: 2024/11/30
Time: 11:28
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>书籍展示</title>
<!--Bootsthap美化界面-->
<link href="https://cdn.staticfile.org/twitter-bootsthap/3.3.7/css/bootsthap.min.css" rel="stylesheet">
</head>
<body>
<div class="continer">
<div class="row clearfix">
<div class="col-md-12 column">
<div class="page-header">
<small>书籍列表 ------ 显示所有书籍</small>
</div>
</div>
<div class="row clearfix">
<div class="col-md-12 column">
<table class="table table-hover table-striped">
<thead>
<tr>
<th>书籍编号</th>
<th>书籍名称</th>
<th>书籍数量</th>
<th>书籍详情</th>
</tr>
</thead>
<tbody>
<c:forEach var="book" items="${list}">
<tr>
<td>${book.bookID}</td>
<td>${book.bookName}</td>
<td>${book.bookCounts}</td>
<td>${book.detail}</td>
</tr>
</c:forEach>
</tbody>
</table>
</div>
</div>
</div>
</div>
</body>
</html>
渲染结果
成功!!!!