欢迎参观我的个人博客:无敌牛 -- 技术/著作/典籍/分享等
介绍
golang有很多日志包,通过设置和修改都能实现日志轮转和自定义日志格式。例如:log、zap、golog、slog、log4go
等等。
本次给出的示例使用 logrus 和 lumberjack 两个库实现日志轮转和自定义日志格式。
代码
总共涉及到4个文件:
- go.mod 模块命名;
- tlog.go 包含主函数,用来测试;
- tlog1.go 实现了单例模式返回日志对象;
- tlog2.go 用来测试在别的代码文件中的使用。

go.mod
module tlogrus
tlog.go
package main
import ("time")
func main() {
// 获取日志记录器的单例实例
log := GetLogger("")
// 使用日志记录器记录不同级别的日志
log.Debug("This is a debug message.")
log.Info("This is an info message.")
log.Warn("This is a warning message.")
time.Sleep(time.Second)
log.Error("This is an error message.")
Test()
// 下边这行输出将导致程序退出
log.Fatal("This is a fatal message.")
}
tlog1.go
package main
import (
"os"
"bytes"
"runtime"
"fmt"
"strings"
"time"
"path/filepath"
"github.com/sirupsen/logrus"
"github.com/natefinch/lumberjack"
)
// Logger 单例模式的 logger 结构体
var logger *logrus.Logger = nil
// 自定义格式化结构
type CustomFormatter struct{}
// 自定义格式化结构成员函数,用于格式化日志输出的内容
func (f *CustomFormatter) Format(entry * logrus.Entry)([]byte, error) {
// 申请日志缓冲空间
b := &bytes.Buffer{}
// 1、初始化日期输出格式
timestamp := entry.Time.Format(time.DateTime)
// 2、日志级别格式化
level := strings.ToUpper(entry.Level.String())
fmt.Fprintf(b, "%s %s ", timestamp, level)
// 3、获取进程号
fmt.Fprintf(b, "pid=%d ",os.Getpid())
// 4、获取运行时程序调用的相关信息 调用文件 和 所在行号
_, file, line, ok := runtime.Caller(6)
if !ok {
// 没获取到,给出默认值
file = "???"
line = 0
}
fmt.Fprintf(b, "%s:%d : ", filepath.Base(file), line)
// 5、获取调用函数信息
if pc, ok := entry.Data["caller"]; ok {
funcName := runtime.FuncForPC(pc.(uintptr)).Name()
fmt.Fprintf(b, "%s ", funcName )
}
fmt.Fprintf(b, "%s\n", entry.Message)
return b.Bytes(), nil
}
// GetLogger 创建或返回日志记录器的唯一实例
func GetLogger(logFileName string) *logrus.Logger {
if logger != nil {
return logger
}
// 1、创建 logger 对象
logger = logrus.New()
// 2、使用 lumberjack 进行日志轮转, 并添加到 logrus.Out
logger.Out = &lumberjack.Logger{
Filename: func (logFileName string) string { if logFileName == "" { return "logs/tlogrus.log" ; } else { return logFileName ;} } (logFileName) ,
MaxSize: 1, // MB
MaxBackups: 3,
MaxAge: 28, // days
Compress: true,
}
// 3、设置日志格式为 自定义的格式
logger.SetFormatter(&CustomFormatter{})
// 4、设置日志级别 Debug
logger.SetLevel(logrus.DebugLevel)
return logger
}
tlog2.go
package main
import ("time")
func Test() {
llog := GetLogger("xxxx.log")
for {
llog.Debug("This is a debug message.=====")
llog.Info("This is an info message.")
llog.Warn("This is a warning message.")
llog.Error("This is an error message.")
time.Sleep(time.Microsecond)
}
}
测试
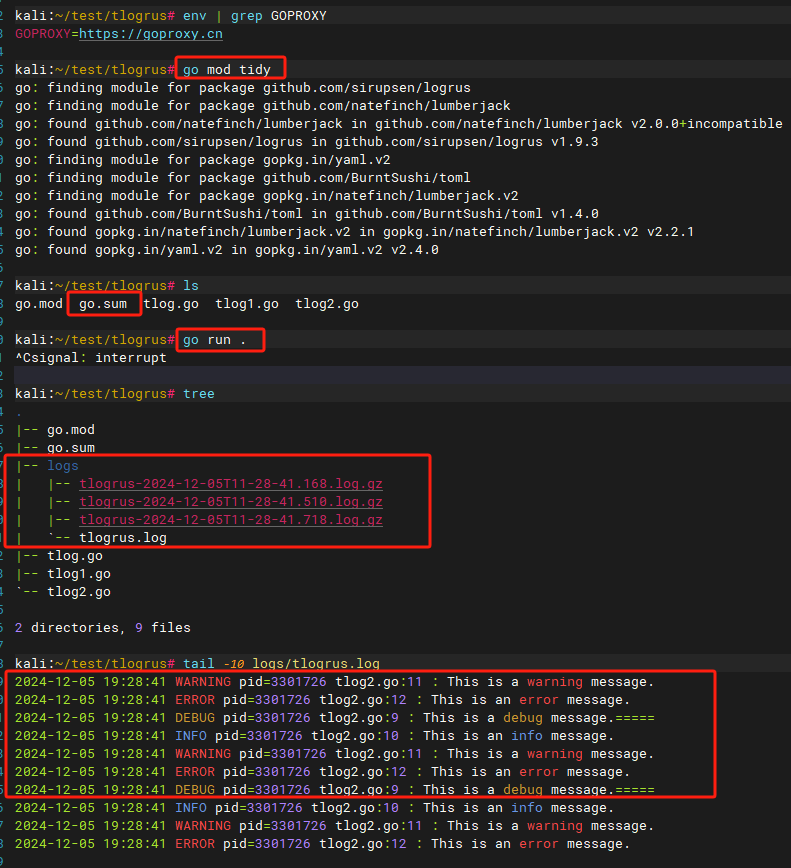