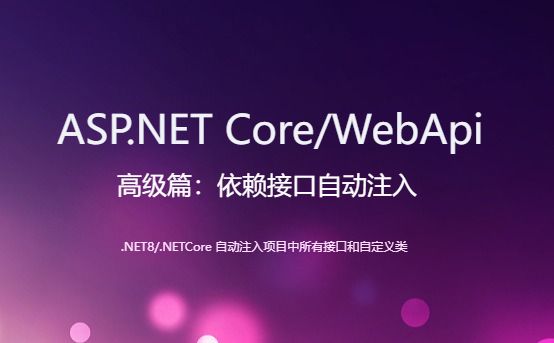
.NET8/.NETCore 依赖接口注入:自动注入项目中所有接口和自定义类
目录
自定义依赖接口
需要依赖注入的类必须实现以下接口。
C#
/// <summary>
/// 依赖接口
/// </summary>
public interface IDependency { }
/// <summary>
/// 注入接口,生命周期:Transient
/// </summary>
public interface ITransientDependency : IDependency { }
/// <summary>
/// 注入接口,生命周期:Scoped
/// </summary>
public interface IScopedDependency : IDependency { }
/// <summary>
/// 注入接口,生命周期:Singleton
/// </summary>
public interface ISingletonDependency : IDependency { }
扩展类:HostExtensions AddInjectionServices方法
C#
public static class HostExtensions
{
/// <summary>
/// 自动注入接口, 注入到服务容器IServiceCollection
/// </summary>
/// <param name="services"></param>
/// <returns></returns>
public static IServiceCollection AddInjectionServices(this IServiceCollection services)
{
//服务生命周期映射
Dictionary<Type, ServiceLifetime> map = new Dictionary<Type, ServiceLifetime>
{
{ typeof(ITransientDependency),ServiceLifetime.Transient },
{ typeof(IScopedDependency),ServiceLifetime.Scoped },
{ typeof(ISingletonDependency),ServiceLifetime.Singleton },
{ typeof(IDependency),ServiceLifetime.Scoped },
};
//获取程序集所有实体模型Type
var listTypes = GlobalAssemblies.GetTypes();
foreach (var type in listTypes)
{
map.ToList().ForEach(aMap =>
{
//依赖注入接口
var interfaceDependency = aMap.Key;
if (interfaceDependency.IsAssignableFrom(type) && interfaceDependency != type && !type.IsAbstract && type.IsClass)
{
//注入实现
Console.WriteLine("注入实现:" + type.FullName + ", " + aMap.Value.ToString());
services.Add(new ServiceDescriptor(type, type, aMap.Value));
//获取当前类的所有接口
var interfaces = listTypes.Where(x => x.IsInterface && x.IsAssignableFrom(type) && x != interfaceDependency).ToList();
//有接口,注入接口
if (interfaces.Count > 0)
{
interfaces.ForEach(@inteface =>
{
Console.WriteLine("注入接口:" + type.FullName + "," + @inteface.FullName + ", " + aMap.Value.ToString());
services.Add(new ServiceDescriptor(@inteface, type, aMap.Value));
});
}
}
});
};
return services;
}
}
GlobalAssemblies 全局静态类
加载程序集Assembly。
作用:
- 用于初始化CSFramework.EF组件(注册实体模型)
- 用于获取所有接口和类,依赖注入服务
C#
public static class GlobalAssemblies
{
/// <summary>
/// 加载程序集Assembly。
/// 作用:1.用于初始化CSFramework.EF组件(注册实体模型)
/// 2.用于获取所有接口和类,依赖注入服务
/// </summary>
/// <param name="hostBuilder"></param>
/// <returns></returns>
public static void LoadAssemblies()
{
//加载以下程序集(包含所有实体模型、自定义服务的程序集)
GlobalAssemblies.Assemblies = new List<System.Reflection.Assembly>
{
//如:CSFramework.LicenseServerCore.dll
System.Reflection.Assembly.Load("CSFramework.LicenseServerCore"),
System.Reflection.Assembly.Load("CSFramework.Models"),
};
}
/// <summary>
/// WebApi框架所有程序集
/// </summary>
public static List<System.Reflection.Assembly> Assemblies { get; set; }
/// <summary>
/// WebApi框架所有类型Types
/// </summary>
public static List<System.Type> GetTypes()
{
return Assemblies.SelectMany(m => m.GetExportedTypes()).ToList();
}
}
测试
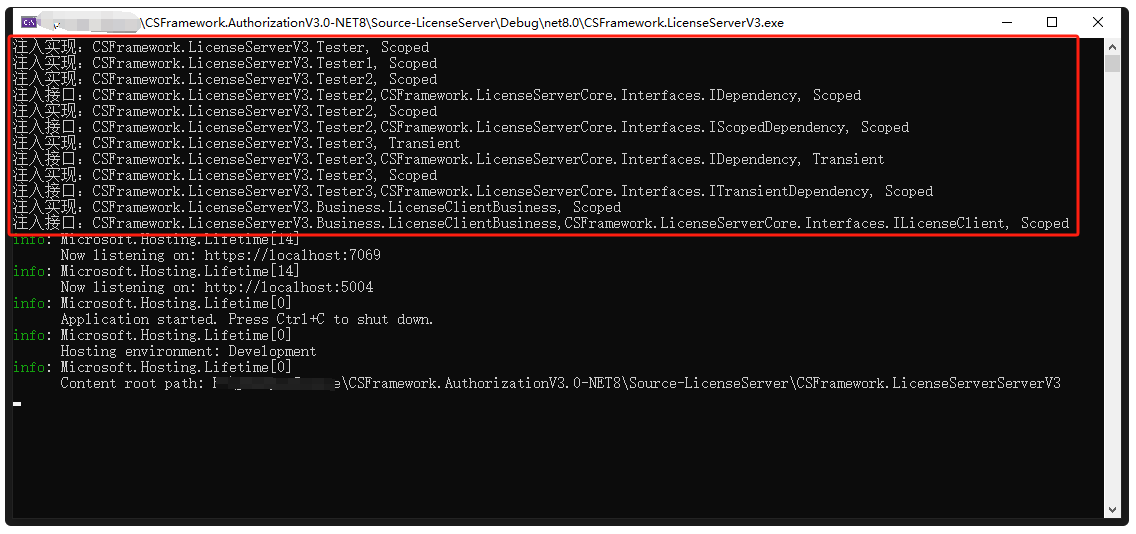