场景描述:
在父元素 div 内,包含一个子元素 div 和父元素的伪元素 ::after,伪元素需显示在父元素下方,而子元素仅需展示部分内容。因此,需实现对子元素 div 进行溢出隐藏,同时确保父元素的伪元素不受影响。有点抽象,举个 🌰。
HTML 结构:
html
<div class="list-item">
<div class="clipped"></div>
</div>
CSS 样式:
css
.list-item {
position: relative;
width: 200px;
height: 200px;
border: 1px solid #ff788e;
border-radius: 5px;
}
.clipped {
transform: rotate(45deg);
position: absolute;
top: -15px;
right: -15px;
z-index: 1;
border-radius: 5px;
width: 30px;
height: 30px;
background-color: #ff788e;
}
.list-item::after {
content: '我是伪元素';
position: absolute;
bottom: -40px;
left: 50%;
transform: translateX(-50%);
background-color: #ffc4c4;
z-index: 2;
padding: 4px 8px;
border-radius: 5px;
color: #ff5e79;
}
展示为:
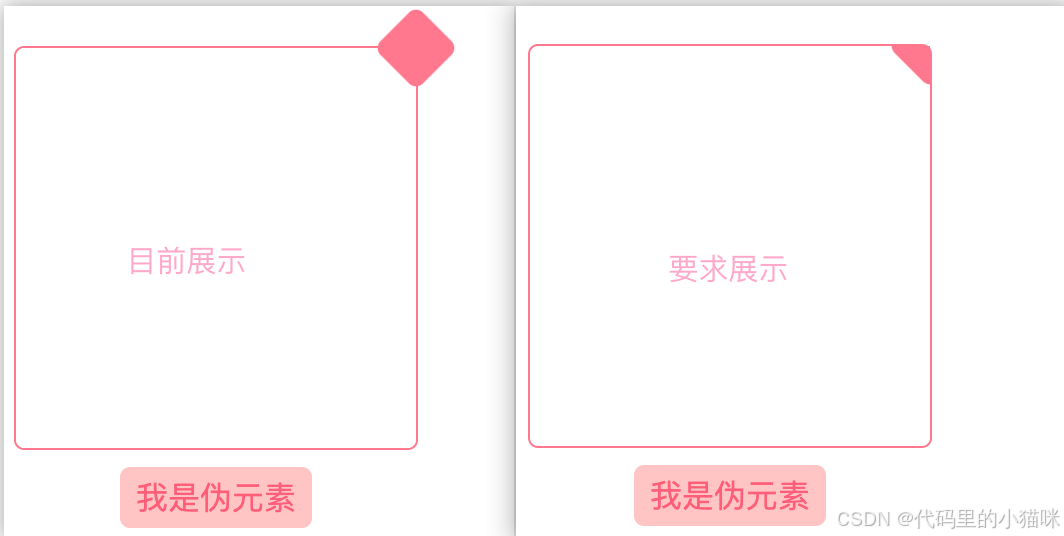
如何实现呢?
第一反应肯定是添加属性 overflow: hidden,没错,那给谁添加呢?给最外层 div 也就是父元素添加?试试看!
css
.list-item {
overflow: hidden;
position: relative;
width: 200px;
height: 200px;
border: 1px solid #ff788e;
border-radius: 5px;
}
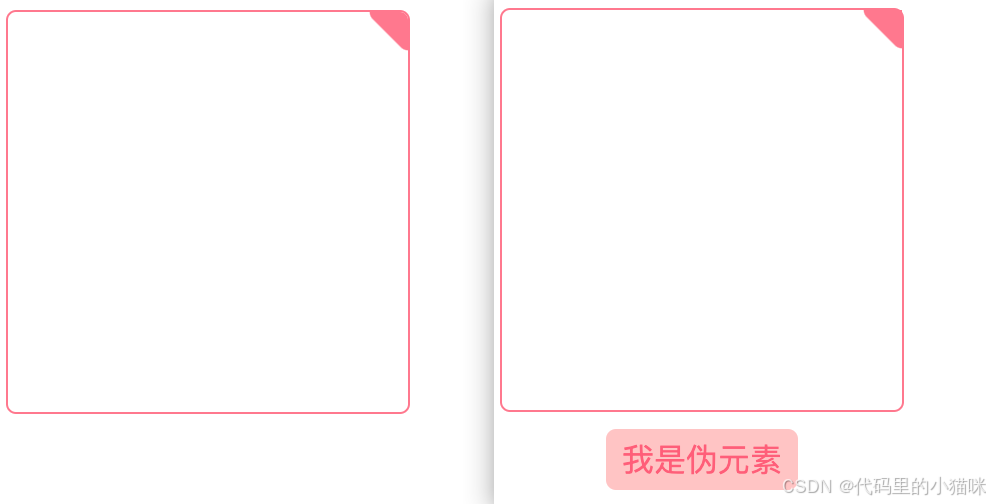
显然,标记问题解决,但是伪元素被隐藏,因为它也超出父元素的区域,那应该怎么办呢?
那就改变 HTML 结构,在需要隐藏的子元素外层嵌套一层 div,然后溢出隐藏,父元素保持可见。
HTML 结构:
html
<div class="list-item">
<div class="clip-wrapper">
<div class="clipped"></div>
</div>
</div>
CSS 样式:
css
.list-item {
position: relative;
width: 200px;
height: 200px;
border: 1px solid #ff788e;
border-radius: 5px;
}
.clip-wrapper {
overflow: hidden;
position: absolute;
top: 0;
right: 0;
width: 30px;
height: 30px;
}
.clipped {
transform: rotate(45deg);
position: absolute;
top: -15px;
right: -15px;
z-index: 1;
border-radius: 5px;
width: 30px;
height: 30px;
background-color: #ff788e;
}
.list-item::after {
content: '我是伪元素';
position: absolute;
bottom: -40px;
left: 50%;
transform: translateX(-50%);
background-color: #ffc4c4;
z-index: 2;
padding: 4px 8px;
border-radius: 5px;
color: #ff5e79;
}
OK,完美解决。
这是一个静态场景,但如果是动态组件场景,比如 Upload 组件本身呢,我们不能直接修改 HTML 结构,只能通过动态方式来处理样式和结构的调整。
举个 🌰,我需要在 Upload 组件上传后,下面添加文本「官网图片」。
目前展示为:
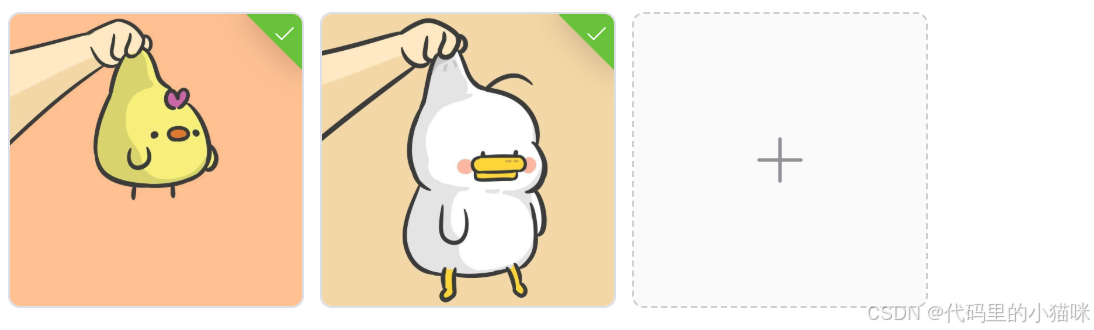
要求:
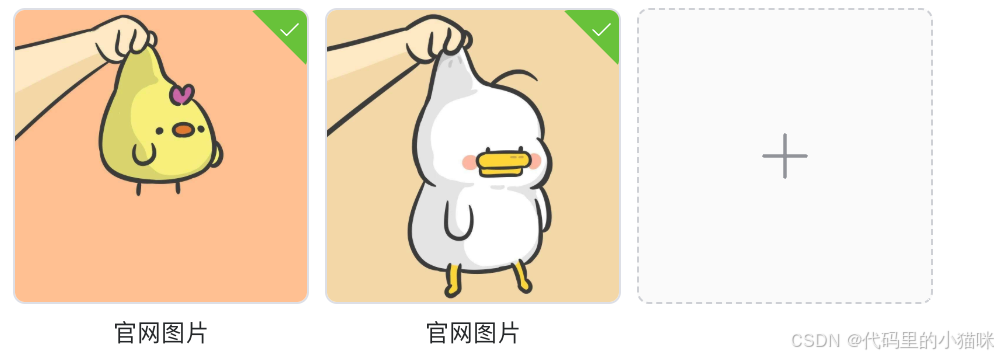
看完上面静态实现内容后,是不是感觉非常熟悉:
每一个图片都对应一个 li 元素,需要在 li 元素上添加伪元素"官网图片",但这样会导致右上角绿色的上传成功标记溢出。如图所示:
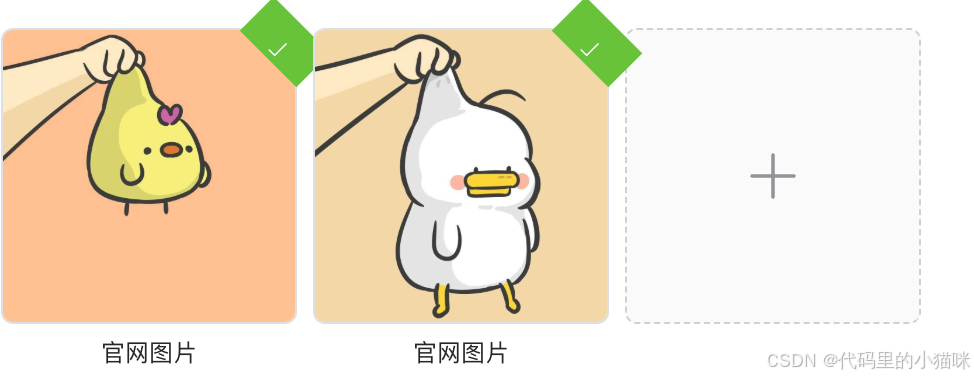
看一下元素结构,按照静态的方法书写。
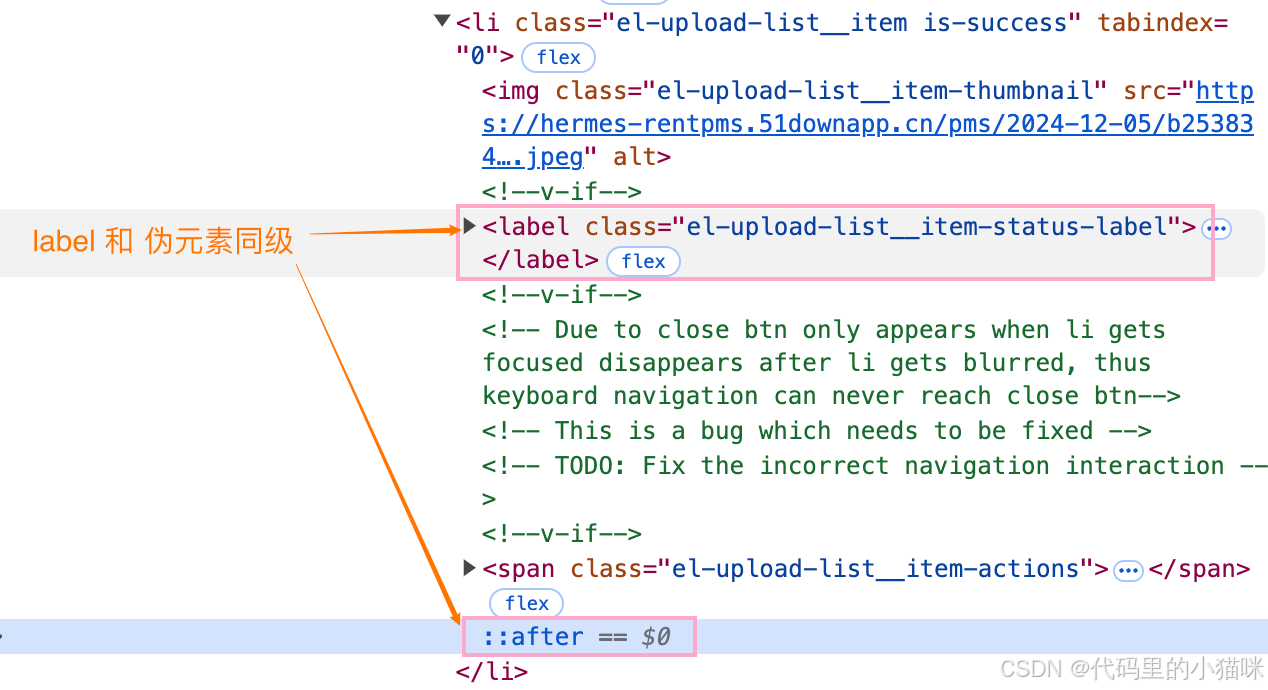
一模一样的方法,只是将直接改变 HTML 结构变成了动态修改。
核心代码:
javascript
/** 让溢出的✅显示在图片上 */
const renderSpecialUpload = async (hasAfter?: boolean, file?: { url: string }) => {
await nextTick(); // 等待 DOM 渲染完成
// 获取每个 li 元素
const uploadContainer = uploadRef.value.$el as HTMLElement;
if (!uploadContainer) return;
const uploadItems = uploadContainer.querySelectorAll<HTMLLIElement>('.el-upload-list__item.is-success');
uploadItems.forEach((item) => {
// 上传图片不添加伪元素内容
if (hasAfter && file && file.url) {
const img = item.querySelector('img');
if (img && img.src === file.url) {
// 动态移除伪元素样式
item.style.setProperty('--after-content', 'none');
}
}
const label = item.querySelector('.el-upload-list__item-status-label');
// 检查是否已经有外层容器,避免重复包裹
if (label && label.parentElement && !label.parentElement.classList.contains('clip-wrapper')) {
const wrapper = document.createElement('div');
wrapper.className = 'clip-wrapper';
wrapper.style.overflow = 'hidden';
// 将 label 包裹在新创建的 wrapper 中
label.parentNode?.replaceChild(wrapper, label);
wrapper.appendChild(label);
}
});
};
为什么多加了一个处理 img 的方法呢?为了实现新上传的图片不包含伪元素提示。
详细内容见:动态添加or删除「伪元素」-CSDN博客
最终展示为:
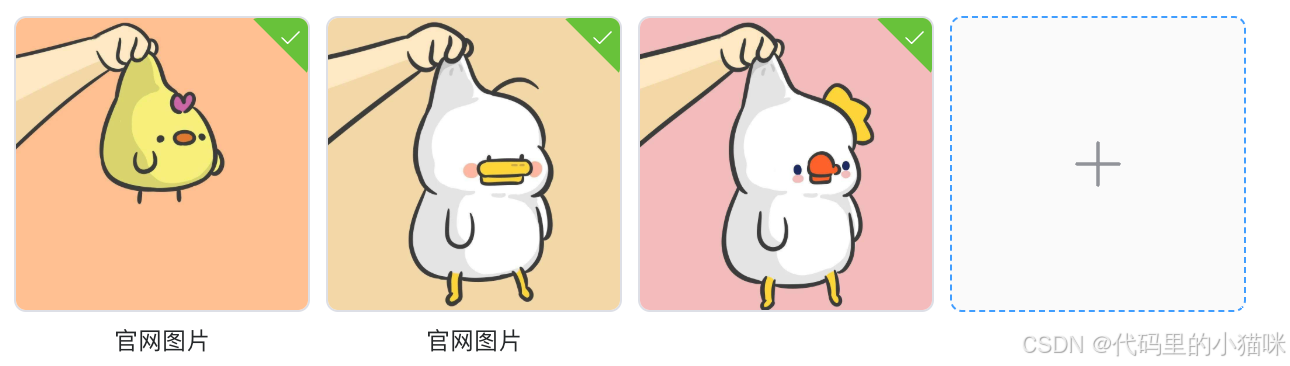
完美解决问题,┏(^0^)┛。